In C++, the `std::min` and `std::max` functions are used to determine the smaller or larger of two values, respectively.
Here’s a code snippet demonstrating their usage:
#include <iostream>
#include <algorithm> // Needed for std::min and std::max
int main() {
int a = 5, b = 10;
std::cout << "Min: " << std::min(a, b) << std::endl; // Output: Min: 5
std::cout << "Max: " << std::max(a, b) << std::endl; // Output: Max: 10
return 0;
}
Understanding the Basics of Min and Max
What are Min and Max?
In programming, minimum and maximum values are crucial for comparisons and decision-making processes. The min function is used to determine the smallest value among its parameters, while the max function identifies the largest value. These functions play a significant role in various algorithms, especially in scenarios that require sorting, searching, and optimizing values.
Syntax of Min and Max Functions in C++
In C++, the `std::min` and `std::max` functions are defined in the `<algorithm>` header. They utilize a simple and intuitive syntax:
#include <algorithm>
int a = 5;
int b = 10;
int minimum = std::min(a, b); // returns 5
int maximum = std::max(a, b); // returns 10
These functions can take two parameters of the same type and return the minimum or maximum value, respectively.

The Power of Templates in Min and Max Functions
How Min and Max Functions Work with Different Data Types
C++ leverages templates to allow `std::min` and `std::max` to function with various data types. This means you can apply these functions on integers, floats, characters, or user-defined types without additional overloads. Consider the following example:
double x = 3.14;
double y = 2.71;
double minDouble = std::min(x, y); // returns 2.71
double maxDouble = std::max(x, y); // returns 3.14
This flexibility is a powerful feature of C++, allowing for code reusability and generalization.
Handling User-Defined Data Types
In some cases, you may need to find the minimum or maximum of custom data types, such as classes or structs. This can be achieved by overloading the corresponding operators. Here’s an example using a `Point` struct:
struct Point {
int x, y;
bool operator<(const Point& other) const {
return (x * x + y * y) < (other.x * other.x + other.y * other.y);
}
};
Point p1{3, 4};
Point p2{1, 1};
Point minPoint = std::min(p1, p2); // returns Point(1, 1)
Point maxPoint = std::max(p1, p2); // returns Point(3, 4)
In this example, the `<` operator is overloaded to compare points based on their distance from the origin, showcasing how you can tailor comparisons to suit your needs.
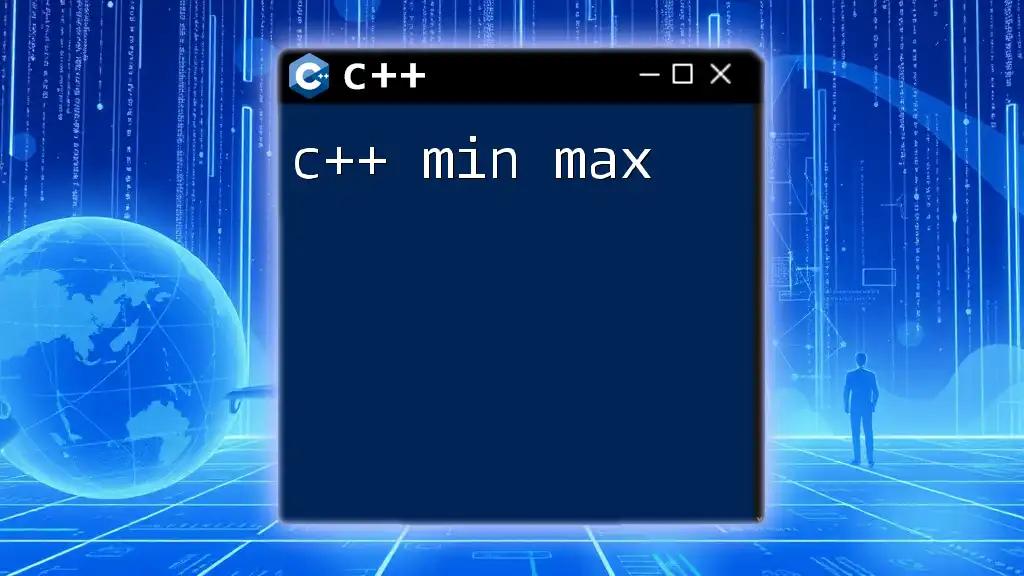
Using Min and Max with Arrays and Containers
Finding Min and Max in Arrays
When working with arrays, using `std::min_element` and `std::max_element` functions can efficiently find the minimum or maximum value. Here’s how you can do it with a C-style array:
#include <algorithm>
int arr[5] = {3, 1, 4, 2, 5};
int minArray = *std::min_element(arr, arr + 5); // returns 1
int maxArray = *std::max_element(arr, arr + 5); // returns 5
These functions traverse the specified range within the array to determine the minimum or maximum, providing an efficient way to handle collections of values.
