In C++, the `.h` file is used for declaring classes, functions, and variables (header files), while the `.cpp` file contains the implementation of those declarations (source files).
// Example of a header file: MyClass.h
#ifndef MYCLASS_H
#define MYCLASS_H
class MyClass {
public:
void display();
};
#endif // MYCLASS_H
// Example of a source file: MyClass.cpp
#include "MyClass.h"
#include <iostream>
void MyClass::display() {
std::cout << "Hello, World!" << std::endl;
}
What Are .h Files?
Definition of .h Files
Header files, commonly recognized by their .h extension, serve as the structural foundation for many C++ programs. They consist primarily of declarations and are crucial for informing the compiler about the types of functions, classes, constants, and variables that are used in other files. By utilizing header files, you can outline what will be included in the corresponding source files without repeating code.
Common Uses of .h Files
-
Function Prototypes: Header files frequently declare functions that will be defined in a .cpp file. This declaration allows the compiler to understand the return type and any parameters the function may take.
-
Class Definitions: Classes defined in header files can be instantiated in any .cpp file that includes that header. This promotes reusability and modular design.
-
Constants and Macros: Storing constant values or macro definitions in header files enables easy adjustments and improves readability throughout the code.
Example of a .h File
Here’s a simple illustration of a header file:
// example.h
#ifndef EXAMPLE_H
#define EXAMPLE_H
class Example {
public:
void displayMessage();
};
#endif // EXAMPLE_H
In this example, `#ifndef`, `#define`, and `#endif` are preprocessor directives that ensure the header file is included only once, thus preventing multiple declaration errors.
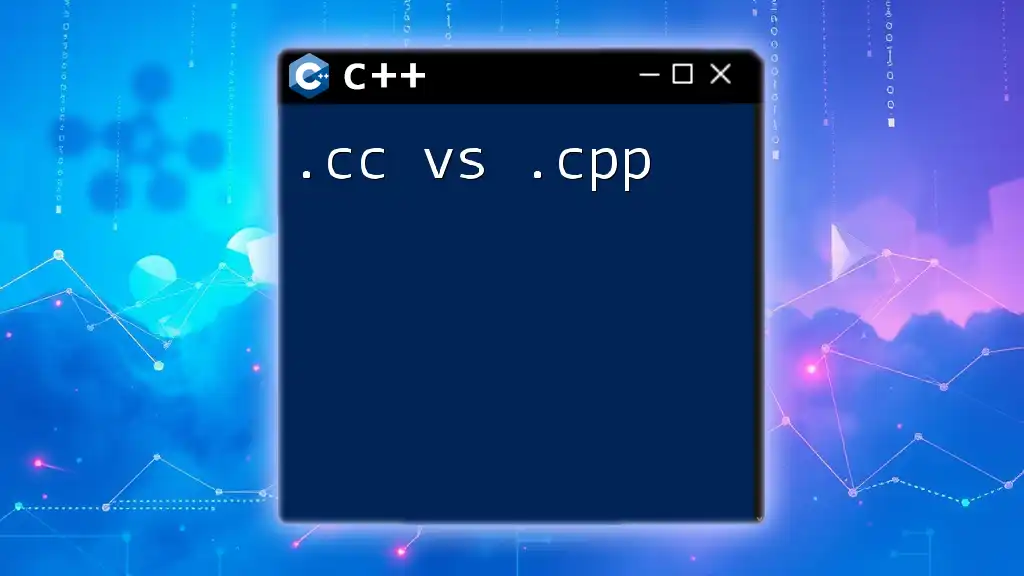
What Are .cpp Files?
Definition of .cpp Files
Source files in C++, denoted by the .cpp extension, are where the actual implementation of functions and methods occurs. These files compile into object code that forms the executable portion of your application.
Common Uses of .cpp Files
-
Function Implementations: In the .cpp files, you provide the concrete definition for the functions that were declared in the header files. This is where the logic and behavior of your program come to life.
-
Class Method Definitions: Methods corresponding to classes defined in header files are implemented in .cpp files, ensuring a clean separation of interface and implementation.
-
Running Code: Generally, .cpp files contain the `main()` function, which is the entry point for any C++ program, allowing for execution of the program.
