"Brief C++" refers to the art of succinctly utilizing C++ commands to efficiently perform tasks without unnecessary complexity.
Here’s a simple example that demonstrates a quick C++ command to output "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++
What is C++?
C++ is a high-level programming language that was developed by Bjarne Stroustrup at Bell Labs in the early 1980s. It is an extension of the C programming language, incorporating object-oriented features, which makes it a favored choice for system/software, game development, and high-performance applications.
The significance of C++ in modern programming cannot be understated; it's the foundation of many important software projects, frameworks, and libraries. Today, C++ powers applications across various industries, from gaming engines to embedded systems.
Features of C++
C++ boasts multiple features that distinguish it from other programming languages. Understanding these features provides insight into why C++ remains a relevant choice for developers.
-
Object-Oriented Programming (OOP): C++ fully supports OOP, which allows developers to create classes and objects. This paradigm enhances code reusability and modularity, leading to cleaner and more maintainable code. Key principles of OOP include:
- Encapsulation: Bundling data and methods that work on the data within one unit (class).
- Inheritance: Building new classes from existing ones to foster code reusability.
- Polymorphism: Allowing methods to do different things based on the object it is acting upon.
-
Low-Level Manipulation: C++ enables fine control over system resources and memory, making it suitable for low-level programming tasks, such as operating systems or hardware drivers.
-
Memory Management: Unlike many modern languages with automatic garbage collection, C++ requires programmers to manage memory manually. This provides greater control but also demands a deeper understanding of memory allocation and deallocation.
-
Standard Template Library (STL): The STL is a powerful component of C++ that provides a set of common data structures and algorithms. This library allows developers to easily implement complex data structures like lists, stacks, queues, and more.
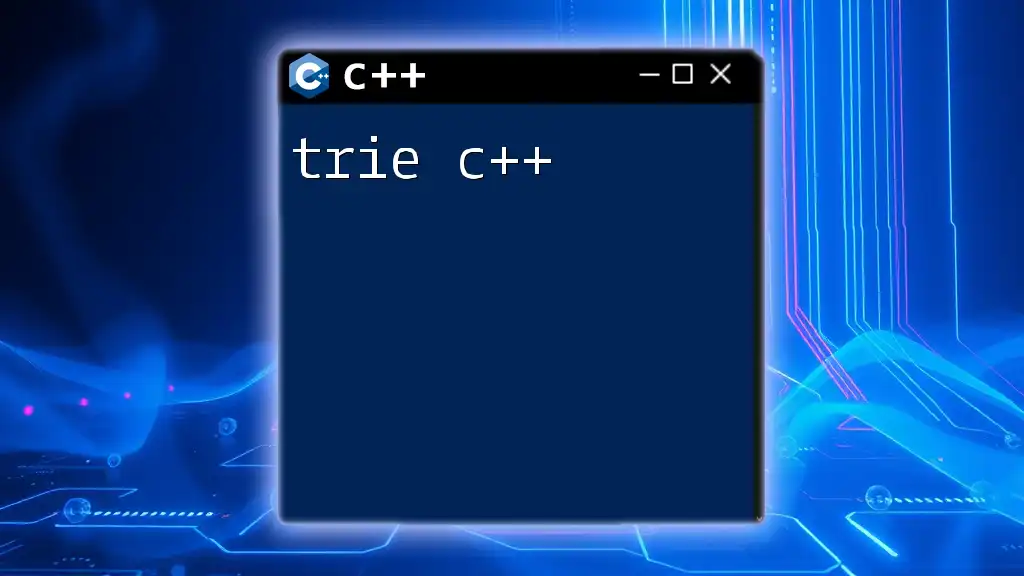
Getting Started with C++
Setting Up the Environment
To start coding in C++, you first need to set up an effective development environment:
- Choosing an IDE: The selected Integrated Development Environment (IDE) can greatly impact your coding experience. Popular choices include:
- Visual Studio: Known for its rich feature set and strong support for Windows development.
