In C++, FIFO (First-In-First-Out) is typically implemented using a queue, which allows data to be added to the back and removed from the front.
Here's a simple example using the C++ Standard Library:
#include <iostream>
#include <queue>
int main() {
std::queue<int> fifoQueue;
fifoQueue.push(1);
fifoQueue.push(2);
fifoQueue.push(3);
while (!fifoQueue.empty()) {
std::cout << fifoQueue.front() << " ";
fifoQueue.pop();
}
return 0;
}
Introduction to FIFO
What is FIFO?
FIFO stands for First In, First Out, a method in concept and data structure design where the first element added to the queue will be the first one to be removed. This is akin to a line at a grocery store: the first person in line is the first to be served. FIFO is a crucial paradigmatic design followed in programming, especially when managing collections of data.
Importance of FIFO in Programming
Understanding FIFO is essential for effective data management. Using FIFO could enhance the performance of applications that need to maintain an order of execution or processing tasks. FIFO finds applications in areas such as task scheduling, data buffering in streams, and managing buffers in network protocols. Overall, it helps simplify the flow of data with predictable behavior, improving code readability and maintainability.
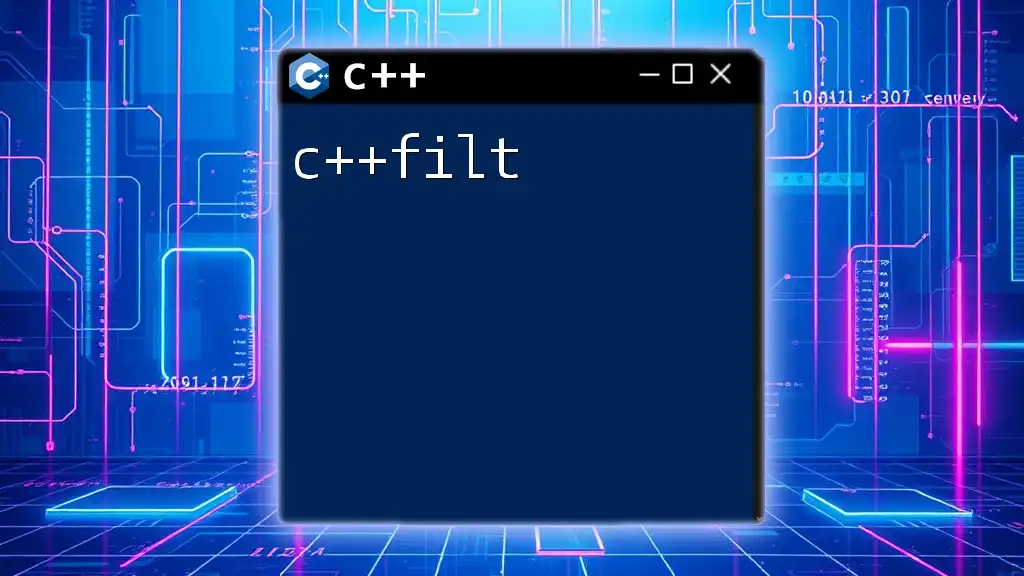
Understanding C++ Data Structures
Overview of Data Structures
In C++, different data structures help organize and store data in memory efficiently. Respectively, they can vary in size, access time, and algorithms associated with them. Understanding how these structures work is crucial for leveraging them effectively.
Queues as a Data Structure
A queue is a linear data structure that follows the FIFO principle. In a queue, elements are added to the rear and removed from the front. This model is simple yet powerful for various applications, enabling orderly processing of tasks, events, or messages in a controlled manner.
