The RF24 library for C++ allows users to quickly set up and manage hope channels for wireless communication, which can be found on GitHub for easy access and implementation.
Here's a code snippet to demonstrate how to initialize the RF24 library and set a hope channel:
#include <SPI.h>
#include <RF24.h>
// Create a Radio object
RF24 radio(9, 10);
void setup() {
// Start the radio
radio.begin();
// Set the channel (e.g., channel 76)
radio.setChannel(76);
// Set other parameters as needed
radio.openReadingPipe(1, 0xF0F0F0F0E1LL);
radio.startListening();
}
void loop() {
// Your loop code here
}
Understanding RF24
What is RF24?
The RF24 library serves as a powerful communication interface for working with nRF24L01 modules, which are widely used in IoT applications such as remote control systems and sensor networks. This library simplifies the communication process, allowing developers to focus on building impressive projects rather than getting bogged down in the complexities of wireless communication.
Key Features of RF24
Among the standout attributes of RF24 are its:
- Low Power Consumption: Ideal for battery-operated devices, RF24 minimizes energy usage while maintaining efficient communication protocols.
- Ease of Use: The straightforward API of the library reduces the learning curve, enabling hobbyists and professionals alike to implement it effortlessly.
- Excellent Performance: The RF24 library excels in environments that contain noise, providing reliable data transfer across various distances.

Hop Channels: An In-Depth Explanation
What are Hop Channels?
In wireless communication, hop channels refer to the practice of rapidly switching frequencies during data transmission. This method is crucial for reducing interference and enhancing the overall reliability of communication. Hop channels can significantly improve communication resilience, making it more difficult for unauthorized users to capture signals and causing disruptions.
The Concept of Frequency Hopping
Frequency Hopping Spread Spectrum (FHSS) is a technique employed within hop channels, where the transmitter and receiver switch between different frequency channels. This hopping mechanism boosts signal reliability, allowing systems to adapt to changing channel conditions seamlessly and providing improved resistance against signal jamming and interference.

Setting Up RF24 for Frequency Hopping
Prerequisites
Before diving into configuring hop channels, ensure you have the following:
- Hardware Requirements: You will need nRF24L01 modules, an Arduino board, and appropriate connecting wires.
- Software Requirements: Download the Arduino Integrated Development Environment (IDE) and install the RF24 library obtained from GitHub.
Installing RF24 Library
To harness the capabilities of RF24, you'll need to install the library from its GitHub repository. Follow these steps for installation:
- Download the RF24 library by navigating to the official GitHub page.
- Extract the contents into the Arduino libraries folder, usually found in `Documents/Arduino/libraries/`.
- Restart the Arduino IDE to load the new library.
You can begin your sketch by including the library with the following command:
#include <RF24.h>
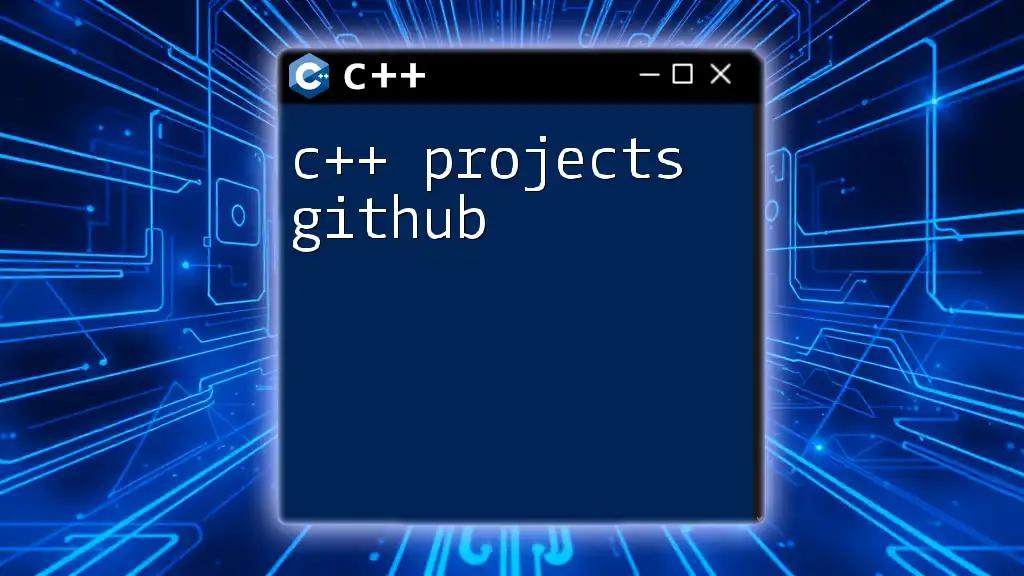
Configuring Hop Channels
Basic Configuration Setup
Getting started with RF24 requires understanding a few key configuration parameters:
- Channel Selection: Selecting an appropriate channel is critical for effective communication.
