In C++, a declaration introduces a name and its type to the program without allocating storage, while a definition both introduces the name and allocates storage (if needed); for example, declaring a variable and then defining it would look like this:
// Declaration
extern int a;
// Definition
int a = 5;
Understanding C++ Declarations
What is a C++ Declaration?
A C++ declaration introduces a name and its type without allocating memory for the variable or object. It's primarily used to let the compiler know what a variable or function is supposed to represent, but it does not define it. This is crucial for enabling code organization across different files.
For instance, the following line is a declaration:
extern int myVariable; // Declaration of myVariable
In this example, `myVariable` is declared as an integer, but its storage is not allocated at this point. Instead, it informs the compiler that `myVariable` exists and that it will be defined later.
Characteristics of Declarations
Declarations have some distinctive features that set them apart:
- No Memory Allocation: A declaration tells the compiler the type of the variable, but it does not allocate memory for it.
- Specifies Type but Not Storage: A declaration confirms that a variable or function exists, but it doesn't provide the storage or implementation details.
Consider the following example of a function declaration:
void myFunction(); // Declaration of the function
Here, `myFunction()` is declared, indicating to the compiler its existence without providing any implementation or allocating memory.
Common Uses of Declarations
Declarations are frequently used in various contexts, including:
- Global Variables: When you want a variable accessible across multiple files, you can declare it using `extern`.
- Function Prototypes: Before you define a function, declaring it allows other functions to call it.
For example, in a header file, you may have:
// header.h
extern int myGlobalVar; // Declaration
This declaration allows any source file that includes `header.h` to access `myGlobalVar`, without needing to know its definition right away.
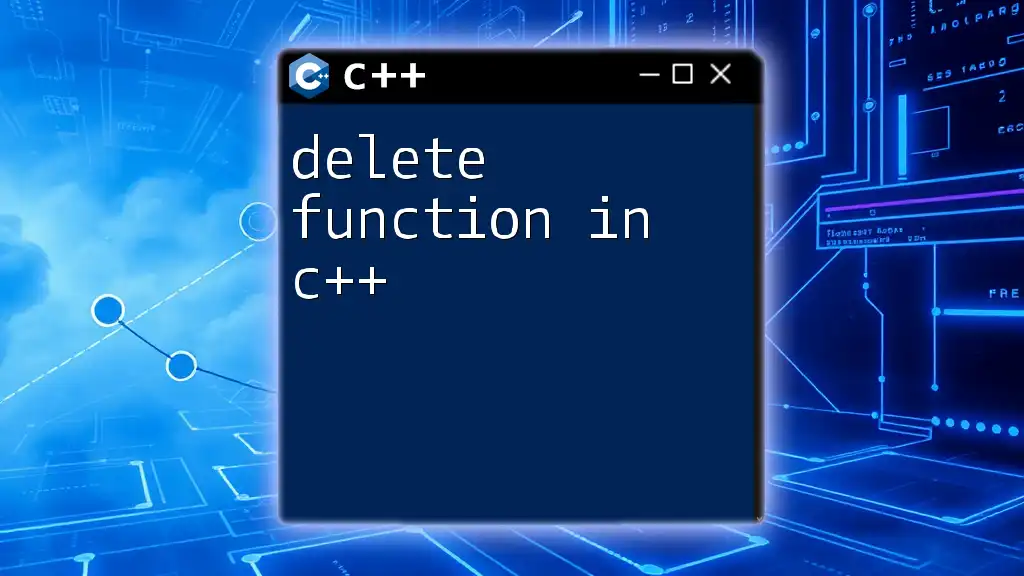
Understanding C++ Definitions
What is a C++ Definition?
In contrast to a declaration, a C++ definition not only introduces a name but also allocates memory for it. It provides the implementation necessary for variables, functions, and objects. A definition includes both the type and the actual value assigned or the complete function body.
For instance, the following line represents a definition:
int myVariable = 5; // Definition and initialization of myVariable
Here, `myVariable` is not only declared to be an integer, but memory is allocated for it, and it is initialized with the value `5`.
Characteristics of Definitions
Definitions come with their own important attributes:
- Memory Allocation Occurs: A definition allocates memory, allowing the program to use the variable or function.
- Includes Both Type and Value: Definitions specify what the variable or function is in terms of both type and content.
