In C++, the size of a dynamic array can be obtained by dividing the total allocated memory by the size of its element type using the `sizeof` operator, as shown in the following code snippet:
#include <iostream>
int main() {
int* dynamicArray = new int[5]; // Creating a dynamic array of size 5
std::cout << "Size of dynamic array: " << sizeof(dynamicArray) / sizeof(dynamicArray[0]) << std::endl; // Output the size
delete[] dynamicArray; // Don't forget to free allocated memory
return 0;
}
Understanding Dynamic Arrays in C++
What is a Dynamic Array?
A dynamic array is an array that can change in size during runtime. Unlike static arrays, which have a fixed size determined at compile time, dynamic arrays offer flexibility, allowing you to allocate memory based on your program's needs. This makes them particularly useful when dealing with large or unknown amounts of data.
Dynamic arrays are stored in the heap, a region of memory that can be dynamically allocated. This method contrasts with static arrays, which are stored in the stack. The main advantages of using dynamic arrays include:
- Flexibility: You can easily allocate and deallocate memory as needed.
- Efficient Memory Use: Allowing for memory to only be used when necessary.
How to Create a Dynamic Array in C++
Creating a dynamic array in C++ requires the use of the `new` operator. Here's how you can do it:
int* dynamicArray = new int[10];
In this example, a dynamic array of integers with a size of 10 is created. It's essential to remember that, unlike static arrays, you must initialize and handle the memory management of dynamic arrays.

Measuring the Size of Dynamic Arrays
The sizeof Operator
C++ provides the `sizeof` operator to determine the size of a variable or type in bytes. However, it's crucial to understand that using `sizeof` directly on a dynamic array does not yield the number of elements in the array:
int* dynamicArray = new int[10];
std::cout << "Size of dynamic array: " << sizeof(dynamicArray) << std::endl; // This will not return the size of the array
In this case, `sizeof(dynamicArray)` returns the size of the pointer itself, not the allocated memory. As such, relying on `sizeof` for dynamic arrays is not effective or accurate.
Understanding Memory Allocation
Dynamic arrays are allocated in the heap, which is a form of memory that supports dynamic memory allocation. The significance of this lies in how memory is handled.
When you create a dynamic array, the operating system allocates a block of memory on the heap, and you get a pointer to that block. Understanding how memory is laid out helps in understanding why tracking the size manually is often necessary.
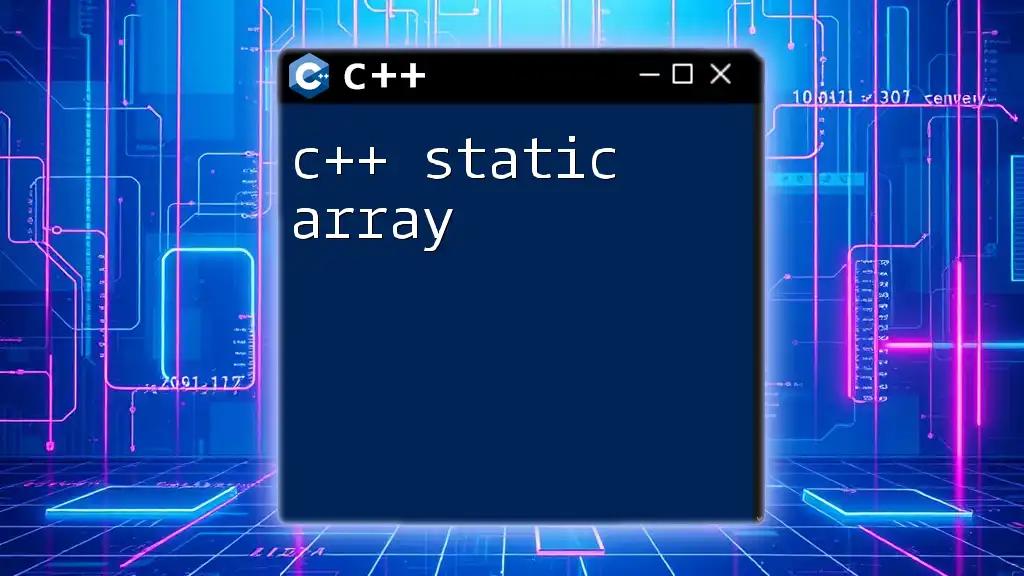
Finding the Size of a Dynamic Array
Manual Tracking
Since dynamic arrays do not provide inherent size information, tracking the size manually is a common practice. This approach involves maintaining a separate variable to store the array's size, ensuring you can access it whenever you need:
int size = 10; // Manual size tracking
int* dynamicArray = new int[size];
In this code snippet, `size` keeps track of the number of elements allocated for `dynamicArray`. It's crucial to ensure that this variable is updated if the array is resized or changed.
Using Wrapper Classes
An effective alternative to raw dynamic arrays is the utilization of C++ Standard Library containers, such as `std::vector`. The `std::vector` class internally manages the memory, and it provides a convenient way to access the size of the dynamic array:
#include <vector>
std::vector<int> vec(10);
std::cout << "Size of dynamic vector: " << vec.size() << std::endl;
By using a `std::vector`, there’s no need for manual size tracking, as the class handles resizing and memory management behind the scenes, reducing the risk of memory leaks and improving code readability.

