The `trunc` function in C++ is used to truncate a floating-point number to its integer value, effectively removing the fractional part.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <cmath>
int main() {
double number = 5.75;
double truncated = std::trunc(number);
std::cout << "Truncated value: " << truncated << std::endl; // Outputs: Truncated value: 5
return 0;
}
What is `trunc`?
The `trunc` function in C++ is a part of the standard library and is primarily used to truncate a floating-point number. Truncation means to remove the decimal part of a number, effectively reducing it to its integer component without any rounding. It's essential to grasp the difference between truncation and rounding; where truncation simply cuts off the fraction without any adjustments, rounding might change the integer part based on the value of the decimal portion.
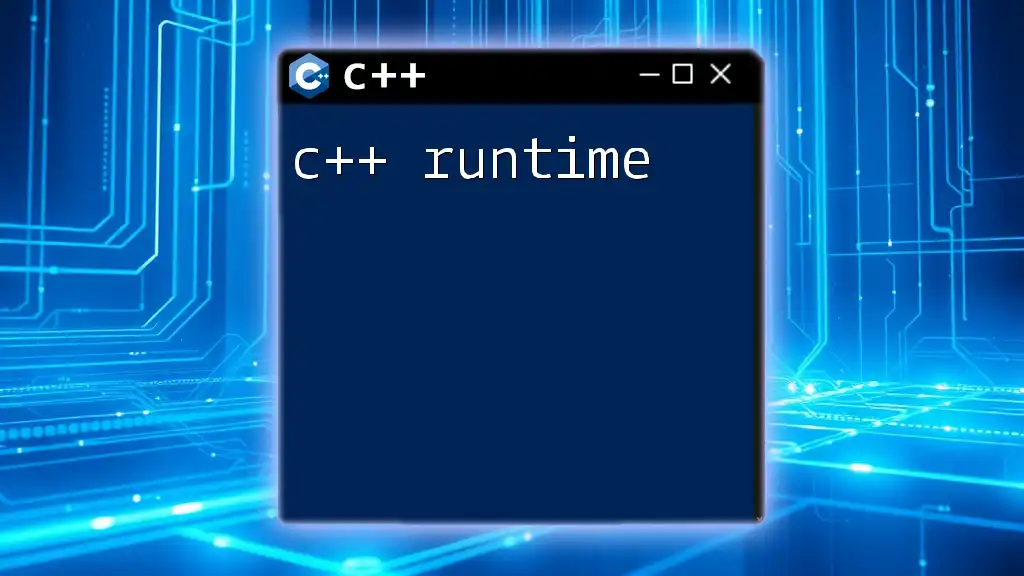
How `trunc` Works
The `trunc` function operates on floating-point numbers, converting them to their integer representation by discarding the decimal component. It is included in the `<cmath>` header file, along with other mathematical functions.
Example of Basic Usage:
#include <cmath>
#include <iostream>
int main() {
double x = 5.9;
double truncatedValue = trunc(x);
std::cout << "Truncated value of " << x << " is " << truncatedValue << std::endl; // Outputs: 5.0
return 0;
}
In the example, the variable `x` holds the value `5.9`, and calling `trunc(x)` gives `5.0`.
