In C++, the stack is used for static memory allocation and local variables, while the heap is used for dynamic memory allocation, allowing for more flexible memory management.
Here's a simple code snippet demonstrating the difference:
#include <iostream>
int main() {
int stackVar = 10; // Stack allocation
int* heapVar = new int(20); // Heap allocation
std::cout << "Stack variable: " << stackVar << std::endl;
std::cout << "Heap variable: " << *heapVar << std::endl;
delete heapVar; // Free the allocated memory on the heap
return 0;
}
What is Memory Management in C++?
Memory management in C++ is a critical skill for developers, ensuring that applications run efficiently and without memory-related errors. Efficient memory management encompasses how memory is allocated, used, and freed during the program's execution. It helps reduce memory consumption and enhances the overall performance of a program, especially in larger applications.
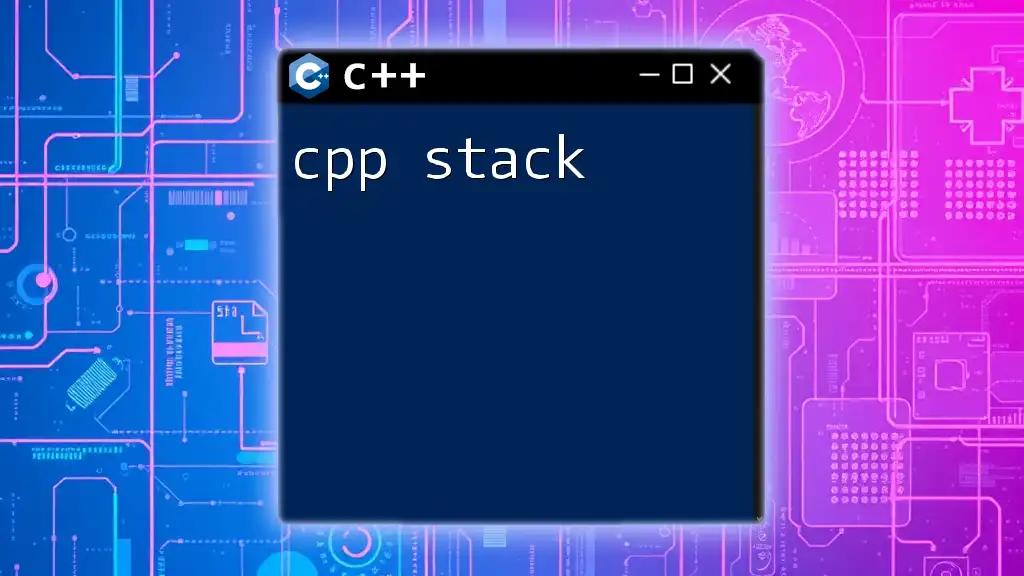
C++ Memory Types
In C++, memory can be classified into different types based on allocation methods:
Static Memory
Static memory allocation occurs at compile time. This means that the size is fixed and cannot change while the program is running. Variables declared in a function (local variables) are stored in stack memory and are cleaned up automatically when the function is exited.
Dynamic Memory
Dynamic memory allocation occurs at runtime. It allows developers to allocate memory to variables that can be adjusted based on the program's needs. This flexibility is crucial for complex applications, where the exact amount of required memory isn't known before execution.

Stack Memory
What is Stack Memory?
Stack memory is a region of memory that stores temporary variables created by a function. It follows a Last In, First Out (LIFO) principle, whereby the last variable added to the stack is the first to be removed.
Stack Memory Allocation
Stack allocation happens automatically when a function is called. When the function exits, the allocated memory is released. This is done by the program without any additional user intervention.
Code Example:
void stackExample() {
int a = 10; // Integer allocated on the stack
int b[5] = {1, 2, 3, 4, 5}; // Array allocated on the stack
} // Memory for 'a' and 'b' is released automatically
Advantages of stack memory include faster access times than heap memory and automatic memory management. Since the memory is automatically allocated and deallocated, developers do not need to worry about freeing up memory, thereby reducing the risk of memory leaks.
Limitations of Stack Memory
However, stack memory does have its limitations. There is a maximum size that varies by system, which can lead to stack overflow if too much memory is allocated on the stack. This occurs when a program uses more stack memory than is allowed, often due to excessively deep recursion or large allocations in functions.