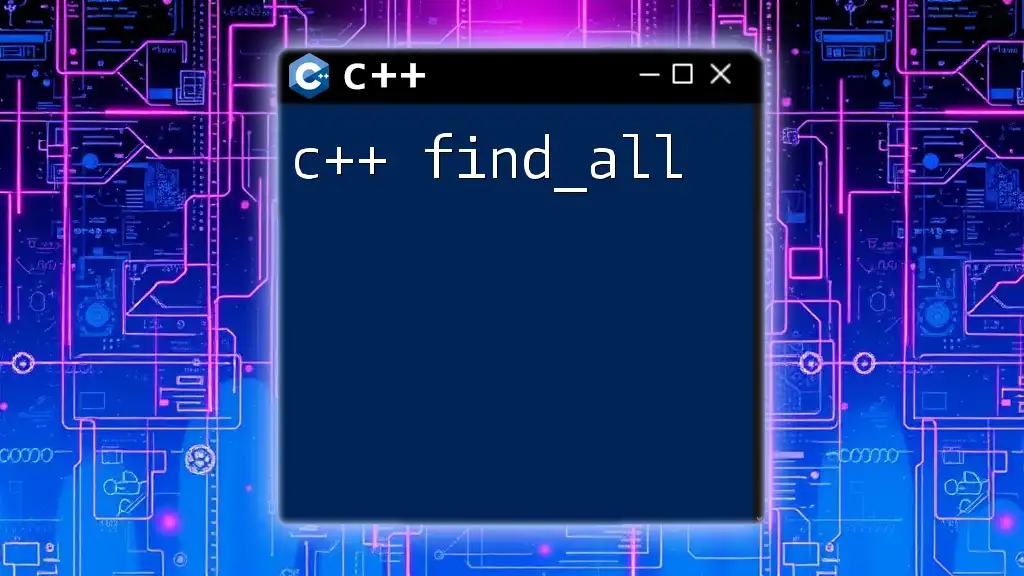
Implementing FIFO in C++
Using STL (Standard Template Library)
C++ provides powerful structures through STL, which includes template-based classes that are highly efficient. Among these, `std::queue` is dedicated to implementing the FIFO behavior.
Basic Components of FIFO Implementation
The `std::queue` class allows you to add elements to the end using the `push()` method and remove them from the front using the `pop()` method. It also provides convenient functions like `front()` to access the first element without removal and `back()` to access the last element.
Setting Up Your Environment
To get started with C++ and its FIFO implementation, ensure your development environment is set up with the necessary libraries. You typically need the `<iostream>` and `<queue>` headers for input/output operations and queue functionalities, respectively.
Here’s a sample project structure to consider:
/my_cpp_fifo_project
├── main.cpp
├── Makefile
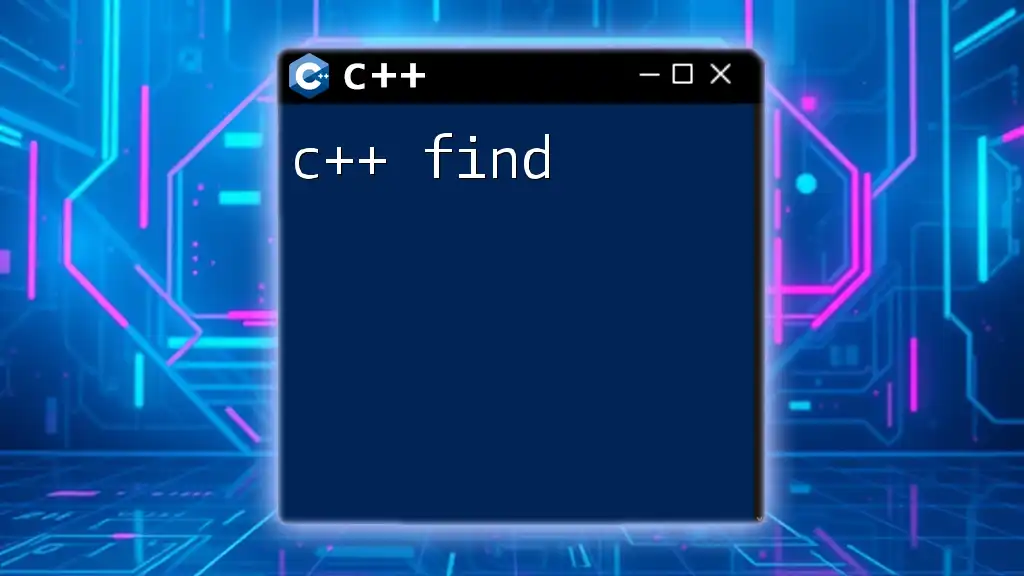
Code Snippets: Basic FIFO Implementation
Creating a Basic FIFO Queue
Here’s a simple example demonstrating the power of `std::queue` in C++:
#include <iostream>
#include <queue>
int main() {
std::queue<int> myQueue;
// Adding elements to the queue
myQueue.push(10);
myQueue.push(20);
myQueue.push(30);
// Displaying the front element
std::cout << "Front element: " << myQueue.front() << std::endl;
// Removing the front element
myQueue.pop();
std::cout << "Front element after pop: " << myQueue.front() << std::endl;
return 0;
}
In this code, we first create a queue of integers. We add three integers to the queue using `push()` and display the front element using `front()`. When we call `pop()`, the first element (10) is removed, and the new front (20) is shown. This straightforward functionality exemplifies how easy it is to manage FIFO with C++.
