C++ programming on a Mac can be efficiently done using the Terminal with the g++ compiler to compile and run C++ programs.
// Example C++ code: Hello World program
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
To compile and run this code, save it as `hello.cpp`, then in the Terminal, use the commands:
g++ hello.cpp -o hello
./hello
Setting Up Your Mac for C++ Development
Installing Xcode
What is Xcode?
Xcode is Apple's official Integrated Development Environment (IDE) for creating apps for macOS, iOS, watchOS, and tvOS. It provides a robust platform for writing C++ code alongside Objective-C and Swift. By using Xcode, developers can leverage Apple's framework of tools for performance monitoring, debugging, and software design.
How to Download and Install Xcode
To get started with C++ development on a Mac, you first need to install Xcode. Follow these straightforward steps:
- Open the App Store on your Mac.
- Search for "Xcode."
- Click on "Get" and then "Install."
Once installed, you will have access to a powerful coding environment tailored for a variety of programming languages, including C++.
Setting Up Command Line Tools
For compiling C++ projects directly from the terminal, you will need to install command line tools. To do this, open the terminal and execute the following command:
xcode-select --install
These tools include essential commands that facilitate the building and debugging of C++ applications.
Installing Additional Compilers
GCC and Clang
While Xcode comes with Clang as the default compiler, you may want to use GCC (GNU Compiler Collection) for certain features or to maintain compatibility with projects.
How to Install GCC via Homebrew
If you haven't already, you will need to install Homebrew, a package manager for macOS. Open the terminal and enter the following command to install Homebrew:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
After successfully installing Homebrew, you can install GCC by entering:
brew install gcc
Using Clang
Clang is the default compiler for macOS and is included with Xcode. It is particularly optimized for macOS and can compile C++ files quickly. To compile a basic C++ file using Clang, use the following command in the terminal:
clang++ -o my_program my_program.cpp
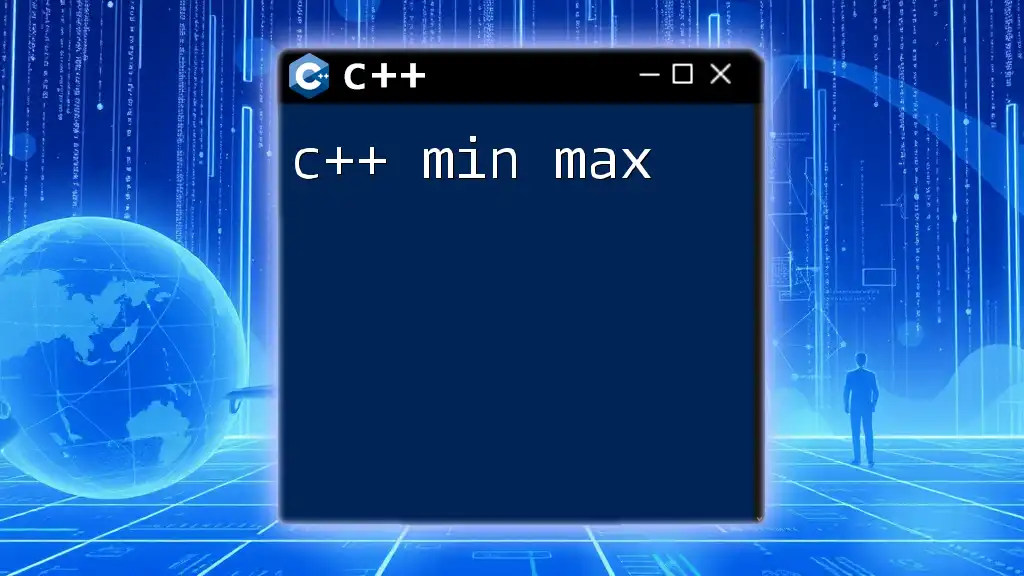
Writing Your First C++ Program on a Mac
Creating Your First C++ File
Using Text Editors
You can use a variety of text editors to write C++ code. While some developers prefer GUI IDEs like Xcode, others may find command-line editors like Nano or Vim sufficient. For a beginner-friendly experience, consider using Visual Studio Code, which offers syntax highlighting and debugging capabilities.
Basic C++ Program Structure
A fundamental understanding of C++ structure is pivotal for writing effective programs. Here's a simple "Hello, World!" example to get started:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This program includes the `<iostream>` header file, which enables input and output operations. The `main()` function is the entry point of any C++ application, where program execution begins.
