"Mastering C++ programming from problem analysis to program design, as outlined in the 8th edition, empowers learners to effectively transform user requirements into well-structured code solutions."
Here’s a brief C++ code snippet demonstrating a simple program design:
#include <iostream>
using namespace std;
int main() {
// Problem Analysis: We want to sum two integers
int a, b, sum;
// Input
cout << "Enter two integers: ";
cin >> a >> b;
// Process
sum = a + b;
// Output
cout << "The sum is: " << sum << endl;
return 0;
}
Understanding C++ Fundamentals
What is C++?
C++ is a powerful and versatile programming language that is widely used in various domains, from system software to game development. It was designed with performance in mind, providing low-level access to memory without sacrificing the convenience of high-level programming constructs. Unlike many other languages, C++ supports both procedural and object-oriented programming paradigms, making it a great choice for developers who wish to have both flexibility and control.
Key features of C++ include:
- Abstraction: The ability to create complex data types.
- Encapsulation: Grouping data and functions that operate on that data within classes.
- Inheritance: Allowing new classes to derive properties from existing classes.
- Polymorphism: Enabling methods to do different things based on the object using them.
Essential C++ Syntax
Variables and Data Types
C++ utilizes a variety of data types, each serving a specific purpose. Fundamental data types include:
- int: for integers.
- float: for floating-point numbers.
- char: for single characters.
- bool: for Boolean values (true/false).
Here’s a simple code snippet demonstrating variable declarations:
int age = 25; // Integer variable
float height = 5.9; // Float variable
char initial = 'J'; // Char variable
bool isStudent = true; // Boolean variable
Control Structures
Control structures are vital for managing the flow of a C++ program. They include conditional statements and loops. For example, the if statement allows you to execute code based on conditions, while loops enable you to repeat a block of code.
Consider this example of a `for` loop that prints numbers from 0 to 4:
for(int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}

Problem Analysis
Identifying the Problem
The first step in the C++ programming from problem analysis to program design is effectively identifying the problem at hand. This often involves techniques such as brainstorming and creating user stories, which help you understand the core requirements and user expectations.
Example Problem Statement
For instance, let’s say we want to create a program that calculates the area of different geometric shapes. This statement succinctly describes the goal of the program and sets the stage for further analysis.
Defining Inputs and Outputs
Once the problem is identified, it’s imperative to define the inputs and outputs. Inputs might include the type of shape and its dimensions, while outputs would be the calculated area. You could visualize this with a use case diagram that illustrates the relationship between user inputs and expected results.

Program Design
Designing Algorithms
An algorithm is a step-by-step procedure to solve a problem. The effectiveness of the algorithm directly impacts the efficiency of your C++ program.
Pseudocode Development
Before diving into actual coding, converting your problem statement into pseudocode can be immensely helpful. This method emphasizes the logic of the solution without being bogged down in syntax. Here’s an example for our area calculation:
FUNCTION calculateArea(shape, dimensions)
IF shape is "circle" THEN
RETURN π * dimensions.radius^2
ELSE IF shape is "rectangle" THEN
RETURN dimensions.length * dimensions.width
Structured Programming Concepts
Structured programming emphasizes modularity and clarity in code. By breaking your program into functions, you enhance readability and maintainability.
Creating Functions
Here’s how to create a simple function to calculate the area of a circle in C++:
double calculateArea(std::string shape, double radius) {
if (shape == "circle") {
return 3.14 * radius * radius;
}
return 0; // Default case if not a circle
}
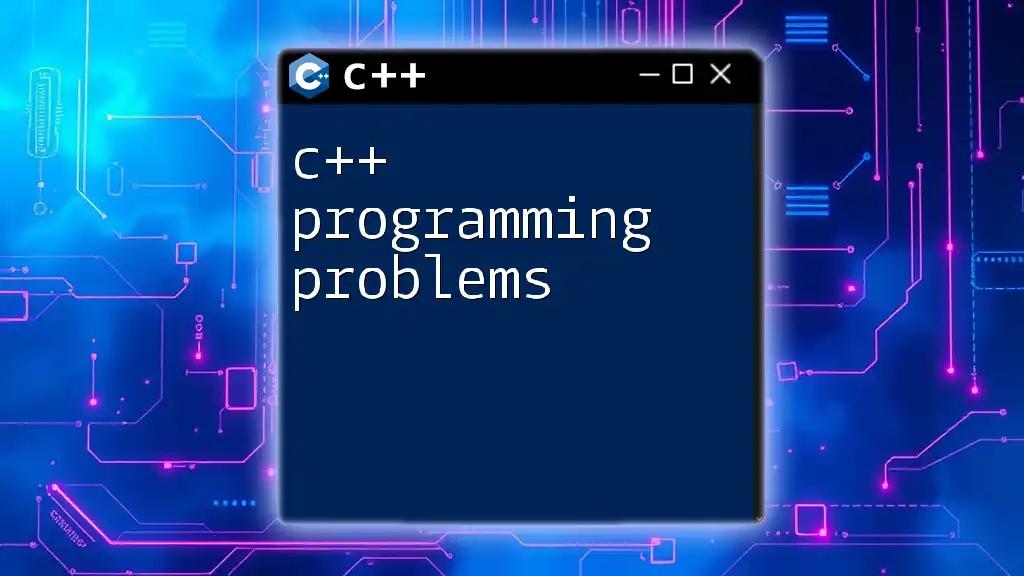
Implementation
Writing C++ Code
Setting up a proper C++ development environment is key. Tools like integrated development environments (IDEs) such as Visual Studio or Code::Blocks streamline coding, compiling, and debugging processes.