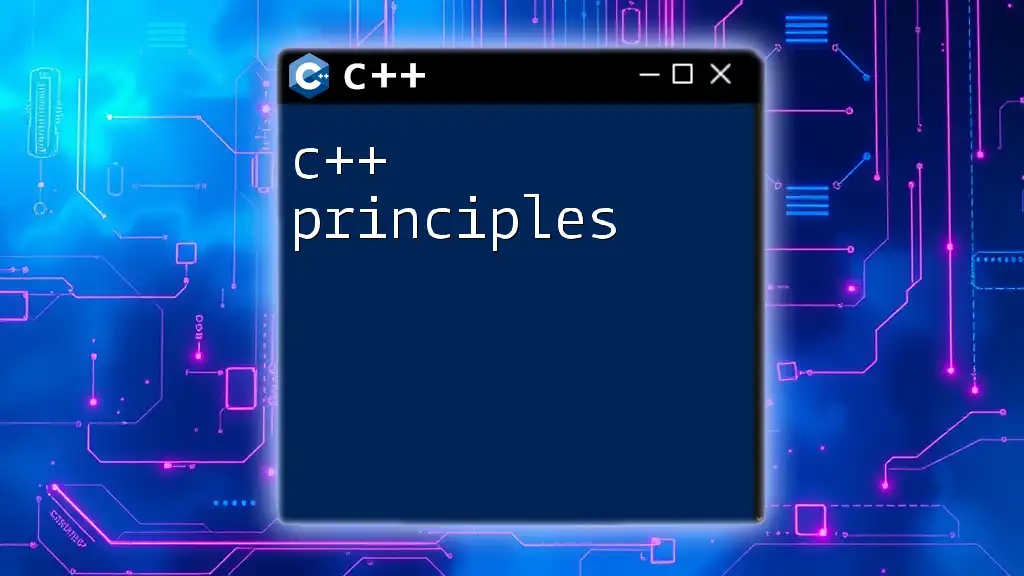
Syntax of `trunc` in C++
The syntax for using `trunc` is straightforward. Below is a detailed breakdown:
double trunc(double x);
This function takes a single argument of type `double` and returns the truncated value of that number as a `double`. Make sure to include the necessary header file at the beginning of your code to use this function:
#include <cmath>
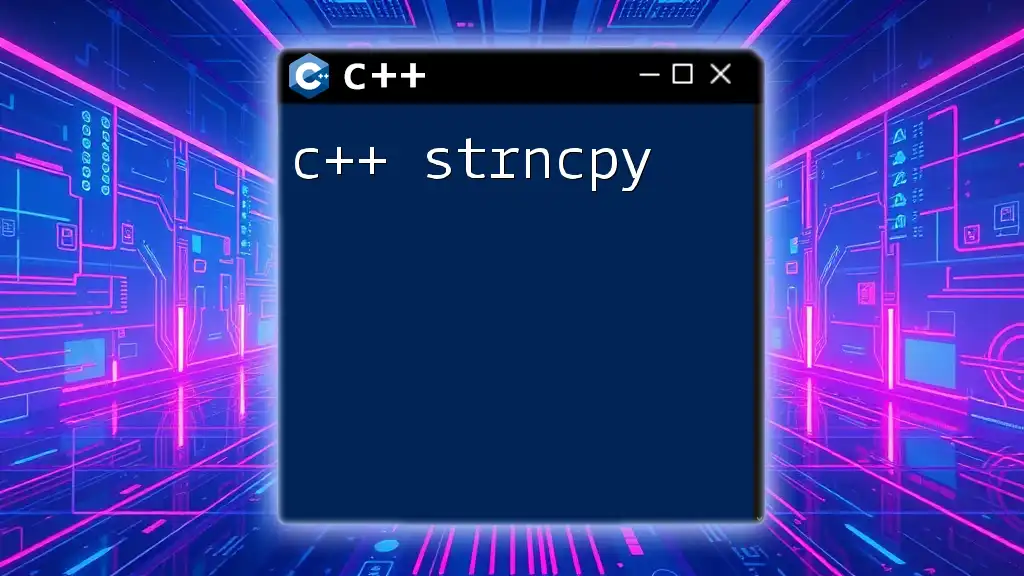
Mathematical Background
What is Truncation?
In a mathematical context, truncation refers to the process of shortening a number by removing one or more digits after the decimal point. In practice, this offers a cleaner number that is still comparable in magnitude. For instance, truncating `3.14` results in `3`, while truncating `-2.99` results in `-2`.
Truncation differs from the floor function, which will round down to the nearest integer. The floor of a number will always return the closest integer that is less than or equal to that number, while trunc merely strips away the fraction part.
Truncation in C++ Standards
The `trunc` function is part of C++'s `<cmath>` library and adheres to the C standard library's behavior. It's particularly important in applications where precision matters, and you want to control how values are handled mathematically. In contrast, other functions such as `ceil` will round up, and `round` will round to the nearest integer based on the fractional part.
