The error "multiple definition of first defined here" occurs in C++ when the same function or global variable is defined in multiple translation units, leading to linkage conflicts during the build process.
Here's an example that triggers this error:
// file1.cpp
int myFunction() {
return 5;
}
// file2.cpp
int myFunction() { // Error: multiple definition of 'myFunction'
return 10;
}
To resolve this, you can use `inline` functions or declare the function in a header file and include it properly in a single `.cpp` file.
Understanding C++ Linking Errors
Linking errors are a common hurdle in C++ programming that arise during the linking stage of the compilation process. They can prevent your program from compiling successfully. Understanding these errors is critical for developers, as resolving them ensures that programs run as intended.
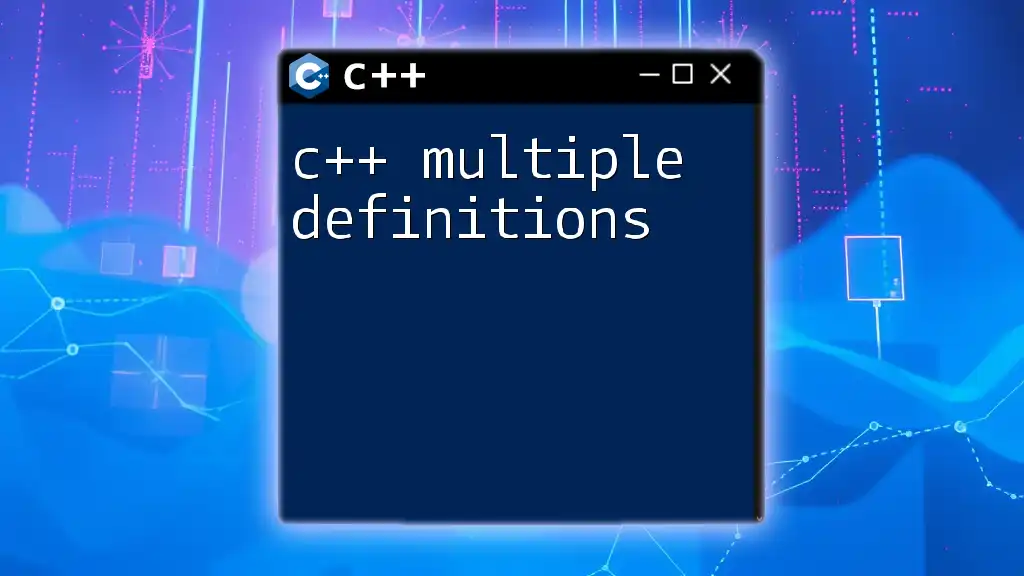
What Does "Multiple Definition" Mean?
Multiple definitions refer to the situation in C++ where the same symbol—whether a function, variable, or class—has been defined more than once within the same or linked translation units. This situation creates ambiguity for the linker, which does not know which definition to use.
When Does This Error Occur?
There are several common scenarios that can lead to a "multiple definition" error:
-
Functions: If you define a function in a header file and include that header in multiple translation units, you will end up with multiple definitions of that function.
-
Global Variables: Global variables defined directly in header files can lead to the same problem as functions.
-
Classes: While classes themselves usually don't cause this type of error, their static member variables can, if not handled properly.
