In C++, `FLT_MIN` is a constant representing the smallest positive normalized floating-point value that can be represented by the `float` data type, typically used to avoid underflow in calculations.
Here's a code snippet that demonstrates how to use `FLT_MIN`:
#include <iostream>
#include <cfloat> // Include for FLT_MIN
int main() {
std::cout << "The minimum positive normalized float value is: " << FLT_MIN << std::endl;
return 0;
}
Understanding Floating-Point Representation
What is a Floating-Point Number?
A floating-point number is a way to represent real numbers in programming languages, allowing for a vast range of values. The representation consists of three parts: the sign, the exponent, and the mantissa (or significand). This form enables the representation of very large or very small numbers efficiently.
IEEE 754 Standard
The IEEE 754 standard defines the format for binary floating-point arithmetic in computers. C++ adheres to this standard, which outlines how to handle floating-point numbers, including their precision, representation, and behavior in mathematical operations. The standard specifies various types, such as:
- float: Typically 32 bits, representing single-precision.
- double: Typically 64 bits, representing double precision.
- long double: Often 80 bits or more, providing extended precision.
Understanding how C++ implements these formats is critical for effective programming.
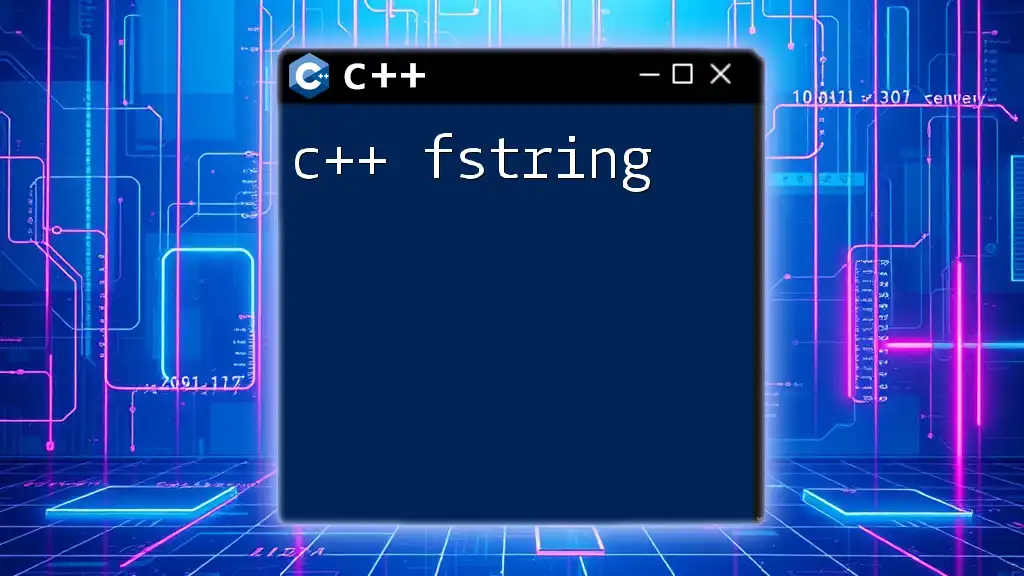
The flt_min Macro and Constants
Defining flt_min
The flt_min constant is a predefined macro in C++, found in the `<cfloat>` (or `<float.h>`) header file. It represents the smallest positive normalized value for a floating-point number of type float. Essentially, flt_min is crucial when precision and small positive values are required.
How flt_min Relates to Other Constants
flt_min is part of a larger set of constants that define the limits of floating-point types. To grasp its importance, it's essential to understand its relationship with other constants like flt_max, which denotes the largest representable float value.
Here is a code snippet showing these constants:
#include <iostream>
#include <cfloat> // for flt_min and flt_max
int main() {
std::cout << "Smallest positive float: " << FLT_MIN << std::endl;
std::cout << "Largest float: " << FLT_MAX << std::endl;
return 0;
}
In the above example, the output will display the values of both FLT_MIN and FLT_MAX, providing you with an understanding of the floating-point range in C++.