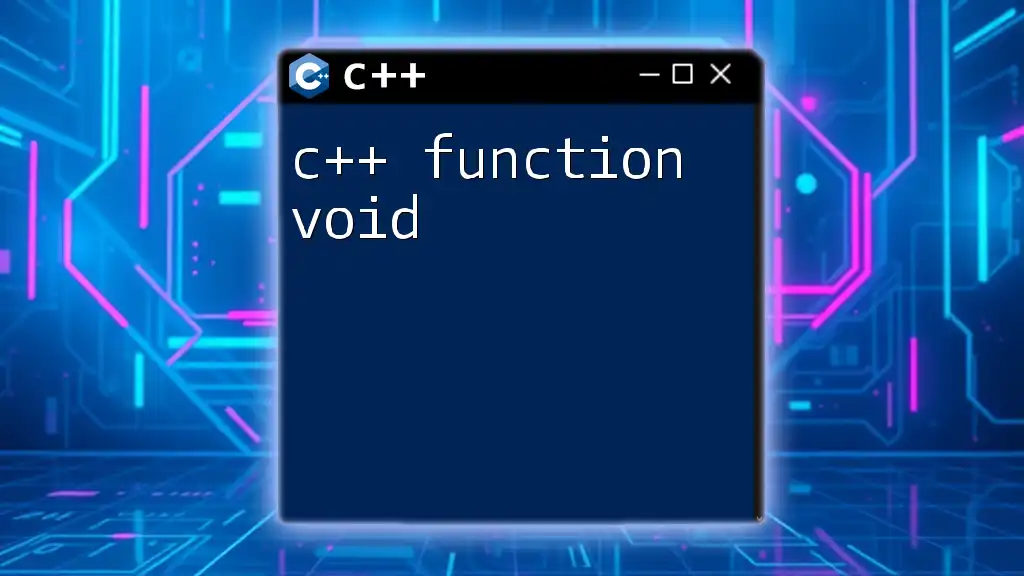
Using `trunc` in Real-World Applications
Common Use Cases for `trunc`
There are various scenarios where truncation is particularly beneficial:
- Financial Calculations: When dealing with monetary values, truncating to eliminate fractions might prevent overestimation.
- Engineering Applications: In scenarios where only whole units are required, truncation provides a straightforward way to ensure values meet expected integer conditions.
- Data Processing: When preparing datasets for analysis, truncating readings to remove insignificant digits can simplify inputs for certain algorithms.
Handling Edge Cases
Understanding how `trunc` behaves with negative numbers is crucial. When you truncate a negative floating-point number, `trunc` still moves towards zero.
Example:
#include <cmath>
#include <iostream>
int main() {
double y = -3.7;
double negativeTrunc = trunc(y);
std::cout << "Truncated value of " << y << " is " << negativeTrunc << std::endl; // Outputs: -3.0
return 0;
}
In this case, `-3.7` becomes `-3.0`, showcasing that truncation does not always simply drop the decimal; it adjusts the integer part as appropriate.
When dealing with extreme values like infinity or NaN (Not-a-Number), `trunc` abides by a predefined behavior in the IEEE standard, where truncating infinity will yield infinity itself, and NaN will remain NaN.
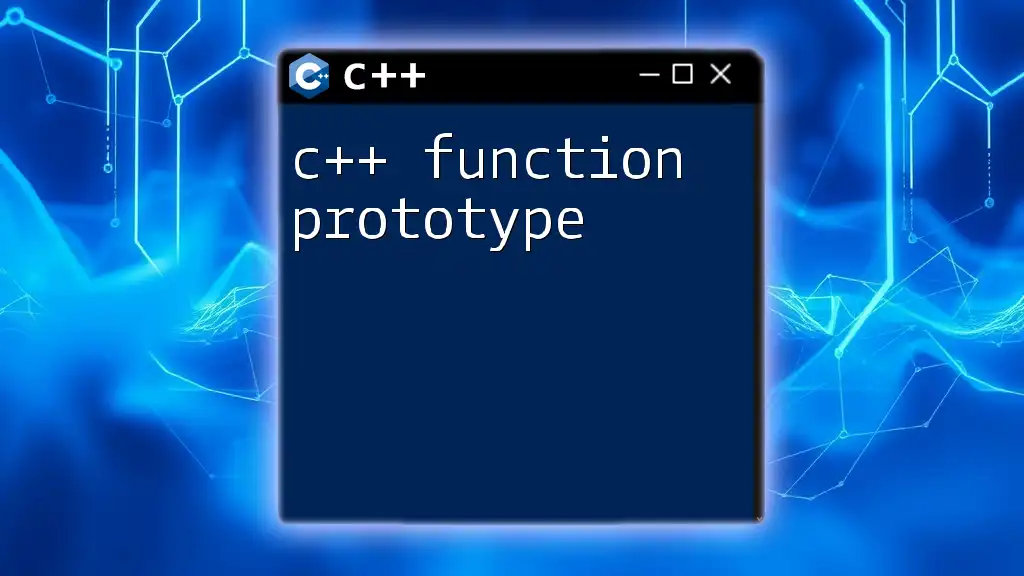
Performance Considerations
Using `trunc` is generally efficient in computational applications, as after inlining, it compiles to relatively simple assembly code. However, if you're frequently calling `trunc` in a loop or on large datasets, consider measuring performance against using other approaches. Often, using native integer operations directly can yield better performance in scenarios where truncation patterns are predictable.
