The `++` operator in C++ is an increment operator that increases the value of a variable by 1, either before (`++x`) or after (`x++`) the variable's value is used in an expression.
Here’s a code snippet demonstrating both forms:
#include <iostream>
int main() {
int x = 5;
std::cout << "Using prefix increment: " << ++x << std::endl; // Outputs 6
std::cout << "Using postfix increment: " << x++ << std::endl; // Outputs 6, then x becomes 7
std::cout << "Value of x after postfix increment: " << x << std::endl; // Outputs 7
return 0;
}
Understanding the Increment Operator in C++
What is the Increment Operator?
The increment operator, denoted by `++`, is a unary operator in C++ that increases the value of a variable by one. This simple yet powerful operator plays a crucial role in numerical operations, control structures, and various algorithm implementations.
Types of Increment Operators
C++ provides two forms of the increment operator: prefix and postfix.
Prefix Increment Operator
The prefix increment operator (`++x`) increments the variable's value before it is utilized in an expression. This means that when using the prefix operator, the updated value is immediately available.
For example:
int x = 5;
int result = ++x; // x becomes 6; result is 6
In this snippet, `x` is incremented first, and then its new value is assigned to `result`. Thus, both `x` and `result` end up holding the value 6.
Postfix Increment Operator
Conversely, the postfix increment operator (`x++`) increases the variable's value after it has been used in the expression. The original value is returned before the increment takes place.
For example:
int x = 5;
int result = x++; // result is 5; x becomes 6
Here, `result` holds the initial value of `x`, which is 5 at the time of the assignment. Only after this assignment does `x` get incremented to 6.
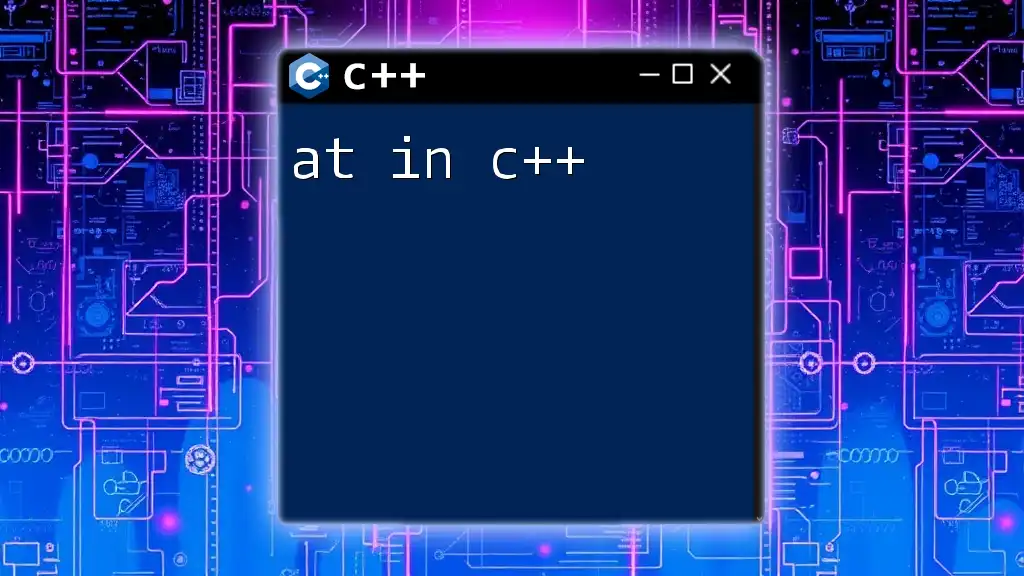
Incrementation in C++
Basic Syntax of C++ Incrementation
Using the increment operator in C++ is straightforward. The syntax simply involves placing `++` either before (prefix) or after (postfix) the variable.
It's important to remember that while both operators achieve the same end goal of increasing the variable's value, the timing of when that increment occurs in relation to other operations can significantly affect your program's logic and output.
Practical Applications of Incrementation
Incrementation is a fundamental operation in various programming scenarios, especially those involving loops or counters. Consider a situation where you want to track iterations in a loop. By using the increment operator, you can succinctly manage and update your counter, which leads to cleaner and more comprehensible code.
