In C++ programming, transitioning from problem analysis to program design involves breaking down the problem into manageable components, creating a structured outline, and implementing it through clear, efficient code.
Here’s a simple code snippet that demonstrates a basic structure for a program that calculates the sum of two numbers:
#include <iostream>
int main() {
int a, b, sum;
std::cout << "Enter two numbers: ";
std::cin >> a >> b;
sum = a + b;
std::cout << "Sum: " << sum << std::endl;
return 0;
}
Problem Analysis
Understanding the Problem
Before you start coding, it is crucial to define the problem clearly. This forms the foundation of your program and prevents unnecessary complications later on. A precise problem statement clarifies what the software needs to accomplish.
Example: If we take the objective of designing an application to manage a library system, the problem could be stated as: "The library system should enable users to check out books, return them, and manage the inventory seamlessly."
Gathering Requirements
Identifying and detailing the requirements of the program helps shape the final solution. Requirements can be broken down into functional and non-functional types:
-
Functional Requirements: These specify what the program should do, such as:
- Users should be able to add new books to the library.
- Users should check out books for a specific period.
-
Non-Functional Requirements: These cover how the program should behave, like:
- The system should handle up to 1,000 simultaneous users.
- The interface must be user-friendly and intuitive.
Creating Use Cases
Use cases are critical in clarifying the interactions between users and the system. They help to explore the possible scenarios in which the software may be used.
For the library management system, a simple use case might involve:
- Use Case: User logs in to issue a book.
- Precondition: User is registered and has valid credentials.
- Main Flow: User enters login details -> System checks credentials -> User is logged in.

Program Design
Architectural Considerations
Selecting the appropriate software architecture is paramount for scalability and maintainability. The architecture determines the relationship between various components of your system.
Some common architectures include:
- Monolithic Architecture: All components are interconnected and run as a single service.
- Microservices Architecture: Each functionality is developed independently as a separate service, enabling better scalability.
For the library system, a microservices architecture might be beneficial as it divides the functionalities (like book management, user management) allowing each to evolve independently.
Data Modeling
Accurate data modeling ensures that you account for all necessary data structures. Understanding how data will interact within your application is fundamental to successful design.
In our library example, a potential data model for books and users can be defined as follows:
struct Book {
int id;
std::string title;
std::string author;
bool isIssued;
};
struct User {
int userId;
std::string name;
std::vector<int> issuedBookIds;
};
Here, the `Book` struct holds details about each book, while the `User` struct keeps track of registered users and the books they have checked out.
User Interface Design
Designing an intuitive user interface (UI) is crucial for enhancing user experience. A well-thought-out UI can make the software accessible and efficient.
Consider sketching wireframes and layouts for how users will interact with the application. Questions to ask during this phase can include:
- How will users navigate through the application?
- What information should be displayed on the dashboard?

Implementation
Setting Up the Development Environment
Before implementing your code, you’ll need to select the right tools for C++ development. Common tools include IDEs like Visual Studio, Code::Blocks, and compilers such as g++. Choosing the right environment can significantly affect your productivity.
Writing the Code
A well-organized codebase enhances readability and maintainability. It is essential to establish a proper file and function organization. For the library management system, consider how to efficiently implement basic features.
An example implementation for adding a book could look like this:
void addBook(std::vector<Book>& library, const std::string& title, const std::string& author) {
Book newBook = {static_cast<int>(library.size() + 1), title, author, false};
library.push_back(newBook);
}
In the snippet above, a new book is added to the library vector with unique identification and basic attributes set.
