C++ advanced concepts encompass features such as templates, polymorphism, and smart pointers that enhance the versatility and efficiency of the language, allowing for more complex and robust software development.
Here's a simple example illustrating templates:
#include <iostream>
using namespace std;
template <typename T>
T add(T a, T b) {
return a + b;
}
int main() {
cout << "Sum of integers: " << add(5, 3) << endl;
cout << "Sum of doubles: " << add(5.5, 3.2) << endl;
return 0;
}
Understanding Advanced C++ Concepts
What are Advanced C++ Concepts?
Advanced C++ concepts delve into sophisticated features and techniques that extend beyond basic programming skills. These include elements of object-oriented programming, templates, the Standard Template Library (STL), smart pointers, and more. Mastering these concepts is essential for optimizing code efficiency, enhancing performance, and effectively managing resources in complex applications.
Why Learn Advanced C++?
Understanding advanced C++ concepts prepares developers for real-world programming challenges. Many industries, such as finance, game development, and system-level programming, rely heavily on C++ for performance-critical applications. Familiarity with advanced C++ concepts ensures robust designs that leverage the full power of the language, leading to better software solutions that stand the test of time.

Advanced Object-Oriented Programming
Inheritance in Depth
Inheritance is a crucial feature of object-oriented programming that allows a class to inherit properties and behavior from another class. It promotes code reuse and enhances maintainability.
- Single Inheritance occurs when a subclass inherits from one superclass.
- Multiple Inheritance allows a subclass to inherit from more than one superclass, providing greater flexibility but also complexity, such as the possibility of the Diamond Problem.
- Multilevel Inheritance is when a class is derived from another derived class.
Each type has distinct benefits and challenges that developers must navigate. Understanding the implications of each type of inheritance helps prevent issues like ambiguity and helps optimize the class hierarchy.
Code Snippet:
class Base {
public:
void display() { cout << "Base class"; }
};
class Derived : public Base {
public:
void display() { cout << "Derived class"; }
};
Polymorphism: The Essence of OOP
Polymorphism allows a single interface to represent different underlying forms (data types). This capability is fundamental in software design since it promotes flexibility and the ability to extend functionalities seamlessly.
Compile-Time Polymorphism
Compile-time polymorphism can be achieved through function overloading and operator overloading, allowing multiple functions with the same name to coexist as long as their signatures are distinct.
Code Snippet:
class Math {
public:
int add(int a, int b) { return a + b; }
double add(double a, double b) { return a + b; }
};
Run-Time Polymorphism
Run-time polymorphism utilizes virtual functions, enabling method overriding in derived classes. The `virtual` keyword signifies that a function's implementation can be completed within derived classes, enhancing flexibility in how objects behave.
Code Snippet:
class Base {
public:
virtual void show() { cout << "Base class"; }
};
class Derived : public Base {
public:
void show() override { cout << "Derived class"; }
};
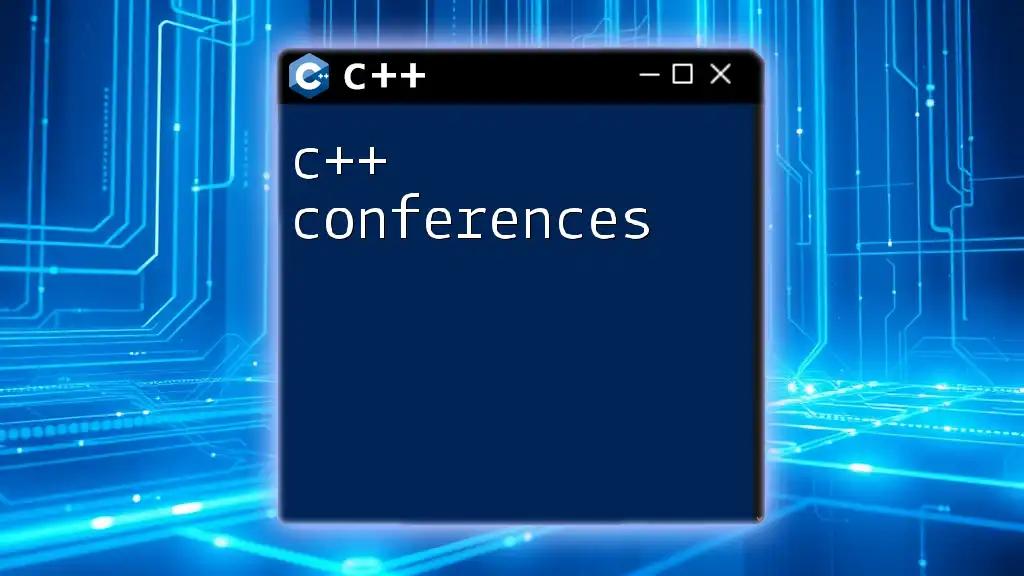
Mastering Templates
What are Templates?
Templates enable C++ developers to create functions and classes that operate with any data type. They are essential for writing type-safe and reusable code without having to implement multiple versions of the same algorithm or class.
Template Specialization
Template specialization allows you to define specific behaviors for particular types within a template. This feature enhances the flexibility of templates while providing tailored functionality for unique data types.
Code Snippet:
template <typename T>
class Container {
T element;
public:
Container(T arg) : element(arg) {}
T getElement() { return element; }
};
template <>
class Container<int> {
int element;
public:
Container(int arg) : element(arg) {}
int getElement() { return element * 2; }
};

