Paho MQTT C++ is a library that facilitates MQTT messaging in C++ applications, and when using CMake to build your project, you can easily link to this library with the following configuration.
# CMakeLists.txt
cmake_minimum_required(VERSION 3.10)
project(MQTTExample)
find_package(PahoMqttCPP REQUIRED)
add_executable(MQTTExample main.cpp)
target_link_libraries(MQTTExample PahoMqttCPP::paho-mqttpp3)
Prerequisites
System Requirements
Before diving into the technical details, ensure that you have the necessary environment set up for your Paho MQTT and C++ development. You can use various operating systems, including Windows, Linux, or macOS. Ensure that you have the following tools and libraries ready:
- A C++ compiler: GCC for Linux and Visual Studio for Windows are popular choices.
- CMake: A widely used build system to manage your project builds.
Installation of Required Tools
Installing CMake is straightforward. Depending on your operating system, you can find specific instructions:
For Ubuntu/Linux:
sudo apt-get install cmake
For Windows:
- Download the installer from the [CMake official website](https://cmake.org/download/).
- Follow the installation wizard.
For macOS: You can easily install CMake using Homebrew:
brew install cmake
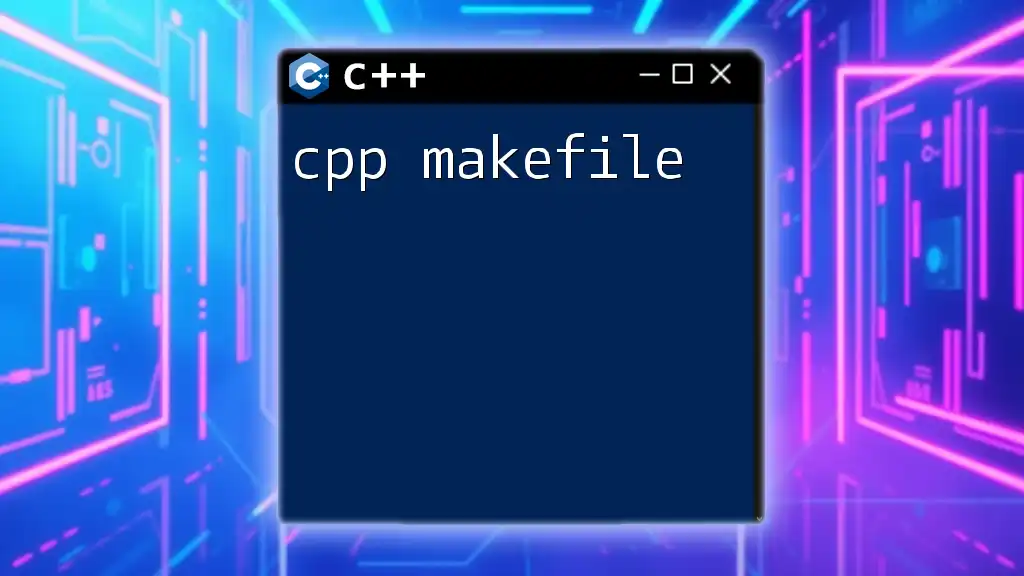
Setting Up Eclipse Paho MQTT C++ Library
Downloading Paho MQTT C++
To begin working with the Paho MQTT C++ library, you'll first need to download the library. You can clone the official repository from GitHub using:
git clone https://github.com/eclipse/paho.mqtt.cpp.git
Building Paho MQTT C++ with CMake
Once you have the library downloaded, you need to compile it using CMake. Here’s how to do it:
-
Navigate to the cloned directory: Change your current directory to the one where you've cloned the Paho MQTT repository.
-
Creating a Build Directory: To keep the source code clean, it's a good practice to create a separate directory for the build:
mkdir build && cd build
- Running CMake Configuration: Next, you'll configure the project using CMake:
cmake ..