Example of a .cpp File
Here's a simple example illustrating a .cpp file:
// example.cpp
#include "example.h"
#include <iostream>
void Example::displayMessage() {
std::cout << "Hello, World!" << std::endl;
}
In this case, `#include "example.h"` includes the declarations from the header file, allowing the .cpp file to implement the `displayMessage()` function.
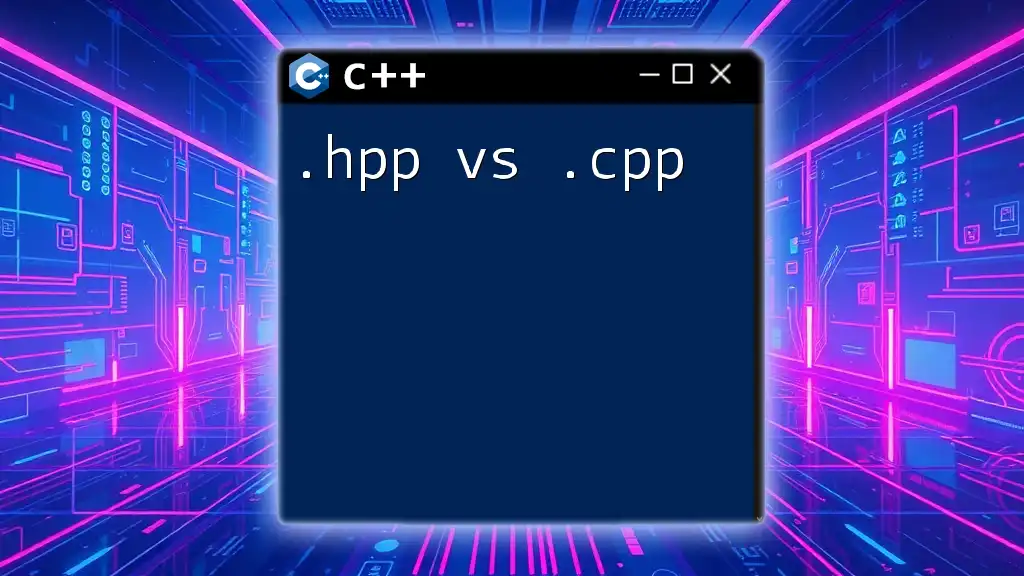
Key Differences between .h and .cpp Files
Purpose and Functionality
The primary distinction is that .h files are primarily for declarations, whereas .cpp files are for definitions. This separation aids in both organization and readability of code, making it easier to navigate larger projects.
Compilation and Linking
When compiling, the compiler treats .h files as interfaces, giving it information about how to link the various pieces together without knowing the full details. On the other hand, .cpp files are where the actual mechanics—how these interfaces work together—are encoded.
Code Organization and Reusability
By separating declarations and definitions, you can organize your code more effectively. This modular structure allows for multiple .cpp files to include the same .h file without redundancies, encouraging reusability across your codebase.
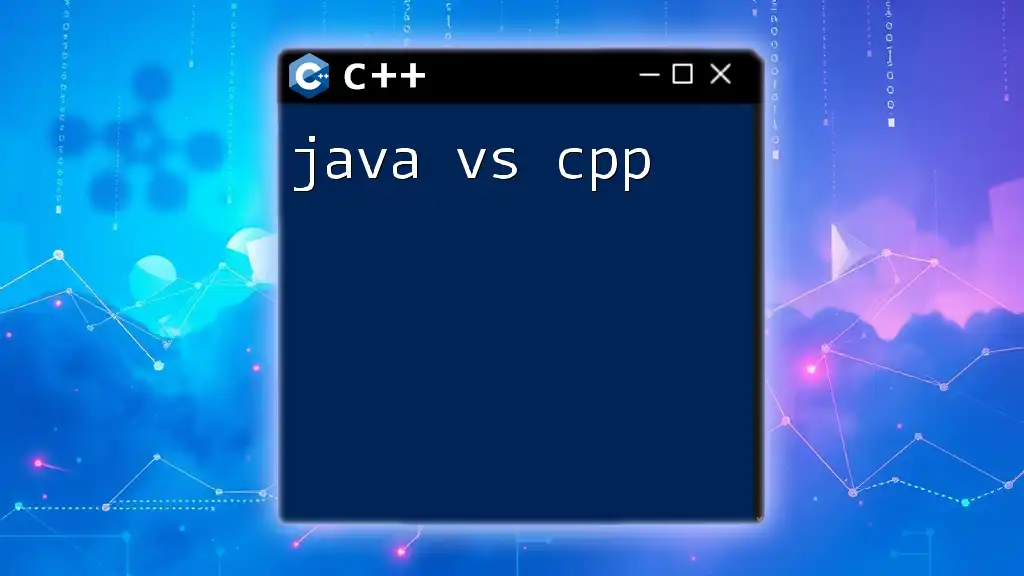
Best Practices for Using .h and .cpp Files
Organizing Your Codebase
It’s advisable to structure your projects such that each module consists of a corresponding .h and .cpp file. A well-organized directory structure not only eases navigation but also facilitates teamwork in larger projects.
