In C++, a function can return a value using the `return` statement, which allows the caller of the function to receive the output of the computation performed within the function.
int add(int a, int b) {
return a + b;
}
Understanding Function Return Values in C++
What is a Function Return Value?
A function return value in C++ is the value that a function sends back to the caller when it completes its execution. This return can be of any data type, including primitive types like integers and floats, as well as complex types such as objects and structures.
Function return values are essential for:
- Data Flow: Enabling the transfer of results between functions.
- Modularity: Breaking code into smaller, manageable sections that enhance readability.
- Error Handling: Providing a systematic way to manage errors by returning specific codes or messages.
Why Use Return Values?
Using return values offers several advantages:
- Facilitates data flow by allowing functions to output results directly to wherever they are called.
- Improves modularity and readability by separating logic into discrete parts.
- Enables effective error handling by using return values as indicators of success or failure.
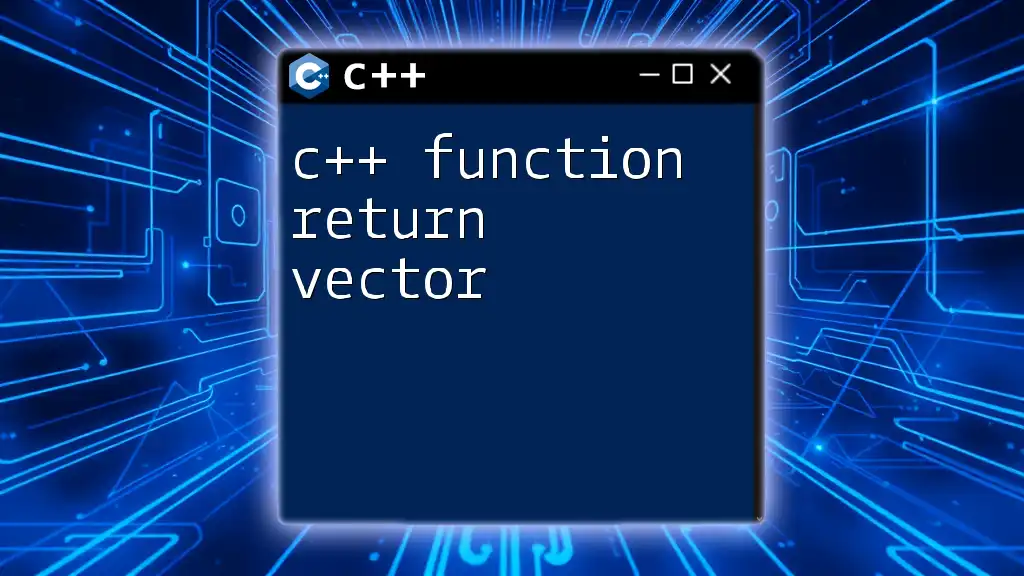
Types of Return Values
Basic Data Types
Integer Return Values
Returning integers is straightforward, and it is commonly used for calculations. Here’s an example:
int add(int a, int b) {
return a + b;
}
In this snippet, the function `add` takes two integers as parameters and returns their sum as an integer.
Floating-point Return Values
For calculations that require precision, such as in scientific computing, floating-point values are essential:
double calculateArea(double radius) {
return 3.14159 * radius * radius;
}
Here, the function `calculateArea` computes the area of a circle and returns it as a `double`.
Character and String Return Values
Functions can also return characters and strings, which are vital for text processing:
char getInitial(char firstNameInitial) {
return firstNameInitial;
}
std::string greet(std::string name) {
return "Hello, " + name + "!";
}
In these examples, the first function returns a single character, while the second returns a greeting message.
Complex Return Types
Returning Structures and Classes
C++ allows functions to return structures and objects, which is a powerful feature for data encapsulation:
struct Point {
int x;
int y;
};
Point createPoint(int x, int y) {
Point p;
p.x = x;
p.y = y;
return p;
}
The `createPoint` function initializes a `Point` structure and returns it, showcasing how to handle complex types.
Returning Pointers
Returning pointers can be efficient, especially for large data structures to avoid copying overhead:
int* createArray(int size) {
return new int[size];
}
In this example, the function creates an array dynamically and returns a pointer to it.