- Compiling the Library: After successfully configuring, compile the library:
make
- Installing the Library: To use Paho MQTT in your projects, you'll need to install the compiled library:
sudo make install
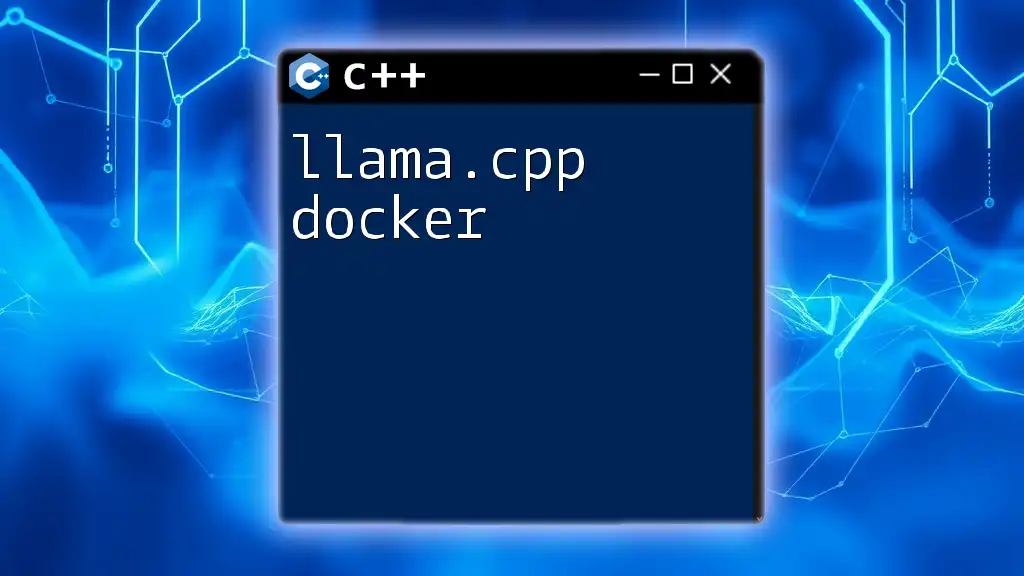
Creating Your First MQTT C++ Application
Setting Up a CMake Project
To start your own MQTT application, you must set up a new CMake project. Choose a directory where you want to create your project and set up the following structure:
/YourProject
/src
publisher.cpp
subscriber.cpp
CMakeLists.txt
Writing Your First MQTT Publisher
Introduction to MQTT Client
An MQTT client acts as both a publisher and a subscriber. Let's first look at how to create a simple MQTT publisher that sends messages to a specific topic.
Code Example: Simple MQTT Publisher
Here's a minimal code example that demonstrates how to create a simple MQTT publisher:
#include <iostream>
#include <string>
#include <mqtt/async_client.h>
const std::string SERVER_ADDRESS("tcp://localhost:1883");
const std::string CLIENT_ID("ExampleClient");
int main() {
mqtt::async_client client(SERVER_ADDRESS, CLIENT_ID);
mqtt::connect_options connOpts;
connOpts.set_clean_session(true);
try {
client.connect(connOpts)->wait();
std::cout << "Connected to the MQTT broker!" << std::endl;
const std::string topic("test/topic");
const std::string payload("Hello, MQTT!");
client.publish(topic, payload.data(), payload.size(), 0, false);
std::cout << "Message published!" << std::endl;
client.disconnect()->wait();
} catch (const mqtt::exception& exc) {
std::cerr << "Error: " << exc.what() << std::endl;
}
return 0;
}
This code connects to an MQTT broker, publishes a message to the specified topic, and disconnects afterward.
Writing Your First MQTT Subscriber
Understanding MQTT Subscriptions
Subscribers are crucial as they listen for messages on specified topics. When a message is published to any topic, all subscribers of that topic receive the message.