Keeping .h Files Clean
Avoid overly cluttered header files. They should contain only declarations, while the implementation details reside in the .cpp files. This promotes cleaner code and more efficient compilation.
When to Use Inline Functions
Inline functions can be defined within header files for performance optimization. For example:
// inline_example.h
#ifndef INLINE_EXAMPLE_H
#define INLINE_EXAMPLE_H
inline int square(int x) {
return x * x;
}
#endif // INLINE_EXAMPLE_H
Using `inline` helps avoid the overhead of function calls, but use this judiciously, as excessive inlining can increase binary size.
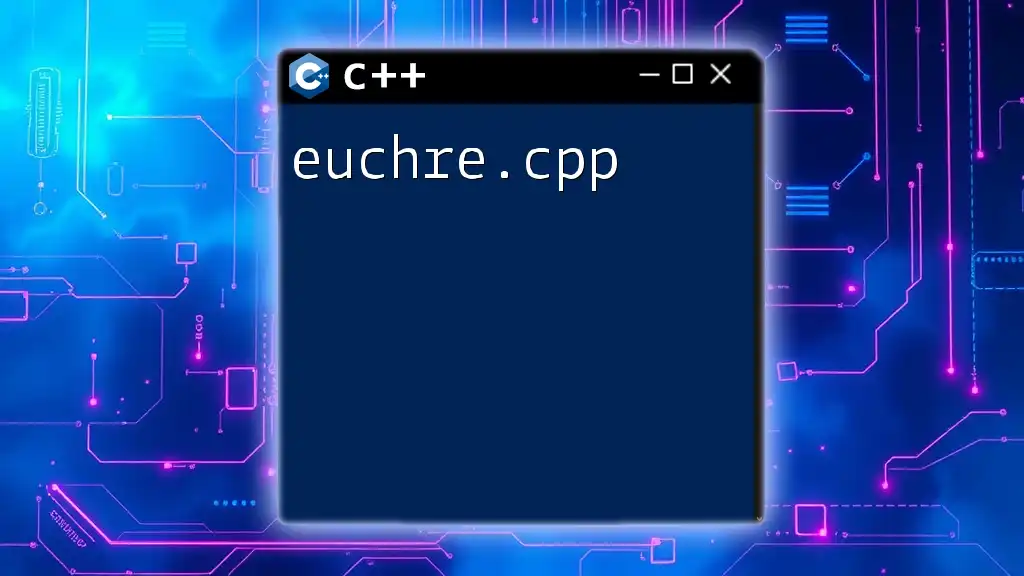
cpp vs h: Performance Considerations
Compilation Time
Having large header files, especially those that include extensive libraries, can slow down compilation time remarkably. To optimize this, consider using forward declarations where possible to mitigate dependencies among files.
Reducing Dependencies
By minimizing dependencies in header files, you can significantly improve the compilation process. This ultimately leads to quicker builds and a more efficient development experience.