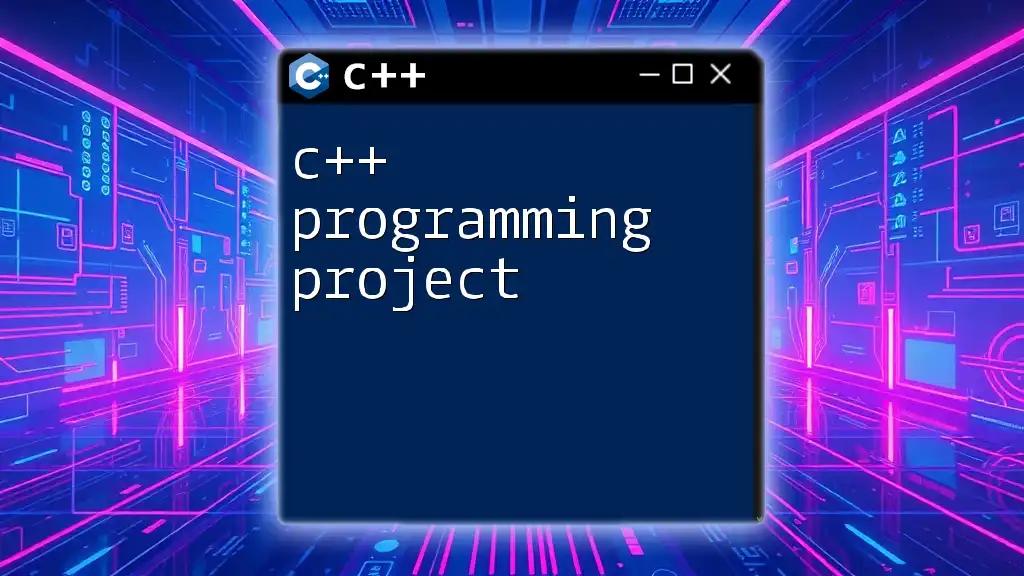
Testing and Debugging
Importance of Testing
Testing your program is vital for ensuring it performs as intended. It helps identify bugs and deficiencies before deployment, enhancing overall software reliability.
Types of Testing
Different types of testing cover various aspects of software quality:
- Unit Testing: Testing individual components for correctness.
- Integration Testing: Testing how components work together.
- System Testing: Validating the complete and integrated software product.
When writing test cases, focus on scenarios based on your design and requirements. Here’s an example unit test for the `addBook` function:
void testAddBook() {
std::vector<Book> library;
addBook(library, "C++ Primer", "Stan Lippman");
assert(library.size() == 1);
assert(library[0].title == "C++ Primer");
}
The snippet demonstrates a straightforward assertion that verifies whether the `addBook` function behaves correctly.
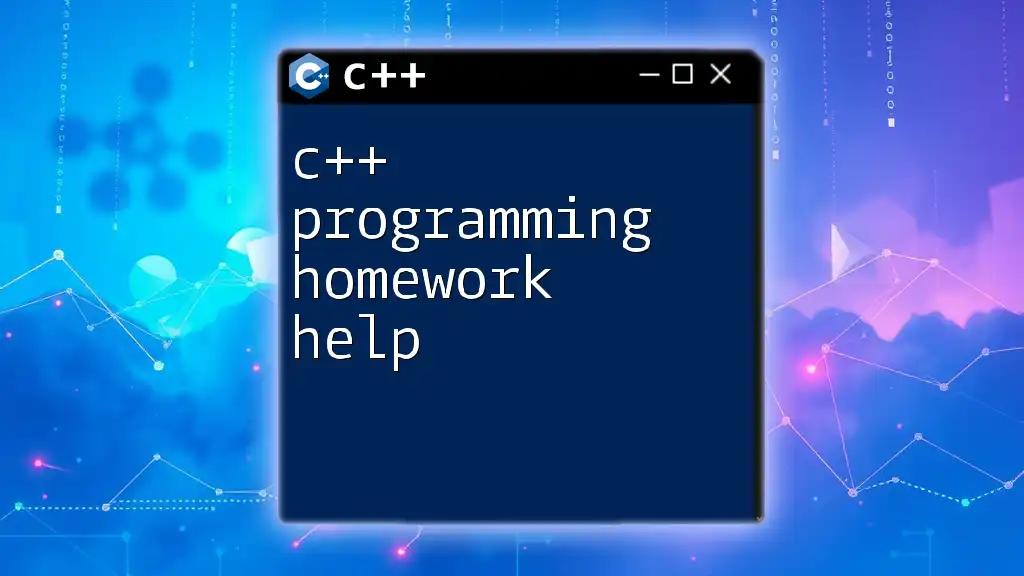
Documentation
Importance of Documentation
Proper documentation is essential for the long-term success of the project. Clear reporting on system functionality, API usage, and internal logic facilitates ease of use and maintenance.
Types of Documentation
Documentation can be divided into various forms:
- User Manuals: Guides for end-users detailing how to navigate and utilize the software.
- API Documentation: Explanations of how to interact with the program's components.
- Inline Comments: Comments within the code that help other developers understand design decisions.