- Power Level: This setting influences the range and clarity of your communications.
Example Configuration Code
Here’s a basic example for getting started with RF24 and selecting an initial channel:
RF24 radio(9, 10); // CE, CSN pins
radio.begin();
radio.setChannel(76); // Set the initial channel
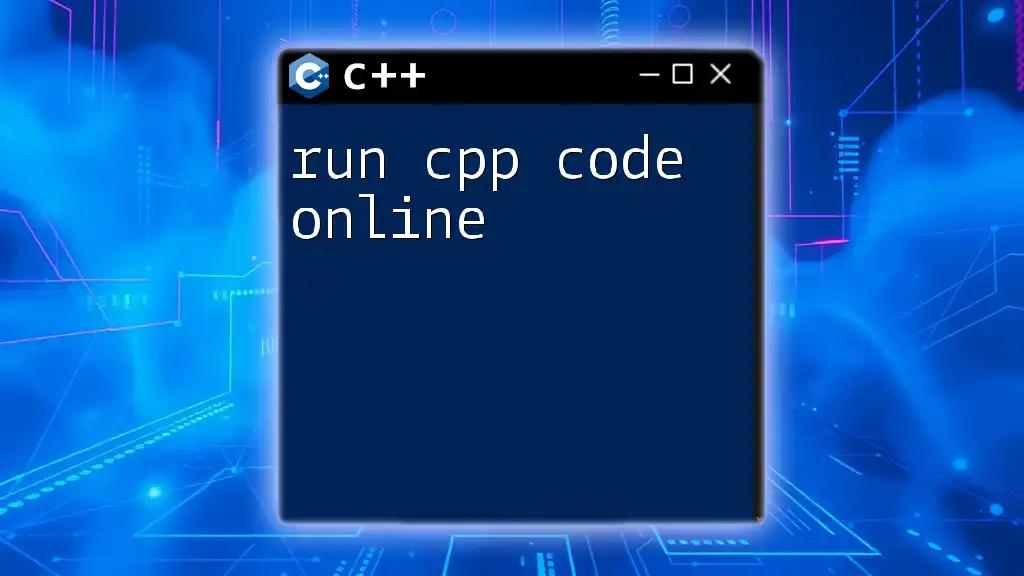
Implementing Frequency Hopping
Creating a Hopping Function
A hopping function allows your device to change its communication frequency dynamically. The design of this function is pivotal for maintaining communication reliability. Below is an example of how to create a simple hopping function:
void hopChannel() {
int newChannel = random(76, 84); // Randomly select a channel
radio.setChannel(newChannel);
}
Integrating Hopping in Communication Loop
Integrate the hopping function into your main loop to implement frequency hopping during operation. Here’s how you can do that:
void loop() {
hopChannel();
radio.write(&data, sizeof(data));
