In C++, the `argc` and `argv` parameters of the `main` function allow you to handle command-line arguments, where `argc` counts the number of arguments, and `argv` is an array of C-style strings representing the arguments.
Here’s a simple code snippet demonstrating their usage:
#include <iostream>
int main(int argc, char* argv[]) {
std::cout << "Number of arguments: " << argc << std::endl;
for (int i = 0; i < argc; ++i) {
std::cout << "Argument " << i << ": " << argv[i] << std::endl;
}
return 0;
}
What Are Command Line Arguments?
Command line arguments in C++ are parameters passed to the `main` function when a program is executed. These arguments enable users to influence the program's behavior without modifying the code directly. They provide a flexible way to input data, control execution flow, or adjust settings dynamically.
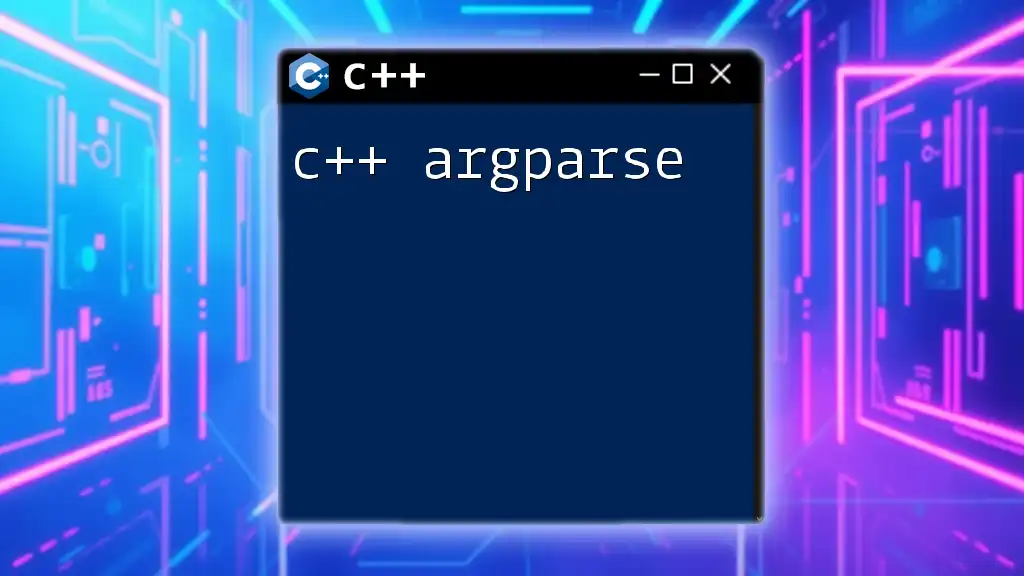
How C++ Handles Command Line Arguments
In C++, command line arguments are handled through the `main` function and its parameters: `int argc` and `char* argv[]`.
- `argc` (argument count) represents the number of command line arguments passed, including the program name.
- `argv` (argument vector) is an array of C-style strings (character pointers) that holds each argument as a string.
This structure allows programmers to access user inputs easily and incorporate them into their applications.
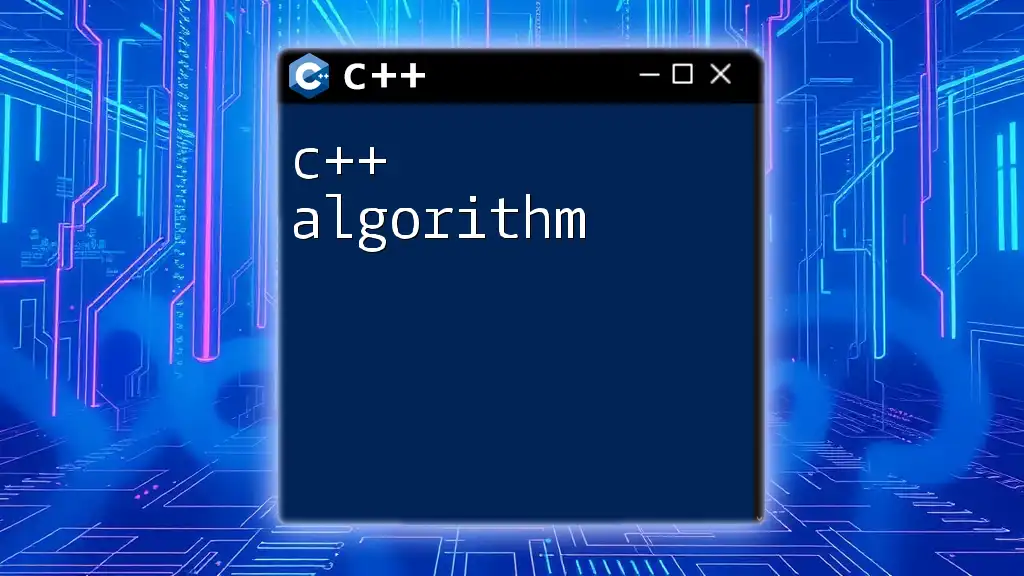
Understanding argc and argv
Defining argc
`argc` is an integer that counts how many arguments are passed to the program. For instance, if you run:
./my_program arg1 arg2 arg3
In this case, `argc` would be `4`, because it counts the program name as the first argument.
