In C++, the `pop_back()` function is used to remove the last element from a `std::vector` or `std::string`, effectively reducing its size by one.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.pop_back(); // Removes the last element (5)
for (int num : numbers) {
std::cout << num << " "; // Output: 1 2 3 4
}
return 0;
}
Understanding Stacks in C++
What is a Stack?
A stack is a collection of elements that follows the Last In First Out (LIFO) principle, meaning the last element added to the stack will be the first one to be removed. This structure behaves similarly to a stack of plates: you can only add or remove the top plate.
Real-world analogies include:
- A stack of books where you can only remove the top book.
- Undo mechanisms in applications where the most recent action is the first to be undone.
Stack Operations
Key Stack Operations
In C++, stacks primarily support three operations:
- Push: This operation adds an element to the top of the stack.
- Pop: This operation removes the top element from the stack.
- Top: This operation retrieves the top element without removing it.
C++ Stack Implementation
C++ provides a convenient way to work with stacks through its Standard Template Library (STL). To implement a stack, you can include the `<stack>` header and utilize the `std::stack` class.
#include <iostream>
#include <stack>
int main() {
std::stack<int> myStack; // Initialize a stack
// Push elements onto the stack
myStack.push(1);
myStack.push(2);
myStack.push(3);
return 0;
}
This simple code initializes an empty stack and pushes three integers onto it.
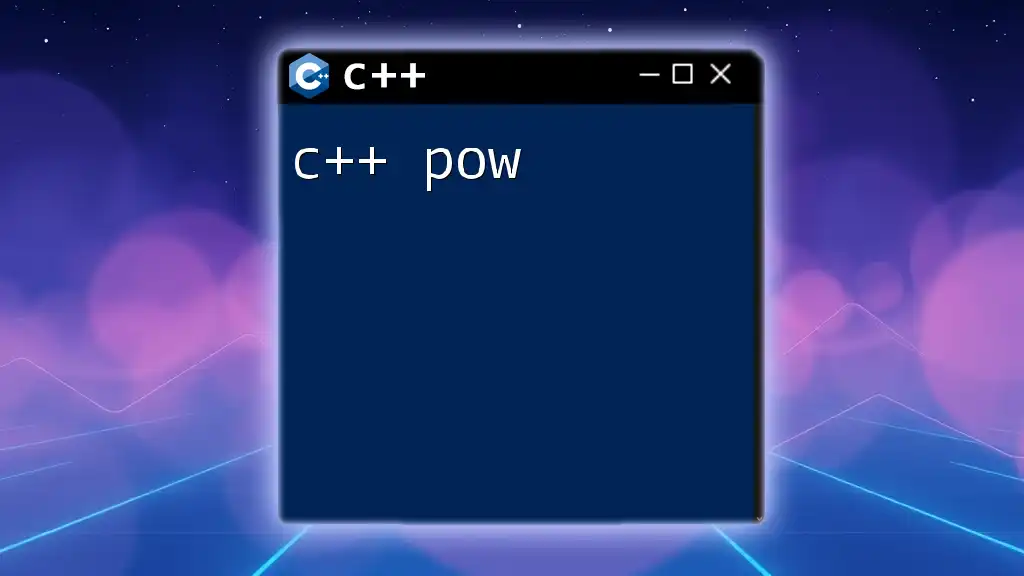
The `pop` Operation
Definition of `pop`
The `pop` operation in C++ is used to remove the top element from a stack. When you use `pop()`, the element that is currently on the top is removed. Importantly, this operation does not return the removed element—it simply discards it. This is why understanding what `pop` does is crucial; you may lose valuable data if not handled correctly.
Syntax of `pop`
The syntax for using `pop` is straightforward:
myStack.pop();
Example of Using `pop` in C++
Here's a basic code example that demonstrates how to use `pop` effectively:
#include <iostream>
#include <stack>
int main() {
std::stack<int> myStack;
// Push elements onto the stack
myStack.push(10);
myStack.push(20);
myStack.push(30);
// Before popping
std::cout << "Top element before pop: " << myStack.top() << std::endl; // Should display 30
// Pop an element
myStack.pop();
// After popping
std::cout << "Top element after pop: " << myStack.top() << std::endl; // Should display 20
return 0;
}
In this example, we first push three integers onto the stack. The `pop` operation removes the top element (30), and when we call `top()` again, we see 20, which is now the new top.
Error Handling with `pop`
Checking Underflow
When working with stacks, it’s important to ensure that you do not attempt to pop from an empty stack, which leads to stack underflow. To avoid this, you can check if the stack is empty before performing a `pop`.
if (!myStack.empty()) {
myStack.pop();
} else {
std::cout << "Cannot pop from an empty stack." << std::endl;
}
Advanced Usage of `pop`
Using `pop` in Algorithms
The `pop` operation is vital in various algorithms, particularly those that rely on depth-first search or expression evaluation.
