To determine how many times you can divide a number by two until it reaches zero, you can use a simple loop in C++. Here's a concise code snippet demonstrating this:
#include <iostream>
int main() {
int num = 64; // Example number
int divisions = 0;
while (num > 0) {
num /= 2;
divisions++;
}
std::cout << "Number of divisions by two until 0: " << divisions << std::endl;
return 0;
}
What is the Concept of Divisions by Two?
The process of repeatedly dividing a number by two is a fundamental concept in programming, especially in C++. Understanding this concept is crucial as it relates not only to mathematical operations but also to algorithm optimization and problem-solving. When we talk about dividing by two until we reach zero, we are exploring how many times we can halve a number before it becomes zero.
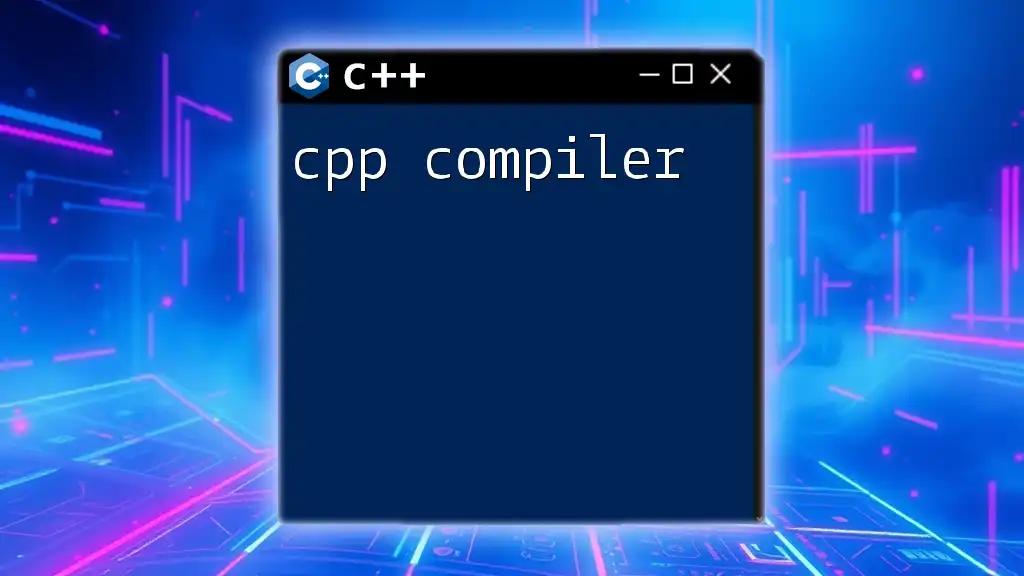
Overview of the Objective
The objective here is to learn how to determine how many times a given number can be divided by two before it reaches zero. This task may sound straightforward, but it's essential for various algorithmic applications, including runtime analysis and recursion.
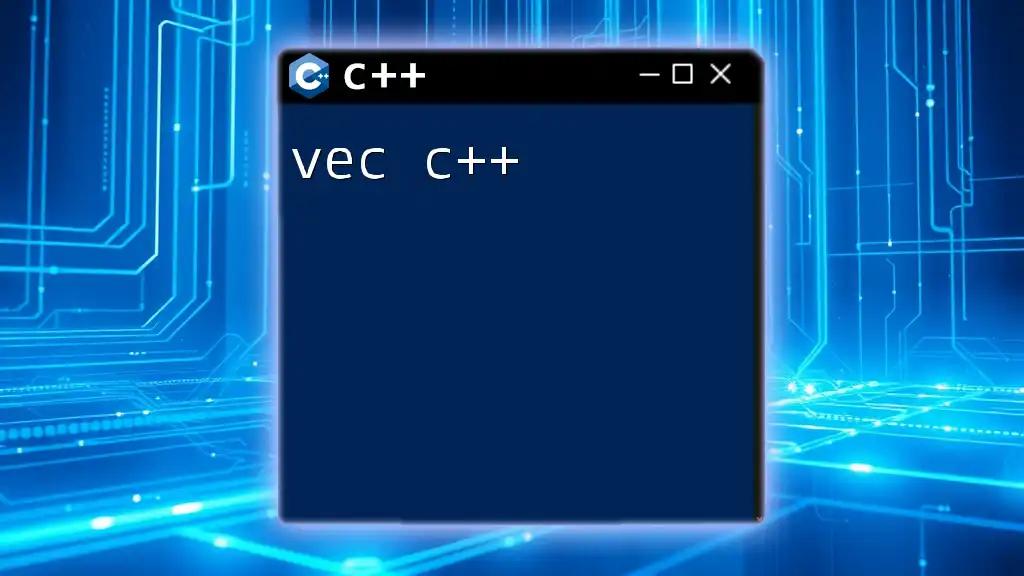
Understanding Division in C++
Basic Arithmetic Operations in C++
In C++, arithmetic operations form the basis of most programming logic. C++ supports four primary arithmetic operations: addition, subtraction, multiplication, and division.