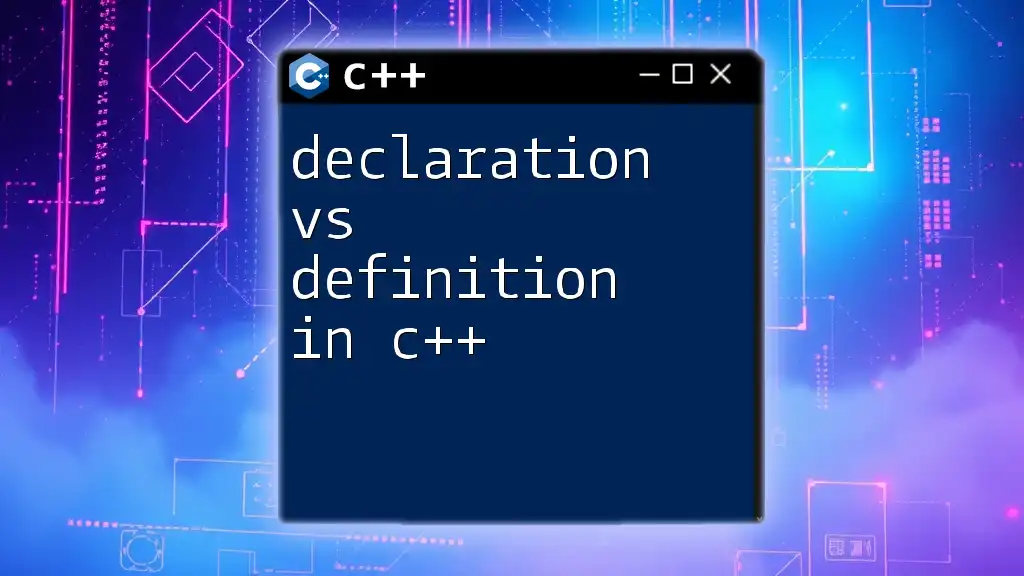
Analyzing the Error Message
When you encounter the error message, "multiple definition of 'symbol'; first defined here," it's crucial to break it down to understand its components.
The message indicates:
- 'symbol': This is the name of the variable or function that has been defined multiple times.
- 'first defined here': This part tells you where the first definition of the symbol was encountered, giving you a starting point to trace back and understand the issue.
For example:
// Example Error
multiple definition of 'foo'; first defined here
This error means that the function or variable named `foo` has been defined in more than one place, causing a conflict when linking.
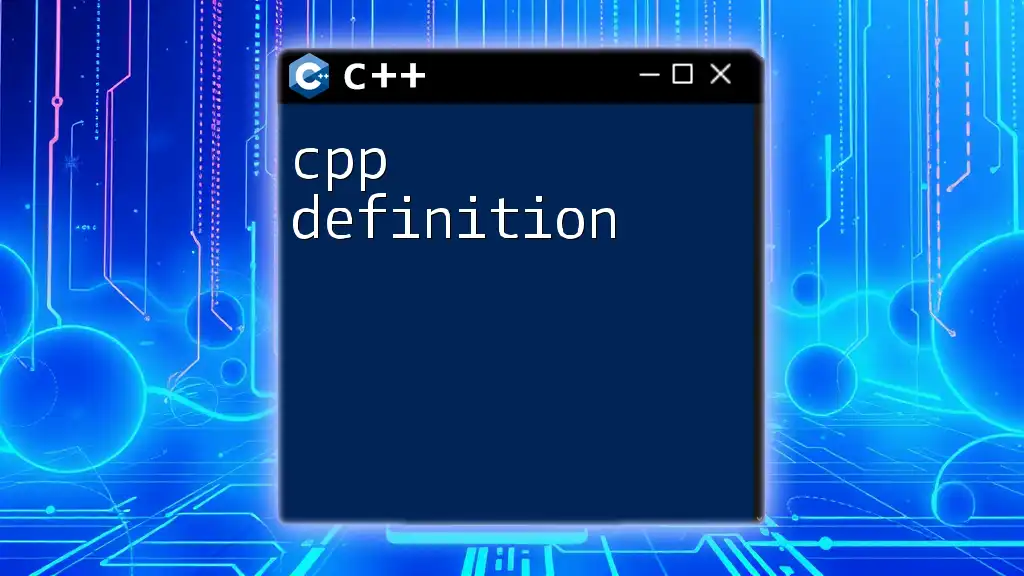
Common Scenarios Leading to Multiple Definitions
Global Variables in Header Files
Global variables defined in header files can cause multiple definitions very easily if that header is included in more than one source file. Here’s an example of incorrect usage:
// global.h
int globalVar = 0; // Defined here
In this scenario, `globalVar` is defined in the header file, and whenever that header is included in multiple `.cpp` files, multiple definitions are created.
Correcting the Issue involves declaring the variable with the `extern` keyword in the header file:
// global.h
extern int globalVar; // Declaration only
Then, you define the variable in a single `.cpp` file:
// global.cpp
#include "global.h"
int globalVar = 0; // Definition here
Functions Defined in Header Files
Another common situation arises when functions are defined in header files without the use of the `inline` keyword. Consider this incorrect usage:
// func.h
void myFunction() {
// Implementation
}
Including this header in multiple source files will cause multiple definitions of `myFunction`.