Using Min and Max with STL Containers
C++ Standard Template Library (STL) containers like `std::vector` or `std::list` can also utilize these functions to find minimum and maximum values seamlessly. Here is an example using `std::vector`:
#include <vector>
#include <algorithm>
std::vector<int> vec = {10, 20, 30, 25, 15};
int minVec = *std::min_element(vec.begin(), vec.end()); // returns 10
int maxVec = *std::max_element(vec.begin(), vec.end()); // returns 30
Leveraging STL functionalities enhances code clarity and readability while optimizing performance.
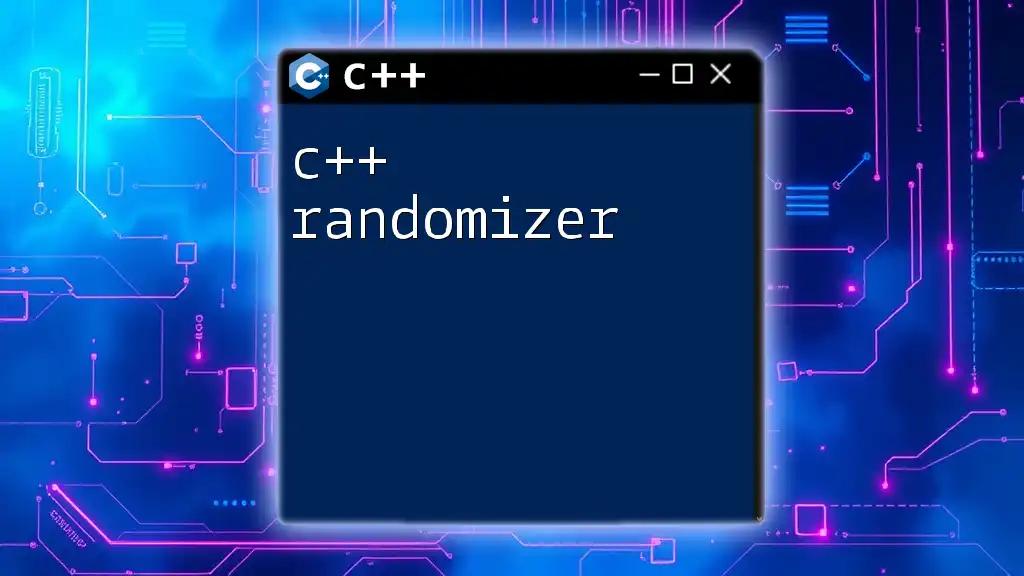
Custom Min and Max Function Implementations
Writing Your Own Min and Max Functions
While the built-in `std::min` and `std::max` are convenient, there are cases where custom implementations may offer added flexibility or specificity. Creating your own versions can improve code readability or optimize performance for particular scenarios. Here’s how:
template<typename T>
T customMin(T a, T b) {
return (a < b) ? a : b;
}
template<typename T>
T customMax(T a, T b) {
return (a > b) ? a : b;
}
Using templates allows these functions to accept any data type, making them versatile and reusable across your codebase.