- Code::Blocks: A free, open-source IDE that works across platforms.
- CLion: A cross-platform IDE provided by JetBrains with excellent refactoring capabilities.
Once you choose an IDE, follow the installation steps on the respective website to set it up.
Compiling Your First C++ Program
Once your environment is ready, you can write your first C++ program. Here's a simple code to print "Hello, World!" to the console:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
This program demonstrates basic syntax, including the use of libraries and the main function, where program execution begins.
C++ Basics
Syntax Overview
Understanding C++ syntax is vital for writing effective code.
-
Comments in C++: Comments help explain the code and are ignored during execution. Use `//` for single-line comments and `/.../` for multi-line comments.
-
Data Types and Variables: C++ provides several data types, including:
- `int`: for integers,
- `double`: for floating-point numbers,
- `char`: for single characters.
Here’s an example of variable declaration and initialization:
int age = 30;
double salary = 55000.50;
char grade = 'A';
- Operators: C++ supports various operators:
- Arithmetic Operators: `+`, `-`, `*`, `/`, `%`
- Relational Operators: `==`, `!=`, `<`, `>`, `<=`, `>=`
- Logical Operators: `&&`, `||`, `!`
Control Structures
Conditional Statements
Control structures direct the flow of program execution based on given conditions.
- If and Else Statements: C++ enables conditional execution using if-else statements. For instance:
if (age >= 18) {
cout << "Adult";
} else {
cout << "Minor";
}
- Switch Case: This provides an alternative to if-else for multiple conditions. Here’s an example:
switch (grade) {
case 'A':
cout << "Excellent!";
break;
case 'B':
cout << "Well done!";
break;
default:
cout << "Grade not recognized.";
}
Loops
C++ offers several looping constructs to execute a set of instructions repetitively.
- For Loop: Commonly used when the number of iterations is known ahead of time. Example:
for (int i = 1; i <= 10; i++) {
cout << i << " ";
}
- While Loop: Utilized when the number of iterations is not known. Example:
int i = 1;
while (i <= 10) {
cout << i << " ";
i++;
}
- Do-While Loop: Similar to the while loop but guarantees at least one execution. Example:
int i = 1;
do {
cout << i << " ";
i++;
} while (i <= 10);
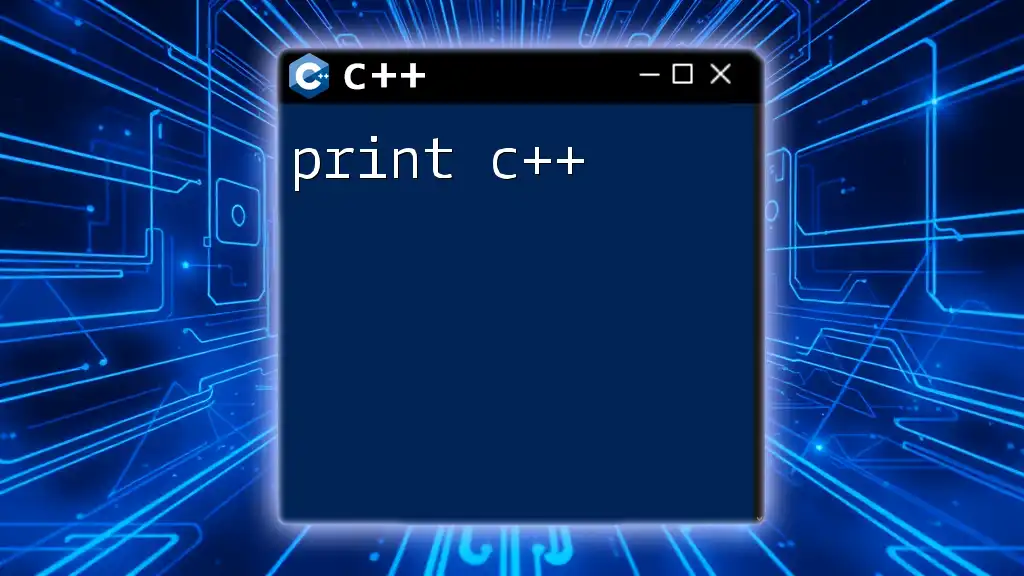
Advanced C++ Concepts
Functions
Functions are building blocks of C++ programs that allow code reusability and modularity.
