In C++, `std::numeric_limits<float>::max()` is used to retrieve the maximum finite value representable by the float data type. Here's a code snippet demonstrating this:
#include <iostream>
#include <limits>
int main() {
std::cout << "Maximum float value: " << std::numeric_limits<float>::max() << std::endl;
return 0;
}
Understanding Floating-Point Types in C++
What are Floating-Point Types?
Floating-point numbers are a way to represent real numbers in a format that can accommodate a wide range of values. Unlike integers, which can only express whole numbers, floating-point numbers can represent fractions, providing significant versatility when dealing with calculations that require precision.
As floating-point types allow for representation of vast ranges of values, they become invaluable in scientific calculations, simulations, and graphics.
Types of Floating-Point Variables in C++
C++ offers several floating-point types, each with distinct capacities and precisions:
float
The `float` type typically consists of 32 bits (4 bytes) and offers a precision of about 6 to 7 decimal digits. It is the most commonly used type for single-precision floating-point representation.
double
The `double` type occupies 64 bits (8 bytes) and provides a higher precision of approximately 15 decimal digits. As a rule of thumb, if greater precision is needed in calculations, opting for `double` over `float` is often advisable.
long double
This type generally utilizes 80, 96, or 128 bits, providing even greater precision than a `double`. The exact size can vary depending on the compiler and platform. Use `long double` when working on highly sensitive calculations that require maximum precision.
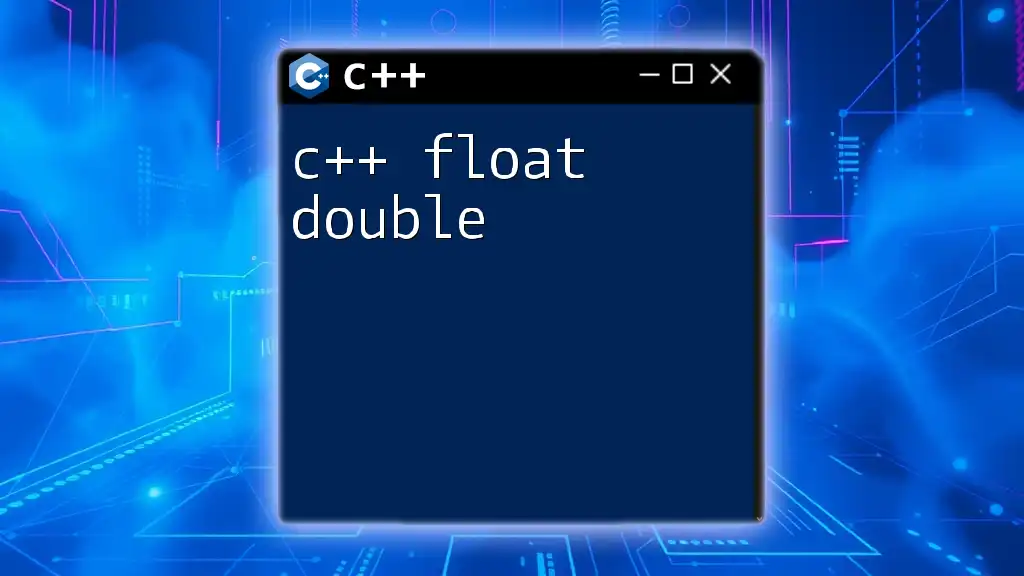
What is `std::numeric_limits`?
Introduction to `std::numeric_limits`
To manage and access the characteristics of floating-point types effectively, C++ provides the `std::numeric_limits` class. This utility enables developers to obtain details about numeric types, including their limits, like maximum and minimum values.
How to Use `std::numeric_limits`
To leverage `std::numeric_limits`, include the `<limits>` header in your code. Below is a sample code that demonstrates its usage:
#include <limits>
#include <iostream>
int main() {
std::cout << "Max float value: " << std::numeric_limits<float>::max() << std::endl;
return 0;
}
The line `std::numeric_limits<float>::max()` retrieves the maximum representable value for the `float` type. This method can be applied similarly to `double` and `long double`.