Exploring argv
`argv` is an array of C-style strings where each element points to the respective argument:
- `argv[0]` is the name of the program.
- `argv[1]` is the first user input (in this example, `arg1`).
- `argv[2]` corresponds to `arg2`.
- `argv[3]` corresponds to `arg3`.
By examining `argv`, you can access and utilize different inputs provided by users during execution.
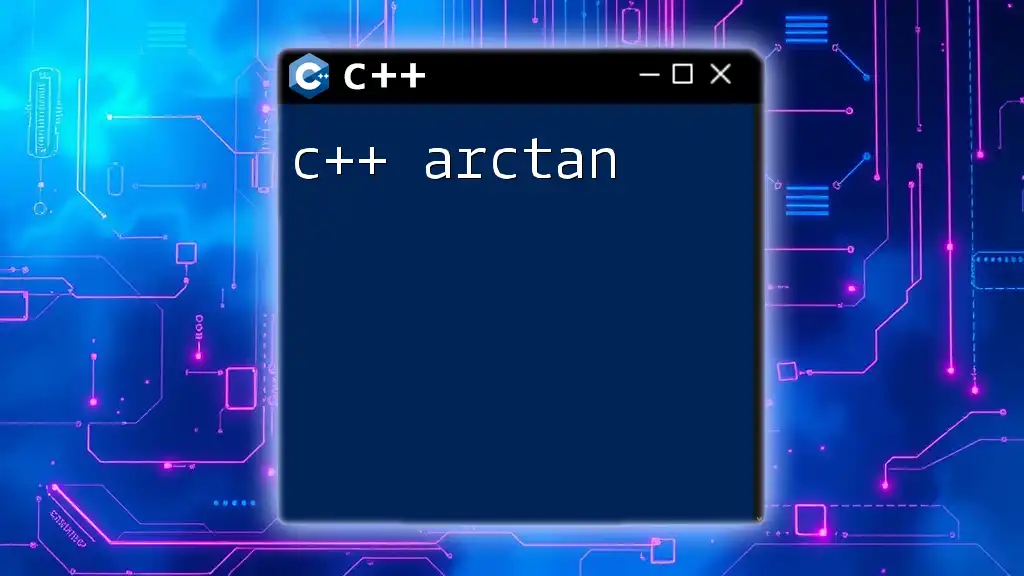
Using Command Line Arguments in C++
Basic Structure of the main Function with Arguments
The typical way to declare the `main` function to utilize command line arguments is:
int main(int argc, char* argv[]) {
// Your code here
}
Here's a simple example demonstrating the use of command line arguments:
#include <iostream>
int main(int argc, char* argv[]) {
std::cout << "Number of arguments: " << argc << std::endl;
for (int i = 0; i < argc; i++) {
std::cout << "Argument " << i << ": " << argv[i] << std::endl;
}
return 0;
}
Common Use Cases for Command Line Arguments
Command line arguments are typically used in various scenarios to enhance program functionality. For example, you might have a program that reads a file specified by the user:
#include <iostream>
#include <fstream>
#include <string>
int main(int argc, char* argv[]) {
if (argc < 2) {
std::cerr << "Usage: " << argv[0] << " <filename>" << std::endl;
return 1;
}
std::ifstream file(argv[1]);
if (!file) {
std::cerr << "Error opening file: " << argv[1] << std::endl;
return 1;
}
std::string line;
while (std::getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
return 0;
}
In this example, if a valid filename is provided as a command line argument, the program reads and outputs the file contents.
