Every C++ program must contain at least one function, and typically that function is the `main()` function, which serves as the entry point for program execution.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Functions in C++
Definition of a Function
A function is a block of code that performs a specific task and can be reused throughout a program. Its main purpose is to organize code, making it more readable and easier to manage. Functions can also take inputs (parameters) and produce outputs (return values), which facilitates tasks such as calculations or data manipulations.
It is crucial to recognize the distinction between functions and methods. While both concepts refer to code blocks that encapsulate behavior, methods are functions that are associated with a class and typically operate on the data within that class.
Anatomy of a Function
To fully grasp how functions work, it is essential to understand their syntax. The general structure of a function declaration encompasses several components:
- Return Type: Specifies the type of value the function will return.
- Function Name: A descriptor that identifies the function.
- Parameter List: A set of inputs the function can take.
- Function Body: The block of code that defines what the function does.
Here is a simple representation of a function declaration:
returnType functionName(parameters) {
// function body
}
For example:
int add(int a, int b) {
return a + b;
}
In this example, the function `add()` takes two integers as parameters and returns their sum.
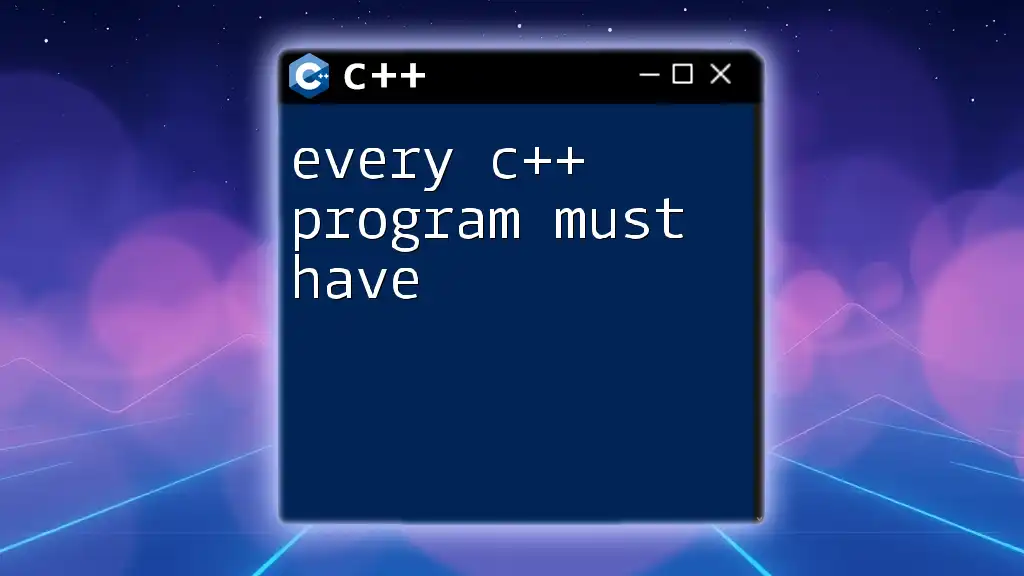
Why Every C++ Program Needs at Least One Function
Entry Point of a C++ Program
Every valid C++ program must contain a function, specifically the `main()` function. This function serves as the entry point for execution, meaning that when a program runs, this is the first function that gets called.
Consider the following simple `main()` function:
int main() {
// Entry point of the program
return 0;
}
In this code, the program starts execution from `main()`, which returns 0—indicating successful completion.
Code Organization
Functions are vital for organizing code. Instead of writing a large block of procedural code, breaking it down into functions allows for modular programming. This not only makes the code easier to read but also enables developers to make changes for debugging or enhancements without sifting through a monolith.