To resolve this issue, you can either declare the function as `inline`:
// func.h
inline void myFunction() {
// Implementation
}
Using `inline` allows the compiler to include the function definition in each translation unit without causing multiple definitions.
Template Classes and Functions
Template classes and functions function differently in C++ and generally do not cause multiple definition errors if used properly. When a template is instantiated, the compiler generates the necessary code at that instance.
Here’s an example of a proper template usage:
template<typename T>
class MyClass {
public:
void myMethod() {
// Implementation
}
};
Templates generate a new definition whenever the template is instantiated with a different type, thus avoiding conflicts.
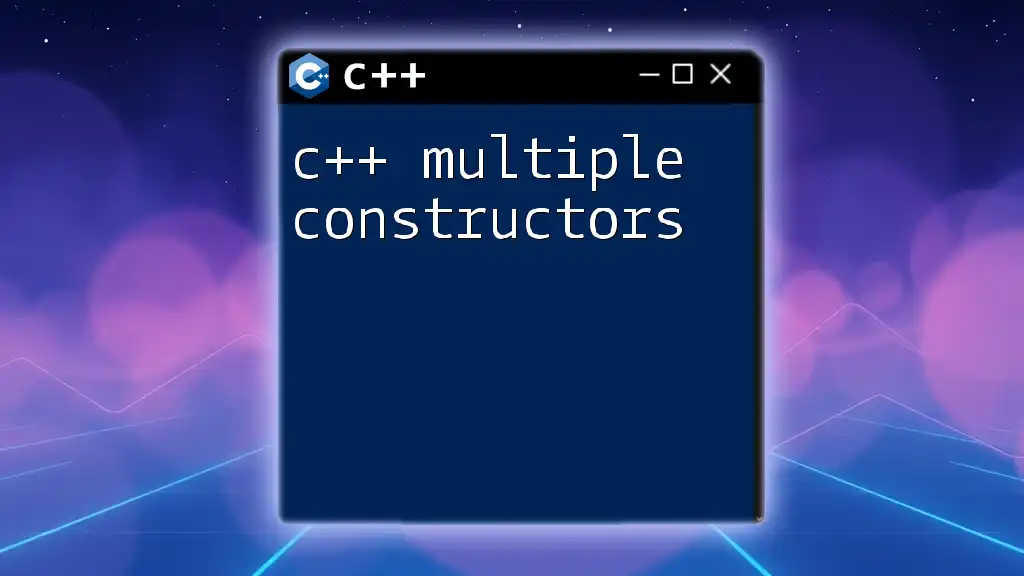
Best Practices to Avoid Multiple Definitions
Using Include Guards
One effective way to prevent multiple definitions is to use include guards. These are preprocessor directives that ensure a header file is included only once during a single compilation. Here’s how you implement them:
#ifndef MY_HEADER_H
#define MY_HEADER_H
// Declarations
#endif // MY_HEADER_H
This technique eliminates the risk of defining symbols multiple times when headers are included in multiple files.
Utilizing `#pragma once`
An alternative to include guards is the `#pragma once` directive. This is a non-standard but widely supported way to achieve the same goal of preventing multiple inclusions.
#pragma once
// Declarations
This method simplifies header management and reduces the likelihood of human error.
Organizational Strategies in Codebases
Maintaining a clean structure in your codebase helps mitigate linking errors. Always separate declarations from definitions. Use header files for declarations and implement your functions in `.cpp` files. This organization prevents the linker from encountering multiple definitions.