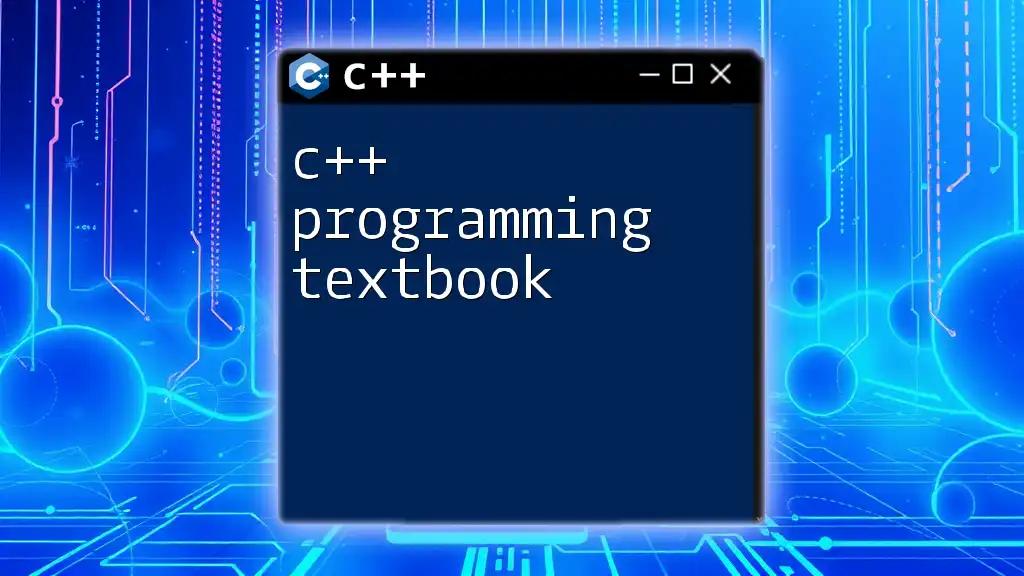
Conclusion
By navigating through each phase from problem analysis to program design, you establish a solid groundwork for developing robust C++ applications. Each step plays a vital role in ensuring the final product meets user expectations and operates efficiently.
Encourage readers to leverage these principles in their programming endeavors, as they will form the backbone of a successful software development lifecycle. Understanding that careful analysis and thoughtful design lead to a more seamless implementation can greatly enhance their C++ programming skills.
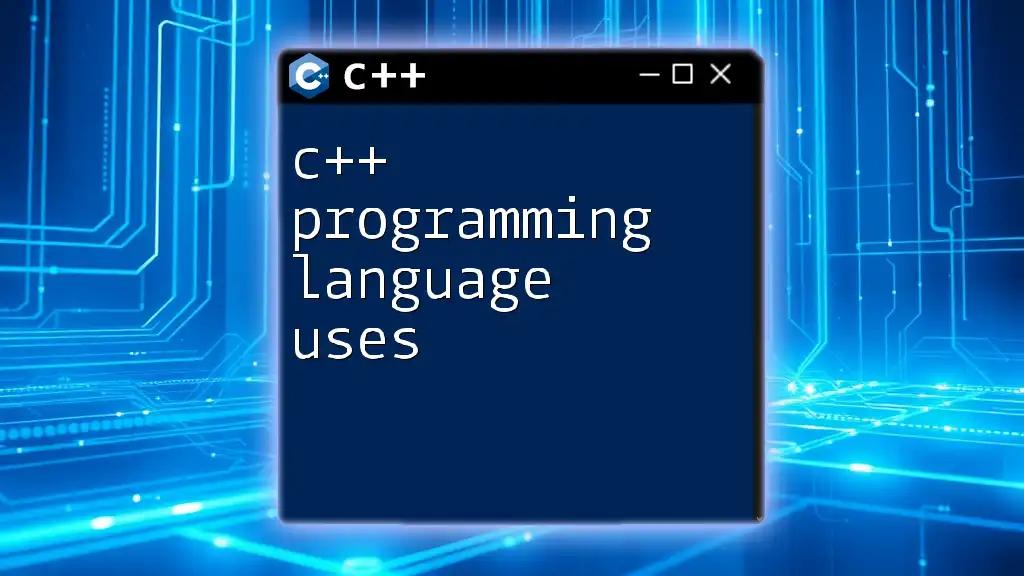
Additional Resources
For those looking to deepen their understanding of C++ and software design, consider utilizing various tools, libraries, and online courses available to foster continued learning and improvement. Each resource brings unique insights, aiding programmers at every skill level to excel in their coding projects.