An example of a function definition is as follows:
void myFunction() {
// Function body
} // Definition of the function
In this instance, the function `myFunction()` is defined in its entirety, including its body, thus making it ready for use.
Common Uses of Definitions
Definitions are essential in various areas, including:
- Variable Initialization: You provide a value and allocate memory when defining a variable.
- Function Implementation: A full function definition includes its logic and behavior.
For example, in a source file, you might see:
#include "header.h"
int myGlobalVar = 10; // Definition
This line completes the declaration made earlier in the header, providing the actual memory allocation needed to make `myGlobalVar` usable.
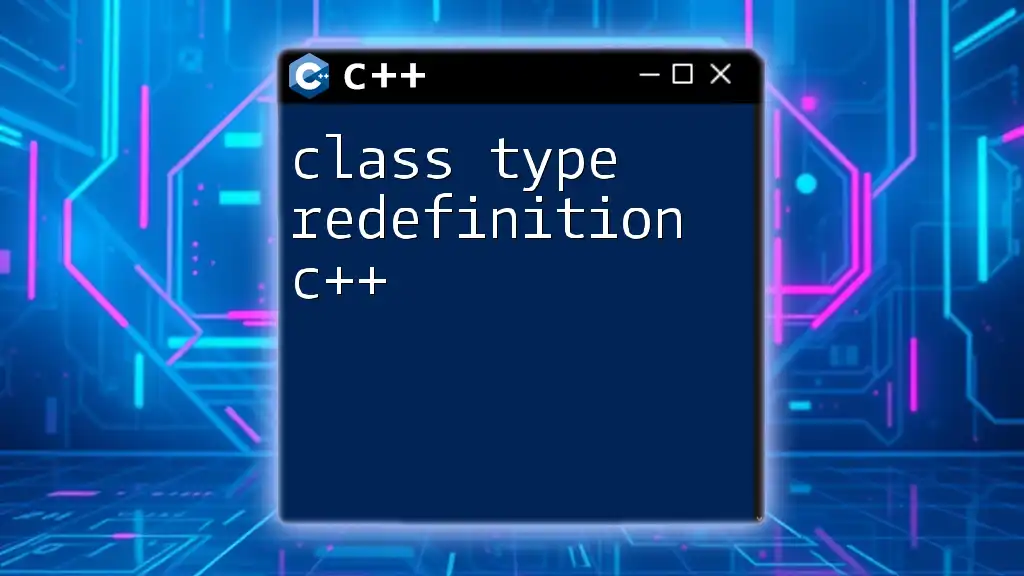
Declaration vs Definition: Key Differences
C++ Declare vs Define: A Side-by-Side Comparison
Understanding the differences between declaration and definition is vital in mastering C++. Here’s a clear comparison:
- Memory Allocation:
- Declaration: No memory allocation occurs.
- Definition: Memory is allocated for the variable or function.
- Usage Frequency:
- Declaration: Can be repeated multiple times across different files.
- Definition: Must be unique in a given scope; there can be only one definition.
To visualize this, consider the following table:
Aspect | Declaration | Definition |
---|---|---|
Memory Allocation | No | Yes |
Type Specification | Yes | Yes |
Initialization | No | Yes |
Multiple Usages | Yes (for extern vars) | No (must be unique) |
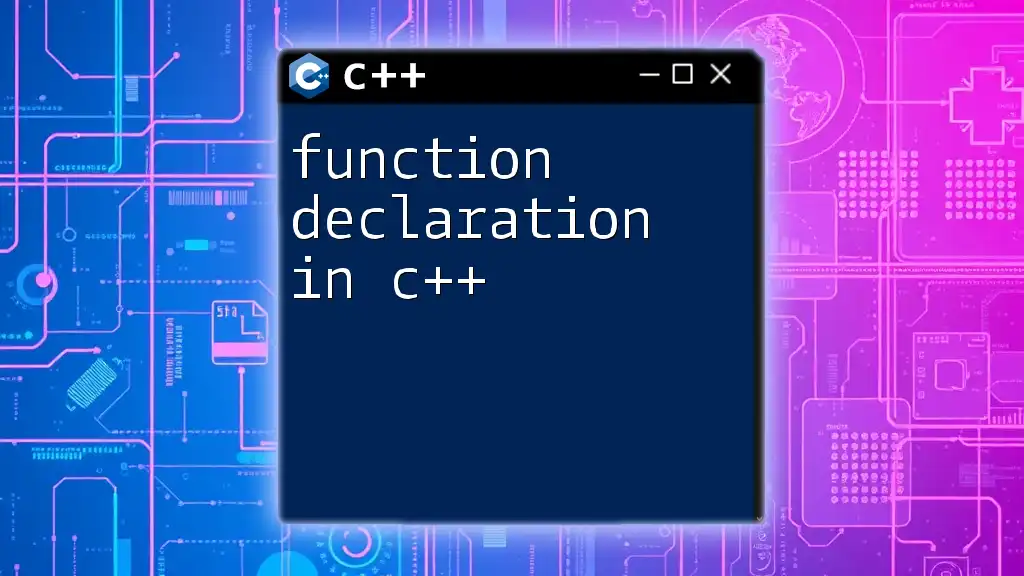
Real-world Examples
Example 1: Variables
To illustrate both concepts in practice, consider the following example for a variable:
extern int myVar; // Declaration
int myVar = 12; // Definition
Here, `myVar` is first declared as an external variable, indicating that it will be defined elsewhere. The second line is its definition, allocating memory and initializing it to the value `12`.