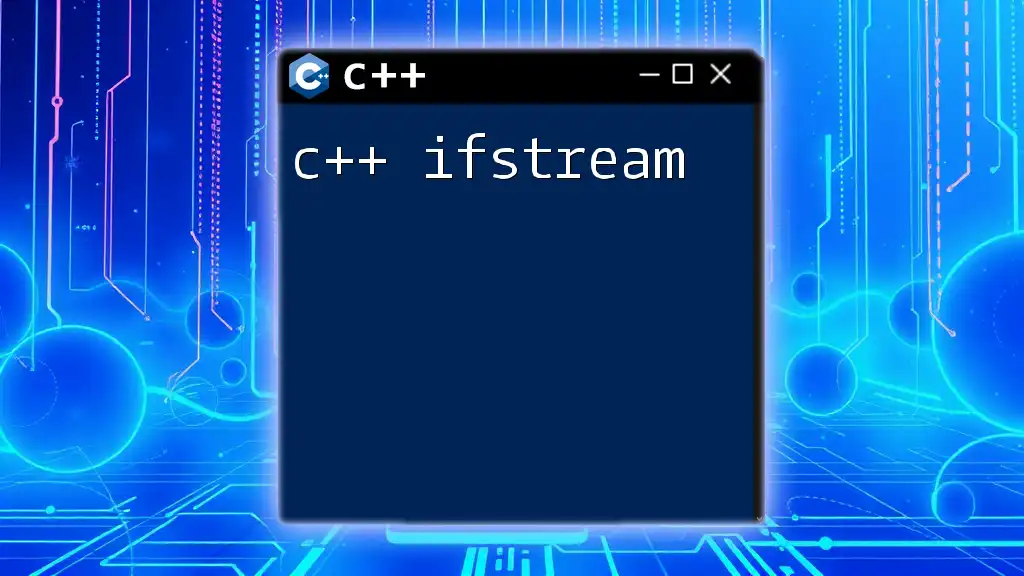
Advanced FIFO Concepts
Priority Queues versus Standard Queues
While `std::queue` implements a strict FIFO order, a priority queue (implemented with `std::priority_queue`) organizes elements based on their priority instead of their order of insertion. The element with the highest priority is always served first, regardless of when it was added.
Here’s a sample code for implementing a priority queue:
#include <iostream>
#include <queue>
int main() {
std::priority_queue<int> myPriorityQueue;
myPriorityQueue.push(10);
myPriorityQueue.push(20);
myPriorityQueue.push(15);
// The top element will be the largest number
std::cout << "Top element: " << myPriorityQueue.top() << std::endl;
return 0;
}
This code demonstrates that by using a priority queue, we get access to the highest element (20) irrespective of when it was added. Understanding these differences can help you choose the right queue depending on your application requirements.
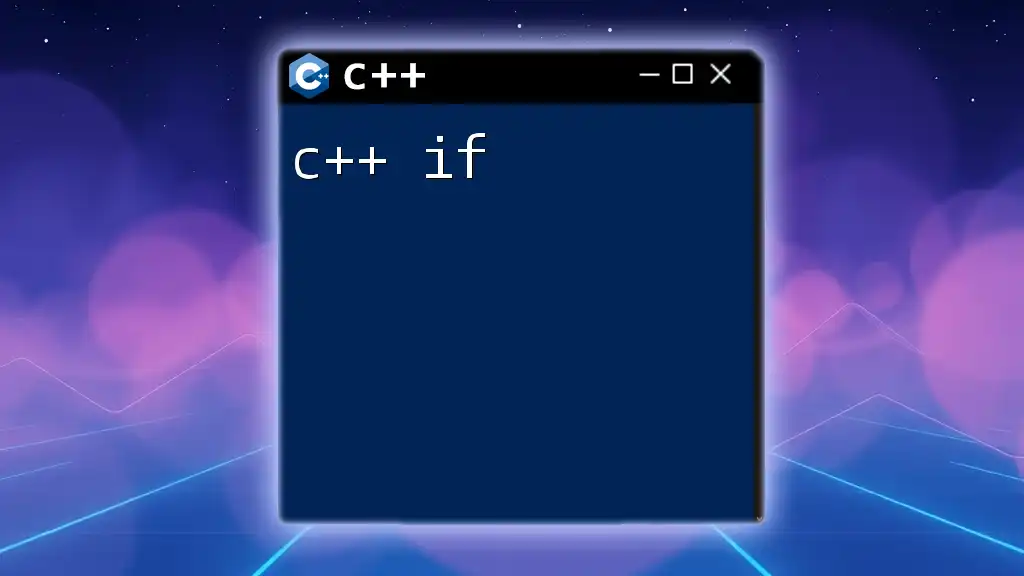
FIFO Applications in C++
Use Cases in Real-World Applications
FIFO structures are widely used in many real-world systems. They are ideal in:
- Event scheduling systems: Managing a series of events where order matters.