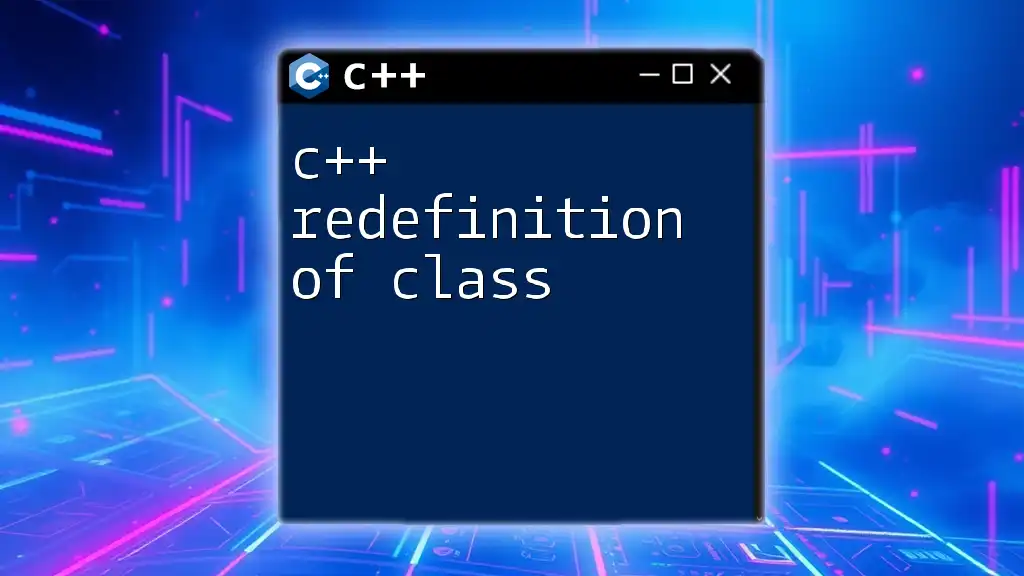
Debugging Multiple Definition Errors
Steps to Identify the Source
If you encounter a linking error, you can utilize compiler flags to gain further insight. For instance, the flag `-Wl,--warn-once` can provide additional warnings and details that may help identify the cause of the multiple definition.
Tools and Techniques
Using a debugger like GDB can help trace the origins of multiple definitions. By inspecting the backtrace and checking included files, you can locate where the conflicting definitions are coming from.
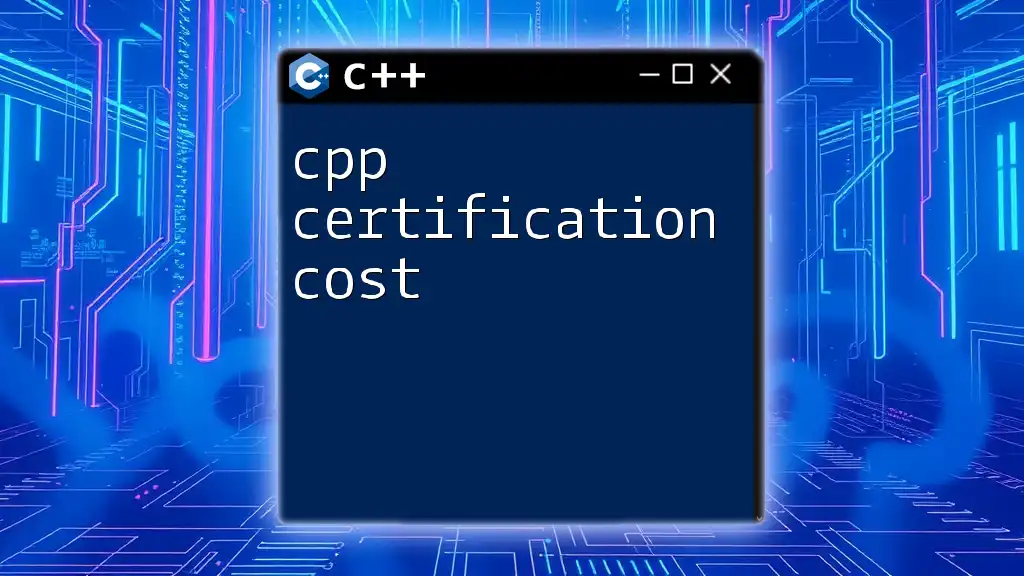
Conclusion
In summary, understanding how and why the "cpp multiple definition of first defined here" error occurs is essential for anyone writing C++ code. By being mindful of your definitions and following best practices, you can avoid this common issue. Always separate declarations from definitions, utilize include guards or `#pragma once`, and keep your code organized for smoother compilation processes.
Through diligent practice and learning, you can effectively manage C++ linking errors, ensuring that your projects compile and run smoothly. Don't hesitate to explore further resources and deepen your understanding of this intricate part of C++.