"Stack smashing detected" is an error message that occurs in C++ when a program writes more data to a buffer than it can hold, potentially leading to a buffer overflow, which can compromise the integrity of the stack.
Here's a simple code snippet that demonstrates stack smashing through an array overflow:
#include <iostream>
void vulnerableFunction() {
char buffer[10];
// This line will overflow the buffer and potentially cause stack smashing
for(int i = 0; i <= 10; ++i) {
buffer[i] = 'A'; // Writing beyond the allocated buffer size
}
}
int main() {
vulnerableFunction();
return 0;
}
Understanding Stack Smashing
Stack smashing is a critical vulnerability in programming, particularly in C++. It occurs when a program writes more data to a buffer than it can hold, leading to the corruption of adjacent memory on the stack. This results in undefined behavior and may cause program crashes or, in the worst cases, security vulnerabilities that hackers can exploit.
The significance of understanding stack smashing cannot be understated. It highlights the need for safe coding practices that prevent this kind of overflow from compromising software integrity and security.
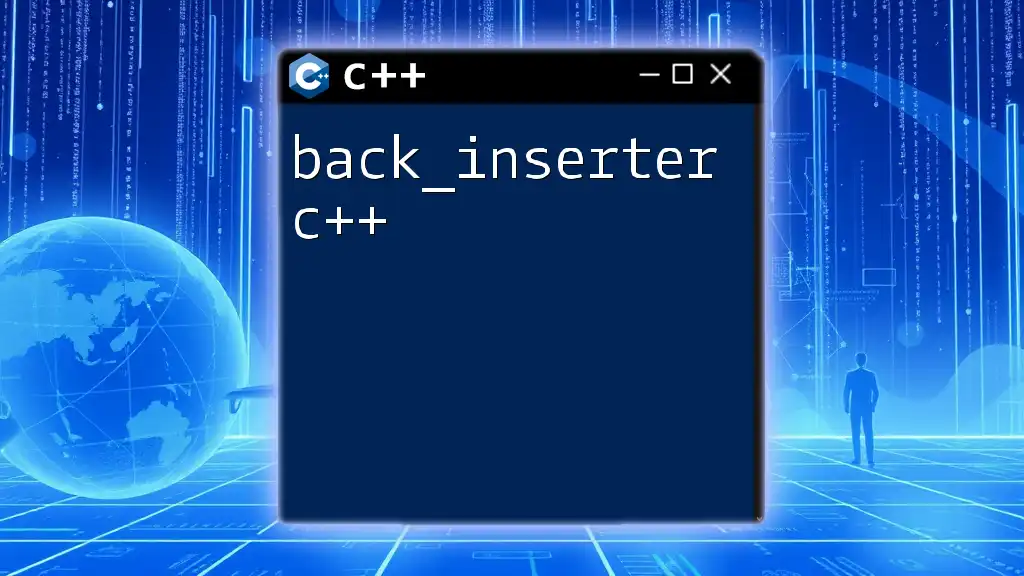
The C++ Stack and Memory Management
What is a Stack?
In C++, the stack is a region of memory used for dynamic memory allocation, typically associated with function calls and local variables. Each time a function is called, a new "frame" is pushed onto the stack, storing parameters and local variables. Once the function completes, this frame is popped off, freeing the memory.
Understanding this model is crucial because the stack has limited size, and exceeding it can lead to serious issues such as stack smashing. Proper management of stack memory is key to efficient and secure programming.
Understanding Memory Overflows
Memory overflow occurs when data written exceeds the buffer size allocated on the stack. This can lead to overwriting adjacent memory addresses, including return addresses and other important stack metadata.
Example Code Snippet: Basic Overflow
#include <iostream>
#include <cstring>
void vulnerableFunction() {
char buffer[10];
strcpy(buffer, "This string is too long"); // Potential overflow
}
In the above example, `strcpy` is used to copy a string into a fixed-size buffer. If the input string exceeds ten characters, it will overflow, leading to potential stack corruption and triggering the "stack smashing detected" message from modern compilers.