For example, consider the division operation:
int a = 10;
int b = 2;
int result = a / b; // result will be 5
Here, `result` holds the value of `5` because `10` divided by `2` equals `5`. Understanding how these operations behave with integers and floating points is vital for designing algorithms that require numerical computations.
Integer Division in C++
In C++, when performing division with integers, the result is also an integer, which means any decimal part is discarded. This behavior is crucial when determining how many divisions can occur before reaching zero.
For instance, if you divide `9` by `2`, you get `4` instead of `4.5`, due to integer truncation. Here’s a quick demonstration:
int num = 9;
int divided = num / 2; // divided will be 4
This property can affect your logic when counting divisions by two.
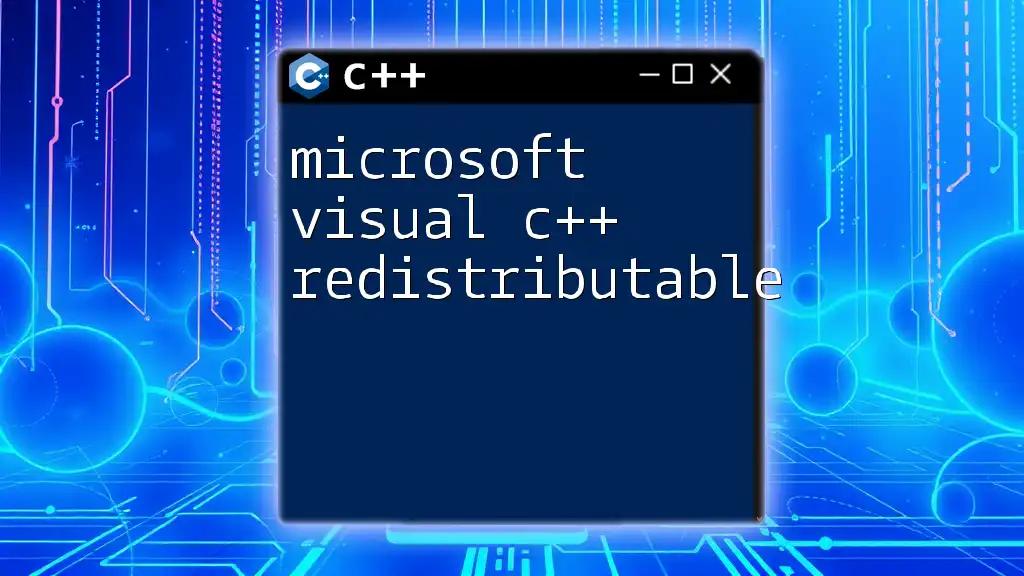
Algorithm to Count Divisions by Two
Conceptualizing the Problem
To tackle the problem of counting divisions by two, we need to visualize the process. The key points to consider are:
- Initial Conditions: What happens if the number is `0` or negative? In these cases, the result will be `0` because there are no divisions that can yield any positive count.
Writing the Algorithm
To count how many times a given number can be divided by two until it reaches zero, we can follow a straightforward algorithm:
- Initialize a Counter: Start a counter variable to zero. This will keep track of the number of divisions.
- While Loop Condition: Use a `while` loop that continues as long as the number is greater than zero.