Function Declaration and Definition
To create a function, you must declare its return type, name, and parameters. Here's an example:
int add(int a, int b) {
return a + b;
}
You can call this function as follows:
int sum = add(5, 3);
Passing Arguments
C++ supports passing arguments by value or by reference. Passing by reference allows the function to modify the original variable:
void swap(int &x, int &y) {
int temp = x;
x = y;
y = temp;
}
Object-Oriented Programming in C++
Classes and Objects
Classes are blueprints for objects. They encapsulate data for the object:
class Car {
public:
string model;
int year;
void start() {
cout << "Starting " << model << "..." << endl;
}
};
You can create an object of the class like this:
Car myCar;
myCar.model = "Tesla";
myCar.year = 2022;
myCar.start();
Inheritance
C++ supports inheritance, allowing new classes to inherit traits from existing ones:
class ElectricCar : public Car {
public:
void chargeBattery() {
cout << "Charging battery for " << model << endl;
}
};
Exception Handling
C++ provides mechanisms to handle exceptions—unforeseen errors during runtime.
Using try-catch blocks allows developers to gracefully handle errors:
try {
// Code that may throw an exception
throw std::runtime_error("A runtime error occurred");
} catch (const std::exception &e) {
cout << "Error: " << e.what() << endl;
}
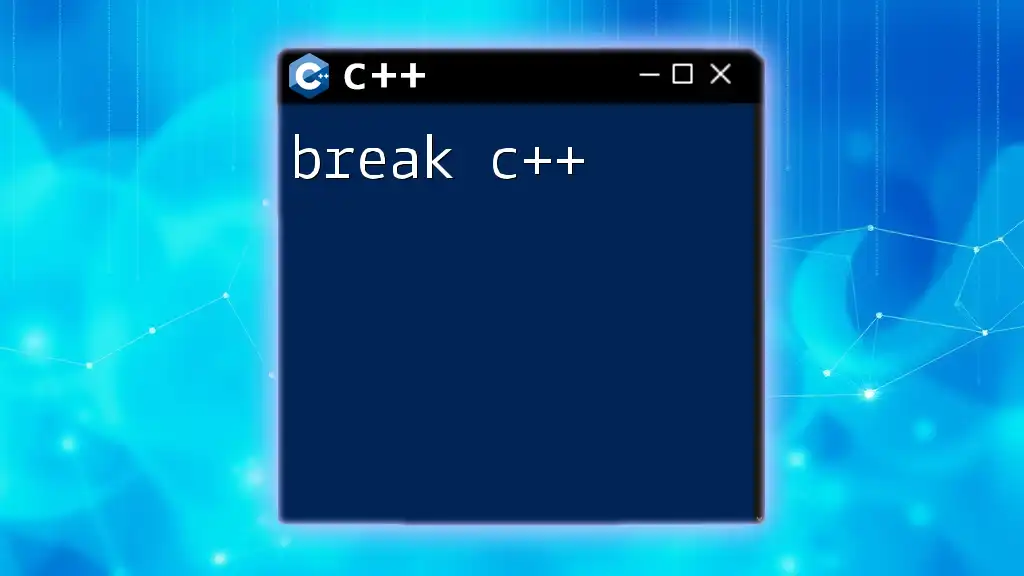
Working with the Standard Template Library (STL)
Overview of STL
The Standard Template Library (STL) is a powerful library that provides ready-to-use classes and functions for various data structures and algorithms. This can greatly speed up development time and enhance performance.