Compiling and Running Your C++ Program
Using Terminal Commands
To compile and run your C++ program written in a file named `hello.cpp`, navigate to the directory containing your file and use the following compilation command:
g++ -o hello hello.cpp
Once compiled, execute your program using:
./hello
You should see "Hello, World!" displayed in the terminal.
Understanding Compilation Errors
As you begin coding, you will likely encounter compilation errors. These errors often indicate issues such as syntax errors, missing semicolons, or undeclared identifiers. Pay careful attention to the messages provided by your compiler; they usually contain the line number where the error occurred, allowing you to pinpoint the problem effectively.
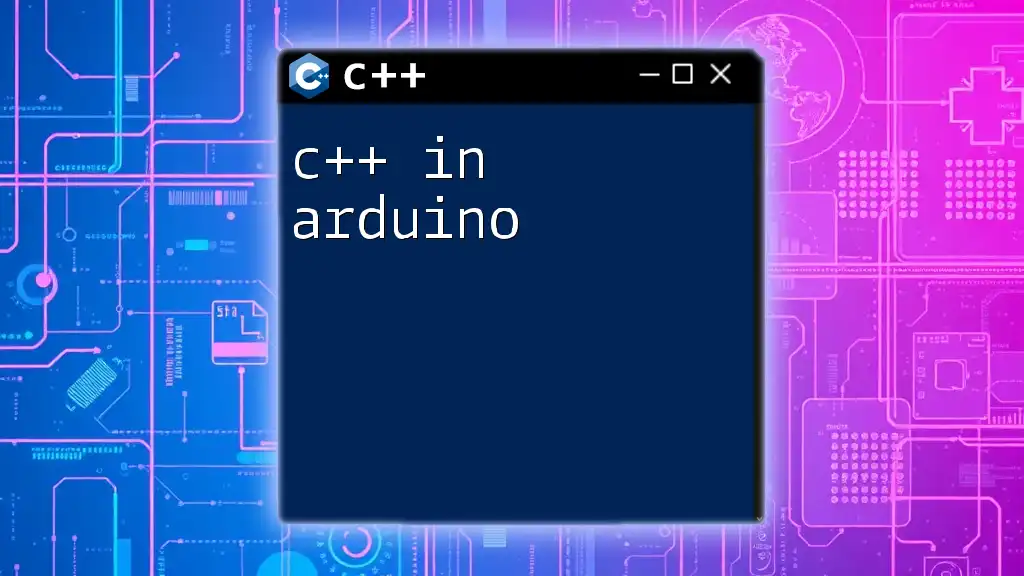
Using IDEs for C++ Development on Mac
Overview of Popular IDEs
Xcode for C++ Development
Xcode is one of the most powerful options available for C++ development on a Mac. It provides a comprehensive feature set, including integrated debugging, refactoring tools, and support for version control systems. To get started, simply create a new project, select a C++ template, and start coding!
CLion
Another highly regarded IDE is JetBrains CLion. This IDE offers superb code assistance with smart code completion, powerful navigation, and support for CMake. It streamlines the development process, enabling developers to focus on creating excellent software rather than managing configurations.
Configuring Your IDE
Setting Up Build Settings
When using Xcode, you can configure your build settings by navigating to the project file's settings. Here, you can adjust the compiler, linker, and optimization settings to suit your specific project needs.
Debugging Tools
Effective debugging is essential for successful software development. Xcode and CLion both offer built-in debugging tools that allow you to set breakpoints, inspect variable values, and step through your code to identify and fix issues swiftly.