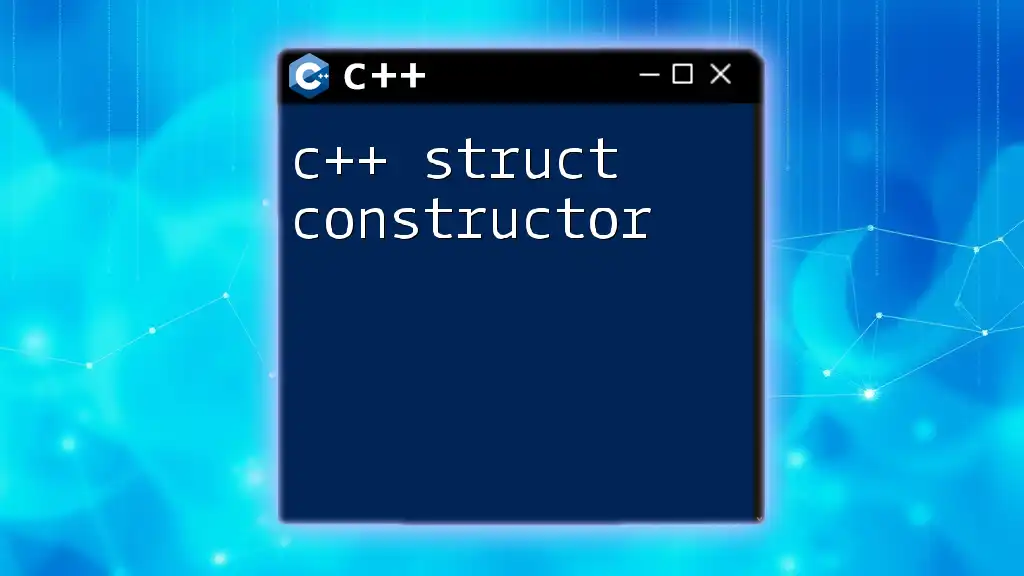
Best Practices for Using `trunc`
To ensure best practices when implementing `trunc`, follow these guidelines:
- Code Readability: Incorporate inline comments to describe the reasons why truncation is applied, especially in complex expressions. This boosts maintainability and clarity.
- Consistent Data Types: Always ensure the result of `trunc` is treated consistently in further calculations. Since `trunc` returns a `double`, be mindful of implicit type conversions that might lead to unexpected results.
- Error Handling: Verify that the value you are truncating is valid and does not involve potential pitfalls like division by zero or undefined values.
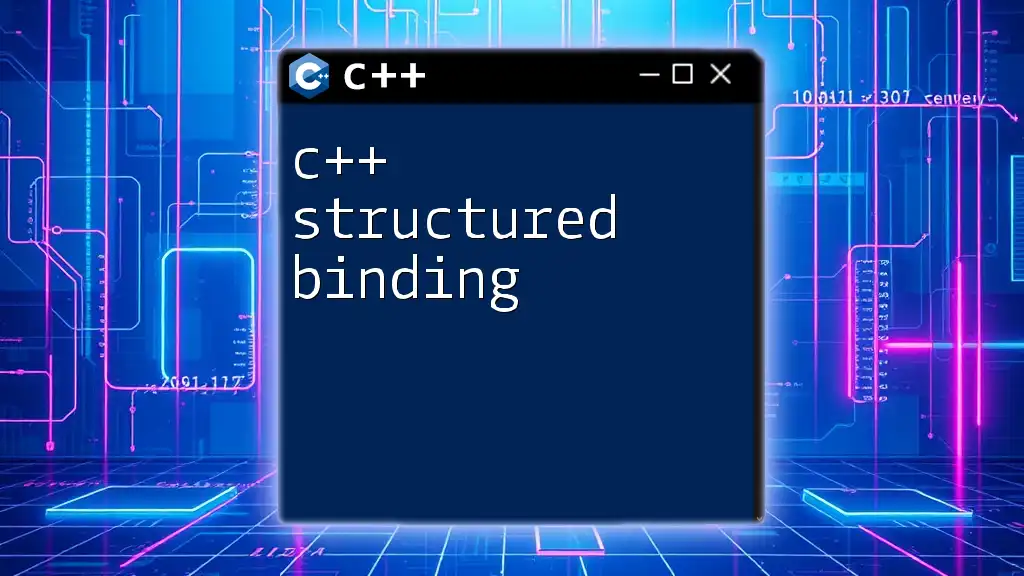
Troubleshooting Common Issues
Misunderstandings with `trunc`
There are several common misconceptions involving `trunc`. One prevalent misunderstanding is that it performs rounding. It is vital to clarify that while the result may seem similar for many positive numbers, truncation outright removes any fractional component, contrasting with rounding behavior.
