C++ can be integrated with R to leverage the speed and efficiency of C++ for intensive computations while utilizing R's powerful statistical capabilities.
#include <Rcpp.h>
using namespace Rcpp;
// [[Rcpp::export]]
double cppSum(NumericVector x) {
return sum(x);
}
Setting Up Your Environment
To get started with C++ and R, you first need to set up your environment. This involves installing R and RStudio, as well as the necessary R packages.
Installing R and RStudio
Begin by downloading and installing R from its official website. Follow the instructions for your operating system—Windows, macOS, or Linux. After installing R, download RStudio, which is an integrated development environment (IDE) that makes working with R more user-friendly.
Installing Rcpp Package
The Rcpp package is crucial for integrating C++ into R. Here’s how to install it via the R console:
install.packages("Rcpp")
Rcpp provides a seamless interface to call C++ functions from R, enabling users to leverage the performance capabilities of C++ alongside the extensive data manipulation strengths of R.
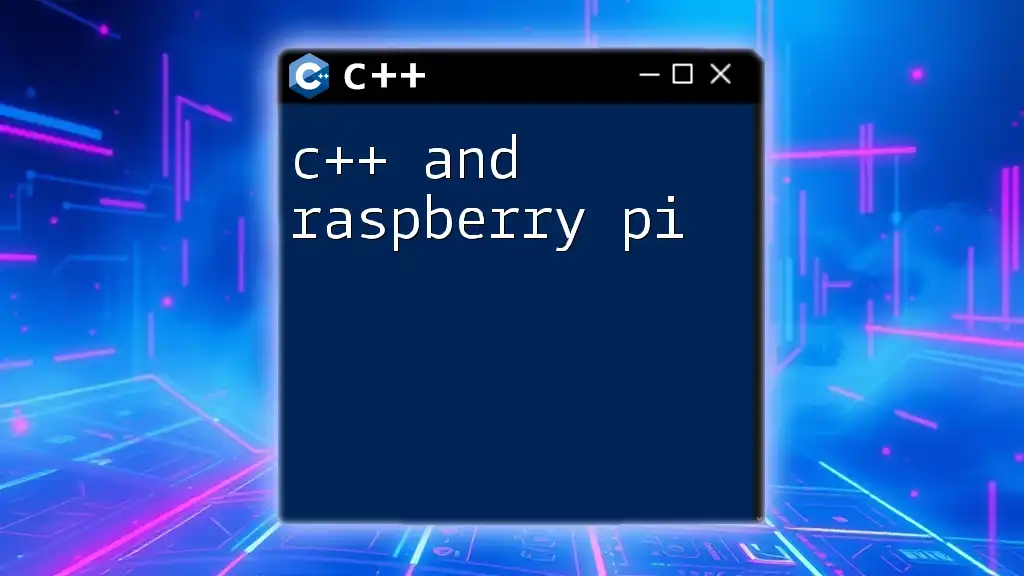
Basics of C++ in R
Understanding Rcpp Basics
Rcpp serves as the bridge between R and C++. It allows R users to write C++ code directly in R scripts and provides functions that facilitate calling C++ functions. The two primary functions you will frequently use are:
- cppFunction(): Allows you to define simple C++ functions inline.
- sourceCPP(): Compiles and sources an external C++ file that you write.
Writing Your First C++ Function in R
Let's start with writing a basic C++ function that adds two numbers. Here’s how to define this function:
// [[Rcpp::export]]
int add(int x, int y) {
return x + y;
}
The `[[Rcpp::export]]` tag indicates that this function can be called from R.