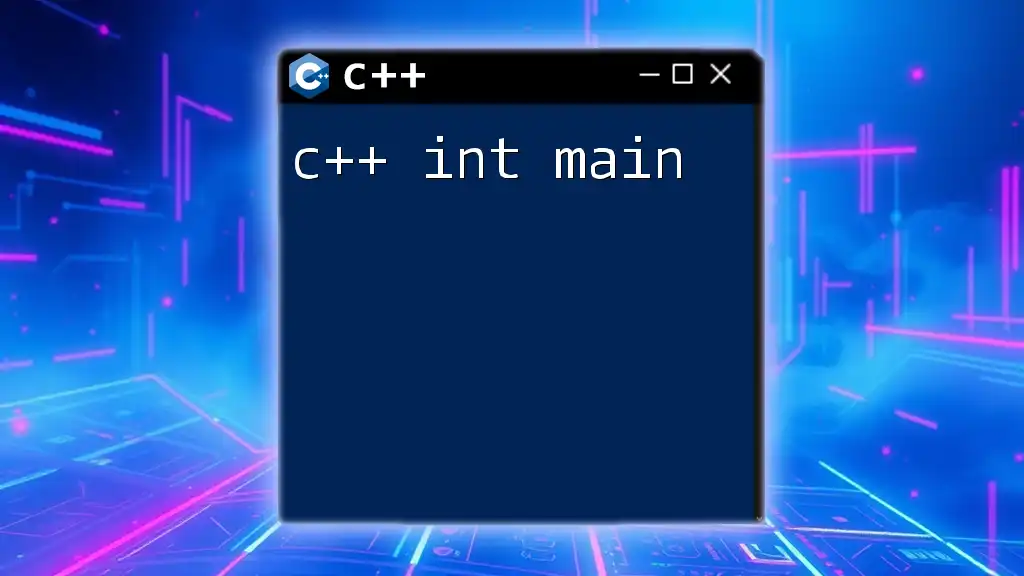
Best Practices for C++ Development on a Mac
Code Structure and Organization
Organizing Your Project Files
Maintaining a clean code structure is vital for larger projects. Using a logical naming convention for your files and folders can significantly improve project manageability. Separating header files from implementation files and using meaningful names will make it easier for you (and others) to navigate your project.
Version Control with Git
Integrating Git for C++ Projects
Implementing version control is crucial for managing changes in your C++ projects. To initialize a Git repository, navigate to your project directory in the terminal and run:
git init
You can add all project files and make your first commit using:
git add .
git commit -m "Initial commit"
Keeping your code in a version control system helps you track changes, collaborate with others, and safeguard your work.
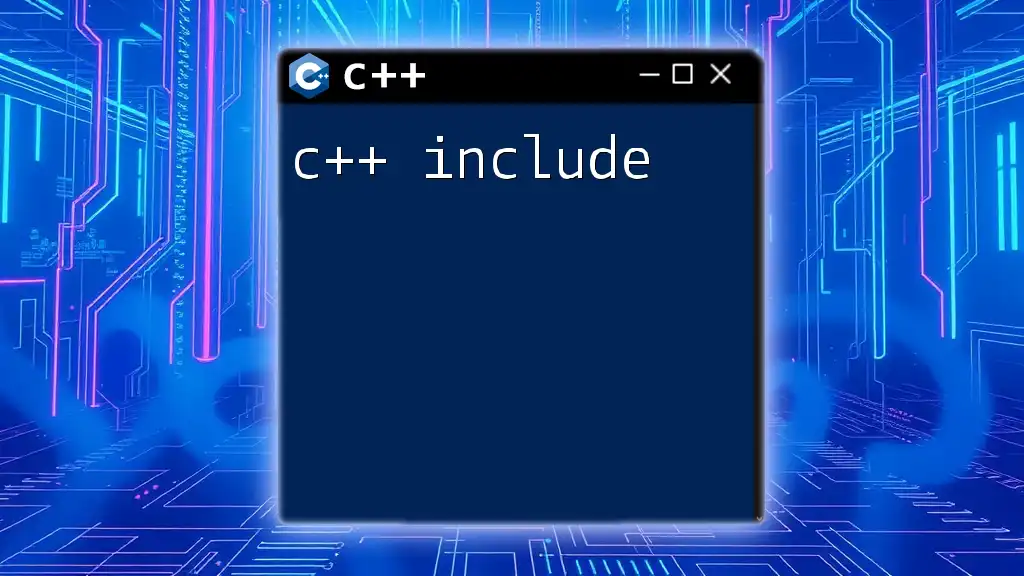
Troubleshooting C++ on Mac
Common Issues and Solutions
Compiler Errors
When developing with C++, compiler errors can be common. Some frequent errors include syntax mistakes, missing semicolons, and type mismatches. Always refer to the error messages for guidance, as they provide valuable information regarding what went wrong.