For example, compare a monolithic approach with a structured one:
Monolithic Code Example:
int main() {
// Calculating the area of a circle
float radius = 5.0;
float area = 3.14 * radius * radius;
// Outputting the area
std::cout << "Area: " << area << std::endl;
}
Structured Code Example Using Functions:
float calculateArea(float radius) {
return 3.14 * radius * radius;
}
int main() {
float radius = 5.0;
float area = calculateArea(radius);
std::cout << "Area: " << area << std::endl;
}
By organizing code into functions, changes become localized, making maintenance more straightforward.
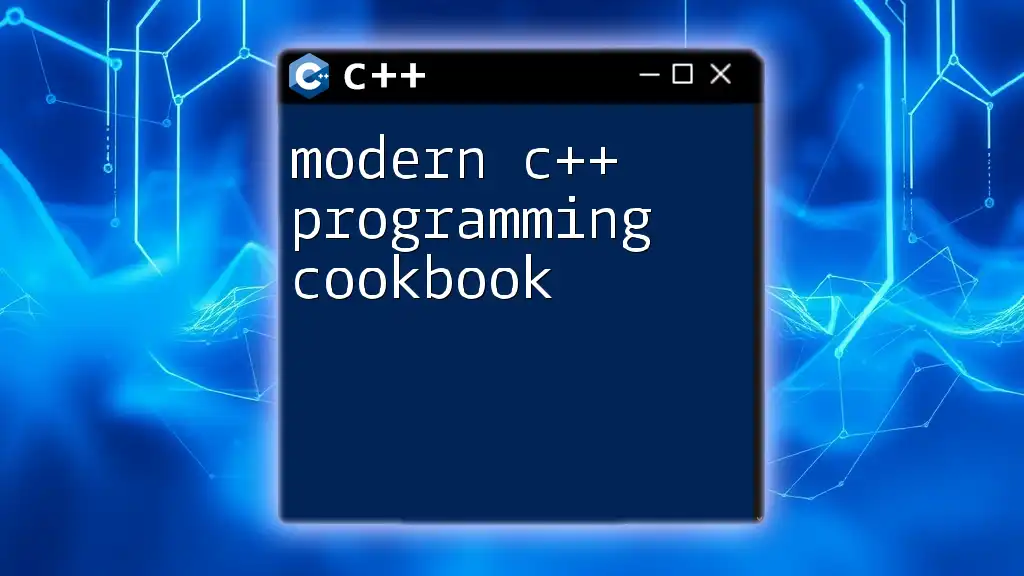
Types of Functions in C++
Built-in Functions vs. User-defined Functions
Functions in C++ can be categorized into built-in functions and user-defined functions. Built-in functions are those provided by the C++ language itself, such as `cout` for console output, or `sqrt` for calculating the square root.
User-defined functions, on the other hand, are created by programmers to perform specific tasks. For example:
void greet() {
std::cout << "Hello, World!" << std::endl;
}
Function Overloading
C++ supports function overloading, a feature that allows multiple functions to have the same name but differ in their parameter lists. This can enhance code readability and usability. Here is an example demonstrating function overloading:
void print(int i) {
std::cout << "Integer: " << i << std::endl;
}
void print(double d) {
std::cout << "Double: " << d << std::endl;
}
In this example, both `print` functions have the same name but handle different parameter types, showcasing how versatile C++ functions can be.