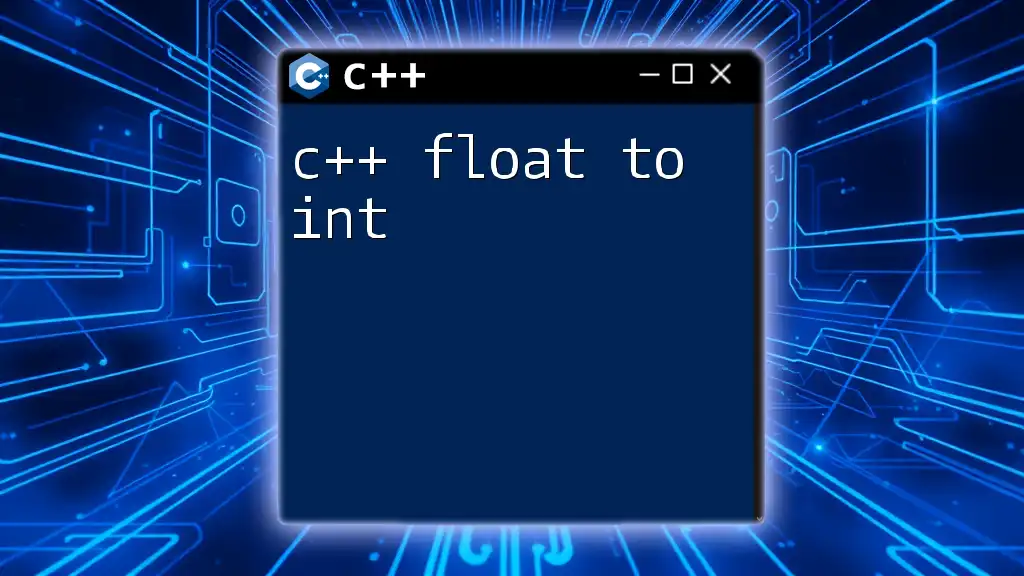
Exploring `float` Maximum Value
Finding `float` Maximum Value
The `float` maximum value, determined by `std::numeric_limits`, signifies the largest positive number that can be represented by this type. This value is essential for performing calculations where boundaries are relevant, and knowing this limit allows for effective error handling in applications.
Here's how you can find it in code:
float maxFloat = std::numeric_limits<float>::max();
std::cout << "Max float: " << maxFloat << std::endl;
Uses and Implications of Maximum Float Value
In many real-world applications, such as simulations and financial computations, knowing the `float` maximum value is crucial. Using values greater than this limit leads to overflow, resulting in unexpected behaviors or errors, like returning Infinity or throwing exceptions in supported environments.
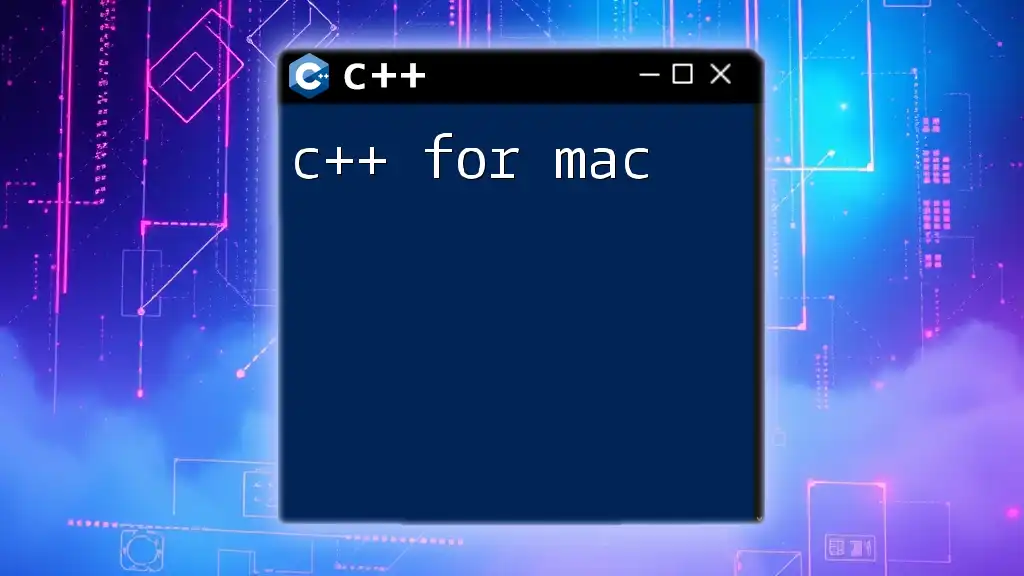
Comparisons with Other Floating-Point Types
Comparison of `float`, `double`, and `long double` Max Values
Understanding the maximum values of `float`, `double`, and `long double` is essential for choosing the appropriate type for your needs. Here are the maximum values provided by `std::numeric_limits`:
std::cout << "Max double value: " << std::numeric_limits<double>::max() << std::endl;
std::cout << "Max long double value: " << std::numeric_limits<long double>::max() << std::endl;
- Maximum values for each type:
- `float`: Around 3.4 × 10^38
- `double`: Around 1.7 × 10^308
- `long double`: Depending on the implementation, can be even larger.