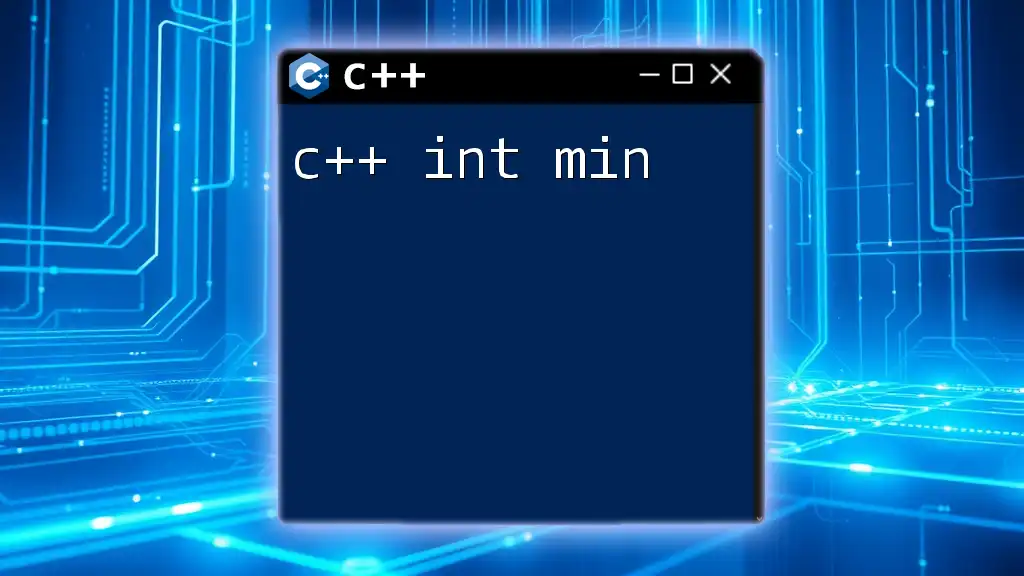
How to Use flt_min in Your C++ Applications
Practical Applications of flt_min
flt_min serves several practical purposes in C++ programming, especially in algorithms requiring a minimum threshold for calculations. Use it in scenarios where you want to avoid division by very small numbers or when implementing algorithms sensitive to underflow.
Here’s an example:
#include <iostream>
#include <cfloat>
void computeWithMin(float value) {
if (value < FLT_MIN) {
std::cout << "Value is too small, using FLT_MIN instead." << std::endl;
value = FLT_MIN;
}
std::cout << "Using value: " << value << std::endl;
}
int main() {
computeWithMin(0.0f); // Example with a number less than FLT_MIN
return 0;
}
This code checks if the provided `value` is smaller than FLT_MIN and uses FLT_MIN as a fallback. This approach prevents potential mathematical errors during calculations.
Example Code Snippets
Here’s a more complex example showcasing how flt_min can be used in a function that calculates the effective value from small inputs:
#include <iostream>
#include <cfloat>
float safeDivision(float numerator, float denominator) {
if (denominator < FLT_MIN) {
std::cout << "Denominator too small, using FLT_MIN to avoid division by zero." << std::endl;
denominator = FLT_MIN;
}
return numerator / denominator;
}
int main() {
float result = safeDivision(10.0f, 0.0f);
std::cout << "Result of safe division: " << result << std::endl; // Outputs result using FLT_MIN
return 0;
}
In this snippet, the function checks if the denominator is below FLT_MIN and substitutes it if necessary, ensuring that the program avoids division by zero errors.