Using the C++ Function in R
To use the above C++ function in R, you need to source the CPP file or use cppFunction() directly. Here’s how to do both:
- If it's in a file named `add.cpp`, you would do:
library(Rcpp)
sourceCPP("path/to/your/add.cpp")
result <- add(5, 7)
print(result) # Output: 12
- Alternatively, using cppFunction() directly:
library(Rcpp)
cppFunction('int add(int x, int y) {
return x + y;
}')
result <- add(5, 7)
print(result) # Output: 12
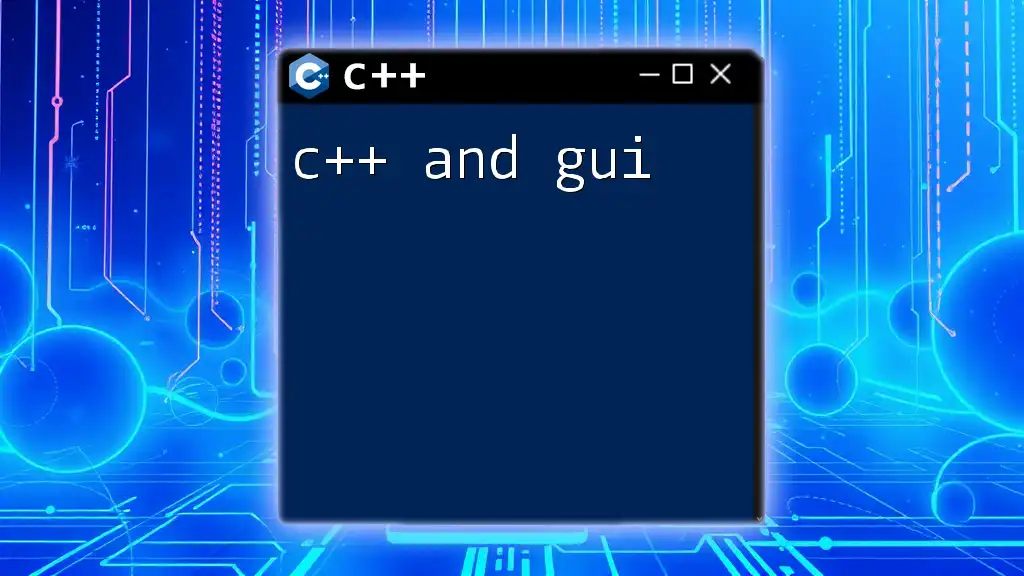
Advanced C++ Features in R
Using Vectors and Matrices
C++ can manipulate R data structures directly, such as vectors and matrices. When you pass R vectors to C++, you can perform operations efficiently. Here’s an example of a C++ function that adds two R vectors:
// [[Rcpp::export]]
NumericVector addVectors(NumericVector x, NumericVector y) {
return x + y;
}
In R, you can call this function like so:
vec1 <- c(1.0, 2.0, 3.0)
vec2 <- c(4.0, 5.0, 6.0)
result <- addVectors(vec1, vec2)
print(result) # Output: 5.0 7.0 9.0
Handling Lists and Data Frames
C++ can also work with more complex R structures like lists and data frames. Here’s how you can manipulate R lists in C++:
// [[Rcpp::export]]
List modifyList(List x) {
NumericVector vec1 = x["vec1"];
NumericVector vec2 = x["vec2"];
return List::create(Named("sum") = vec1 + vec2);
}
In R, calling this function requires you to provide a list:
my_list <- list(vec1 = c(1, 2), vec2 = c(3, 4))
result_list <- modifyList(my_list)
print(result_list) # Output: $sum [1] 4 6
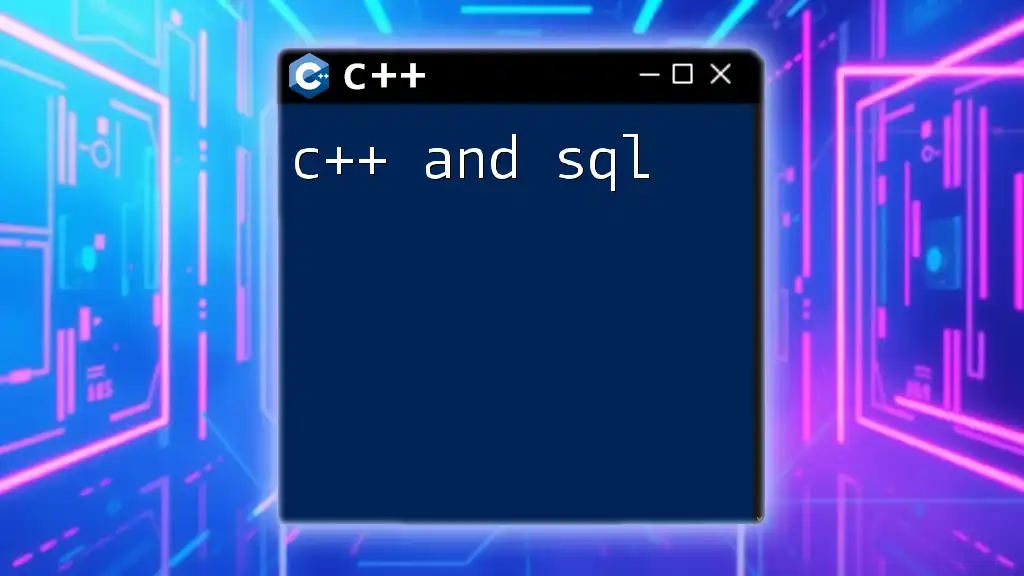
Performance Benefits of Using C++ in R
The combination of C++ and R dramatically enhances performance for computationally intensive tasks. C++ is faster due to its low-level programming capabilities. By integrating C++ into R scripts, you can significantly reduce execution times for algorithms and data processing.