delay(1000); // Wait for a second before hopping again
}
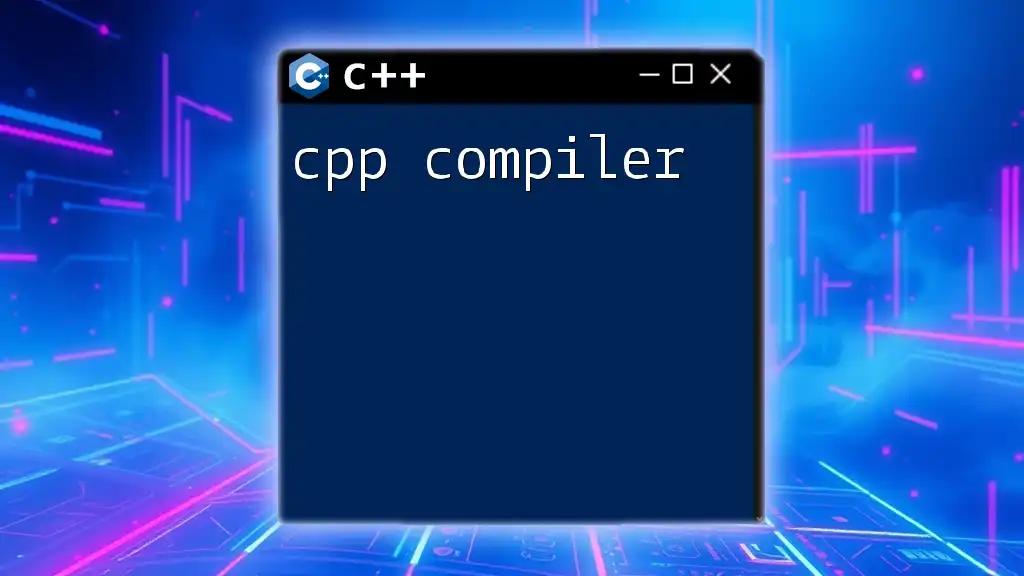
GitHub Resources
Accessing the RF24 GitHub Repository
Visit the official [RF24 GitHub repository](https://github.com/nRF24/RF24) for access to the library, documentation, and community discussions. This repository is an invaluable resource, rich with widely-used examples and support from contributors.
Exploring Examples and Contributions
The GitHub repository features numerous example sketches that showcase the complete potential of the RF24 library. Engaging with this community can enhance your understanding and inspire new project ideas. You’re also encouraged to contribute code or enhancements back to the community.
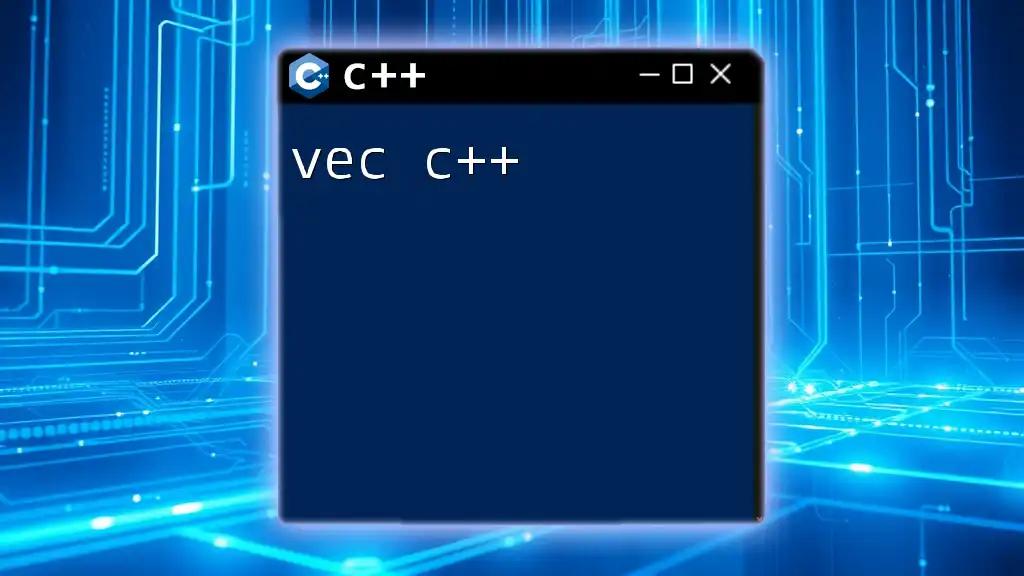
Troubleshooting Common Issues
No Communication Between Nodes
If you find that your devices aren't communicating, take a moment to review:
- Connection: Ensure that your wiring is correct.