Code Example: Simple MQTT Subscriber
Here’s how to implement a simple MQTT subscriber:
#include <iostream>
#include <string>
#include <mqtt/async_client.h>
const std::string SERVER_ADDRESS("tcp://localhost:1883");
const std::string CLIENT_ID("ExampleSubscriber");
class MessageCallback : public virtual mqtt::callback {
public:
void message_arrived(mqtt::const_message_ptr msg) override {
std::cout << "Message arrived: '" << msg->get_payload()
<< "' on topic: " << msg->get_topic() << std::endl;
}
};
int main() {
mqtt::async_client client(SERVER_ADDRESS, CLIENT_ID);
MessageCallback cb;
client.set_callback(cb);
mqtt::connect_options connOpts;
connOpts.set_clean_session(true);
try {
client.connect(connOpts)->wait();
std::cout << "Connected to the MQTT broker!" << std::endl;
const std::string topic("test/topic");
client.subscribe(topic, 0);
// Wait for messages
std::this_thread::sleep_for(std::chrono::seconds(30));
client.disconnect()->wait();
} catch (const mqtt::exception& exc) {
std::cerr << "Error: " << exc.what() << std::endl;
}
return 0;
}
In this example, the subscriber listens for messages on the same topic and prints them out.
Compiling Your Project
Creating a CMakeLists.txt File
You need to create a `CMakeLists.txt` file to guide CMake on how to build your program. Here's an example that includes both the publisher and subscriber:
cmake_minimum_required(VERSION 3.10)
project(MQTTExample)
find_package(PahoMqttCPP REQUIRED)
add_executable(publisher src/publisher.cpp)
target_link_libraries(publisher PahoMqttCPP::paho-mqttpp3)
add_executable(subscriber src/subscriber.cpp)
target_link_libraries(subscriber PahoMqttCPP::paho-mqttpp3)
Building Your Project
Now, you can build your project:
mkdir build && cd build
cmake ..
make
After successful compilation, you will find the `publisher` and `subscriber` executables in the `build` directory.
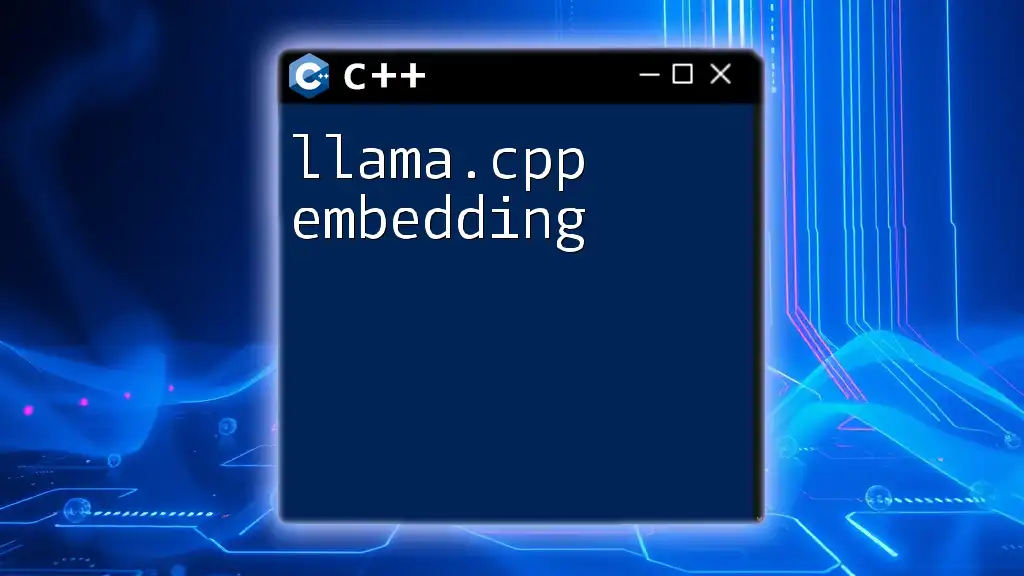
Advanced Features of Paho MQTT C++
Using Quality of Service (QoS)
Understanding QoS Levels
Quality of Service (QoS) is essential in MQTT, allowing you to specify the delivery guarantees of messages. The three levels of QoS are:
- QoS 0: At most once - Messages are delivered at most once, with no guarantee.
- QoS 1: At least once - Messages are guaranteed to be delivered but could be duplicated.