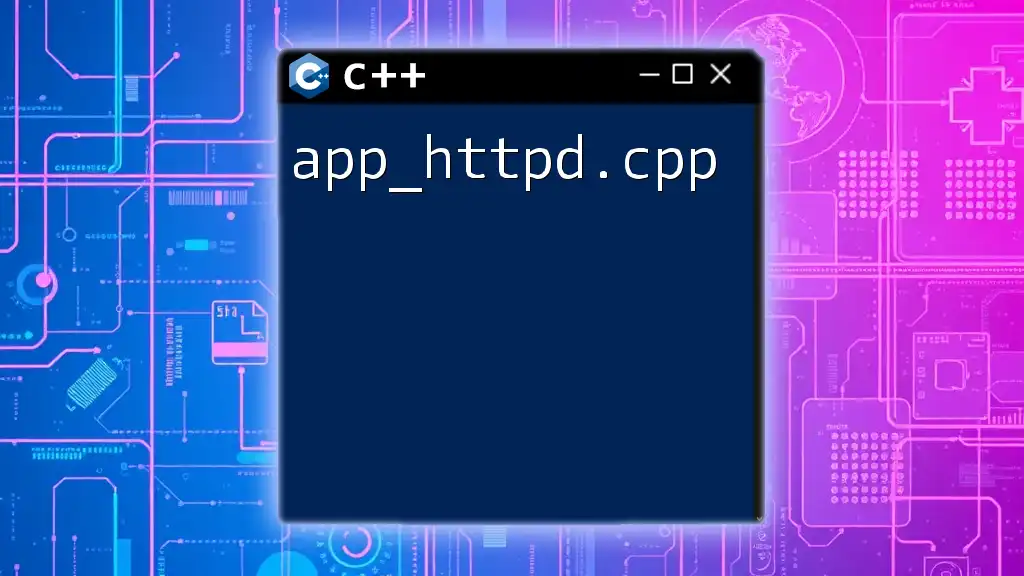
Common Mistakes to Avoid
Misusing Header Guards
One frequent error is neglecting to properly implement header guards, which can lead to multiple definition errors. Every header file should include the preprocessor guards like `#ifndef`, `#define`, and `#endif` to ensure it is only included once during compilation.
Forgetting to Include Headers
Omitting necessary header files can lead to compilation errors, hampering development. Always ensure that your .cpp files include the corresponding header files where required types and declarations are defined.
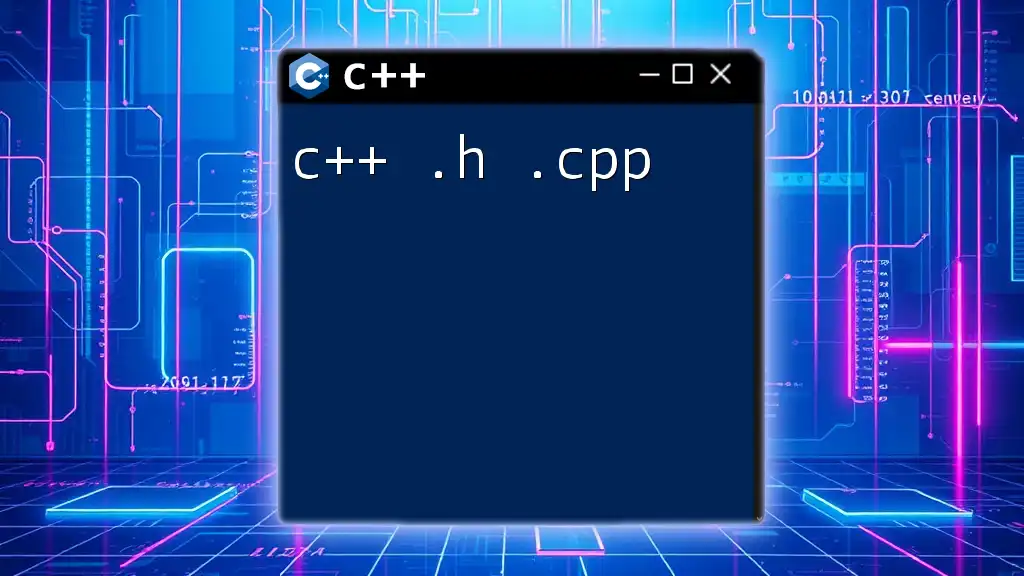
Conclusion
Understanding the distinctions between .h and .cpp files is essential for effective C++ programming. By structuring your projects with a clear separation of declarations and definitions, you not only improve the organization but also enhance code maintainability and performance. By following the best practices outlined here, programmers can ensure their code is clean, efficient, and easy to navigate. With a solid grasp of these concepts, you’re well on your way to mastering C++ development.