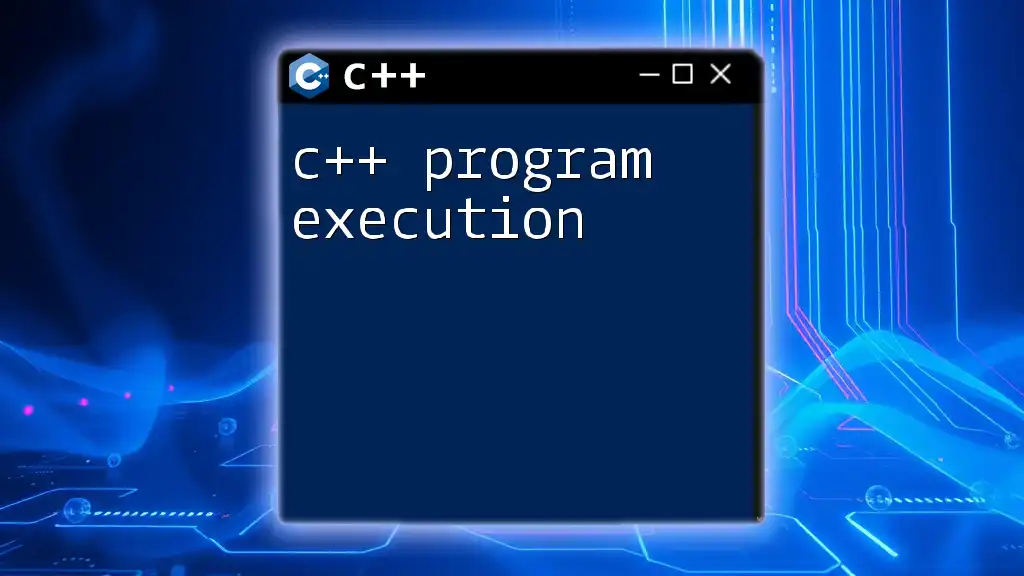
Function Parameters and Return Types
Understanding Parameters
Functions can take parameters (inputs) to operate. They can be passed by value, reference, or pointers.
- Value: A copy of the argument is passed to the function.
- Reference: The function receives a reference to the argument, allowing it to modify the original value.
- Pointer: Similar to references, but instead includes a memory address.
Here's an example illustrating different parameter types:
void modifyValue(int &x) {
x += 10; // modifies the original value
}
int main() {
int value = 5;
modifyValue(value);
std::cout << value; // Output will be 15
}
Return Values
A function can return a value using a specified return type. If a function doesn’t return a value, the return type should be `void`. The importance of return types cannot be overstated, as they define the data type of the output:
float multiply(float a, float b) {
return a * b; // returns a float
}
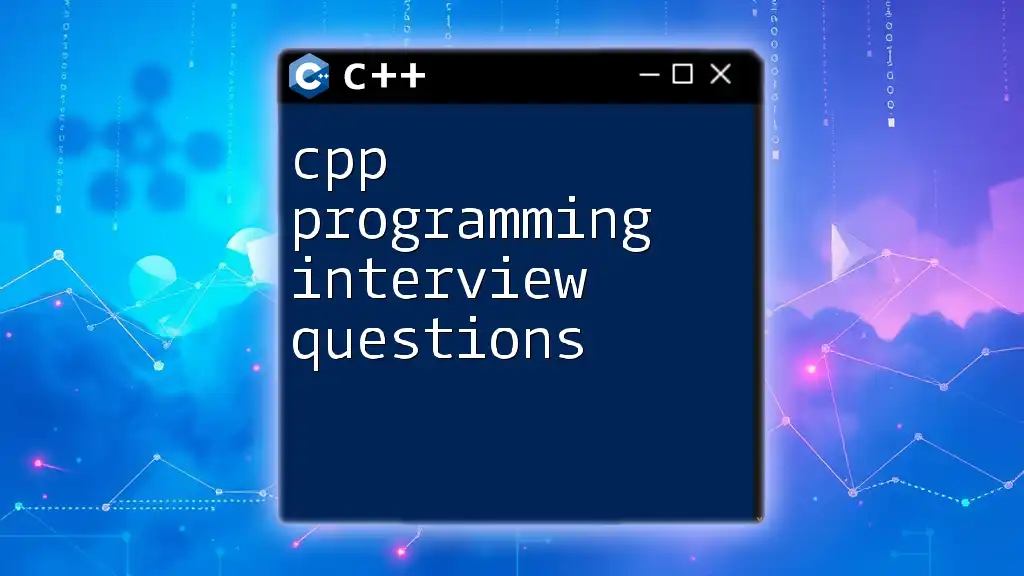
Scope and Lifetime of Variables in Functions
Local vs. Global Variables
Understanding scope is essential for function usage in C++. Local variables are defined within a function and cannot be accessed from outside that function. Conversely, global variables are accessible throughout the entire program.