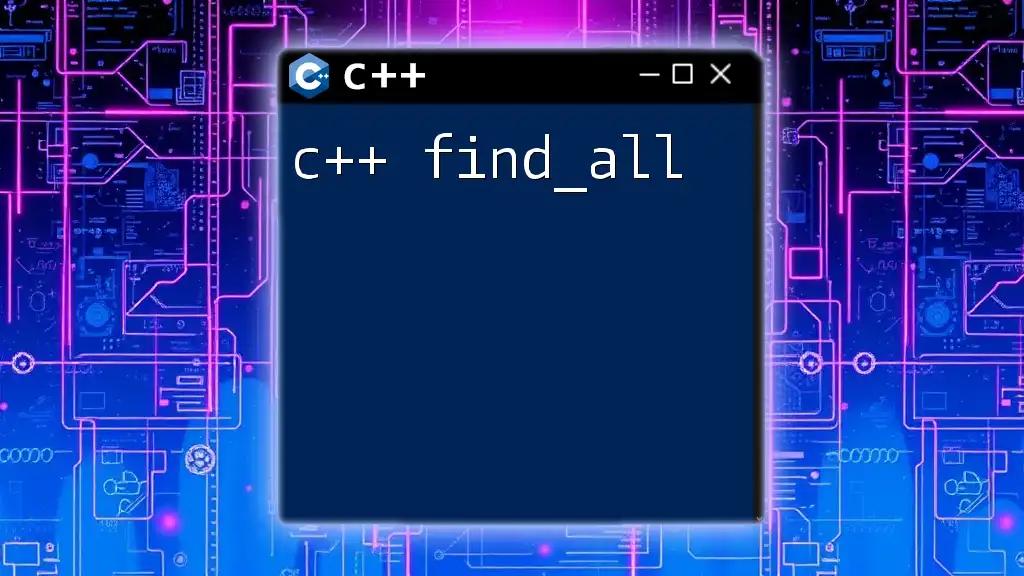
Performance Considerations
Complexity of Different Approaches
The time complexity for using `std::min` and `std::max` with single comparisons is O(1). However, when iterating through arrays or collections, the complexity becomes O(n), where `n` is the number of elements in the dataset. Understanding these complexities can help you decide when to use these functions in performance-sensitive applications.
Avoiding Edge Cases
When dealing with multiple elements that may share minimum or maximum values, ensure that your program logic can handle these situations effectively. For example, if two or more elements share the same maximum value, your logic must account for how to deal with the duplicates, depending on the specific requirements of your application.
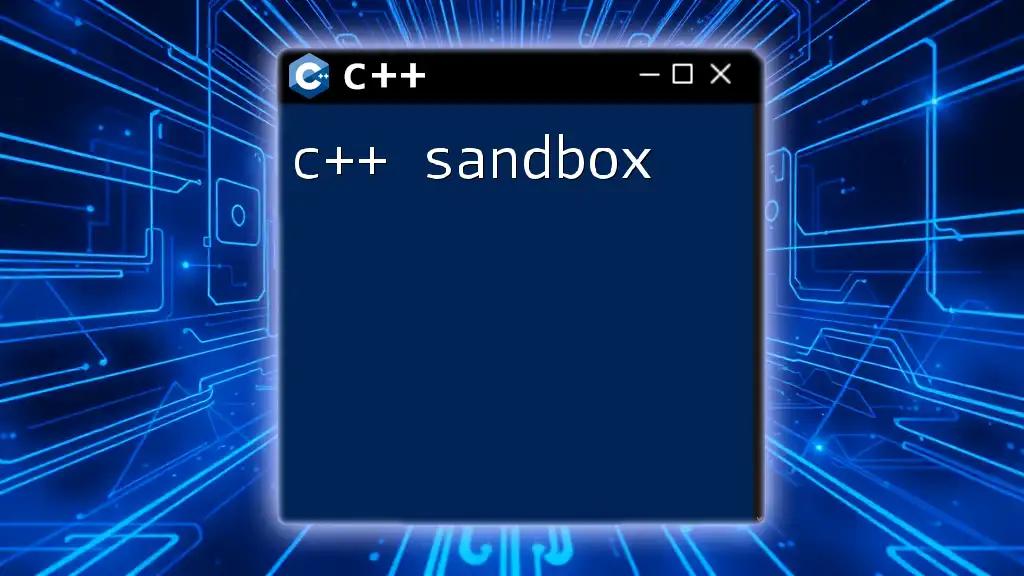
Conclusion
In summary, understanding how to utilize C++ min and max functions can significantly enhance your programming capabilities. These functions simplify comparisons and enable developers to create more efficient and maintainable code. Practice implementing these concepts within your personal projects to master their use in real-world scenarios.
