In C++, you can split a string by a delimiter using the `std::stringstream` class along with the `getline` function. Here's an example:
#include <iostream>
#include <sstream>
#include <vector>
std::vector<std::string> splitString(const std::string& str, char delimiter) {
std::vector<std::string> tokens;
std::stringstream ss(str);
std::string token;
while (std::getline(ss, token, delimiter)) {
tokens.push_back(token);
}
return tokens;
}
int main() {
std::string str = "apple,banana,cherry";
char delimiter = ',';
std::vector<std::string> result = splitString(str, delimiter);
for (const auto& s : result) {
std::cout << s << std::endl;
}
return 0;
}
Understanding Strings in C++
What is a string in C++?
In C++, a string is represented by the `std::string` class, which is part of the standard library. This class provides a way to store and manipulate sequences of characters, enabling developers to work with text efficiently. Unlike traditional C-style strings, which are arrays of characters terminated by a null character, `std::string` automatically handles memory allocation and offers a rich set of functions for string manipulation.
Why Split Strings?
Splitting strings is a fundamental operation in many applications. Whether you are parsing data files, processing user input, or manipulating data structures, the ability to separate a single string into multiple components is crucial. Common use cases include extracting fields from CSV files, breaking text into words, or processing commands entered by users.
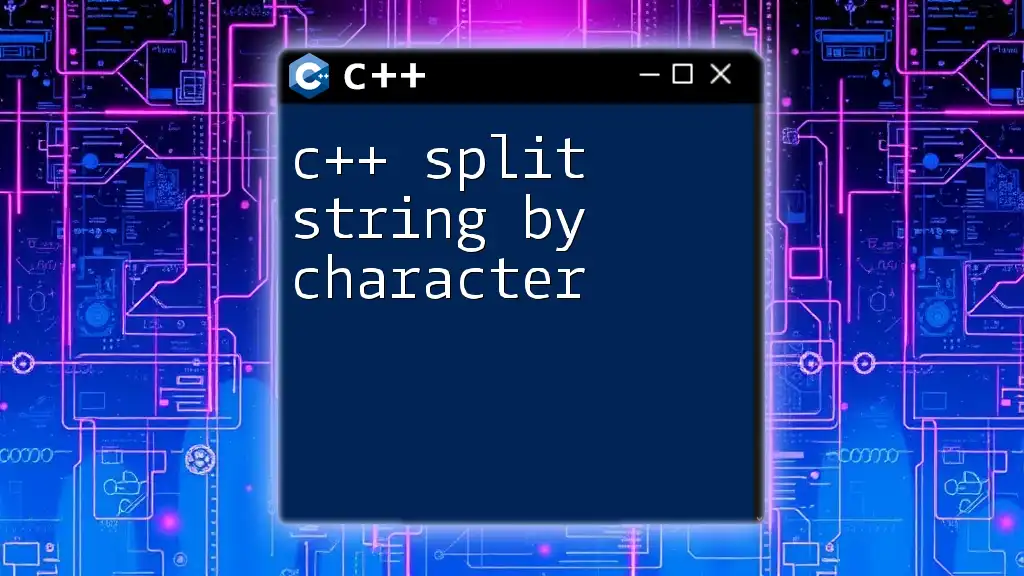
The Concept of Delimiters
What is a delimiter?
A delimiter is a character or sequence of characters that marks the beginning or end of a string segment. In programming, delimiters define where one piece of data ends and another begins. Common delimiters include commas, spaces, semicolons, and tabs.
Common Delimiters
- Comma (`,`): Primarily used in CSV (Comma-Separated Values) formatting.
- Space (` `): Commonly employed to separate words in sentences.
- Semicolon (`;`): Often used in configuration files or as a separator in various data formats.