- Power Supply: Check that your modules are correctly powered.
- Library Settings: Confirm that the settings such as channels and addresses are properly configured.
Signal Interference Problems
Signal interference can seriously impair communication. Here are strategies to mitigate interference effectively:
- Choose empty frequency channels: Use channels with the least noise for more reliable transmissions.
- Test different positions: Changing the physical layout of the devices may lead to improved communication.
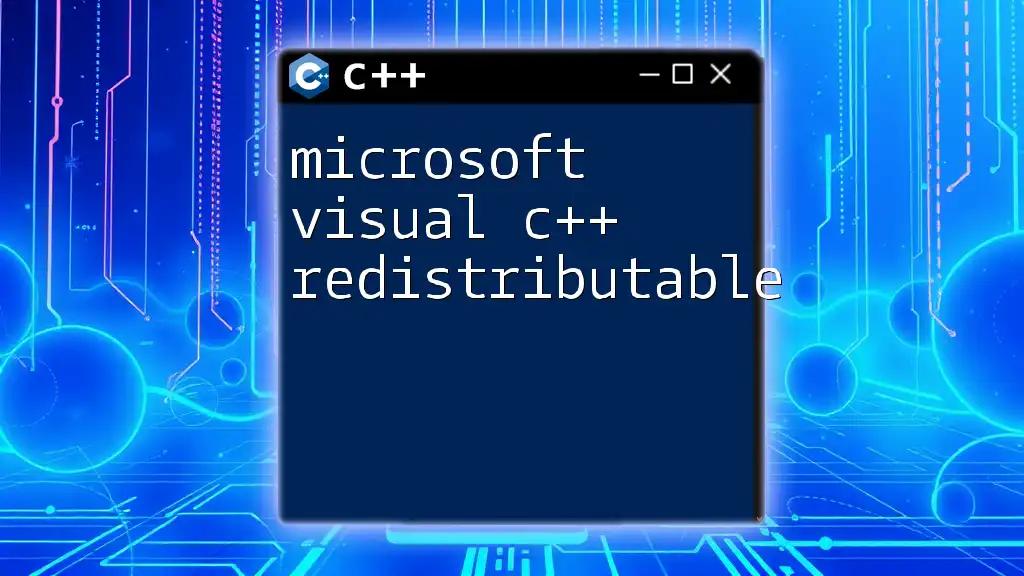
Conclusion
In summary, hop channels in RF24 communication play a critical role in enhancing the reliability of wireless communication. With the knowledge acquired from this guide, you are encouraged to explore the RF24 library further, experiment with its capabilities, and take part in the community discussions. Utilize the resources available on GitHub, and consider collaborating with others to expand your skills and create innovative IoT solutions.