- Components of STL:
- Containers: Such as `vector`, `list`, and `map`.
- Algorithms: Functions that operate on containers, including sorting and searching.
- Iterators: Objects that allow programs to traverse through the elements of a container.
Using Basic Containers
Vectors
Vectors are dynamic arrays that provide an efficient way to store and manage data. Here's how to create and manipulate a vector:
#include <vector>
#include <iostream>
using namespace std;
int main() {
vector<int> numbers = {1, 2, 3, 4, 5};
numbers.push_back(6);
for (int num : numbers) {
cout << num << " ";
}
return 0;
}
Maps
Maps are key-value pairs that store unique keys to access associated values. They make lookups efficient:
#include <map>
#include <iostream>
using namespace std;
int main() {
map<string, int> age;
age["Alice"] = 30;
age["Bob"] = 25;
for (const auto& pair : age) {
cout << pair.first << ": " << pair.second << endl;
}
return 0;
}
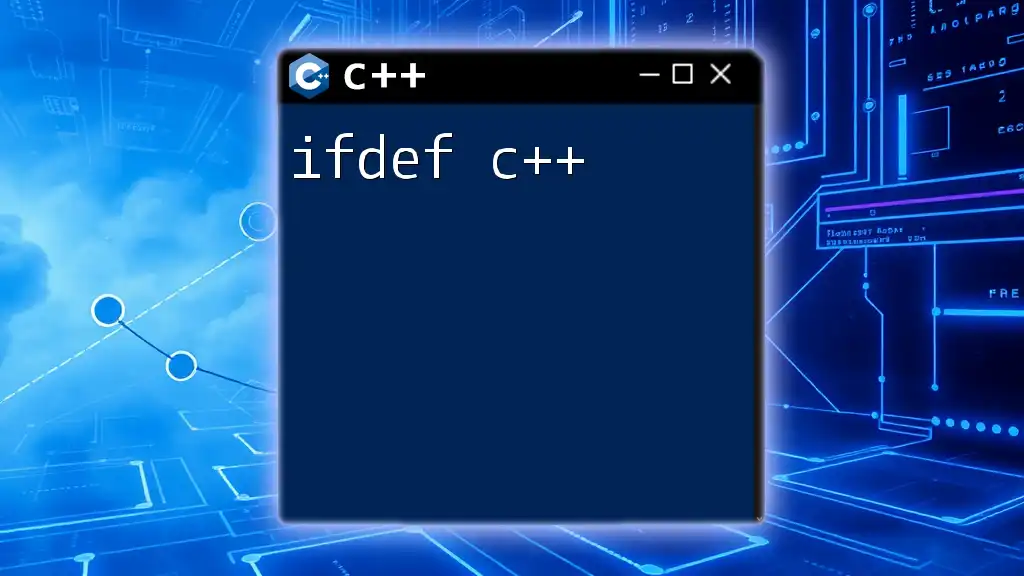
Best Practices in C++
Code Readability
Coding readability significantly enhances collaboration and maintenance. Important points include:
-
Importance of Comments: Use comments effectively to explain non-obvious parts of the code, making it easier for others (and yourself) to understand in the future.
-
Naming Conventions: Adopt clear and consistent naming conventions. For instance, use `camelCase` for function names and `snake_case` for variable names.
Memory Management
Proper memory management is critical in C++. Key aspects include:
- Dynamic vs. Static Memory Allocation: Use `new` and `delete` for dynamic memory allocation, but prefer using smart pointers like `std::unique_ptr` and `std::shared_ptr` to automate memory management and prevent memory leaks.
In conclusion, mastering C++ requires time and practice, given its rich features and complexity. By understanding the fundamentals, advanced concepts, and best practices, aspiring programmers can use C++ effectively, creating efficient and robust applications. Keep exploring and experimenting with C++, and most importantly, practice regularly to reinforce your skills!