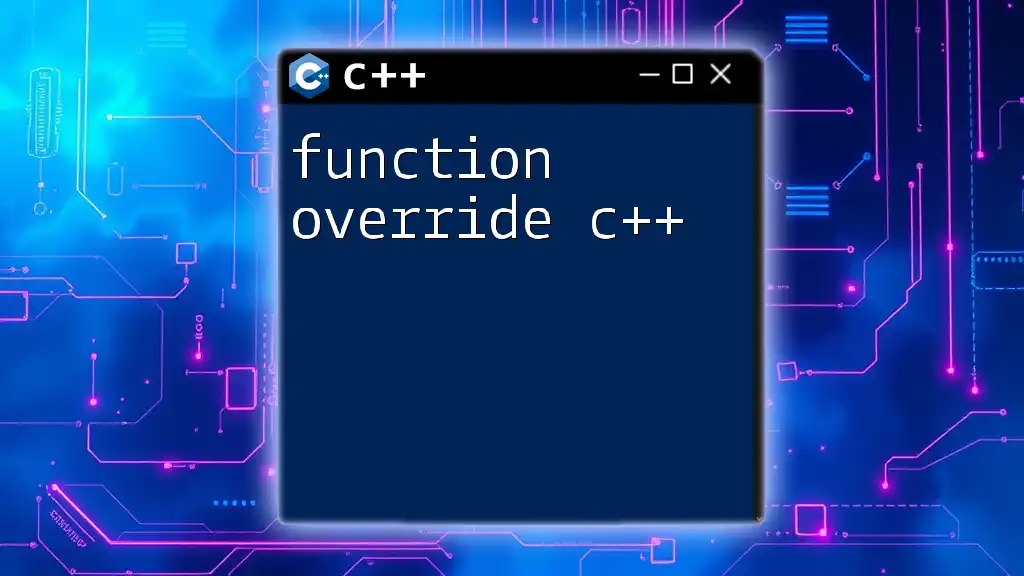
Return Value Syntax in C++
Basic Syntax Breakdown
A function's return type is declared at its beginning, followed by the function name and parameters. The return statement must match the declared return type:
double divide(double numerator, double denominator) {
if (denominator != 0) {
return numerator / denominator;
} else {
return 0; // Or handle the error appropriately
}
}
Using Return in Different Contexts
When calling functions, the context may dictate the use of return values differently. For instance, in nested functions, return values can be passed through several layers of calls.
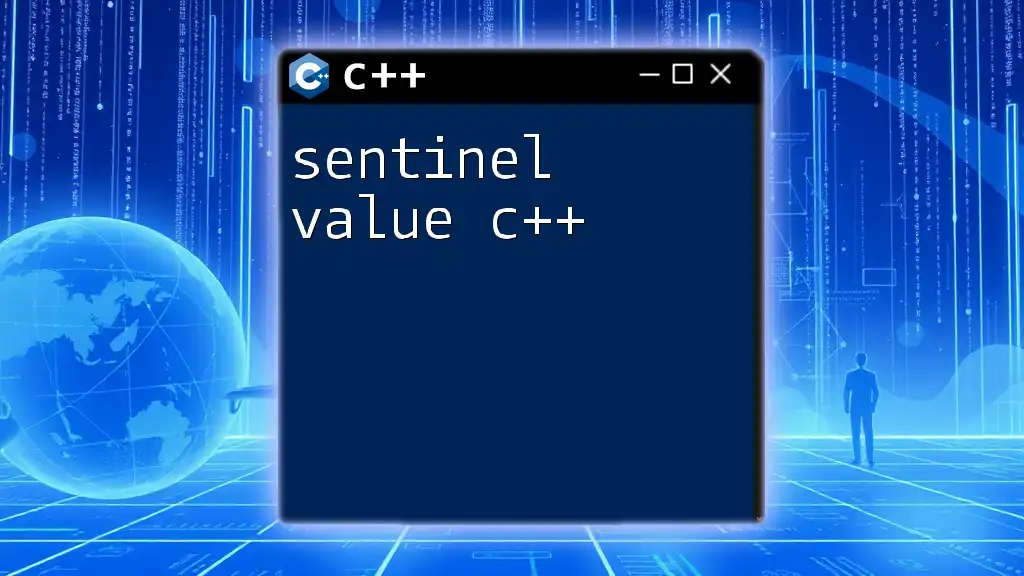
Returning Multiple Values in C++
Using Standard Structures
To return multiple values, consider using `std::tuple` from the Standard Library:
#include <tuple>
std::tuple<int, double> getStatistics() {
return std::make_tuple(5, 12.5);
}
Here, the `getStatistics` function returns both an integer and a double wrapped in a tuple.
Using Output Parameters
Another way to retrieve multiple values is via output parameters, which are passed by reference:
void getCoordinates(int& x, int& y) {
x = 10;
y = 20;
}
In this case, `getCoordinates` modifies the parameters passed by reference to output multiple results.