When to Use Each Floating-Point Type
When to use each type depends on your application requirements:
- Use `float` for applications that demand lower precision and where performance is critical, such as graphics programming.
- Use `double` when greater precision is required, particularly in scientific calculations.
- Use `long double` for scenarios that incredibly sensitive to any rounding errors.
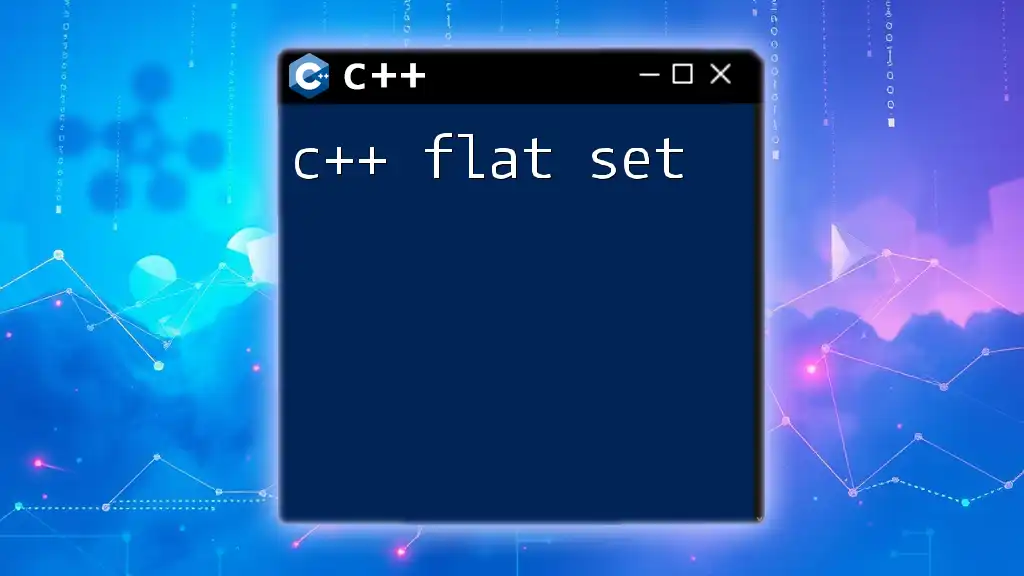
Handling Overflow and Underflow
What is Overflow?
Overflow occurs when a calculation produces a number larger than what can be represented by the type. When this happens with `float`, it typically results in a special value, `Infinity`.
What is Underflow?
Conversely, underflow occurs when a calculation yields a number closer to zero than what the type can represent. For `float`, a very small positive number may lead to underflow and ultimately return `0`.
Techniques to Handle Overflow/Underflow
To manage overflow and underflow, implement the following techniques:
- Input Validation: Always validate inputs to ensure they fall within acceptable ranges.
- Error Handling: Use exceptions or condition checks after operations that may lead to overflow/underflow scenarios.