For example, in a countdown timer or a scoring system, incrementing a variable can help track points or time elapsed without requiring additional logic or overhead.
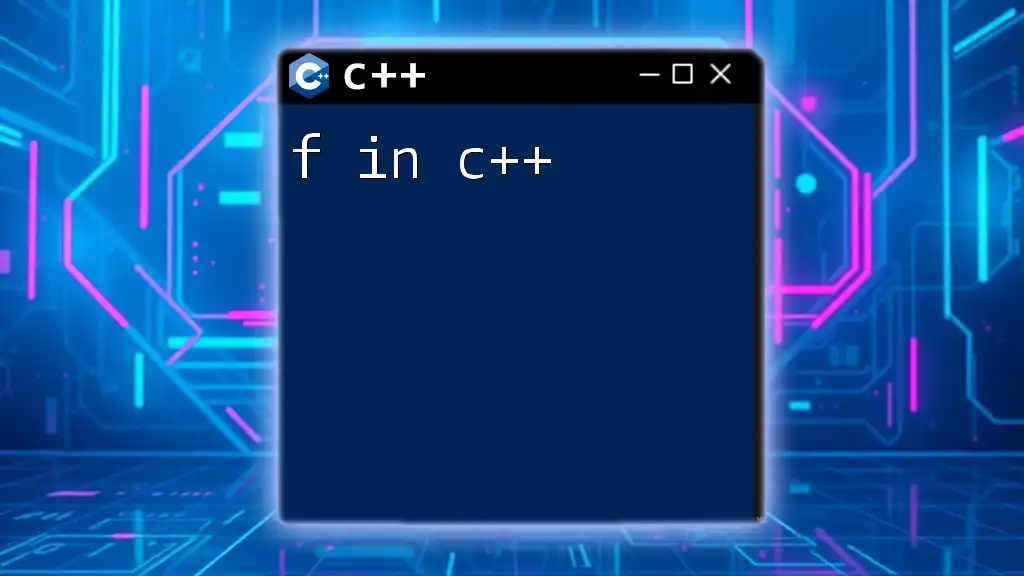
Increment and Decrement Operators in C++
The Decrement Operator
Similarly, the decrement operator (`--`) is used to decrease a variable's value by one. Understanding both the increment and decrement operators gives you powerful tools for managing variable states in your programs.
Combining Increment and Decrement
There may be scenarios in which you find it useful to utilize both operators in conjunction. When used carefully, combining the two can enhance the effectiveness of your code.
For instance:
int x = 5;
int y = ++x + x--; // y becomes 12; x becomes 6
In this example, the prefix increment first sets `x` to 6 while calculating `y`, and then the postfix decrement reduces `x` back to 6 after its value was used in the operation for `y`.
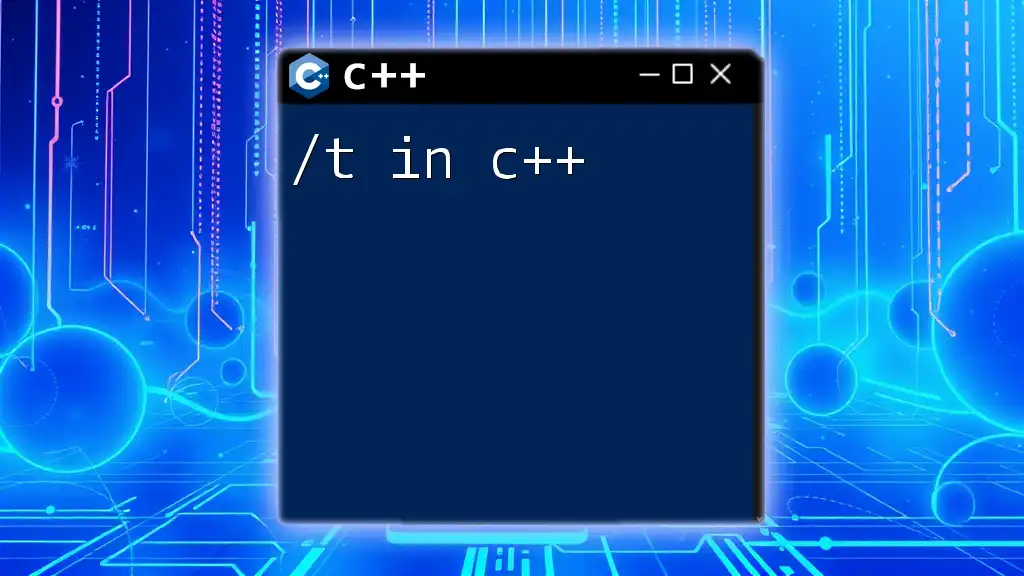
Incrementing and Decrementing in Loops
Using Increment and Decrement in For Loops
In control structures like the `for` loop, incrementing can drive the looping mechanism itself:
for (int i = 0; i < 5; ++i) {
cout << i << " "; // Outputs: 0 1 2 3 4
}
Here, `i` starts at 0 and increments until it reaches 5. Each iteration, `i` is increased by one, allowing the loop to run exactly 5 times.
