"Undefined symbols for architecture arm64" typically indicates that the linker could not find the definition of a function or variable declared in your C++ code, often occurring when the compiled object files are missing or not linked properly.
Here’s a simple example that might lead to such an error:
// my_functions.h
void myFunction();
// my_main.cpp
#include "my_functions.h"
int main() {
myFunction(); // This will cause an "undefined symbol" error if myFunction is not defined elsewhere.
return 0;
}
Ensure that you define `myFunction` in a corresponding source file, like so:
// my_functions.cpp
#include "my_functions.h"
#include <iostream>
void myFunction() {
std::cout << "Function executed!" << std::endl;
}
Understanding Undefined Symbols
Definition of Undefined Symbols
In C++, undefined symbols arise when the compiler encounters a declaration for a function or an object that lacks a corresponding definition during the linking phase. When this happens, the linker fails to resolve the reference, which leads to compilation errors. Understanding this concept is crucial, as undefined symbols are among the most common errors encountered by C++ developers, especially when compiling for specific architectures like arm64.
Common Causes of Undefined Symbols
Missing Implementations
One of the most prevalent causes of undefined symbols is the existence of function declarations without their implementations. For instance:
// Example header file
void myFunction(); // Declaration
If `myFunction()` is not defined anywhere in the codebase, invoking this function results in an undefined symbol error during linking. To avoid such errors, always ensure that all declared functions have valid implementations.
Incorrect Linker Flags
Linker flags play an essential role in the build process. If the appropriate flags are not set for a given architecture, such as arm64, it can lead to undefined symbols. For example, when using `g++` to compile, the right flags should include:
g++ -o myProgram mySource.cpp -L/path/to/libs -lmylib
Here `-L/path/to/libs` specifies the path to libraries, and `-lmylib` tells the linker to include `mylib`. Ensure that all necessary libraries are properly linked, especially when working in an arm64 environment.
Namespace and Scope Issues
C++ features namespaces to organize code and avoid name collisions. However, improper use of namespaces can result in undefined symbols. For instance, if a function is declared in a namespace but called outside it without qualification, you will encounter a linking error.
Consider the following example:
// Example.cpp
namespace MyNamespace {
void myFunction(); // Declaration
}
// Attempting to call without namespace qualification
myFunction(); // This will result in an undefined symbol error
To successfully link, you should call the function as follows:
MyNamespace::myFunction(); // Correct way to call the function
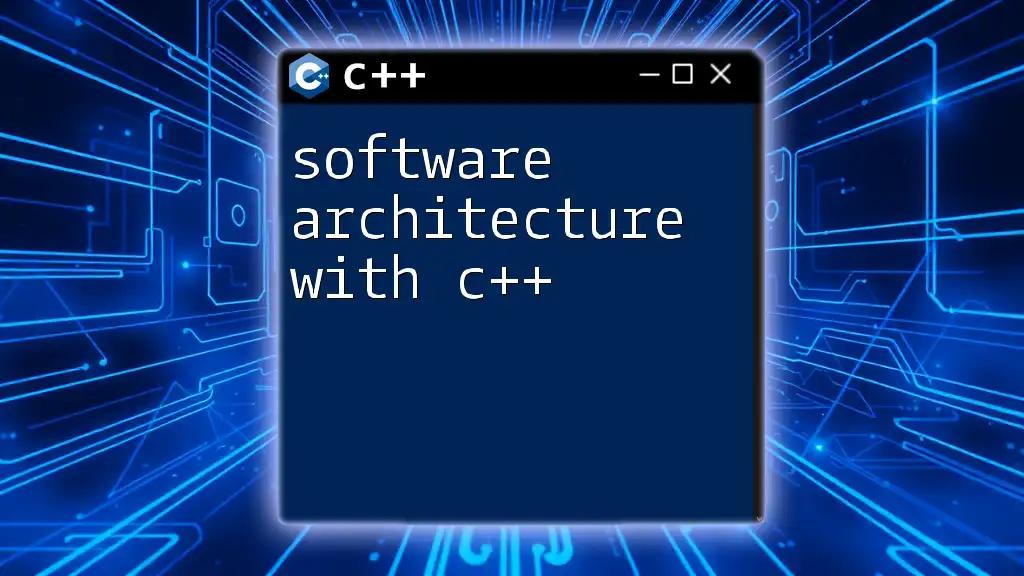
Specific Issues Related to arm64
Architecture-Specific Linking Challenges
Each architecture can have unique linking challenges. The arm64 architecture, known for its efficiency and performance in mobile devices, often requires special attention during the linking process. For example, certain libraries might need architecture-specific versions, and failure to specify the correct architecture in your build tools can lead to undefined symbols.
Toolchain and Environment Setup
Setting up your development environment correctly is vital in mitigating undefined symbols errors. Here’s how you can configure your toolchain:
- Choose an IDE that supports arm64, such as Xcode or Visual Studio.
- Install the appropriate toolchain (like Clang for arm64).
- Set your targets correctly in your project settings.
For example, in Xcode, you may need to set your project's architecture in the build settings under "Architectures" to ensure your application builds for arm64.
Example Scenarios of Undefined Symbols on arm64
Scenario 1: Misconfigured xcodeproj
A typical mistake when using Xcode is failing to configure your project file properly. For instance, forgetting to add source files can lead to undefined symbols:
- Create a new Xcode project.
- Add your source files by dragging them into the project navigator (or using "Add Files to...").
To diagnose, check your Build Phases tab and ensure the necessary files are included under Compile Sources. Misconfiguration here may result in linking issues.
Scenario 2: Multiple Compiling Units
When working with multiple source files, ensure that each file is correctly compiled and linked. Consider the following two files:
File1.cpp:
#include "File2.h"
void testFunction() {
myFunction(); // Assuming myFunction is declared in File2.h
}
File2.h:
#ifndef FILE2_H
#define FILE2_H
void myFunction(); // Declaration
#endif
File2.cpp:
#include "File2.h"
void myFunction() {
// Implementation here
}
If you forget to include `File2.cpp` during the build process, you’ll encounter an undefined symbol error for `myFunction` when compiling `File1.cpp`. Ensure that your build system compiles all necessary units.