For example, consider this:
int globalVar = 10; // global variable
void display() {
int localVar = 5; // local variable
std::cout << "Global Var: " << globalVar << ", Local Var: " << localVar << std::endl;
}
In this case, `display()` can access `globalVar`, but `localVar` cannot be accessed outside of the function where it is defined.
Lifetime of Variables
The lifetime of a variable refers to how long a variable exists in memory. Local variables exist only while the function is executing, while global variables exist for the duration of the program.
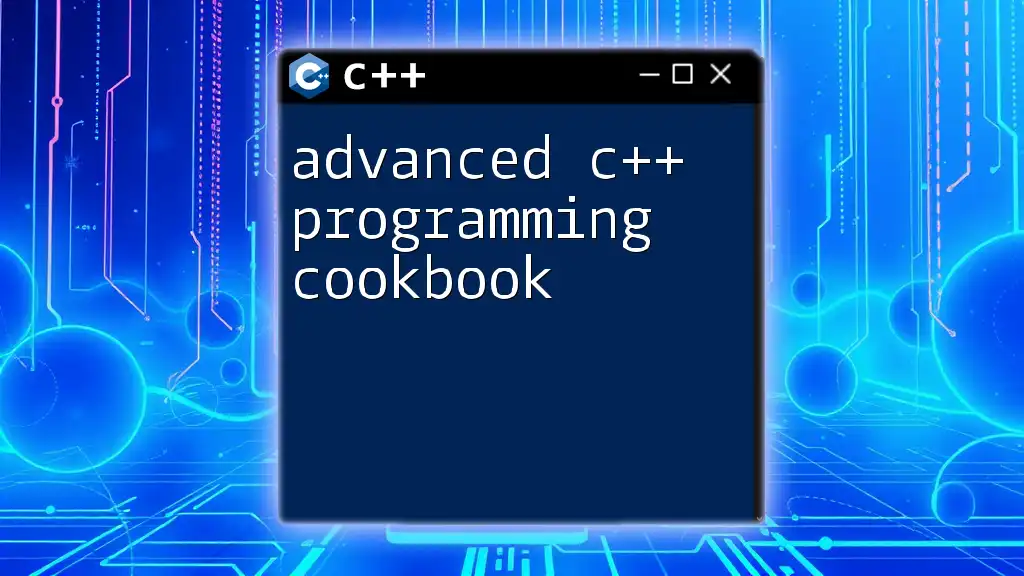
Best Practices for Writing Functions
Naming Conventions
Opting for descriptive function names can significantly improve code readability. A function name should reflect its purpose clearly, such as `calculateArea()` rather than something vague like `func1()`.
Keeping Functions Concise
To enhance readability and maintainability, strive to keep functions concise. Create functions that perform a single task, ensuring they are easier to test and debug.