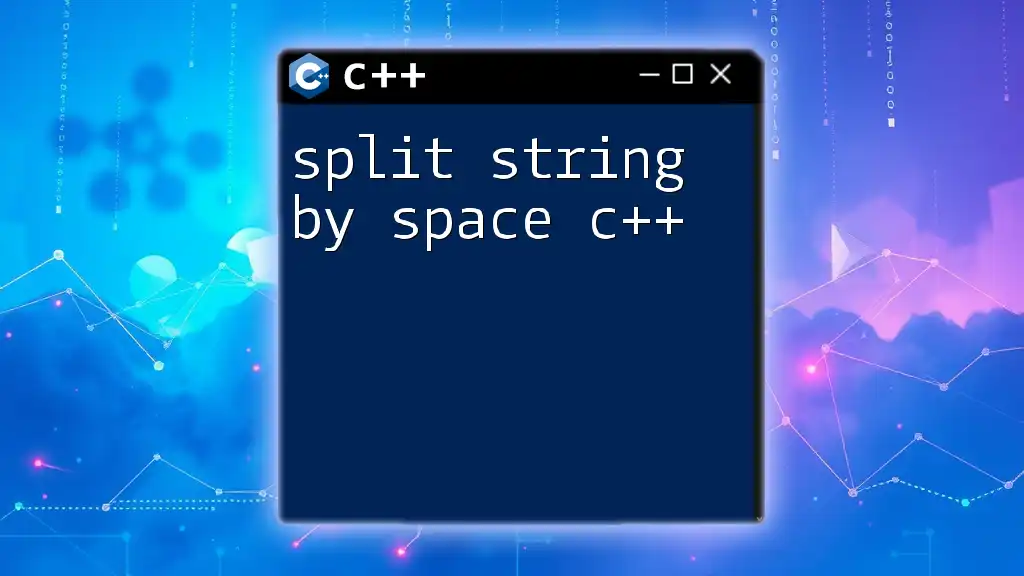
How to Split a String in C++
Using the `std::string::find` and `std::string::substr` methods
One effective way to split a string is by leveraging the member functions `find` and `substr`. These methods allow you to locate the position of delimiters and extract substrings accordingly.
Here’s a practical implementation of string splitting using these functions:
#include <iostream>
#include <sstream>
#include <vector>
std::vector<std::string> splitString(const std::string &str, char delimiter) {
std::vector<std::string> tokens;
std::string token;
std::istringstream tokenStream(str);
while (std::getline(tokenStream, token, delimiter)) {
tokens.push_back(token);
}
return tokens;
}
Code Example: Basic String Split
Here’s an example demonstrating how to use the aforementioned function to split a string.
int main() {
std::string str = "apple,banana,cherry";
std::vector<std::string> result = splitString(str, ',');
for (const auto& s : result) {
std::cout << s << std::endl;
}
return 0;
}
When you run the above code, it will output:
apple
banana
cherry
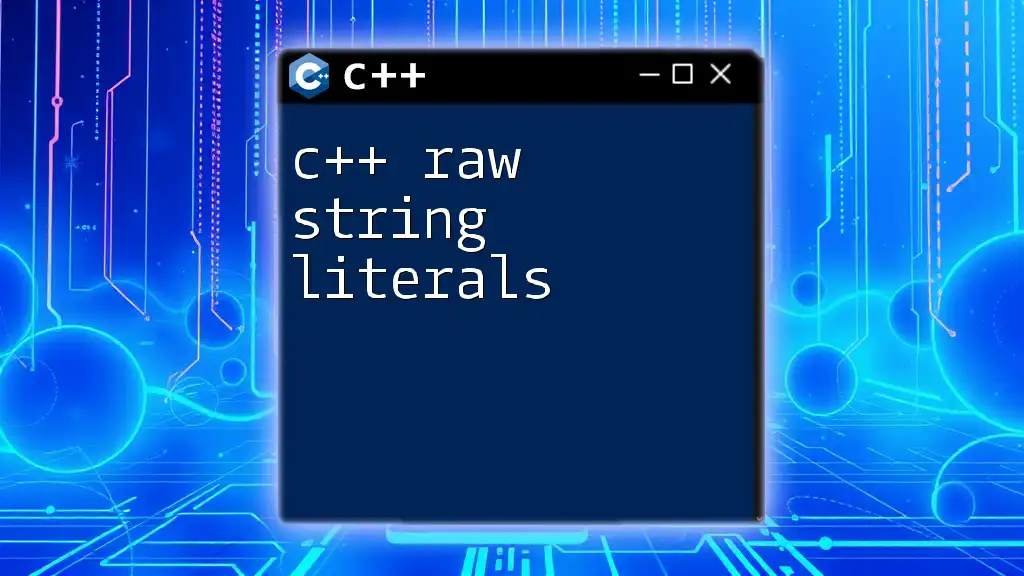
Parsing Strings with Multiple Delimiters
Using `std::regex` for Advanced Splitting
For more complex scenarios where you may need to split a string by multiple delimiters, regular expressions can be exceptionally powerful. They allow for sophisticated string parsing capabilities.