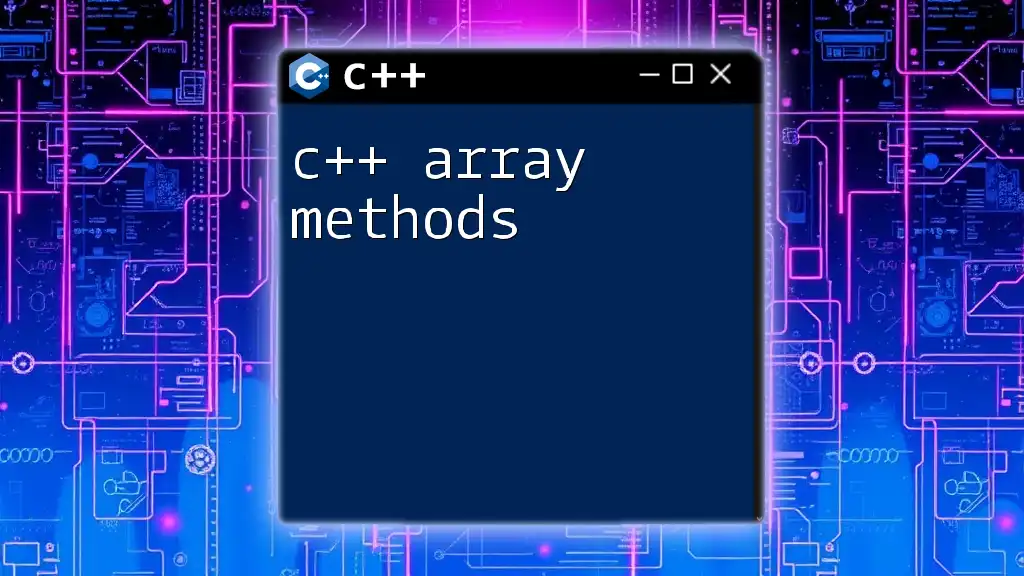
Validating Command Line Arguments
Importance of Argument Validation
Validating command line arguments is crucial for ensuring your program behaves as expected and prevents errors due to invalid input. It’s essential to check if the number of arguments is correct and if the given inputs meet the expected criteria.
Implementing Basic Validation in C++
Here’s a code snippet demonstrating basic validation:
#include <iostream>
int main(int argc, char* argv[]) {
if (argc != 3) {
std::cerr << "Error: You must provide exactly two arguments." << std::endl;
return 1;
}
// Further code processing
std::cout << "Arguments received: " << argv[1] << " and " << argv[2] << std::endl;
return 0;
}
Here, the program checks if exactly two arguments are supplied. If not, it returns an error message.
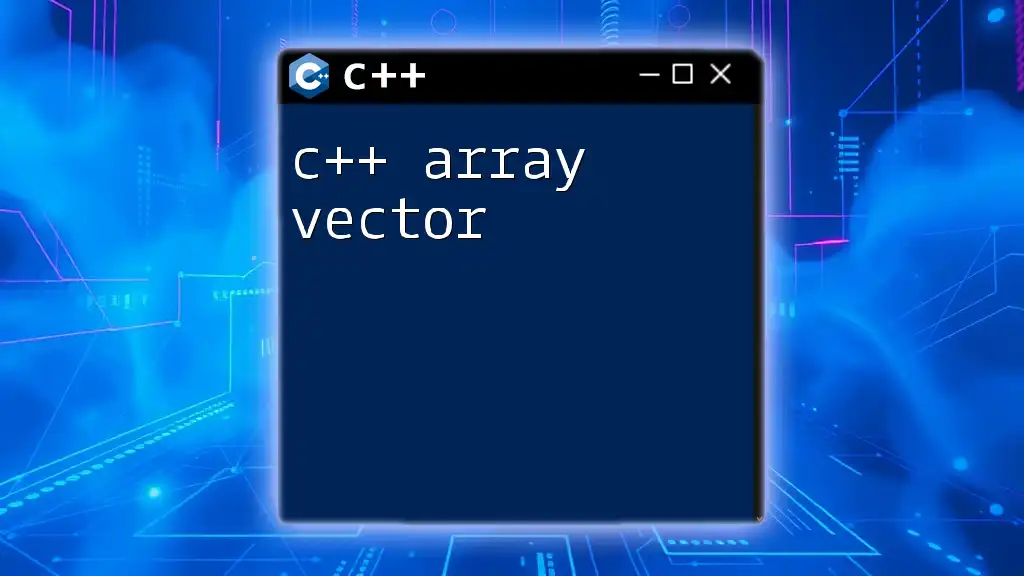
Advanced Usage of Command Line Arguments
Passing Flags and Options
Command line arguments can also include flags or options that modify the program's behavior. For instance, you might allow users to pass a `-h` or `--help` option to display usage information.