Heap Memory
What is Heap Memory?
Heap memory is a region of memory used for dynamic memory allocation. Unlike stack memory, the heap does not have a predetermined size limit, and memory allocation occurs at runtime. This gives developers greater control over memory usage.
Heap Memory Allocation
Allocating memory on the heap is done using operators like `new` in C++. Unlike stack memory, developers must explicitly allocate and free this memory.
Code Example:
void heapExample() {
int* ptr = new int(10); // Allocating memory for an integer on the heap
// Do something with the allocated memory
delete ptr; // Important to deallocate memory to prevent memory leaks
}
The advantages of heap memory stem from its flexibility, allowing for larger and resizable data structures. However, this comes at a cost—the need for manual memory management.
Limitations of Heap Memory
The primary limitation of heap memory is its access speed, which is generally slower than stack memory due to the overhead of dynamic allocation. Furthermore, improper management can lead to memory leaks, where memory that is no longer needed is not released back to the system, causing inefficient memory usage and potential application crashes.

Key Differences Between Stack and Heap
Time of Allocation
Stack memory is allocated at compile time, while heap memory is allocated at runtime. This distinction impacts how developers choose to manage memory based on their application's requirements.
Size and Lifetime
Stack variables are removed automatically after the function returns, while heap variables persist until they are explicitly deallocated. This difference affects how variables are used and when they can be accessed.
Code Example:
void compareStackAndHeap() {
int stackVar = 20; // Stack variable
int* heapVar = new int(30); // Heap variable
// stackVar is cleaned up automatically at function exit,
// but heapVar must be deleted manually
delete heapVar;
}
Use Cases
Stack memory is ideal for small, temporary variables, especially in simple functions. In contrast, heap memory is better suited for larger data structures or when the size of the data isn't known until runtime, such as with dynamic arrays or linked lists.

Best Practices for Using Stack and Heap in C++
When to Use Stack vs Heap
Understanding when to use stack vs heap is crucial. Use stack memory for small, short-lived variables where speed is essential. Utilize heap memory for large data structures or when the lifespan of an object exceeds the scope of the function it was created in.
Managing Memory Effectively in C++
To prevent memory leaks, always ensure that every `new` has a corresponding `delete`. Moreover, consider using smart pointers from the C++ Standard Library, such as `std::unique_ptr` or `std::shared_ptr`, to automate memory management.
Code Example with Smart Pointers:
#include <memory>
void smartPointerExample() {
std::unique_ptr<int> numPtr(new int(100)); // Automatically cleans up
// No need to manually delete, it will be cleaned up automatically
}
Performance Considerations
The choice between stack and heap can significantly influence an application’s performance. Developers should measure the impact of memory allocation strategies in their specific context, as creating too many heap allocations can lead to performance penalties.
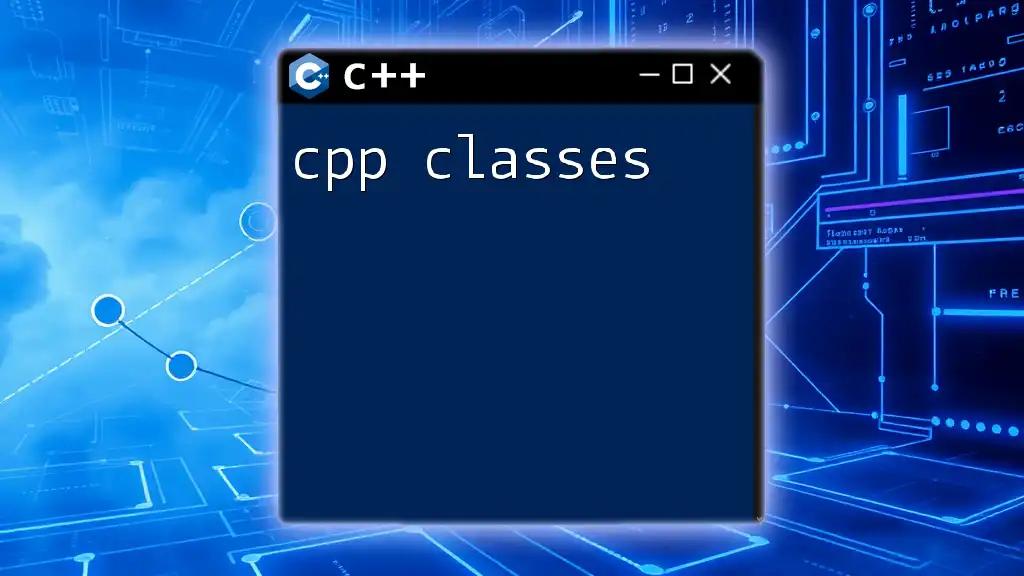
Conclusion
Understanding cpp heap vs stack is fundamental for effective memory management in C++. By recognizing their differences, advantages, and limitations, developers can make informed decisions that enhance their application’s performance and stability. As you dive deeper into memory management in C++, consider how these principles apply to your coding practices and improve your ability to write efficient, reliable code.

Additional Resources
For those interested in continuing their journey in C++ programming, explore books, online tutorials, and community forums dedicated to advanced memory management and performance optimization techniques. Following this guide will also keep you updated with more C++ programming insights and best practices.