Deep Dive into the Standard Template Library (STL)
Understanding STL Components
The Standard Template Library is a powerful library in C++ that provides a set of reusable components, including containers (like `vector`, `list`, and `map`), iterators, algorithms, and function objects. STL allows developers to write efficient and concise code, reducing the effort required to implement common data structures and algorithms.
Using Standard Containers
Containers are key components of STL, designed to manage collections of objects. Each container offers specific functionalities catering to different needs, such as performance and memory usage.
Code Snippet:
#include <vector>
#include <iostream>
using namespace std;
vector<int> nums = {1, 2, 3, 4, 5};
for (auto num : nums) {
cout << num << " ";
}
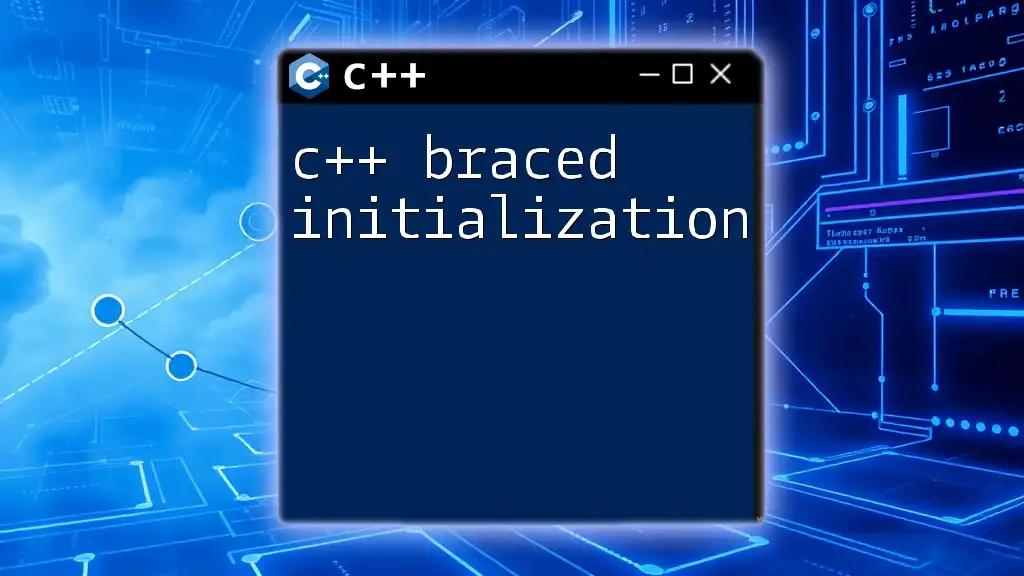
Smart Pointers: Memory Management in Modern C++
Introduction to Smart Pointers
Smart pointers provide a robust way to manage dynamic memory in C++. Unlike raw pointers, they automatically handle resource management, significantly reducing memory leaks and pointer-related errors.
Smart pointers include `unique_ptr`, `shared_ptr`, and `weak_ptr`, each serving different use cases. For example, `unique_ptr` owns its resource exclusively, while `shared_ptr` allows multiple smart pointers to share ownership of the same resource.
Managing Resource Leak with Smart Pointers
Using smart pointers simplifies memory management by ensuring that resources are released automatically when no longer in use, contributing to safer code.
Code Snippet:
#include <memory>
#include <iostream>
using namespace std;
void example() {
std::unique_ptr<int> p(new int(10));
cout << *p;
}

Advanced Techniques in C++
Operator Overloading: Customizing Behavior
Operator overloading allows developers to define custom behavior for operators when applied to user-defined types. This enhances the intuitiveness of the code and allows objects to be manipulated in familiar ways.
Code Snippet:
class Complex {
float real, imag;
public:
Complex operator + (const Complex& obj) {
return Complex(real + obj.real, imag + obj.imag);
}
};
Exception Handling
C++ provides a robust mechanism for error handling through exceptions. This advanced feature allows for cleaner error management and enhances program resilience. Understanding how to effectively use try, catch, and throw statements is critical for writing robust applications.
Code Snippet:
try {
// code that may throw an exception
} catch (const exception& e) {
cerr << "Exception caught: " << e.what();
}

Conclusion
In conclusion, C++ advanced concepts are essential for developers aiming to create efficient, maintainable software applications. Mastering these concepts not only bolsters your programming skill set but also prepares you for tackling complex challenges in the field. Continuous learning and practice in these areas will set you apart as a proficient C++ developer, paving the way for innovative and high-performance coding solutions.

Call to Action
Ready to deepen your knowledge of advanced C++ programming? Subscribe for more insightful programming tutorials and hands-on workshops. Share your questions and experiences as you embark on your C++ learning journey!