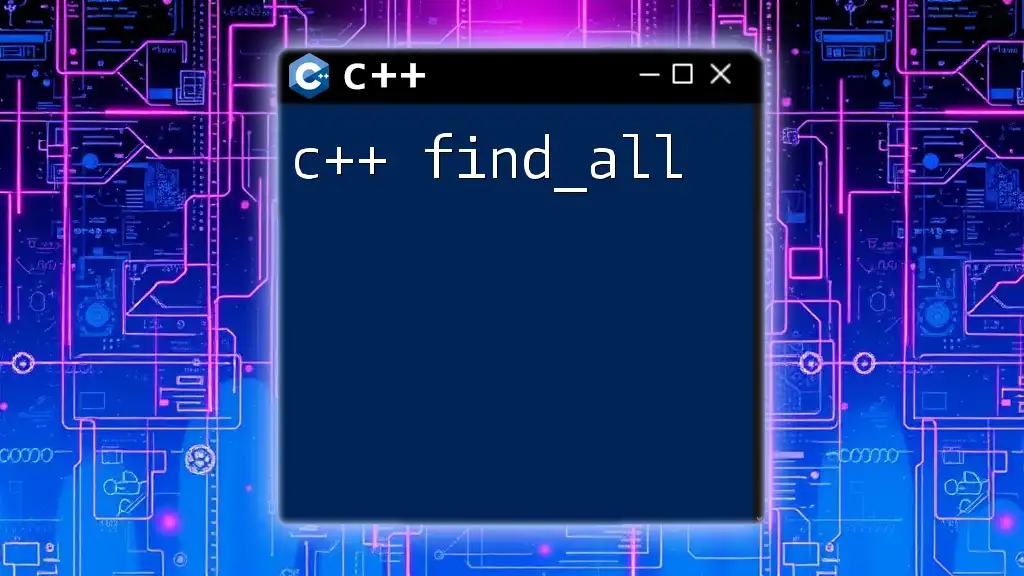
Common Pitfalls When Using flt_min
Limitations of flt_min
While flt_min serves a vital function, it does have limitations. For example, if calculations exceed the boundaries set by floating-point representation, you may encounter errors, precision loss, or unexpected behavior. It's crucial to understand the limits of floating-point representation to use it effectively.
Tips for Avoiding Mistakes
To avoid mistakes related to flt_min, consider implementing best practices, such as:
- Always check values before performing operations.
- Use appropriate types for calculations where precision matters.
- Be aware of floating-point arithmetic rules, as they can lead to unintended results due to rounding errors.
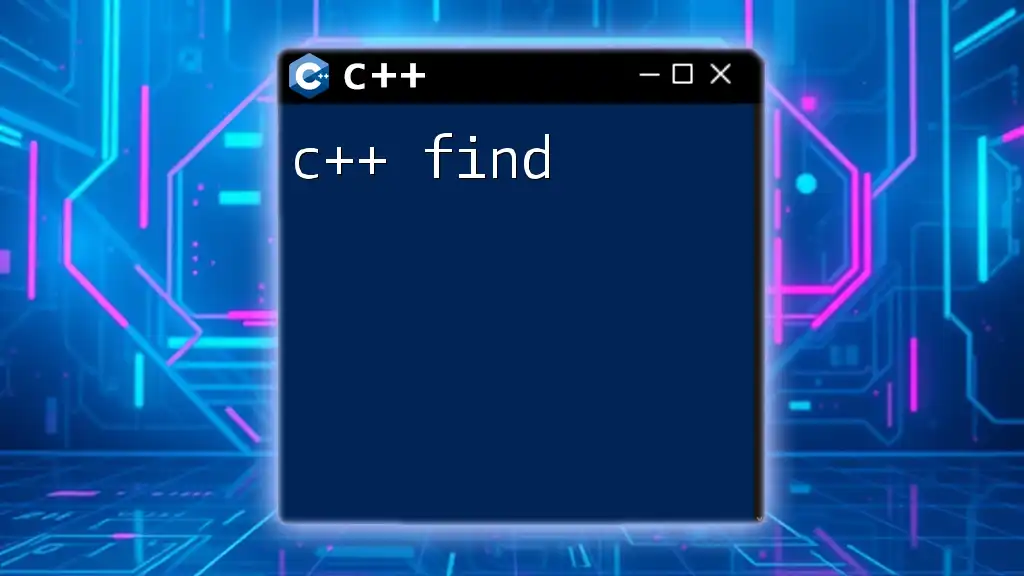
Performance and Value of flt_min
Why Consider Performance?