For example, during depth-first search for a tree or graph, nodes are typically placed on a stack and removed using `pop` to backtrack to previously visited nodes. Similarly, in expression evaluation, `pop` is used to obtain operands from a temporary stack.
Performance Considerations
The time complexity for the `pop` operation is O(1), meaning it runs in constant time. This efficiency makes stacks highly suitable for scenarios that require frequent additions and removals, compared to other data structures like queues or linked lists.
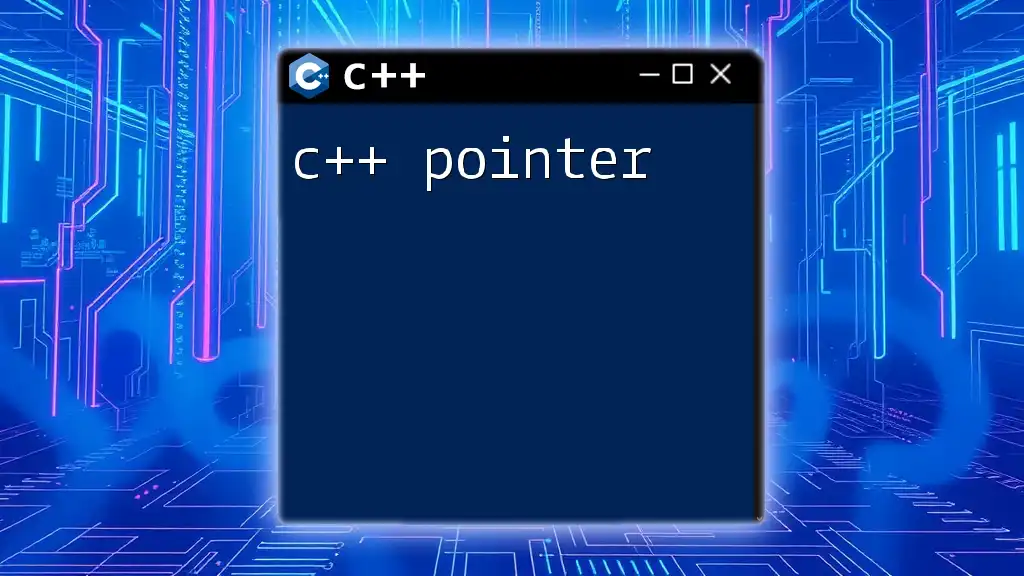
Common Mistakes with C++ `pop`
Not Checking for Empty Stack
One of the most common mistakes is forgetting to check whether the stack is empty before calling `pop`. If you call `pop()` without checking, you may unintentionally cause runtime errors or undefined behavior in your program.
Misunderstanding the Behavior of `pop`
Another common misassumption is thinking `pop` returns the value of the removed element. This misunderstanding can lead to loss of data if the removed value is needed later.
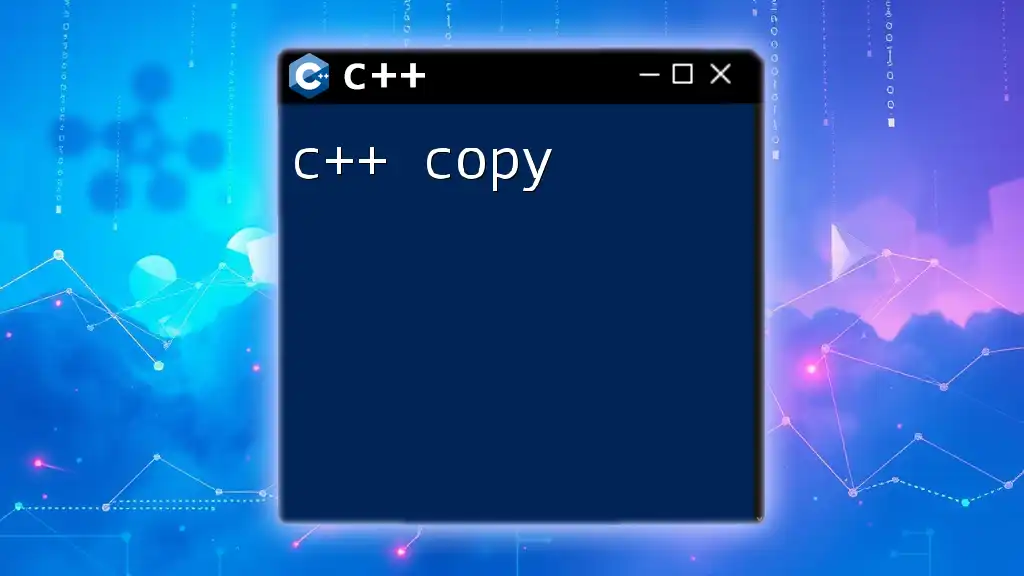
Best Practices for Using `pop`
- Always check if the stack is empty using `empty()` before calling `pop`.