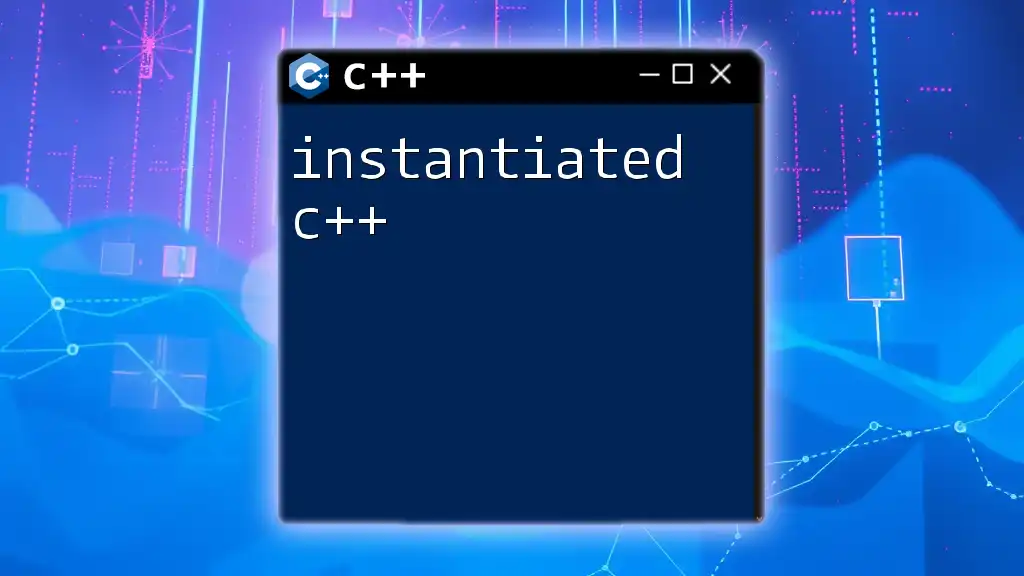
Identifying Stack Smashing
Compiler Warnings and Error Messages
When stack smashing occurs, compilers usually provide warnings or error messages that can help developers diagnose issues. Common phrases associated with such events include “stack smashing detected” or “buffer overflow.”
Understanding these messages is the first step in troubleshooting vulnerabilities and ensuring that buffer overflows do not go unchecked in your C++ applications.
Using Debuggers and Tools
To identify stack smashing effectively, developers can utilize various debugging tools. Tools like Valgrind and AddressSanitizer are invaluable in detecting memory-related issues.
To set up AddressSanitizer, you can compile your code with the `-fsanitize=address` flag:
g++ -fsanitize=address -g -o program program.cpp
Running this command provides detailed information on memory allocation and can reveal instances of stack smashing, making it easier to pinpoint and correct vulnerabilities in your code.
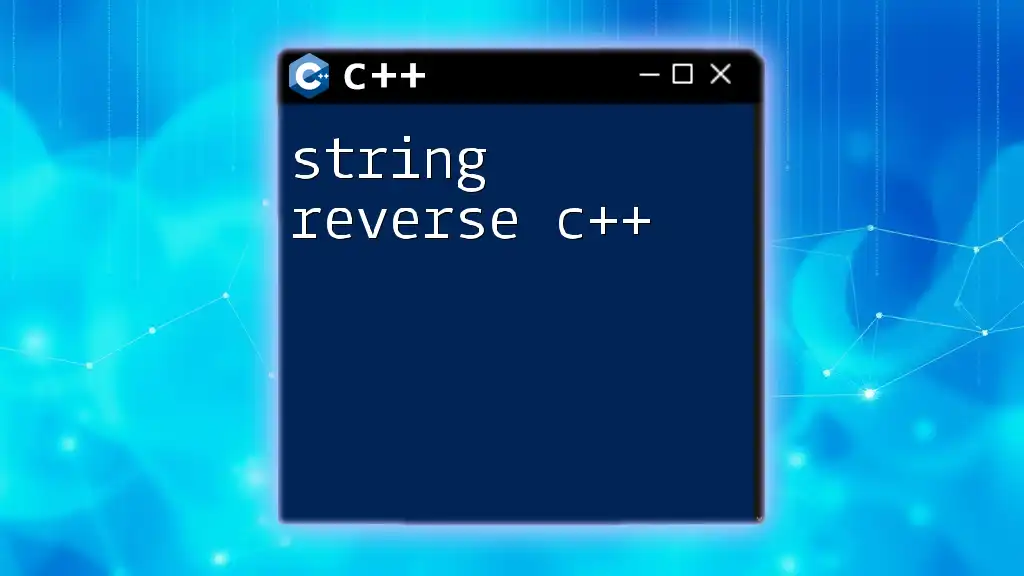
Preventing Stack Smashing
Safe Coding Practices
Implementing safe coding practices plays a crucial role in preventing stack smashing. Here are some strategies to consider:
Bounds Checking
Always ensure that you check the lengths of inputs when using arrays and buffers. This protective measure helps prevent overflow when handling user input or external data.