For example, multiplicative operations or simulations can run much faster when coded in C++ than in pure R. Profiling your R code using tools like `Rprof` or the `microbenchmark` package allows you to measure performance improvements quantitatively.
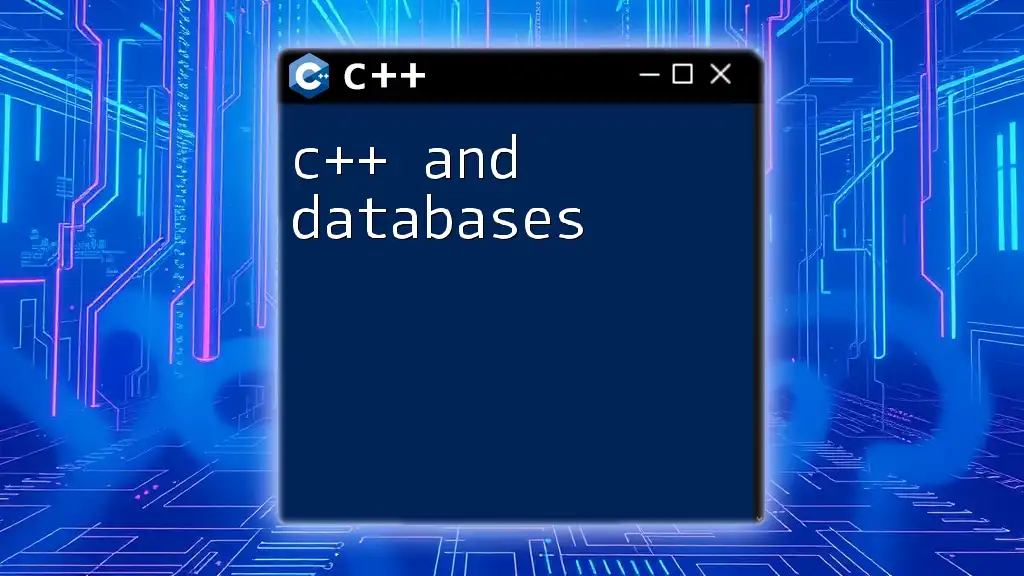
Debugging and Optimization Techniques
Debugging C++ Code in R
Debugging may be challenging when integrating C++ into R. Using a good debugging tool, such as RStudio’s integrated debugger, can help catch common errors. Additionally, familiarizing yourself with usual pitfalls, like mismatched data types or memory allocation errors, is beneficial.
Optimizing C++ Code for R
Performance optimization in C++ can be crucial. Key strategies include:
- Memory Management: Understanding and managing memory can prevent leaks and improve performance.
- Algorithm Efficiency: Implementing more efficient algorithms or data structures frequently yields better performance.
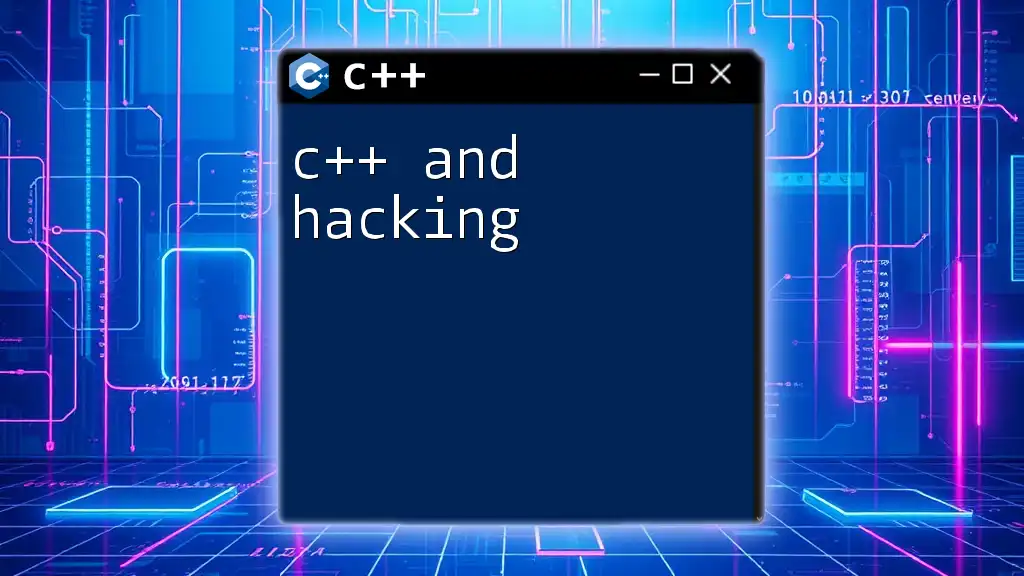
Real-world Applications of C++ in R
The C++ and R integration has found itself in various applications across fields. For statistical modeling, complex regression models can be implemented in C++, allowing for faster computation times.