Example 2: Functions
In terms of functions, the interaction between declarations and definitions is evident.
void myFunction(); // Declaration
void myFunction() {
// Function definition
}
The first line declares `myFunction`, indicating that it exists. The second line provides the full implementation, completing the declaration.
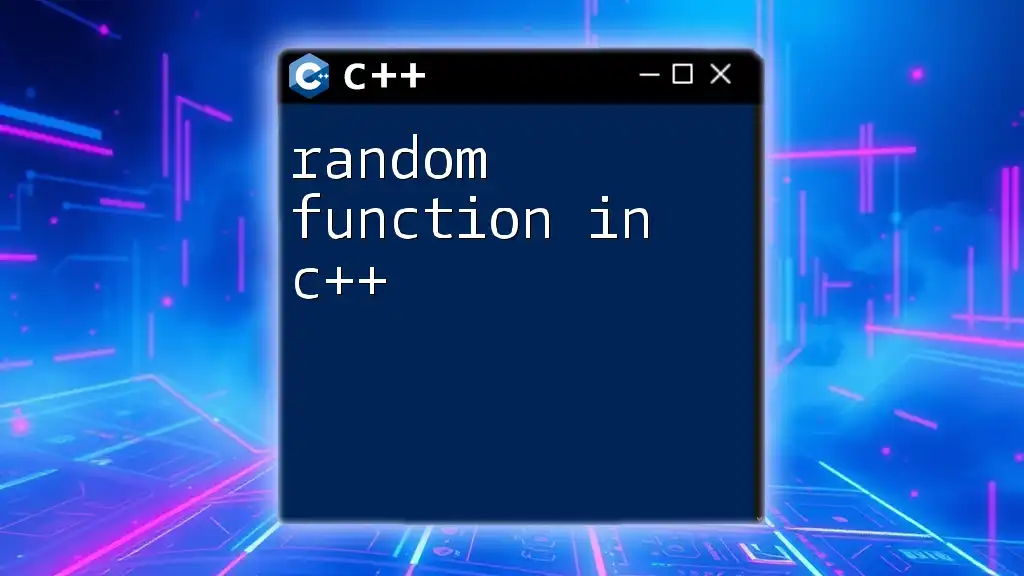
Common Mistakes to Avoid
Misunderstanding Scope
Common pitfalls can arise from misunderstanding scope in relation to declarations and definitions. For instance, if you declare and define a variable in different scopes, this could lead to confusion in your program’s behavior.
extern int a; // Declaration
int a = 10; // Definition
Here, `a` is declared as an external variable and then defined, raising questions about where it lives in memory. Such discrepancies can cause link errors if mismanaged.
Confusing Forward Declarations with Definitions
Another mistake is confusing forward declarations with definitions, especially in class contexts.
class MyClass; // Forward declaration
class MyClass { // Definition
// ...
};
Here, the first line serves merely as a forward declaration, allowing you to inform the compiler of `MyClass`’s existence. The second line then provides the full definition, detailing its members and functions.