The performance impact of using flt_min extends to how floating-point precision affects overall computation. In algorithms that involve many iterations or complex calculations, precision directly influences performance and resource utilization.
Profiling and Benchmarking
To ensure you're making the most of your floating-point operations, consider using profiling tools that help measure performance. Examples include gprof for GNU and Visual Studio Profiler for Windows. Benchmarking allows you to analyze the efficiency of different methods involving flt_min.
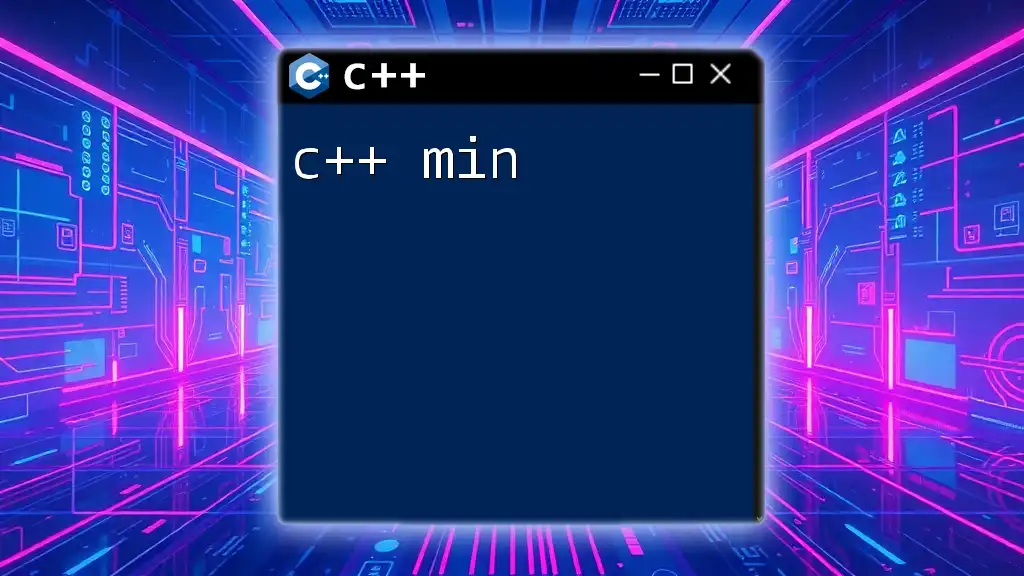
Conclusion
In summary, understanding c++ flt_min opens a window into the delicate handling of floating-point numbers in C++. From preventing underflow to ensuring algorithm correctness, flt_min is a critical component for developers.
Experimenting with flt_min in your projects will deepen your insights into floating-point arithmetic. Mastery of these concepts will enrich your programming skillset and lead to more robust C++ applications.
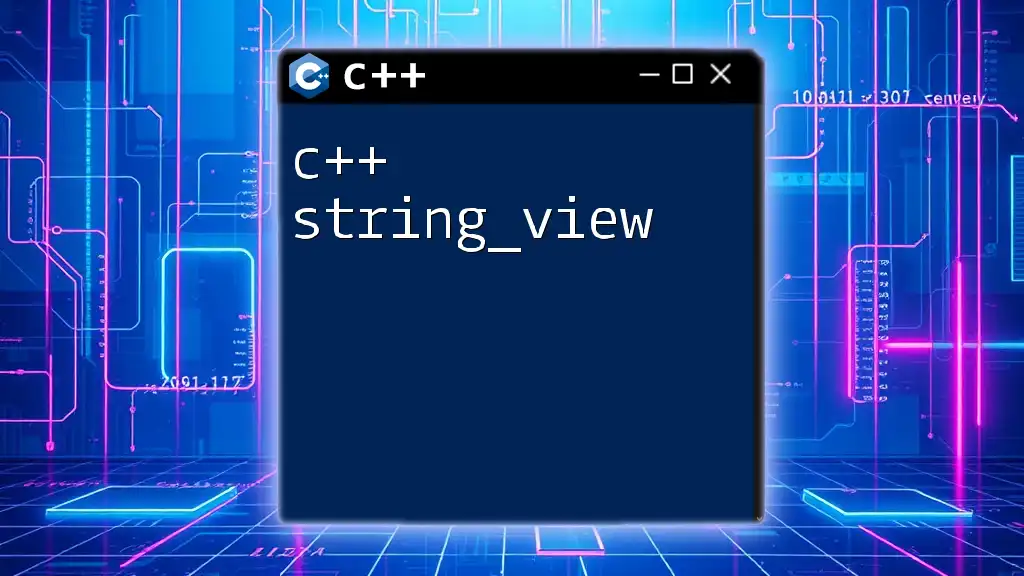
Frequently Asked Questions (FAQ)
What happens when you divide by flt_min?