In machine learning, many R packages rely on C++ for model training, enabling users to exploit advanced algorithms for big data analysis. Lastly, when conducting large data analyses, C++ can handle more complex calculations quickly and efficiently, making it ideal for real-time data applications.
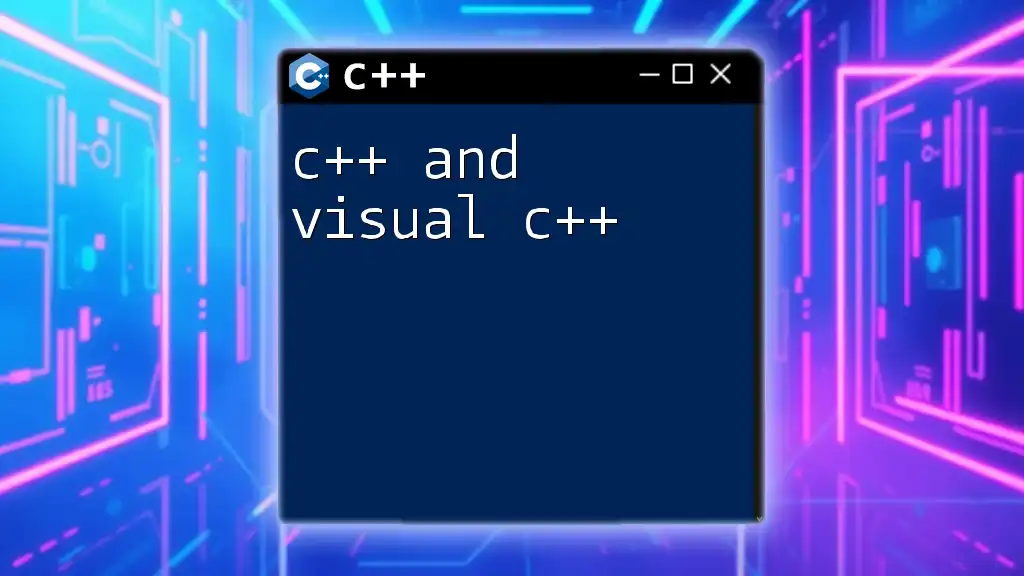
Conclusion
Combining C++ and R provides immense power for developers and data scientists. With the ability to harness the speed of C++, you can enhance R's capabilities for building fast, efficient applications. The growing trend of using C++ with R sets the stage for continued innovation and efficiency in data analysis.
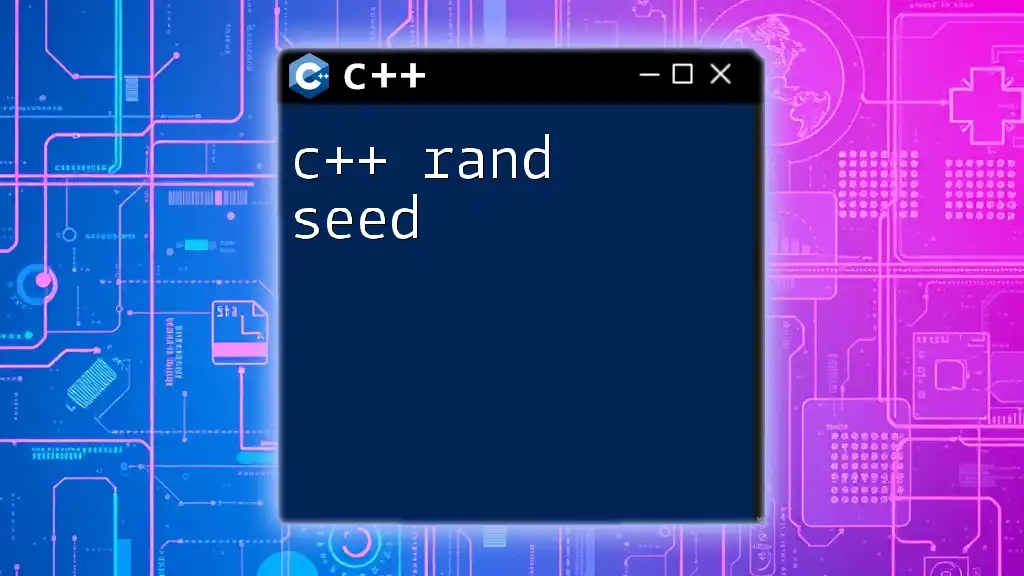
Further Resources
To deepen your knowledge further, consider exploring the following:
- Books and tutorials on integrating C++ with R.
- Online communities and forums where you can ask questions and learn from experienced practitioners.
This combination of resources will support your journey into mastering C++ and R, enabling you to create powerful and efficient analytical tools.