- Task management in operating systems: Queueing processes based on arrival time.
- Data buffering in network programming: Temporarily storing packets while ensuring they are processed in the right order.
Example: Implementing a Simple Task Scheduler
You can leverage FIFO to create an effective task scheduler. Below is a sample implementation:
#include <iostream>
#include <queue>
#include <string>
class TaskScheduler {
public:
void addTask(std::string task) {
tasks.push(task);
}
void executeTask() {
if (!tasks.empty()) {
std::cout << "Executing task: " << tasks.front() << std::endl;
tasks.pop();
} else {
std::cout << "No tasks to execute!" << std::endl;
}
}
private:
std::queue<std::string> tasks;
};
int main() {
TaskScheduler scheduler;
scheduler.addTask("Task 1");
scheduler.addTask("Task 2");
scheduler.executeTask(); // Executes "Task 1"
scheduler.executeTask(); // Executes "Task 2"
scheduler.executeTask(); // No tasks to execute!
return 0;
}
This implementation allows you to add tasks to the scheduler, ensuring they are executed in the order they were received—demonstrating the efficacy of FIFO in managing tasks.
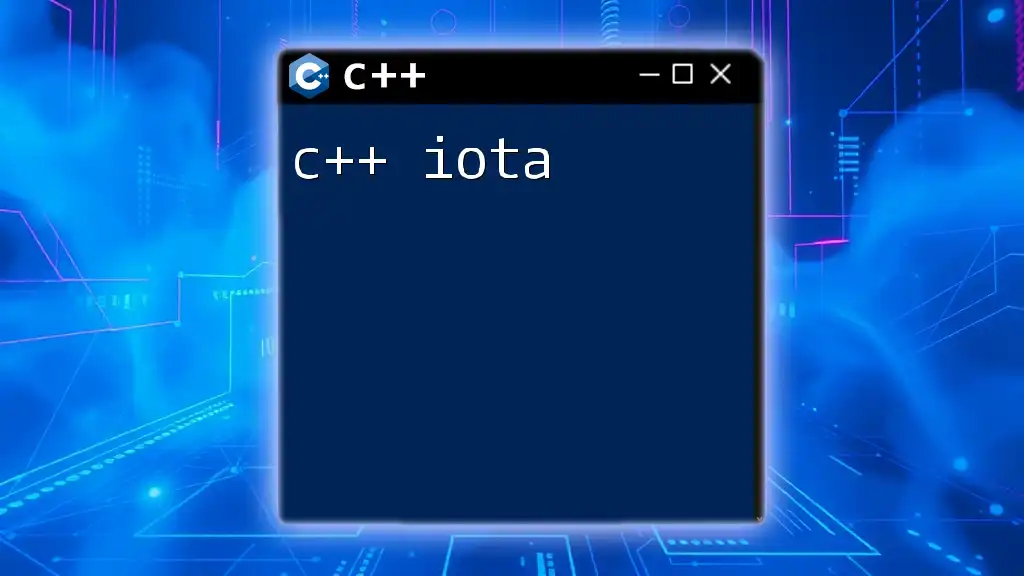
Troubleshooting Common FIFO Issues
Debugging Queue Operations
Common mistakes when using queues include attempting to access or remove an element from an empty queue, which can lead to unexpected behavior or runtime errors. Always check if the queue is empty using `.empty()` before performing operations like `front()` and `pop()`.
Performance Considerations
When implementing FIFO structures, be mindful of the potential bottlenecks. Frequent manipulation of queues, especially in a multi-threaded environment, can lead to performance issues. Using proper synchronization mechanisms like mutexes in C++ can help alleviate these concerns.