Memory Management in C++
Allocating and Deallocating Memory
Proper memory management is crucial when dealing with dynamic arrays in C++. When you allocate memory using `new`, it's your responsibility to release it with `delete`. Failing to do this can lead to memory leaks. The proper syntax to deallocate memory for a dynamic array is as follows:
delete[] dynamicArray; // Release allocated memory for dynamic array
By deallocating memory, you return it to the system, allowing it to be reused for future allocations.
Common Memory Management Mistakes
Many developers encounter pitfalls related to memory management, including:
- Memory Leaks: This occurs when dynamically allocated memory is not freed, causing the application to consume increasing amounts of memory over time.
- Dangling Pointers: These refer to pointers that point to memory that has already been deallocated.
For instance, consider the following code snippet that exemplifies a memory leak:
int* dynamicArray = new int[10];
// Some operations
// Missing delete[] statement here
In this situation, `dynamicArray` will allocate memory, but if the `delete[]` statement is not executed, the allocated memory is lost.
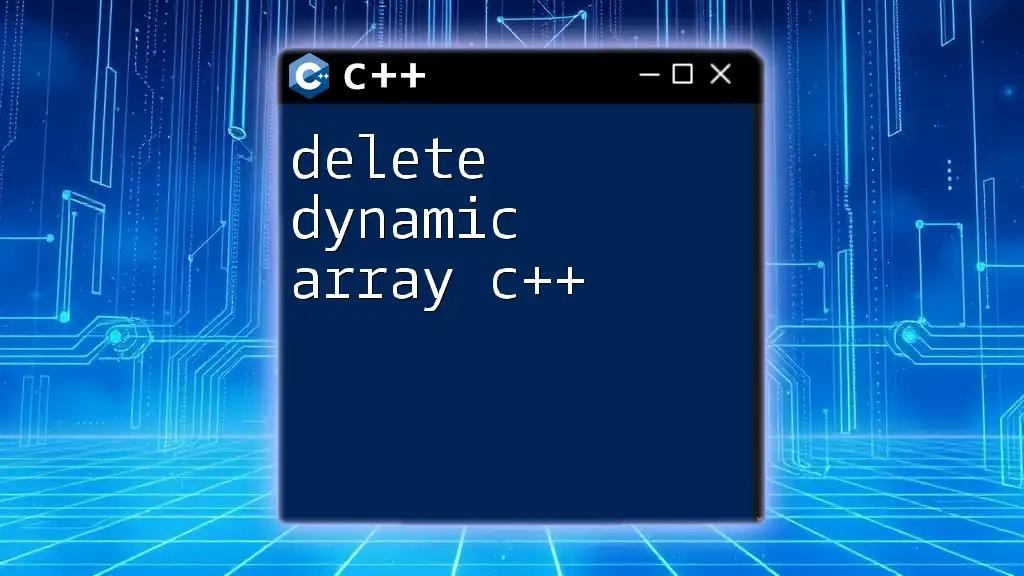
Conclusion
In summary, understanding the C++ size of dynamic array is essential for efficient memory management. Dynamic arrays offer flexibility compared to static arrays, but they also come with the responsibility of manual size tracking and memory management. By utilizing techniques such as manual tracking and C++ Standard Library wrappers like `std::vector`, developers can mitigate common pitfalls and write robust, efficient code.
Continual practice and exploration of C++ memory management concepts will deepen your understanding, preparing you for more advanced topics in the future.
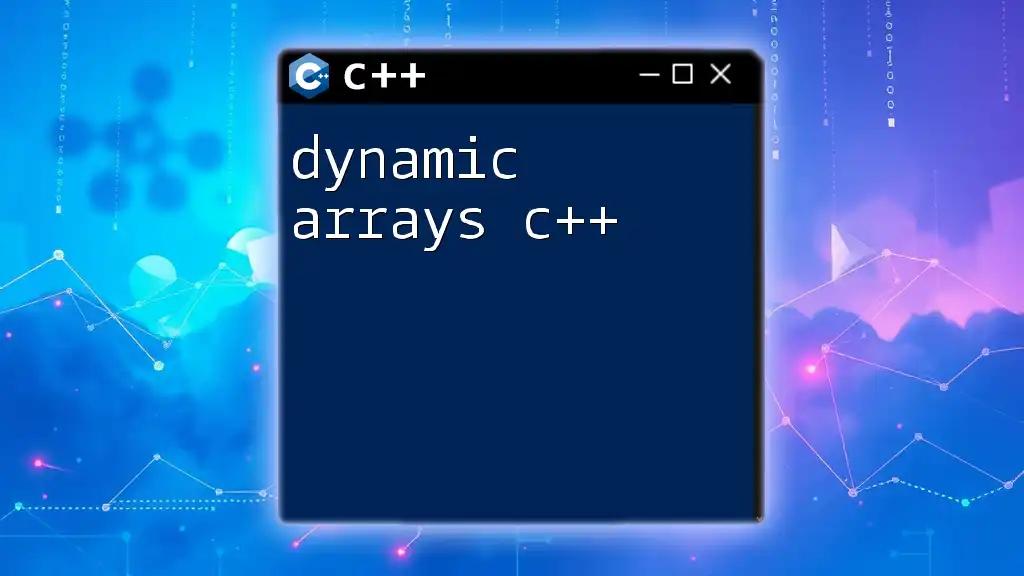
Additional Resources
For further learning, consider exploring recommended books, online courses, and documentation on topics such as memory management, dynamic arrays, and the C++ Standard Library. Engaging with the community through forums can also provide valuable insights and support.

FAQs
What happens if I don’t track the size of my dynamic array?
If you neglect to track the size of your dynamic array, you may encounter issues such as out-of-bounds errors, which can lead to undefined behavior, crashes, or data corruption.
Can I resize a dynamic array?
Yes, while you can't resize a dynamic array directly, you can create a new array with the desired size, copy the elements from the old array, and then delete the old array.
When should I use a static array instead of a dynamic array?
Static arrays are more suitable when the size is known at compile time, and if the array will not grow or shrink during the program's execution. They are also simpler to use when memory management isn't a concern.