#include <iostream>
int main(int argc, char* argv[]) {
if (argc > 1 && (strcmp(argv[1], "-h") == 0 || strcmp(argv[1], "--help") == 0)) {
std::cout << "Usage: " << argv[0] << " [options] [arguments]" << std::endl;
return 0;
}
// Main program logic
return 0;
}
This code checks if the user requested help and displays it accordingly.
Parsing Command Line Arguments
For more complex argument parsing, libraries such as `getopt` can simplify the process. Using `getopt`, you can handle flags, options, and their values elegantly.
Example using `getopt`:
#include <iostream>
#include <unistd.h>
int main(int argc, char* argv[]) {
int option;
while ((option = getopt(argc, argv, "h")) != -1) {
switch (option) {
case 'h':
std::cout << "Usage: " << argv[0] << " [options]" << std::endl;
return 0;
default:
std::cerr << "Unknown option" << std::endl;
return 1;
}
}
// Main program logic
return 0;
}
This piece of code processes options, allowing for easier management of user inputs.
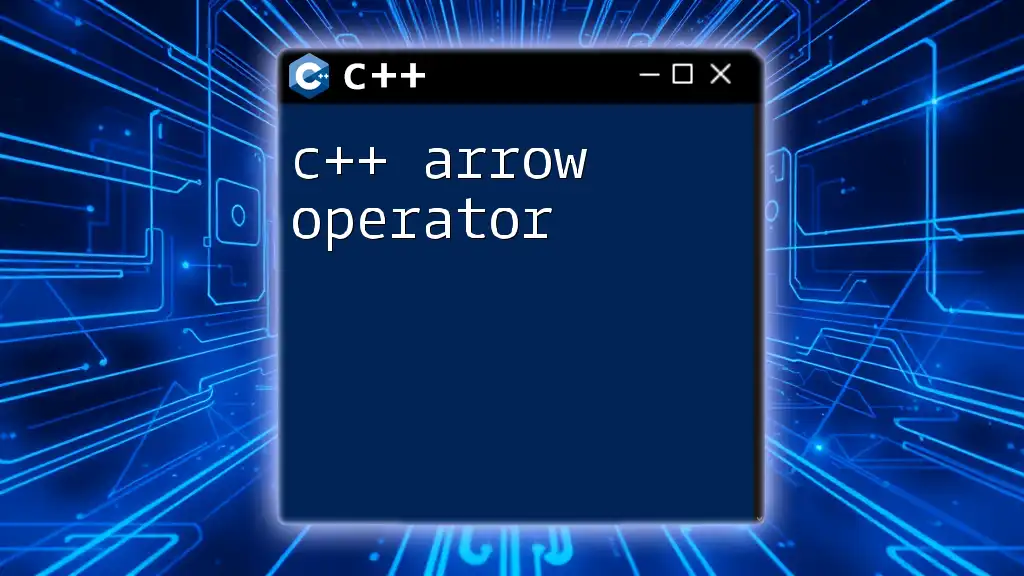
Performance Considerations
Understanding Impact of Command Line Arguments on Performance
The performance impact of command line arguments is usually negligible but can vary based on how many arguments are passed and how complex the processing is. While accessing arguments themselves is efficient, extensive manipulation or validation can impact performance, especially in large applications.
When to Use Command Line Arguments
Command line arguments are excellent for configurations and options. However, for applications where user interaction is more complex, consider graphical user interfaces (GUIs) instead. Striking a balance between usability and input methods is vital for a good user experience.