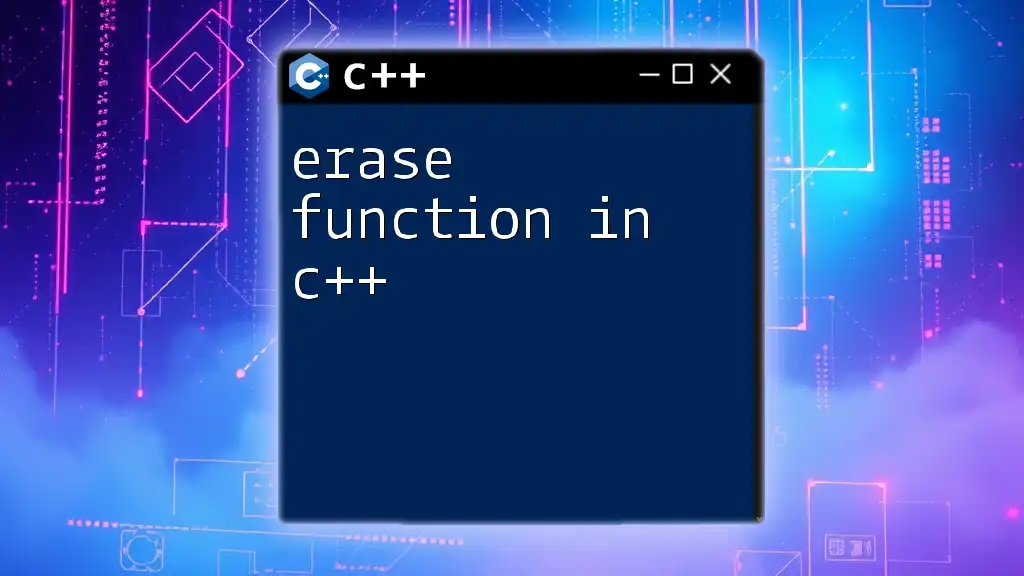
Conclusion
Understanding declaration vs definition in C++ is essential for writing efficient and clear code. Declarations introduce names and types without allocating memory, while definitions fully allocate memory and provide implementations. By distinguishing between the two, you can avoid common mistakes and improve your programming practices. As you continue to explore C++, remember that practice and clarity are key—experiment with your own examples to solidify your understanding!
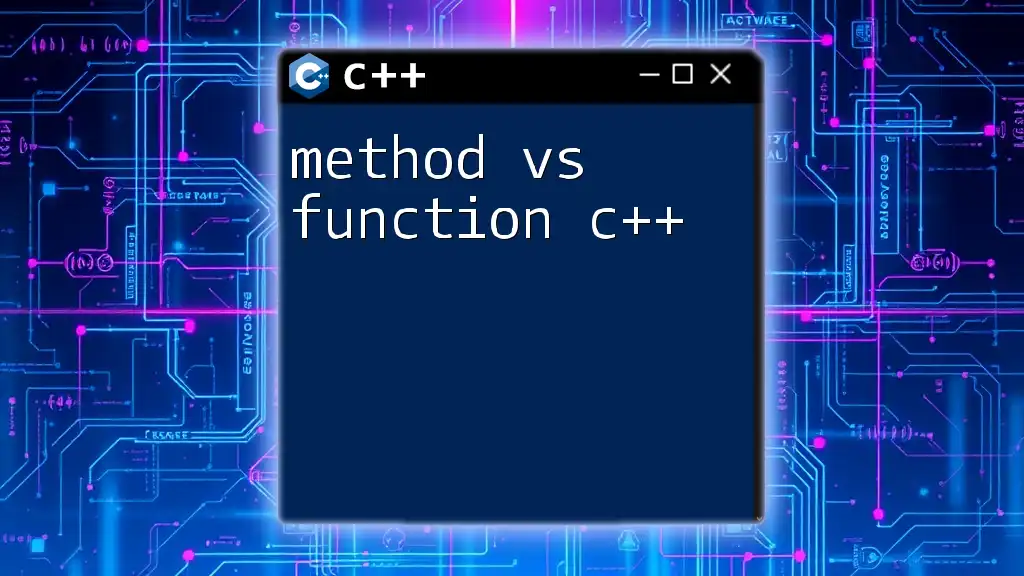
Additional Resources
For further reading, consider exploring in-depth tutorials on declarations and definitions, or try hands-on coding exercises to enhance your grasp of these concepts.