- Perform Division: Divide the number by two and increment the counter by one each time.
- Return the Result: Once the loop exits, return the counter.
Here’s how this looks in code:
int countDivisionsByTwo(int number) {
int count = 0;
while (number > 0) {
number /= 2;
count++;
}
return count;
}
Testing the Function
To ensure the function works correctly, we should test it with various inputs:
#include <iostream>
using namespace std;
int main() {
cout << countDivisionsByTwo(16) << endl; // Output: 4
cout << countDivisionsByTwo(10) << endl; // Output: 4
cout << countDivisionsByTwo(0) << endl; // Output: 0
cout << countDivisionsByTwo(-8) << endl; // Output: 0
return 0;
}
These test cases confirm the function counts the divisions accurately and handles edge cases properly.
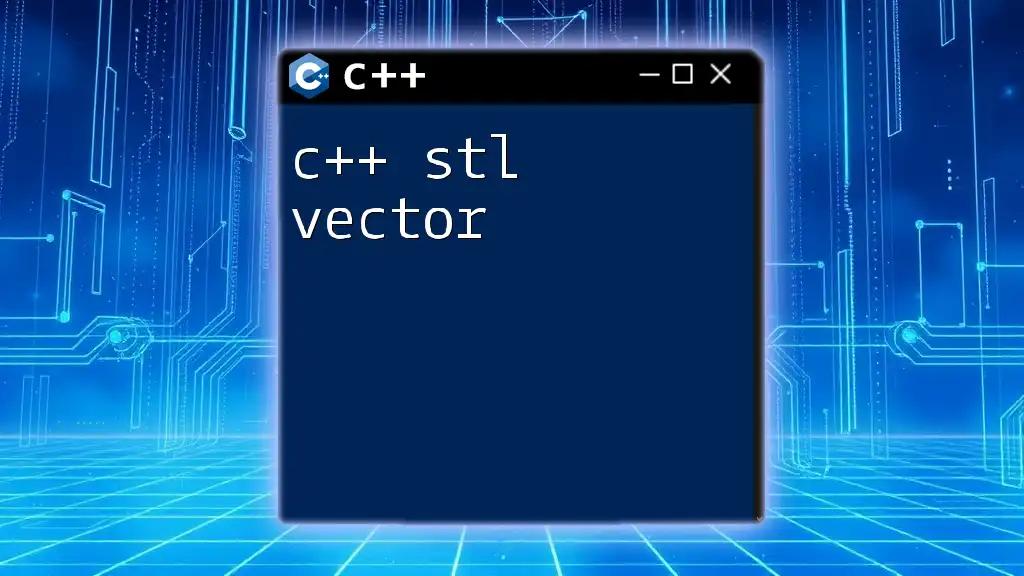
Optimizing the Code
Reducing Complexity
The original implementation of counting divisions runs in linear time, \(O(n)\). While this may suffice for small numbers, performance can suffer with large integers. Thus, optimizing the function is necessary for efficiency.
Alternative Approaches
A more efficient approach utilizes logarithmic calculations. The number of times `x` can be divided by `2` until it reaches zero is equal to \( \text{floor}(\log_{2}(x)) + 1 \).
Here’s an optimized function using logarithmic calculations:
#include <cmath>
int efficientCountDivisionsByTwo(int number) {
return (number > 0) ? static_cast<int>(log2(number)) + 1 : 0;
}
This code achieves a time complexity of \(O(1)\), making it significantly faster for large values.