- QoS 2: Exactly once - Messages are guaranteed to be delivered exactly once, ensuring no duplication.
Implementing QoS in Your Publisher and Subscriber
You can specify QoS levels when publishing or subscribing to messages by modifying the publish and subscribe functions:
Publisher Example:
client.publish(topic, payload.data(), payload.size(), 1, false); // QoS level 1
Subscriber Example:
client.subscribe(topic, 1); // Subscribe with QoS level 1
Handling Callbacks and Messages
Implementing Callbacks for Asynchronous Calls
Callbacks allow you to handle incoming messages asynchronously and react to events (like connection loss). By implementing the `mqtt::callback` interface, you can define your custom handling logic.
Code Example for Message Callbacks
Here's how to set up a callback to handle incoming messages:
class MsgCallback : public virtual mqtt::callback {
void message_arrived(mqtt::const_message_ptr msg) override {
std::cout << "Received: '" << msg->get_payload() << "' on topic: " << msg->get_topic() << std::endl;
}
void connection_lost(const std::string& cause) override {
std::cerr << "Connection lost: " << cause << std::endl;
}
};
Connection Management
Reconnecting and Error Handling
It's crucial to handle failures gracefully. Implement retry logic to reconnect if the connection drops.
Code Example for Connection Handling
Using the example below, you can handle the connection lifecycle, ensuring your application reconnects when necessary:
void connect() {
while (!client.is_connected()) {
try {
client.connect(connOpts)->wait();
} catch (const mqtt::exception& exc) {
std::cerr << "Retrying connection: " << exc.what() << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(5));
}
}
}
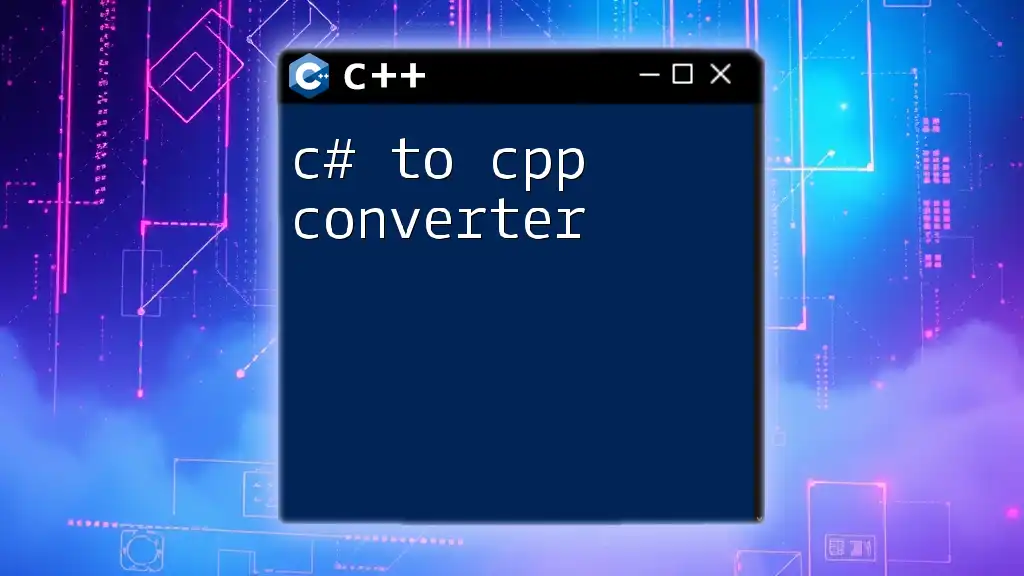
Conclusion
In this guide, you have navigated through the essential steps of using the Paho MQTT C++ library with CMake. From installation to creating your first publisher and subscriber, you’ve seen how straightforward it is to get started with MQTT in C++. Don't forget to explore Quality of Service (QoS) levels, employing callbacks, and managing connections as you build more complex applications.
As you embark on your journey with paho mqtt cpp cmake, consider exploring additional resources and engaging with community forums for ongoing support. Happy coding!