Here’s how you can implement a function that utilizes `std::regex` to split a string:
#include <iostream>
#include <sstream>
#include <vector>
#include <regex>
std::vector<std::string> splitStringRegex(const std::string &str, const std::string &delimiters) {
std::regex re("[" + delimiters + "]");
return std::vector<std::string>(std::sregex_token_iterator(str.begin(), str.end(), re, -1),
std::sregex_token_iterator());
}
Code Example: Splitting with Multiple Delimiters
Consider the following complete example that allows splitting a string using various delimiters:
int main() {
std::string str = "apple;banana orange,grape;kiwi";
std::vector<std::string> result = splitStringRegex(str, "; ,");
for (const auto& s : result) {
std::cout << s << std::endl;
}
return 0;
}
Upon execution, this will result in:
apple
banana
orange
grape
kiwi
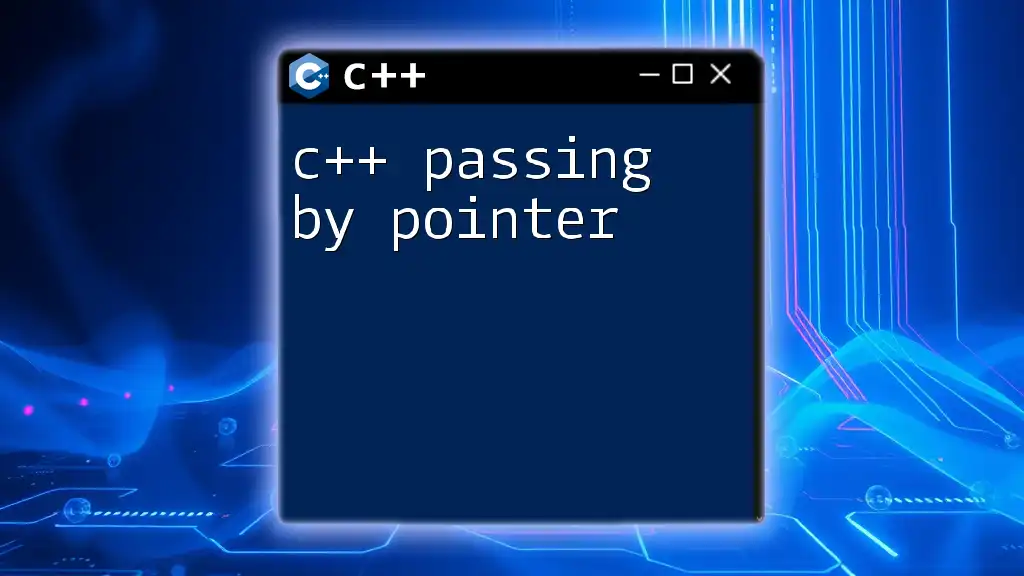
Common Use Cases of Splitting Strings
Data Parsing in CSV Format
The most common application of splitting strings is parsing CSV files. Each line in a CSV file generally contains data fields separated by commas. By employing the `cpp split string by delimiter` strategy, developers can easily extract each field for further processing.
User Input Handling
When users input data, particularly in command-line applications, it’s common to split the input string to differentiate between commands and arguments. This splitting helps in interpreting user intentions accurately.