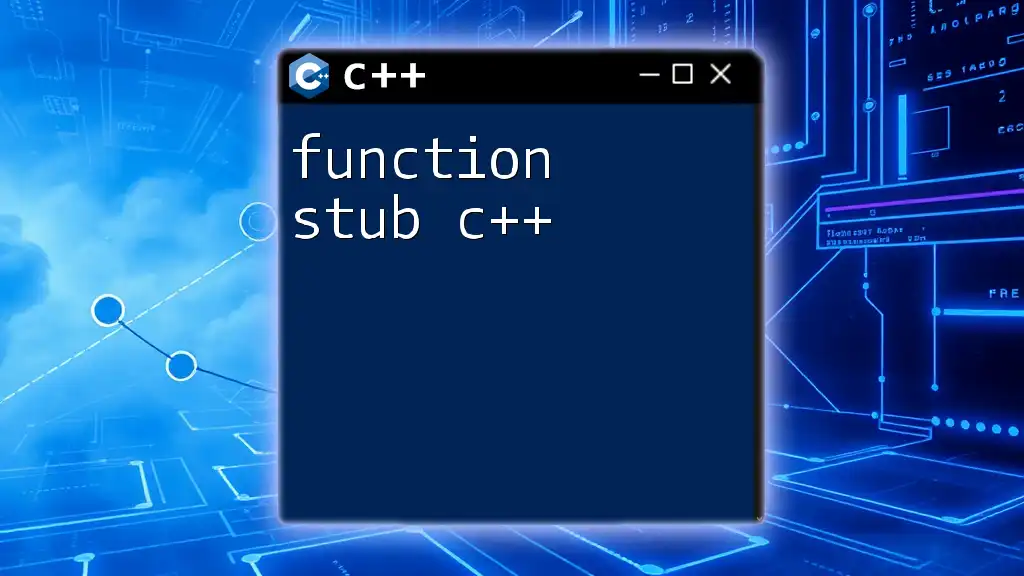
Common Mistakes with Return Values
Forgetting Return Statements
One of the most frequent errors is neglecting to include a return statement:
int getValue() {
// Missing return statement
}
This leads to compilation errors, reinforcing the necessity of always returning a value when expected.
Returning Local Variables
Returning a reference to a local variable can cause undefined behavior because the variable goes out of scope:
int* returnPointer() {
int localVariable = 5;
return &localVariable; // Dangerous! Local variable destroyed.
}
Instead, return using dynamic allocation, or better yet, avoid returning pointers to local variables entirely.
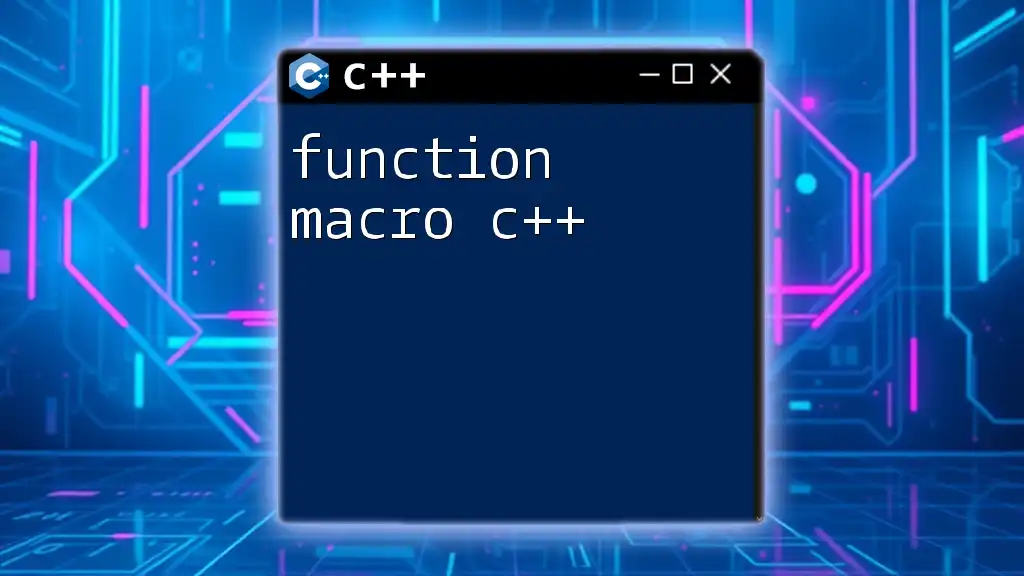
Best Practices for Handling Return Values
Consistent Return Types
Consistency in return types is critical for maintainability. Clearly document what your functions return and ensure that they are consistent throughout.
Error Handling Through Return Values
Leverage return values to indicate success or error states:
int divide(int numerator, int denominator) {
if (denominator == 0) {
return -1; // Error code for division by zero
}
return numerator / denominator;
}
This method allows calling functions to recognize and handle potential errors.