Dividing by flt_min does not cause an error, but it will result in a significantly increased value. This may lead to unexpected results, so always check if your denominator is appropriately sized.
Is flt_min specific to C++, or is it found in other languages?
flt_min is a C++ constant, but similar concepts exist in other languages. For example, Python has the `sys.float_info` module, which provides parameters that include the smallest representable positive float.
How can I ensure my floating-point operations are safe?
To ensure safety in floating-point operations, validate inputs, check against flt_min, and use libraries designed for numerical stability, such as Eigen or Armadillo for C++.
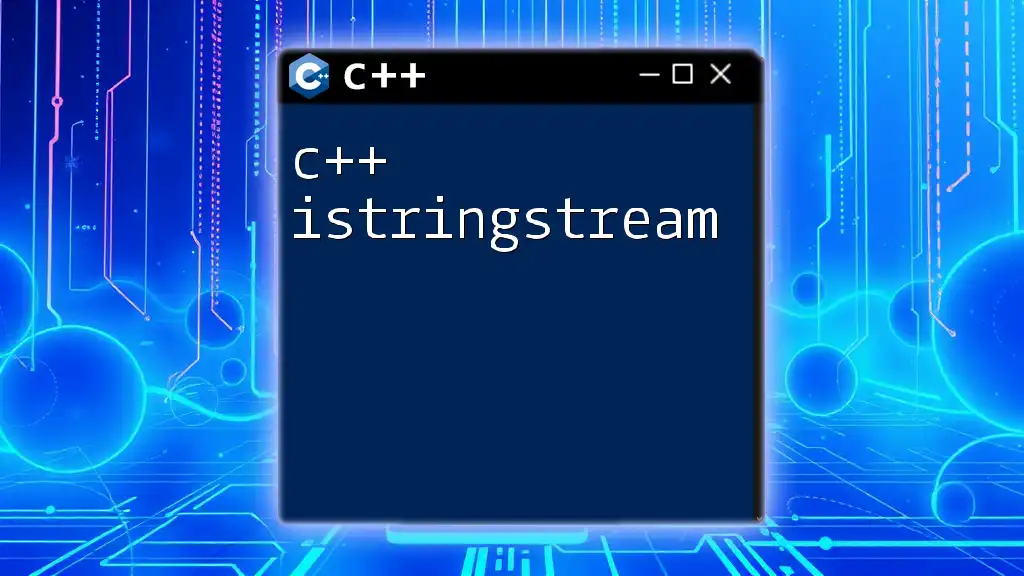
Additional Resources
External Links
- C++ Documentation on `<cfloat>`: [cplusplus.com](http://www.cplusplus.com/reference/cfloat/)
- Recommended Books:
- "Numerical Recipes in C++" – Covers numerical methods and floating-point operations.
Further Learning
Look into available curriculums, online courses, and tutorials concentrating on floating-point precision and numerical methods in C++. Engaging in projects that challenge your understanding of these concepts will significantly enhance your programming capability.