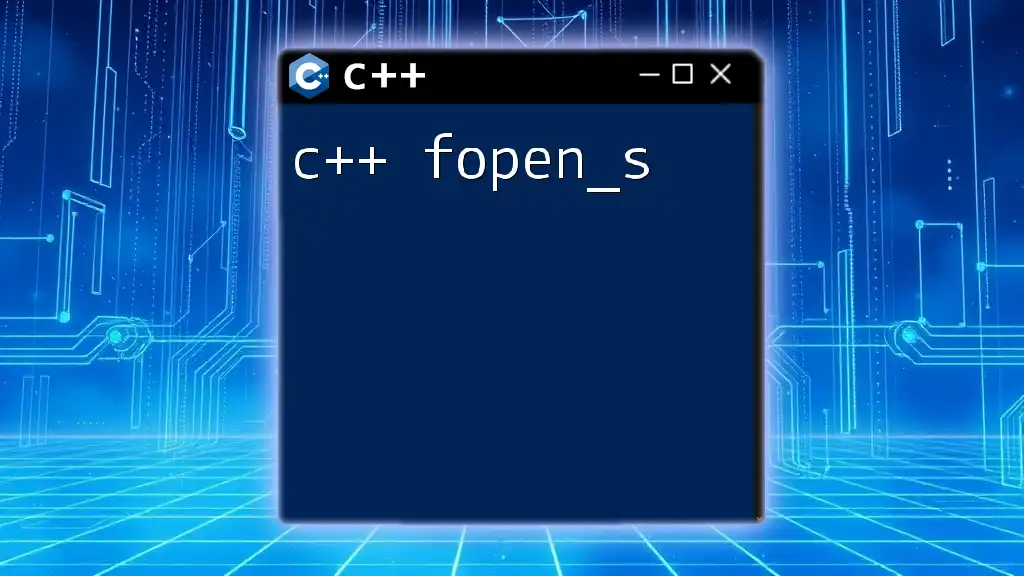
Conclusion
In summary, understanding and implementing C++ FIFO through `std::queue` provides a robust foundation for managing data effectively. With its simplicity and predictability, FIFO structures benefit numerous applications, making it essential knowledge for any C++ developer. I encourage you to experiment with FIFO concepts and tailor these methods to fit your development needs, as mastering these principles can greatly improve the quality of your code.
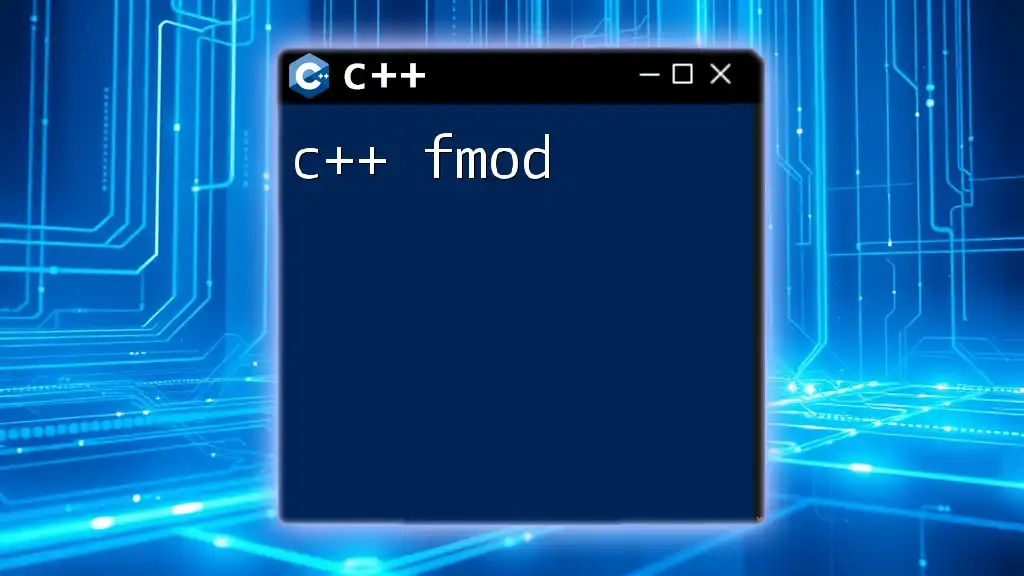
Additional Resources
To further your knowledge, consider exploring recommended books and courses dedicated to C++ and data structures. Engaging with community forums can also be invaluable for asking questions and gaining insights from fellow programmers as you delve deeper into the world of C++.