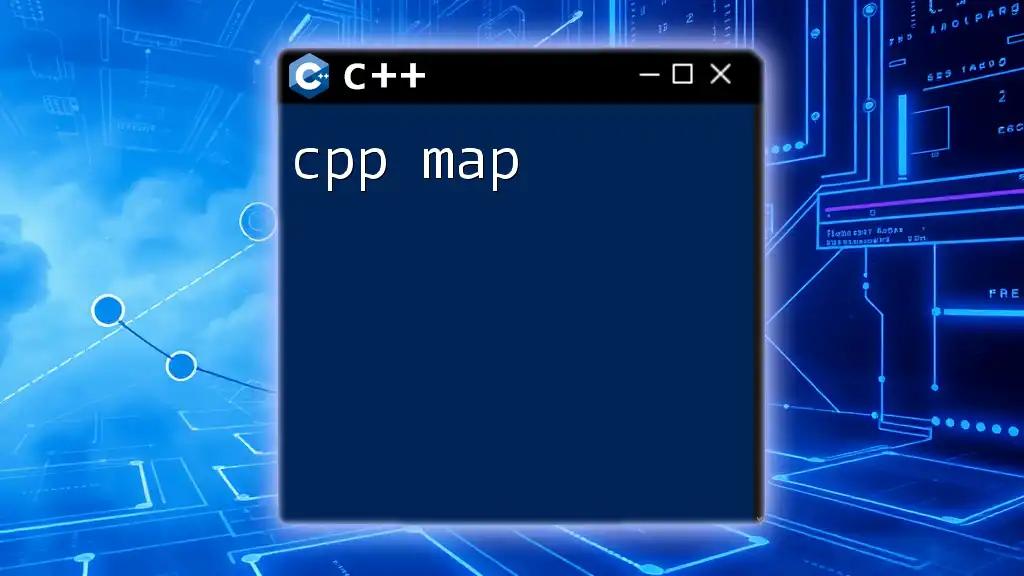
Real-world Applications
Understanding how to count divisions by two has practical applications in various domains, such as:
Use Cases in Software Development
Many algorithms involve operations that need power of two calculations, such as efficient sorting or searching algorithms (e.g., binary search). Knowing how to manipulate numbers through division helps streamline such operations, leading to better performance.
Practical Applications in Competitive Programming
In competitive programming, problems frequently require manipulation of binary representations or logarithmic operations. The skills learned here will aid developers in solving complex challenges rapidly and effectively.
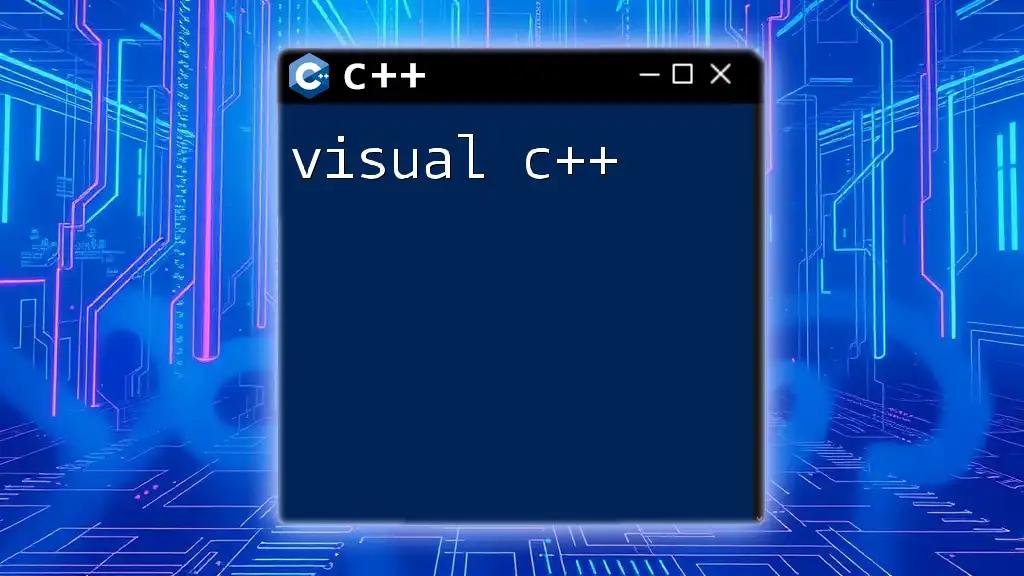
Conclusion
In summary, understanding how to determine how many divisions by two until you reach zero can substantially enhance your programming toolkit in C++. Whether it involves simple loops or advanced logarithmic calculations, mastering this concept offers a solid foundation for improving your coding efficiency and performance.
Feel free to practice similar problems using C++ to sharpen your skills further. Exploring other C++ commands and algorithms will only bolster your capabilities as a programmer.