- Be mindful of the data you are potentially losing when popping from a stack.
- Utilize `top()` frequently to peek at the top element without removing it.
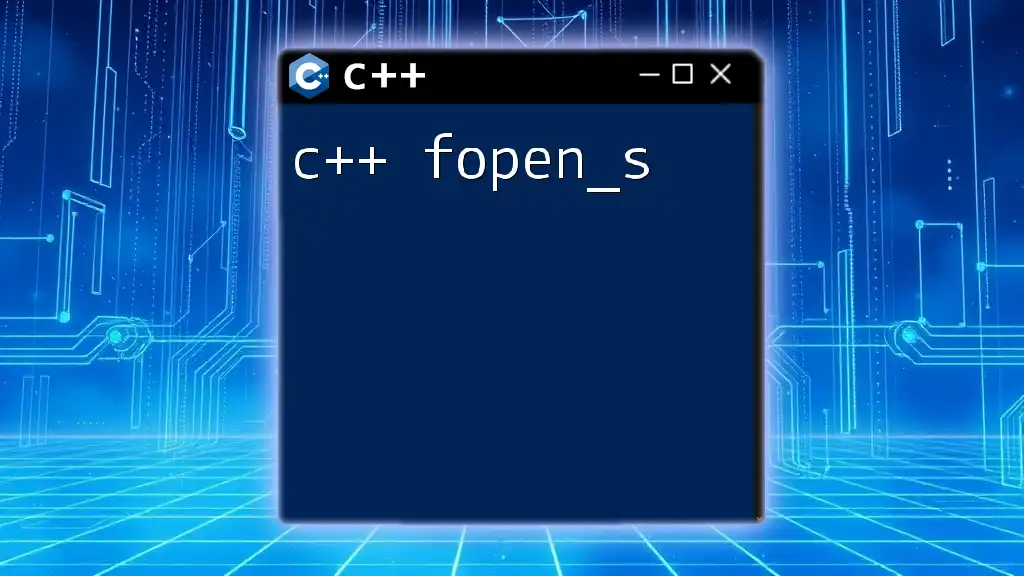
Conclusion
In summary, the c++ pop operation is a fundamental aspect of stack data structure. Understanding its function, along with the importance of checking for empty stacks, is essential for efficient coding practices in C++. With the provided examples and explanations, you are now equipped to effectively utilize the `pop` command in your own C++ projects.
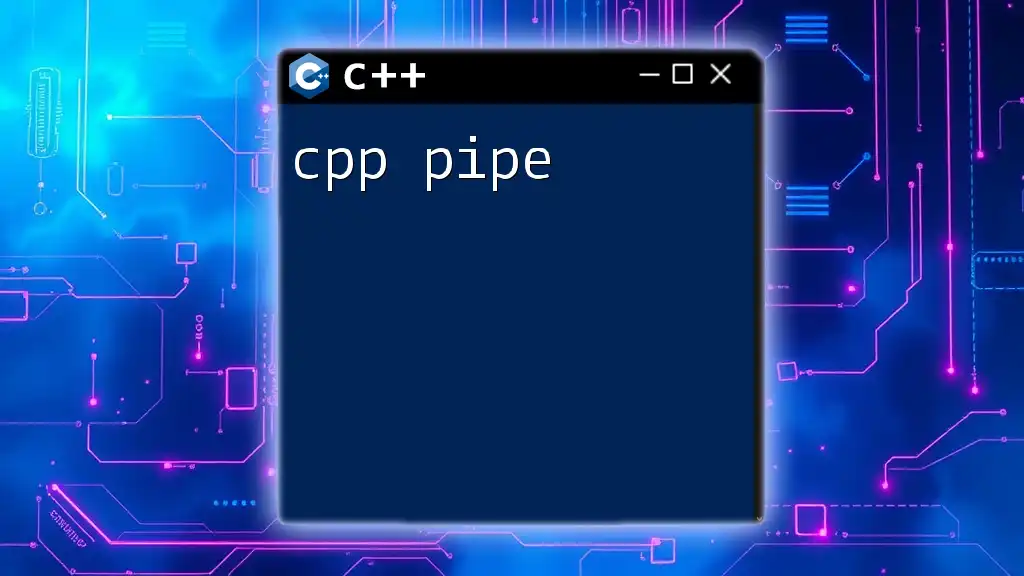
Additional Resources
For further learning, consider reviewing more extensive materials on C++ stacks, data structures, and various algorithms that utilize stack operations. The C++ documentation and community forum discussions can also provide invaluable insights and examples.