Example Code Snippet: Safe String Handling
#include <iostream>
#include <string>
void safeFunction() {
std::string safeBuffer(10, '\0'); // Initialize a safe buffer
std::cin >> safeBuffer; // Safe input handling
}
By using `std::string`, you not only prevent stack smashing through dynamic memory management, but you also improve code readability and safety.
Using Standard Libraries
C++ provides several standard libraries designed to mitigate the risks associated with manual memory management. Utilizing classes such as `std::vector` and `std::string` assures automatic bounds checking and dynamic memory allocation.
Compiler Flags for Security
Enhance your code's safety by enabling specific compiler flags. Options such as `-fstack-protector` add a layer of protection against stack smashing by inserting code that checks for buffer overflows.
Example Compiler Flag for GCC
g++ -fstack-protector -o secure_program secure_program.cpp
This command enables stack protection for your program, helping catch any potential vulnerabilities at runtime.
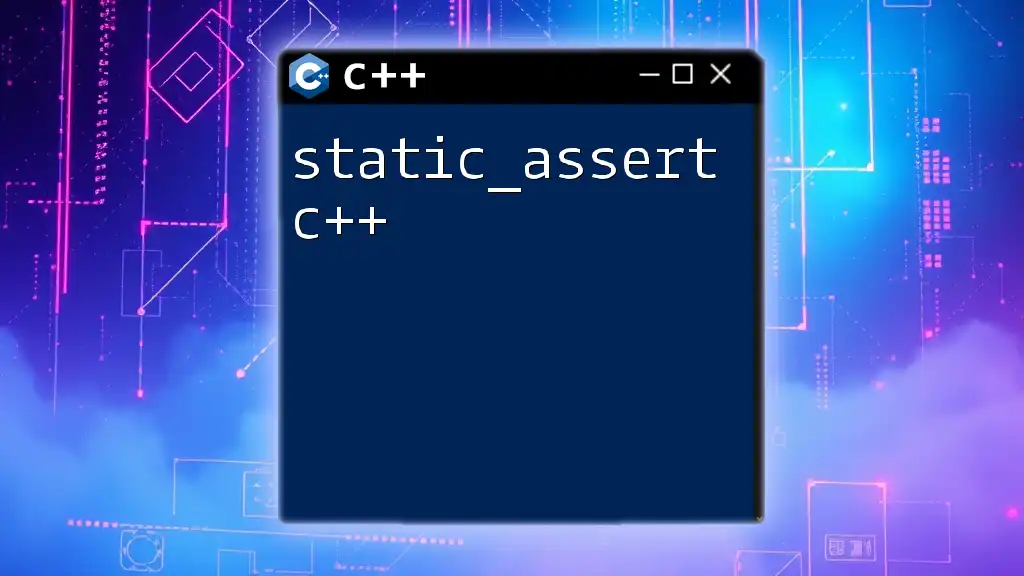
Diagnosing Stack Smashing Issues
Common Patterns of Vulnerability
Certain coding practices increase the risk of stack smashing. Pay close attention to code structures that involve deep recursion or complex loop nesting, which can exhaust stack space, leading to overflow.
Analyzing Core Dumps and Logs
When your program crashes due to stack smashing, analyzing core dumps can provide insight into what went wrong. Core dumps contain a snapshot of the program's memory at the time of the crash, which aids in diagnosing stack corruption.
To examine a core dump, use the following command with gdb:
gdb ./your_program core
This command loads the program and its core dump, allowing you to explore the cause of the stack smashing incident.