Documentation and Comments
Comments in code are fundamental for helping other developers (or yourself in the future) understand how a function operates. Make an effort to clearly document your functions with comments:
// Function to calculate the area of a circle
float calculateArea(float radius) {
return 3.14 * radius * radius; // returns the area
}
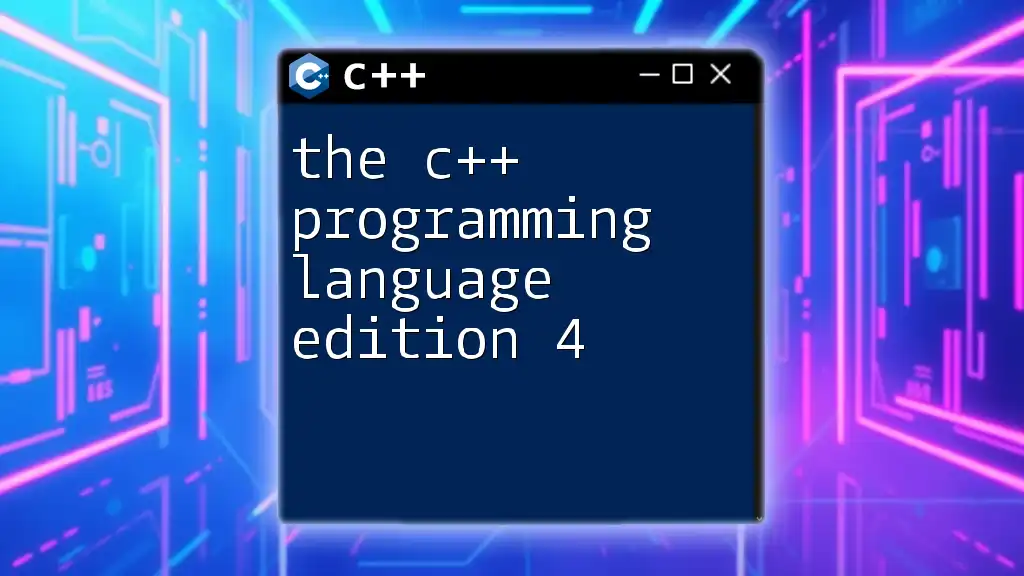
Conclusion
In conclusion, every C++ program must contain a function, serving as the foundation for organizing code and defining behavior. Functions like `main()` are critical to program execution and paves the way for efficient coding practices. By using functions, developers can create readable, maintainable, and reusable code.
Call to Action
Now that you have a foundational understanding of functions in C++, it’s time to practice! Try creating your own simple C++ programs that utilize functions, and observe how they improve your code organization and efficiency.
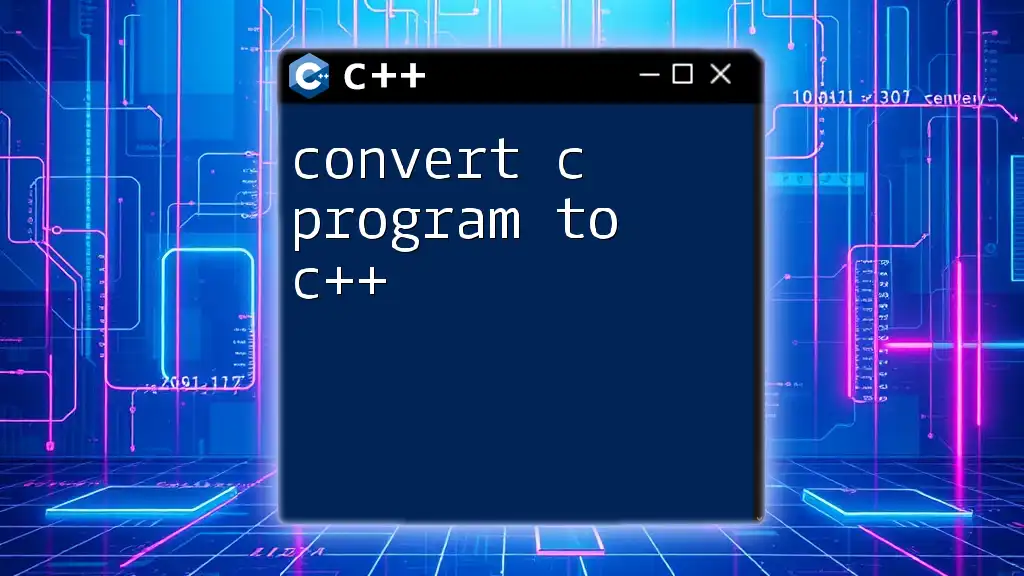
Additional Resources
To further enhance your knowledge, explore a variety of books, websites, or online courses dedicated to C++ programming. These resources will provide deeper insights and practical coding experiences to elevate your skills.