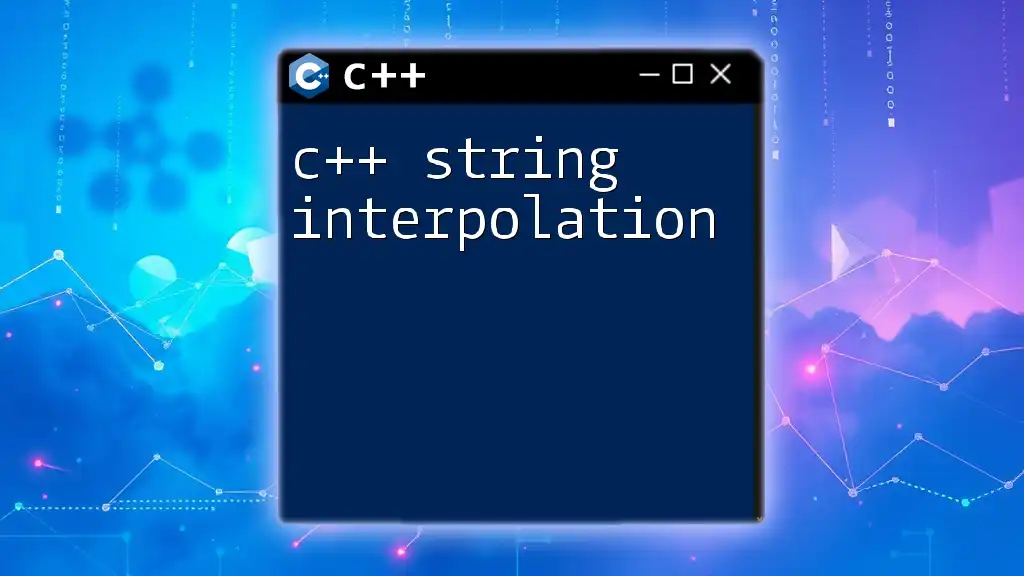
Best Practices for String Splitting
Considerations on Performance
Efficiency is a crucial factor when splitting large strings or processing large datasets. Utilize optimized algorithms and data structures to minimize overhead. In cases of high-volume string processing, consider using `std::regex` with caution as it may introduce performance penalties compared to simpler methods.
Handling Empty Tokens
When working with strings that may have adjacent delimiters or delimiters at the start or end, it’s essential to handle empty tokens gracefully. Implement checks in your splitting logic to either include or exclude empty strings based on the application’s needs.
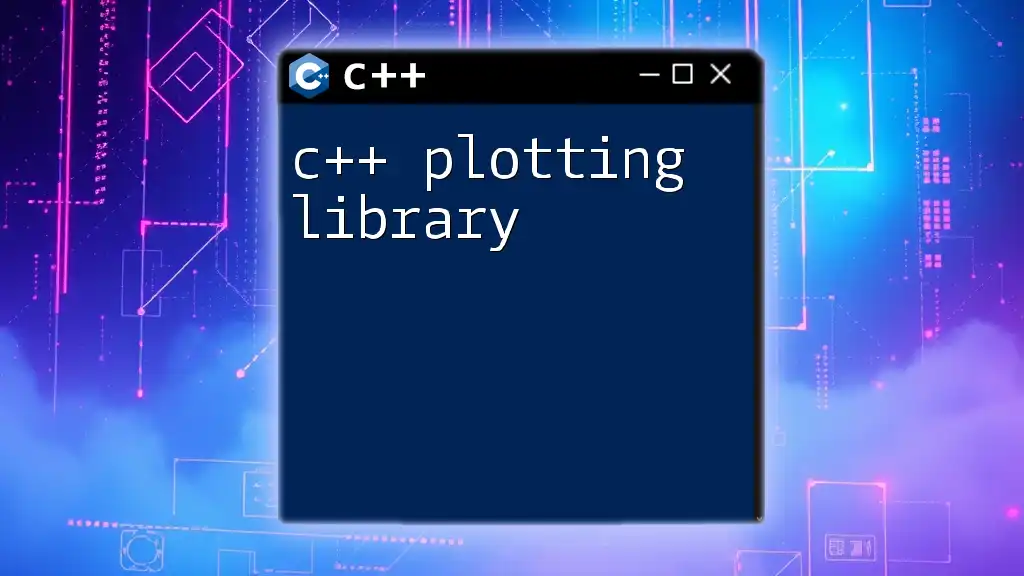
Conclusion
In summary, mastering the ability to `cpp split string by delimiter` is indispensable for any C++ developer. By leveraging the provided techniques and understanding the use of delimiters, you can enhance your string manipulation skills substantially. Practice with various examples and scenarios to gain a deeper understanding of string parsing in C++.