Using Increment and Decrement in While Loops
While loops can also effectively use increment and decrement operators:
int i = 0;
while (i < 5) {
cout << i << " ";
++i; // Same as i++;
}
In this example, `i` serves as a counter until it reaches 5. The increment operation ensures that the loop will eventually terminate.
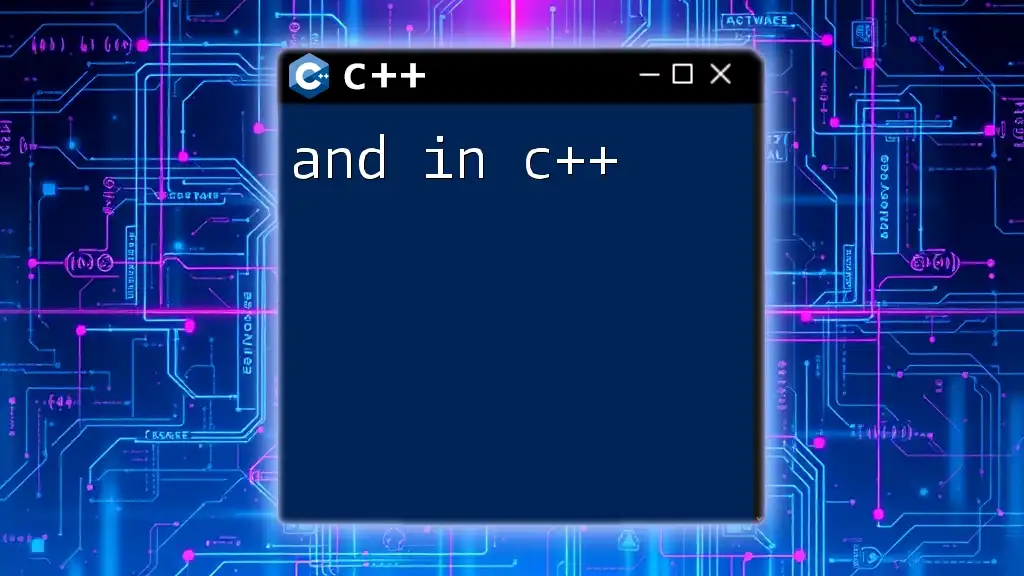
Performance Considerations
Efficiency of Incrementing
From a performance perspective, the incrementing operation is highly efficient and is often optimized by modern compilers. Utilizing increment (`++`) and decrement (`--`) operators can minimize the computation needed in large applications, especially when numerous numerical updates are involved.
Best Practices
While utilizing increment and decrement operators, it's essential to adopt best practices. For instance, ensure clarity in your code—readability is vital. Avoid overly complex expressions that could confuse other developers or yourself in the future.
It's also important to watch out for common pitfalls:
- If you mix prefix and postfix forms without clear reasoning, it can lead to unintended consequences.
- Always maintain a consistent style within your codebase to minimize errors.