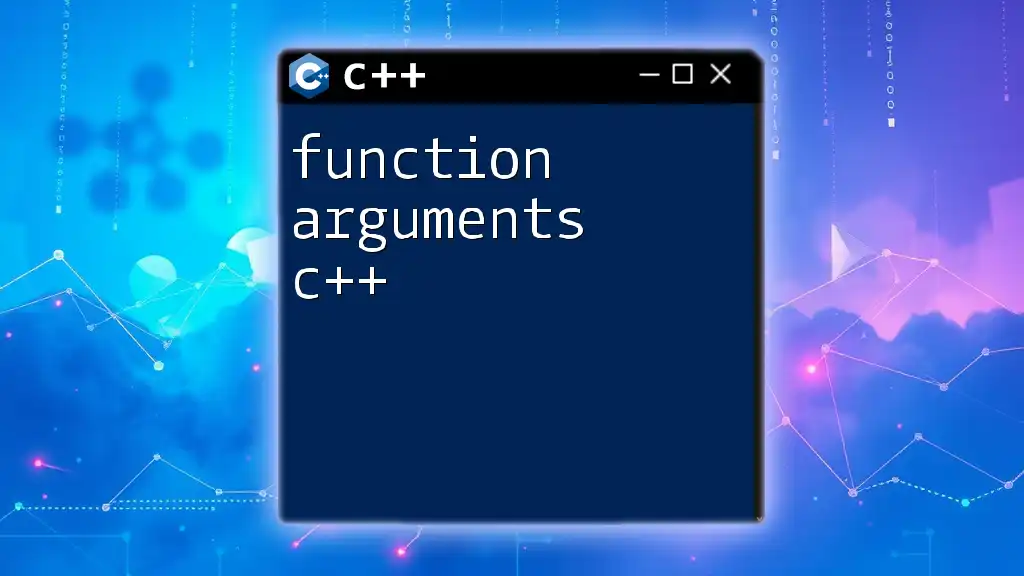
Advanced Topics
Return by Value vs. Return by Reference
When considering return by value and return by reference, it’s important to understand their distinct implications:
- Return by Value creates a copy, ideal for small data sizes and ensuring data integrity.
- Return by Reference avoids copying but does so at the risk of exposing internal state changes or leading to dangling references.
Lambda Functions and Return Types
Lambda functions have become increasingly popular. They provide a powerful shorthand for defining anonymous functions. Here's how they manage return values:
auto multiply = [](double a, double b) {
return a * b; // Implicit deduction of return type
};
The function defined above implicitly deduces its return type based on the return expression.
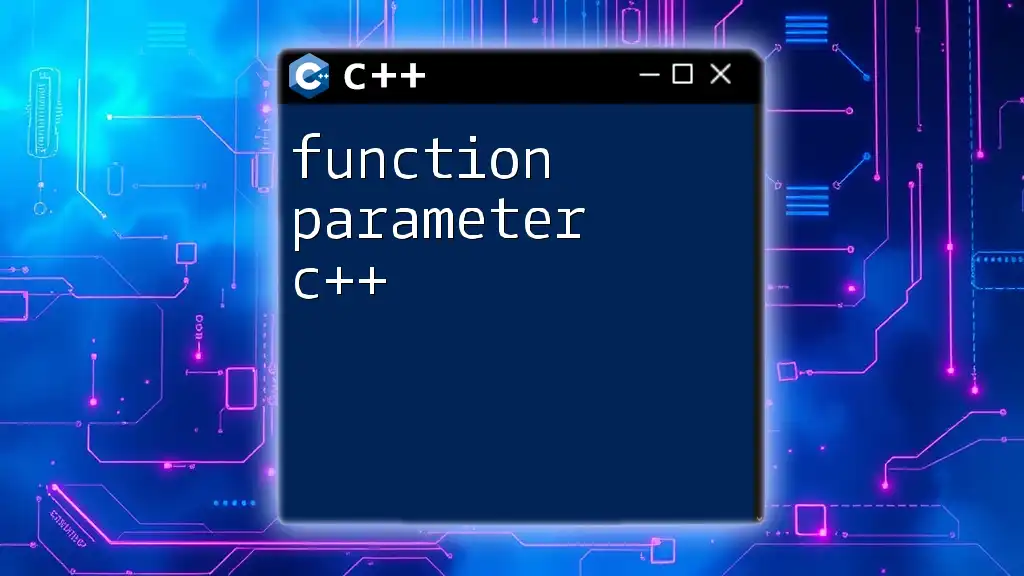
Conclusion
Understanding function return values in C++ is fundamental for writing effective, modular code. From basic data types to complex structures, mastering return values allows you to communicate results efficiently between functions and handle errors gracefully. By applying best practices and recognizing common pitfalls, you can enhance your programming skills and create robust applications with C++.