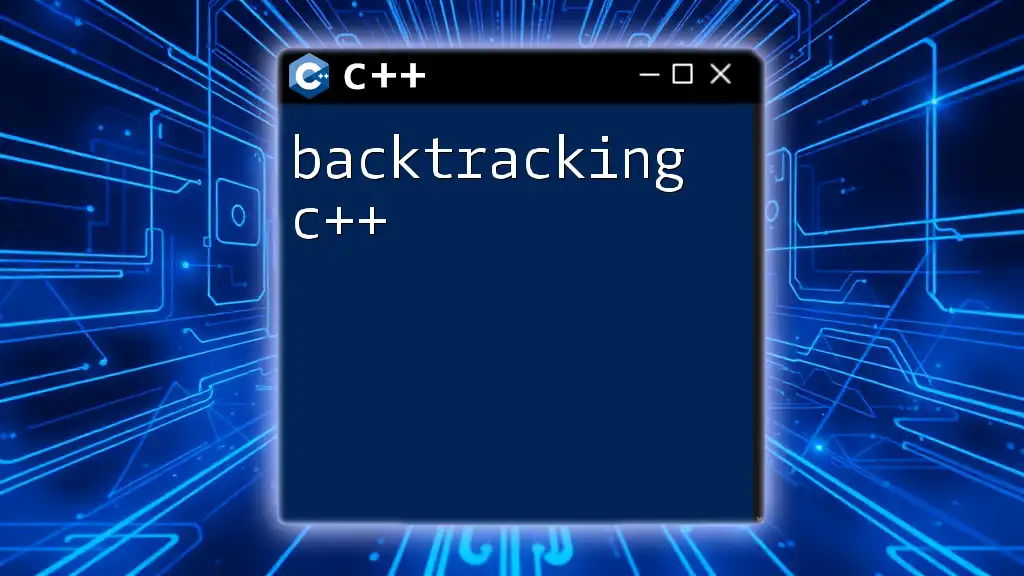
Conclusion
Awareness of stack smashing is crucial for any C++ developer. By understanding the mechanics behind stack memory, identifying potential vulnerabilities, and implementing safe coding practices, you can significantly reduce the risks associated with stack smashing in your applications. Always remember to check for buffer overflows, utilize the powerful tools provided by modern compilers, and employ safe coding techniques—doing so will help ensure that your C++ code remains robust and secure.
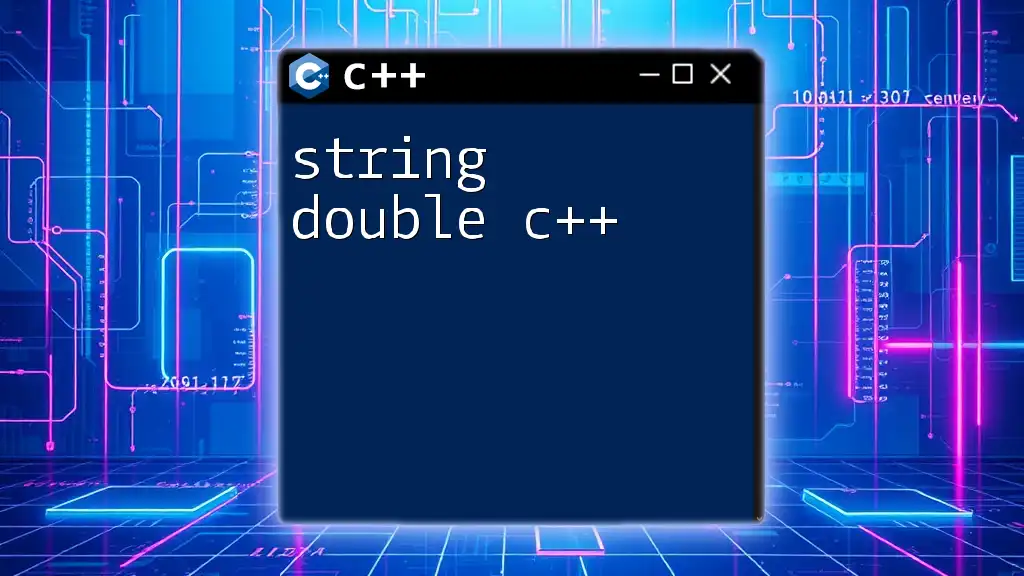
Additional Resources
For those seeking to deepen their understanding of safe C++ coding practices, consider exploring reputable books and online courses that focus on memory management and security vulnerabilities. Additionally, take advantage of useful online tools and community resources to enhance your knowledge and skills in C++.