Here is a complete example of a program to calculate the area of a circle:
#include <iostream>
#include <string>
double calculateArea(std::string shape, double radius) {
if (shape == "circle") {
return 3.14 * radius * radius;
}
// Additional shapes can be added later.
return 0;
}
int main() {
double radius;
std::cout << "Enter radius of the circle: ";
std::cin >> radius;
std::cout << "Area of the circle: " << calculateArea("circle", radius) << std::endl;
return 0;
}
Debugging and Testing
Debugging is an integral part of programming. Using debuggers can help you trace and identify errors in your code. Additionally, incorporating print statements at critical junctures can provide insights into your program's flow.
Equally important is testing. By writing unit tests, you ensure the functionality of your methods. For example, testing the `calculateArea` function with various inputs can help verify that it behaves as expected. Unit testing frameworks like Google Test can streamline this process.
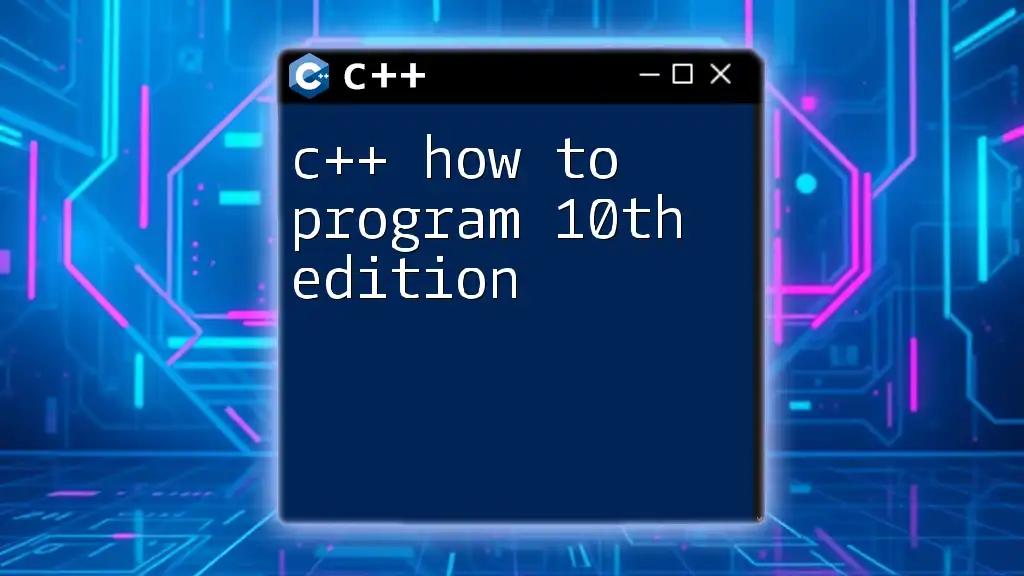
Conclusion
C++ programming from problem analysis to program design is crucial for developing robust and efficient applications. The foundational skills of analyzing problems, designing algorithms, implementing solutions, and debugging are essential to any successful programmer. The 8th edition of "C++ Programming from Problem Analysis to Program Design" serves as an excellent resource for mastering these concepts and advancing your programming skills. As you continue your journey in C++, remember that practice and real-world application will solidify your understanding and expertise.
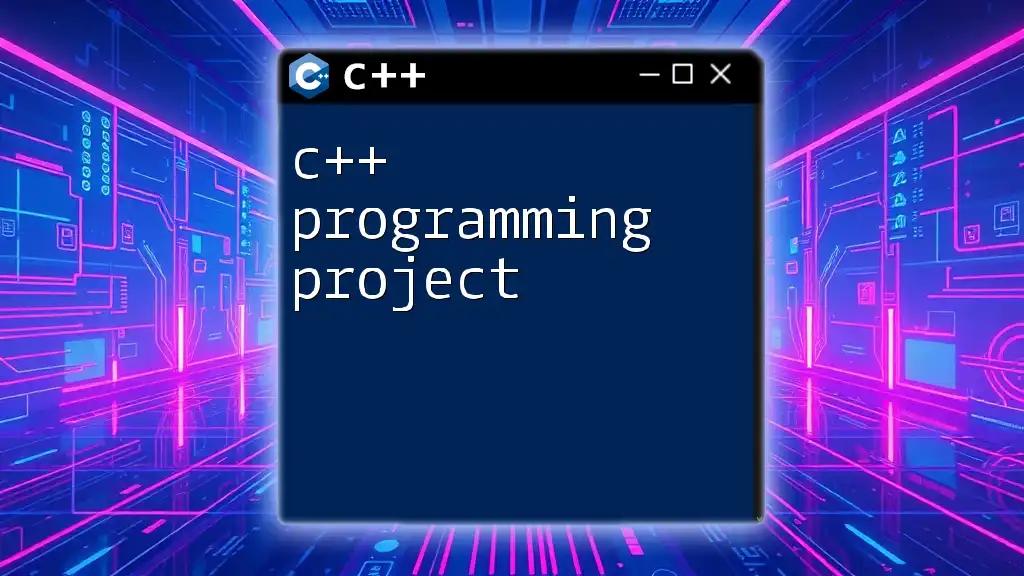
Further Resources
Explore online platforms offering C++ tutorials, engage in programming forums, and consider additional literature to deepen your understanding of concepts discussed in this guide. Embrace the power of community and continuous learning as you advance your C++ programming journey.