Library Linking Issues
When working with external libraries, you may encounter linking errors. To resolve this, ensure that libraries are correctly linked during the compilation process. You can specify library paths using the `-L` flag followed by the library directory and the `-l` flag for the library name. For example:
g++ -o my_app my_app.cpp -L/path/to/libs -lmylibrary
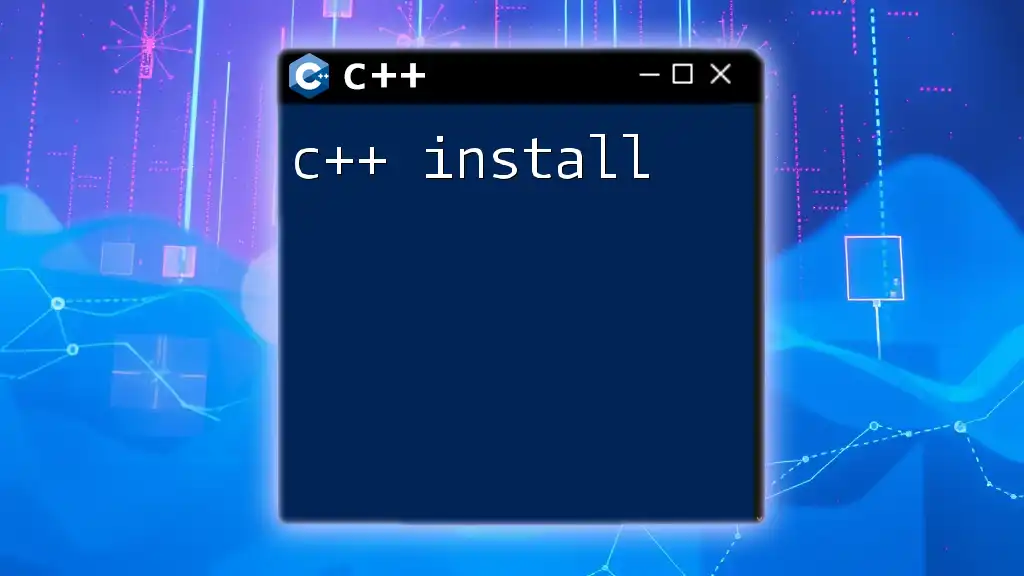
Conclusion
Recapping the essentials of developing C++ applications on a Mac, setting up your environment correctly, organizing your code, and employing effective debugging practices all contribute to a smoother development process. C++ offers significant power and flexibility, and mastering this language on a Mac can open up countless opportunities in the world of software development. Engaging with advanced topics like templates, pointers, and the Standard Template Library (STL) can help you become a proficient C++ developer, equipped to tackle complex projects.
Encouragement to Explore Further
As you progress, don’t hesitate to delve deeper into C++ and explore additional resources such as dedicated courses, community forums, and documentation. The journey of mastering C++ is filled with opportunities for growth and creativity. Happy coding!
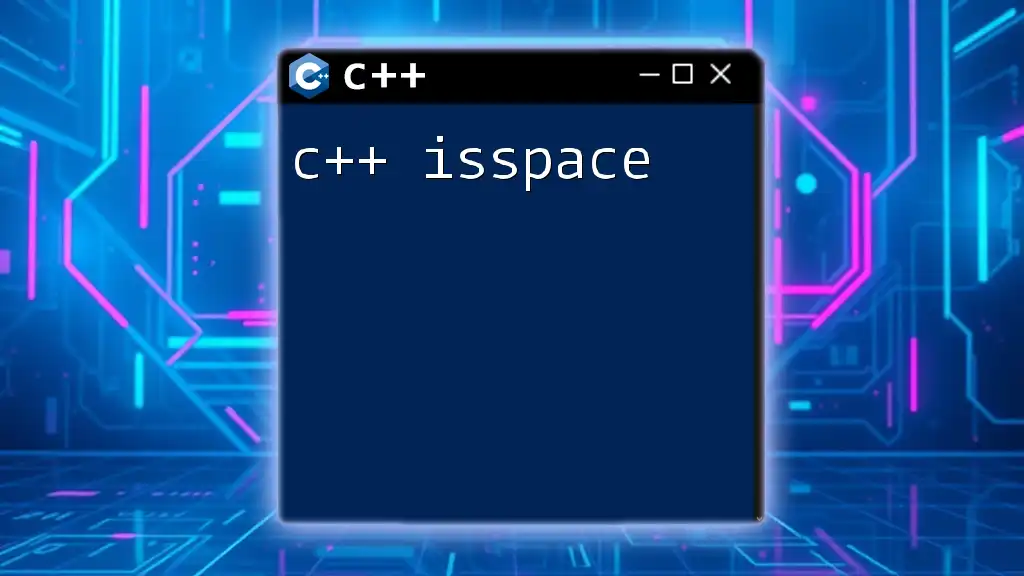
Additional Resources
Links to Documentation
For more details on C++ language features, refer to the official C++ documentation. Additionally, the Apple Developer documentation can provide insights into the usage of Xcode and command-line tools.
Books and Online Courses
Consider investing in reputable books, such as "The C++ Programming Language" by Bjarne Stroustrup, or enrolling in online platforms like Coursera and Udacity that offer comprehensive C++ courses tailored to Mac users.