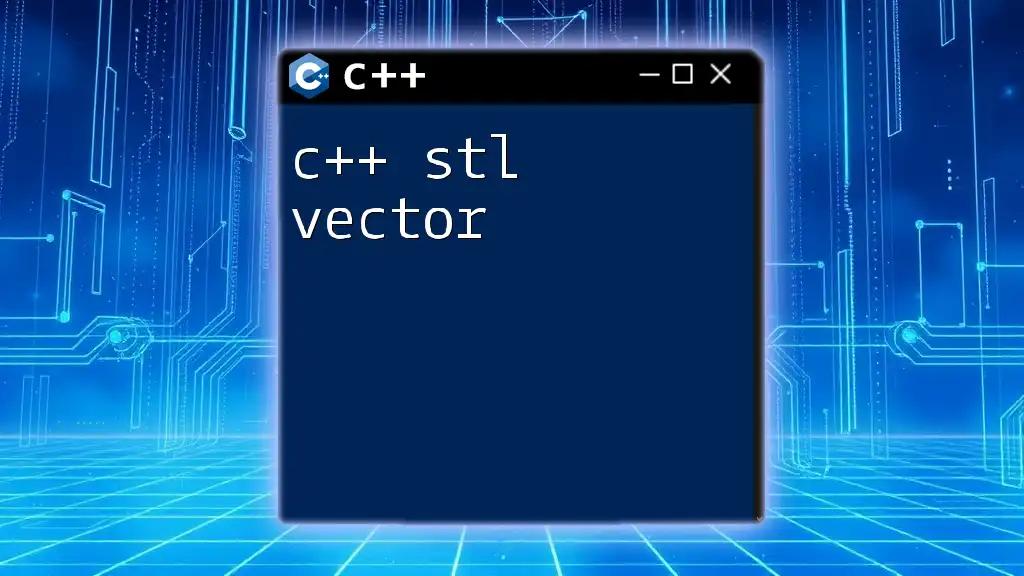
Additional Resources
To deepen your understanding of RF24 and its diverse applications, look into the following resources:
- Official RF24 documentation and example projects on GitHub.
- IoT project tutorials that leverage frequency hopping for enhanced communication.
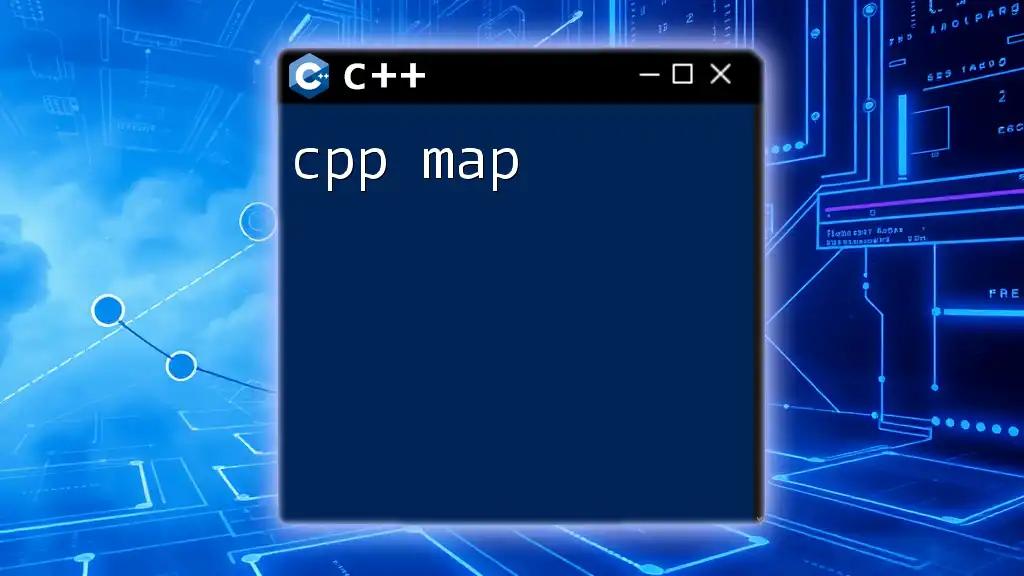
FAQs
What is the maximum range of nRF24L01 modules?
The nRF24L01 modules can achieve ranges of up to 100 meters in open areas. Factors such as interference and obstacles can significantly affect this range.
How do I know which channels to use?
When selecting channels, consider the available frequency bands, aiming to choose channels with the least noise and interference based on your application.
Can I implement hopping without using RF24?
While RF24 is a popular choice due to its simplicity, other libraries exist to achieve similar frequency-hopping functionalities, although they may require more in-depth configuration.