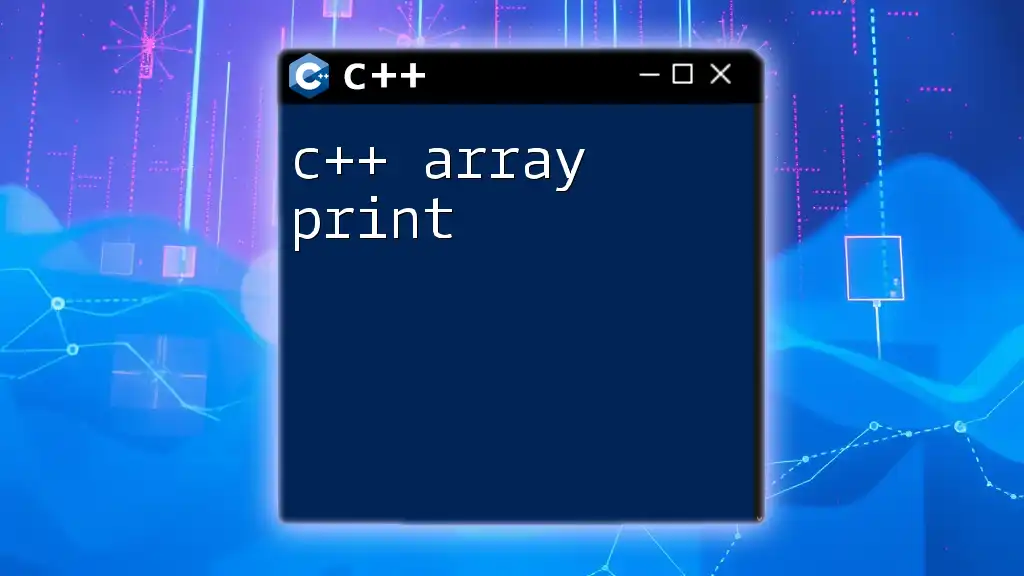
Conclusion
Command line arguments are an essential aspect of C++ programming, providing a dynamic way to enhance user interaction. By understanding `argc` and `argv`, you can create flexible applications that adapt to user needs. With proper validation and advanced parsing techniques, your programs can effectively utilize these inputs to achieve diverse functionalities.
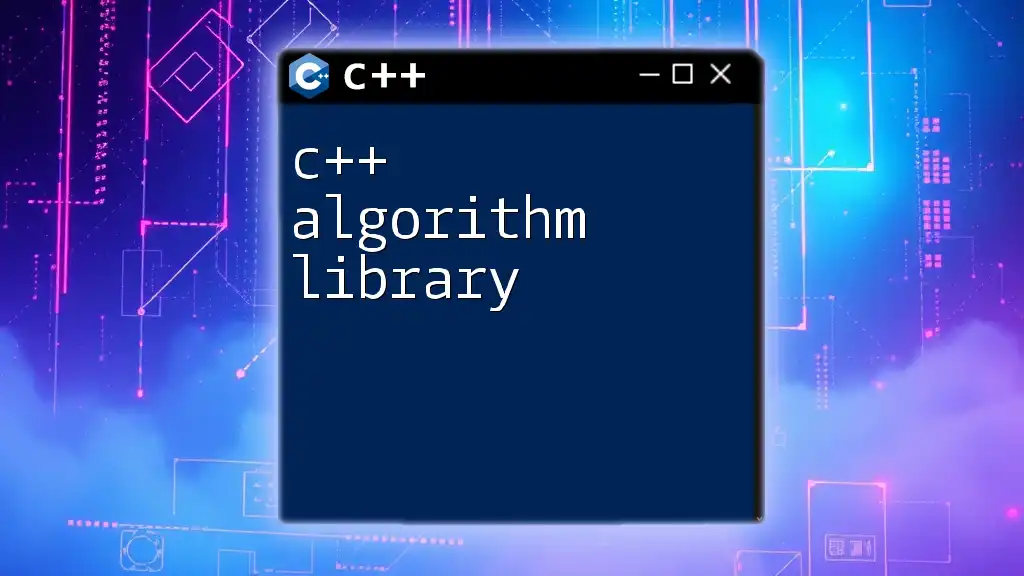
Additional Resources
To further your knowledge on using command line arguments in C++, consider exploring specialized books, online tutorials, and programming courses. Online tools and IDEs can also provide immediate feedback while you experiment with `c++ arg` functionality in your programs.