Debugging Undefined Symbols
Compilation vs. Linker Errors
Understanding the difference between compilation errors and linker errors is crucial. Compilation errors occur when the code is syntactically incorrect, while linker errors arise from unresolved symbols.
When you see an error message like this during the linking phase:
undefined symbol: myFunction
it indicates that the linker could not find the definition of `myFunction`. Identifying whether the issue occurred during compilation or linking will streamline your troubleshooting process.
Tools for Diagnosing Undefined Symbols
Using Compiler Flags for Diagnostics
Using specific compiler flags can significantly help in pinpointing issues with undefined symbols. The following flags are particularly useful:
- `-Wall`: Issues warnings about potential problems.
- `-Werror`: Treats warnings as errors, ensuring you address all outstanding issues.
- `-fdiagnostics-show-options`: Displays additional details about diagnostics.
You can use these flags in your build command:
g++ -Wall -Werror -fdiagnostics-show-options mySource.cpp -o myProgram
This will provide detailed information, helping you identify undefined symbols and their potential causes.
Static Analysis Tools
Static analysis tools such as cppcheck and clang-tidy can also assist in identifying issues in your code. They analyze your code for potential problems without compiling it.
For instance, running `cppcheck` on your codebase might reveal missing definitions for your declared functions, allowing you to address these problems proactively before reaching the linking stage.

Preventive Measures
Best Practices in C++ Development
To minimize the chances of encountering undefined symbols, implementing best practices is crucial:
- Organize your code into logical modules, ensuring that declarations and definitions are appropriately paired.
- Maintain a consistent naming convention and adhere to it to simplify tracking down symbol definitions.
A well-structured project might look like this:
/MyProject
/include
File2.h
/src
File1.cpp
File2.cpp
Building and Linkage Tips
Finally, remember these tips for compiling effectively on arm64:
- Always specify architecture flags in your build system (e.g., `-arch arm64`).
- Use version control tools to manage changes and roll back if misconfigurations occur.
- Test linking frequently during development to catch undefined symbols early in the process.
By adhering to these measures, you can drastically reduce the frequency of encountering undefined symbols for architecture arm64 C++.

Conclusion
Undefined symbols can be a significant roadblock in C++ development, particularly for those working on arm64 architecture. By grasping the concept of undefined symbols, recognizing their common causes, and following best practices, you can ensure a smoother development experience. Engage with your code, experiment with different configurations, and utilize the tools available to troubleshoot effectively. Stay curious and persistent, and remember that understanding the inner workings of your development environment is key to overcoming such challenges.

Additional Resources
For further exploration, consider reading:
- "Effective C++" by Scott Meyers for best practices.
- Compiler documentation for the toolchain you're using, such as GCC or Clang.
Engaging with communities like Stack Overflow or GitHub to discuss and troubleshoot arm64-specific issues can also offer invaluable insights from experienced developers.