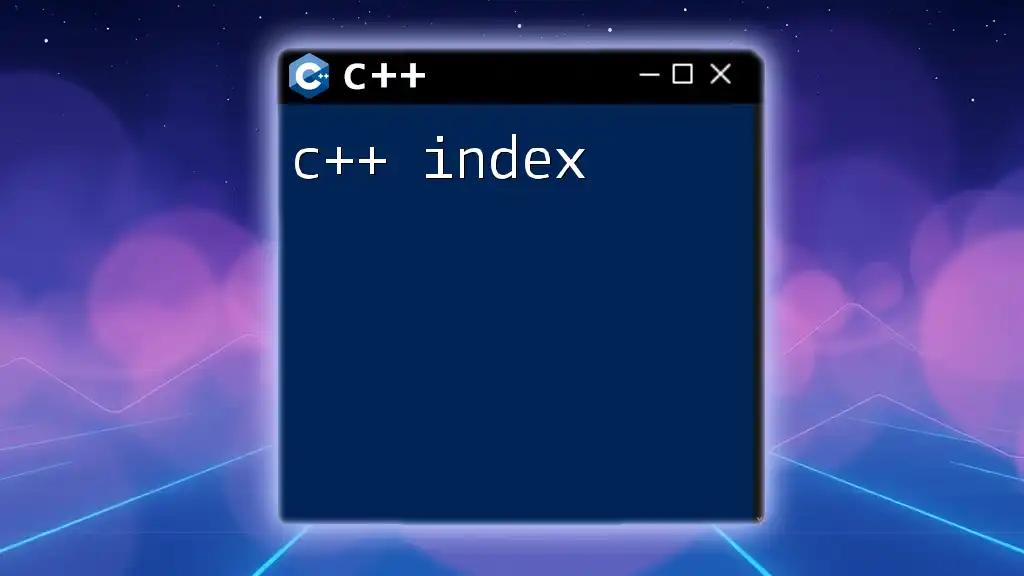
Additional Resources
For further reading on C++ standard library functions, refer to the official documentation. Additionally, exploring books such as "C++ Primer" by Lippman and "Effective C++" by Scott Meyers can provide deeper insights. Online tutorials, courses, and community forums are also great resources for mastering C++ concepts.
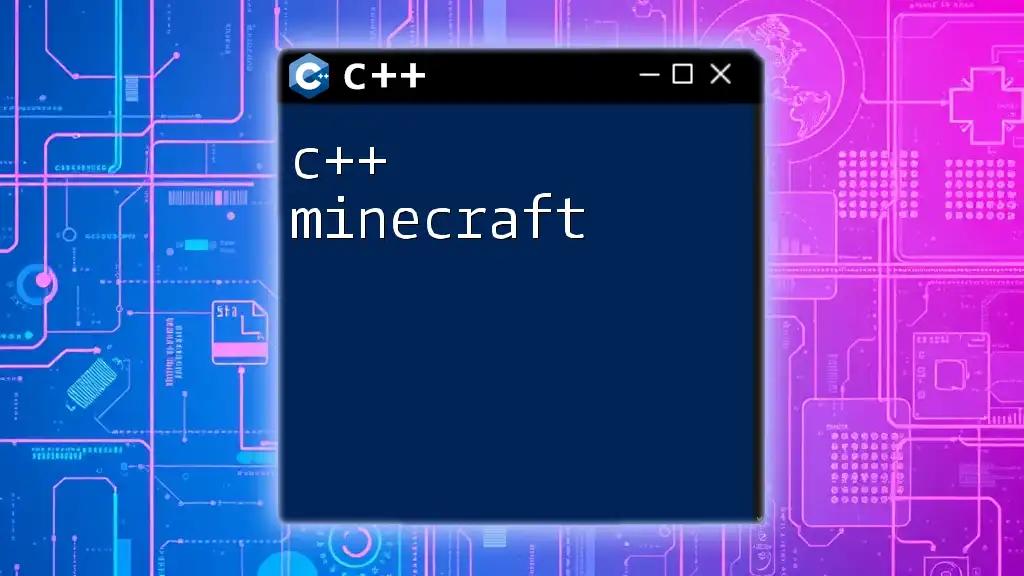
FAQs
What is the difference between min and max in C++ vs other programming languages?
Different programming languages implement their min and max functions uniquely, but the overall concept remains similar across languages. The syntax and additional features may vary based on language paradigms.
Can I use min and max with strings in C++?
Yes, `std::min` and `std::max` can be used with strings in C++. Comparing strings follows lexicographical order, similar to how alphabetical sorting works.
Why should I prefer std::min/std::max over writing my own?
Using `std::min` and `std::max` promotes code consistency and leverages the STL’s optimizations. Built-in functions have been extensively tested and provide a reliable solution without reinventing the wheel.