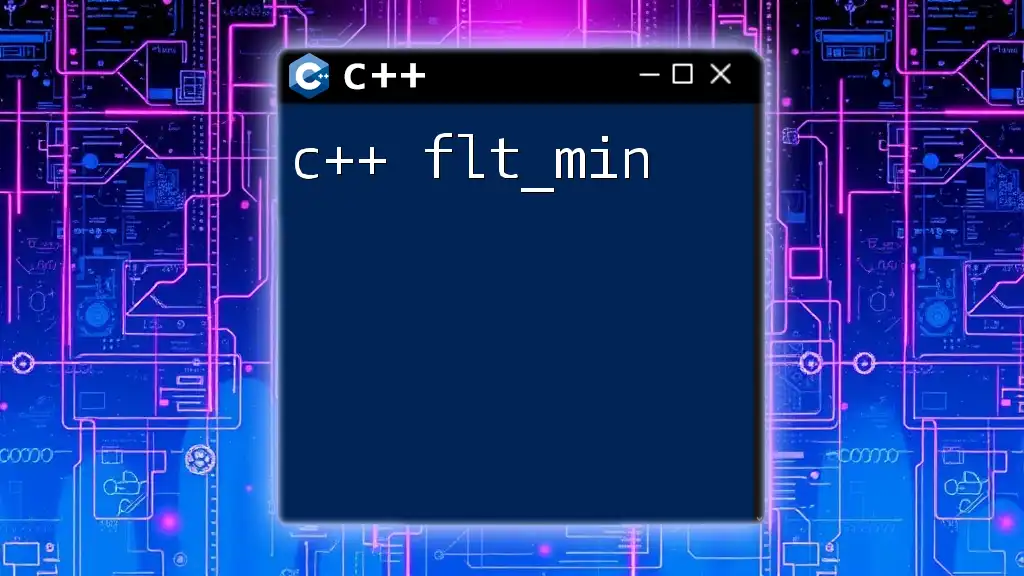
Common Use Cases and Best Practices
Use Cases for Floating-Point Maximum Value
In applications dealing with physics simulations, financial calculations, and real-time data processing, knowing the maximum `float` value enables developers to create effective algorithms capable of handling large datasets without errors.
Best Practices When Working with Floating Points
Several best practices help when working with floating-point numbers:
- Be Aware of Precision: Understand how floating-point arithmetic can introduce inaccuracies. Always choose the appropriate type based on precision needs.
- Mind the Limits: Be cautious of the maximum values and the potential for overflow and underflow in critical calculations.
- Use Libraries: Consider using libraries designed for high-precision arithmetic when necessary.
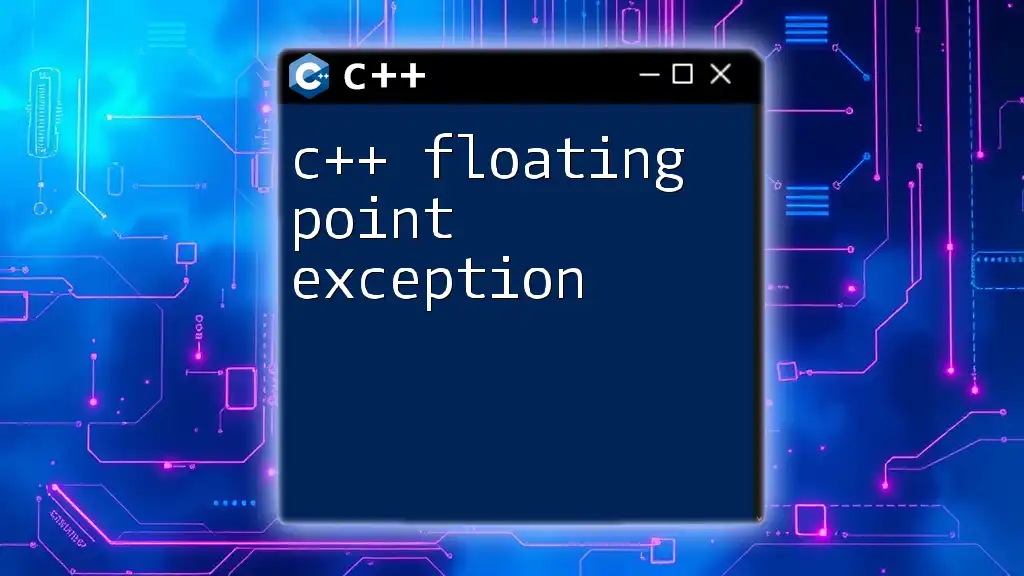
Conclusion
Understanding the concept of C++ float max and the characteristics of floating-point types not only strengthens programming skills but also promotes the development of robust applications. By using `std::numeric_limits`, developers gain essential insights into the boundaries of floating-point variables, enabling better integration of mathematical operations and error handling practices.