Frequently Asked Questions
When faced with unexpected results from `trunc`, always examine the data types you're working with. If calculations yield values like `inf` or `NaN`, ensure that your variables are initialized correctly and that any mathematical operations are being performed as intended.
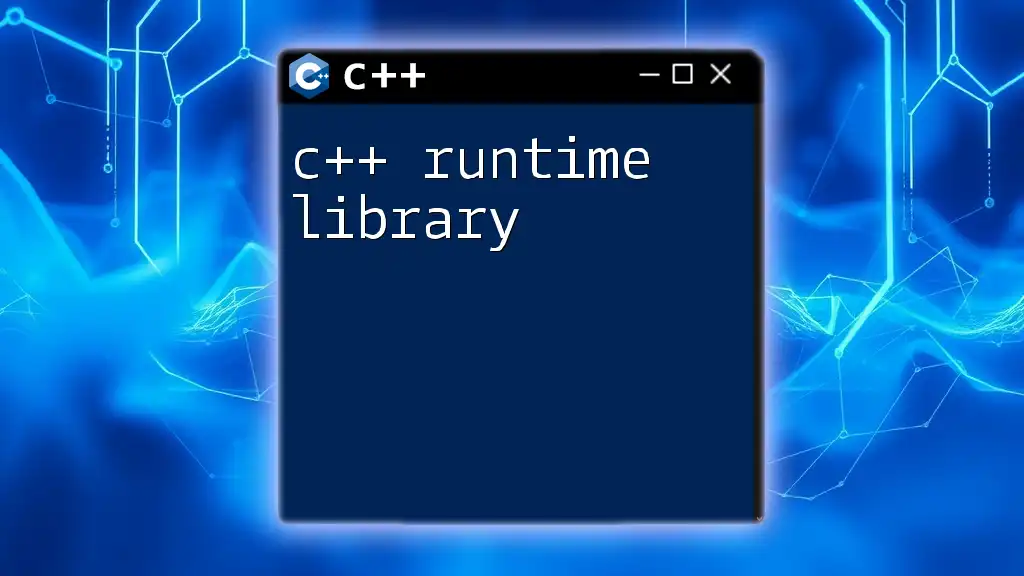
Conclusion
Understanding the `trunc` function in C++ is essential for programmers who want precise control over their numerical calculations, particularly in scenarios involving floating-point arithmetic. By employing truncation, you can maintain accuracy and relevance in your applications while simplifying your codebase. With practice, you'll see how easily it can fit into a variety of programming tasks.
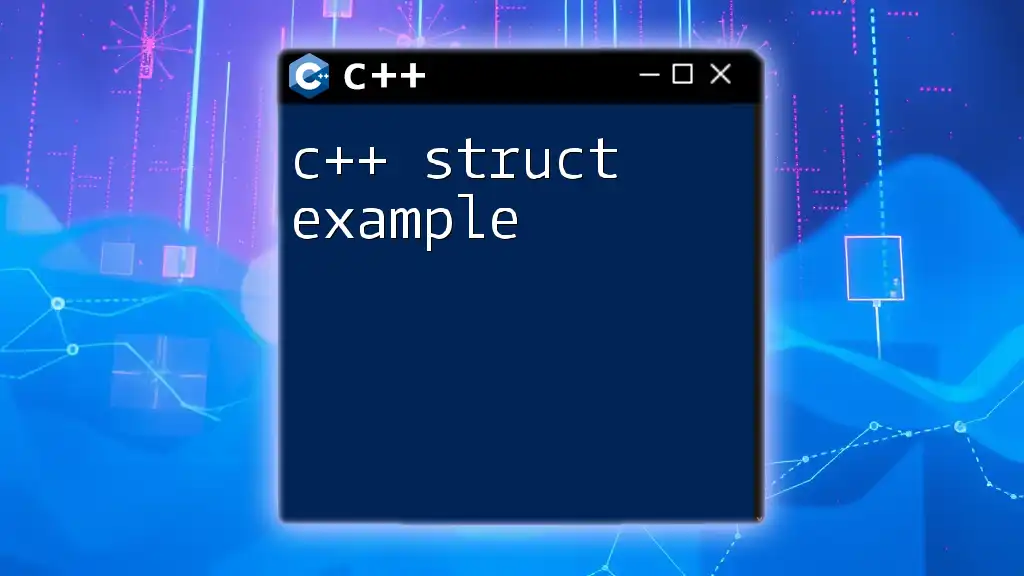
Further Resources
For continued learning, explore the official documentation on the C++ standard library and delve into tutorials focusing on mathematical functions. Expanding your knowledge in this area will provide a solid foundation for tackling numerical challenges in your programming journey.