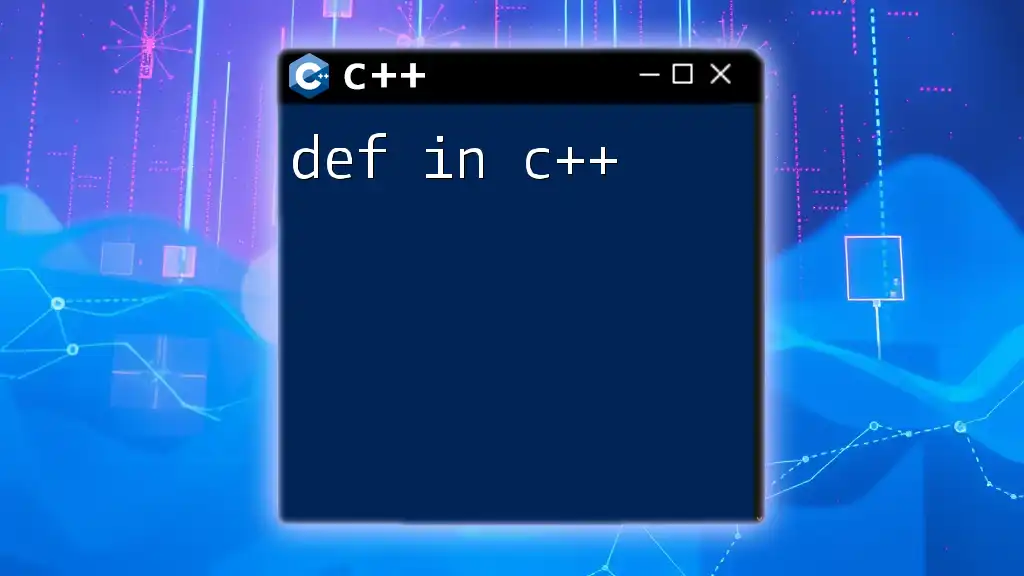
Conclusion
The increment operator (`++`) stands as a simple yet potent tool in the C++ programmer's toolkit. Mastering the `++` operator, along with its counterpart, the decrement operator, provides you with essential techniques for efficient coding and algorithm implementation. By practicing and experimenting with these operators, you can elevate your programming capabilities and pave the way for more advanced computational tasks.
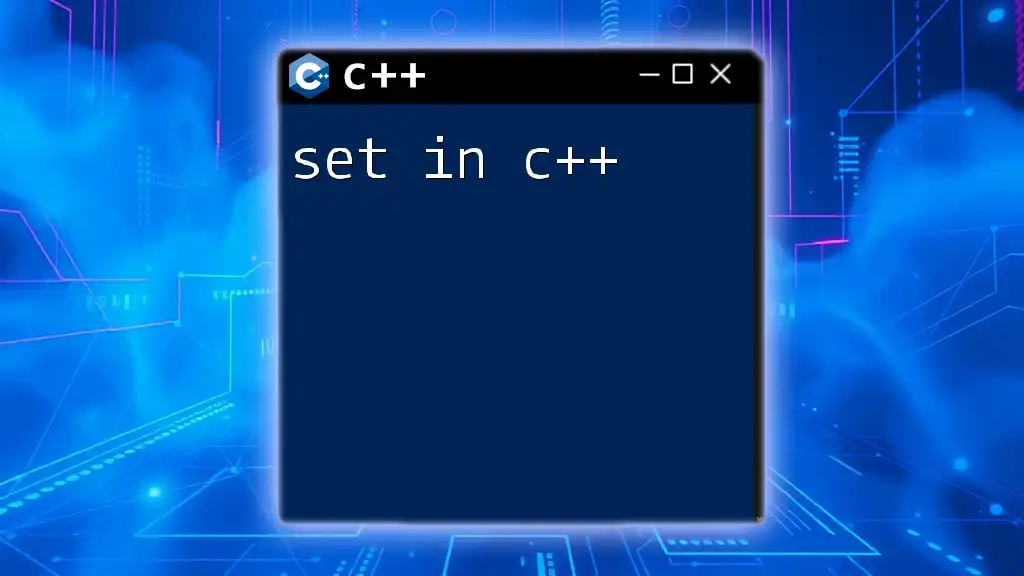
Additional Resources
For those interested in diving deeper, numerous online tutorials, documentation, and coding standards related to C++ increment and decrement operations are available. They can provide further insights and best practices for leveraging these essential C++ operators in more complex scenarios.