The `next` function in C++ is often used to advance an iterator to the next position in a container, allowing for efficient traversal of elements.
#include <iostream>
#include <vector>
#include <iterator>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
auto it = numbers.begin();
// Move to the next element
it = std::next(it);
std::cout << "Next element: " << *it << std::endl; // Output: Next element: 2
return 0;
}
What is C++ Next?
"C++ Next" refers to the latest advancements and features introduced in recent C++ standards, building upon the foundation laid by previous versions of the language like C++11, C++14, C++17, and C++20. As the programming landscape evolves, it's essential to stay updated with these enhancements to leverage more efficient coding practices, improved performance, and enhanced safety features. Embracing C++ Next means adopting a mindset geared towards understanding and utilizing these new capabilities to write modern C++ code.
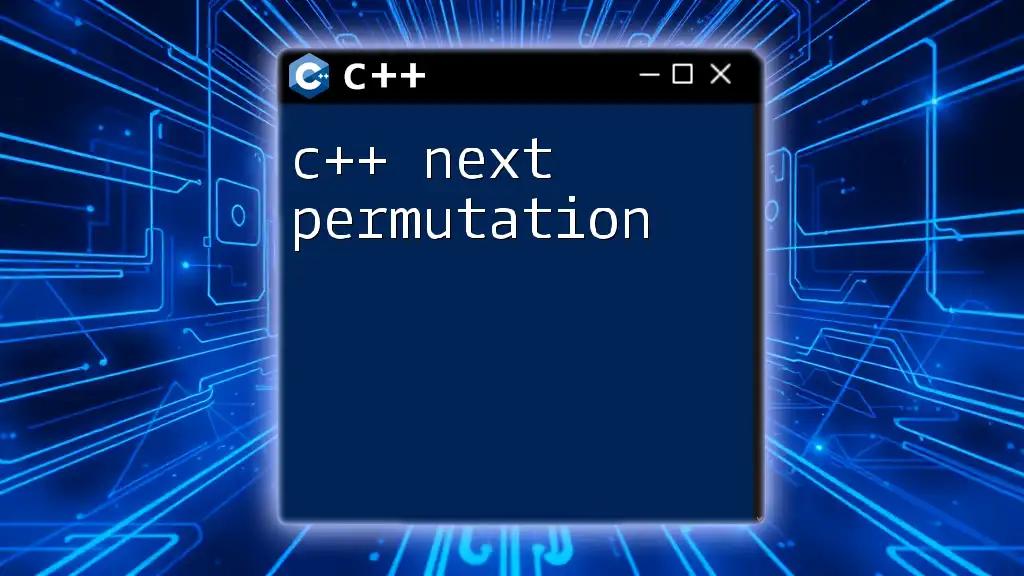
Key Features of C++ Next
Ranges
Ranges are a powerful feature introduced to enhance the way we handle sequences of values in C++. They simplify the process of gathering, filtering, and transforming collections without the need for complex iterator manipulations.
Here’s a simple example using ranges:
#include <vector>
#include <iostream>
#include <ranges>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
auto even_numbers = numbers | std::views::filter([](int n) { return n % 2 == 0; });
for (int n : even_numbers) {
std::cout << n << " ";
}
return 0;
}
In the example above, we utilize the `std::views::filter` function to create a view that only holds even numbers from the original vector. This declarative style improves readability and eliminates the risks associated with manual iterator management. The key takeaway is that ranges streamline data processing, allowing developers to write cleaner and more maintainable code.
Concepts
Concepts introduce a way to refine generic programming through type constraints. This feature helps enforce better type safety within template functions, providing clearer error messages during compilation.
Consider the following example:
template<typename T>
concept Incrementable = requires(T x) { ++x; };
template<Incrementable T>
void increment(T& value) {
++value;
}
Here, the `Incrementable` concept checks if the type `T` can be incremented. This approach allows us to define a function, `increment`, that will only work with types that conform to the `Incrementable` concept. The major advantage lies in its ability to provide more meaningful error messages when a user attempts to use the function with an incompatible type, thus enhancing the overall development experience.
Coroutines
Coroutines represent a new paradigm for asynchronous programming in C++. They allow functions to be paused and resumed, making it easier to write code that performs delayed operations without blocking execution.
Let’s take a look at a basic coroutine example:
#include <iostream>
#include <coroutine>
struct awaitable {
bool await_ready() const noexcept { return false; }
void await_suspend(std::coroutine_handle<>) const noexcept {}
int await_resume() const noexcept { return 42; }
};
auto simpleCoroutine() -> std::coroutine_handle<> {
co_return;
}
In this example, we define an `awaitable` type that fulfills the requirements for a coroutine. Coroutines can significantly simplify complex tasks involving asynchronous I/O operations, allowing developers to write code that resembles a synchronous flow. By using coroutines, you improve not only readability but also maintainability, facilitating cleaner error handling and more manageable code structures.
Modules
Modules are another significant enhancement introduced to aid in the organization and encapsulation of code. Traditional header files are prone to multiple inclusion problems and longer compilation times. Modules alleviate these issues by offering a more robust way to manage dependencies.
Here’s a simple demonstration:
module math_operations;
export int add(int a, int b) { return a + b; }
import math_operations;
int main() {
int sum = add(5, 3);
std::cout << "Sum: " << sum;
return 0;
}
In this example, we define a module named `math_operations` that includes an `add` function. The key benefit here is that modules allow us to hide implementation details and expose only what is necessary. This minimizes the likelihood of name collisions and improves compilation speed by limiting the scope of header files.

Practical Applications of C++ Next Features
Real-World Scenarios
-
Using Ranges in Data Processing: Ranges can be incredibly beneficial when dealing with large datasets, such as filtering user inputs or processing sensor data in real time. Their ability to work with views allows for efficient data manipulation without additional memory allocations.
-
Implementing Coroutines for Async I/O: In server applications, coroutines enable non-blocking operations, allowing servers to handle multiple connections simultaneously. For instance, when a server processes requests for data, it can yield control to processes waiting on I/O operations and then return to finishing the task once the data becomes available.
-
Structural Organization with Modules: Codebases can become unwieldy without proper modularization. By using modules, projects with multiple dependencies and complexities can maintain clearer relationships between components, leading to improved collaboration among developers on larger teams.
Best Practices
- When utilizing ranges, ensure that you consider the readability of your code, as overly complex filter operations can negate the simplicity that ranges provide.
- To get the most out of concepts, define clear and concise constraints to keep your templates manageable and error messages informative.
- With coroutines, be mindful of their usage to prevent overly complicated flow and ensure that your coroutines are designed for easy maintenance and testing.
- Adopting modules gradually can help in organizing legacy codebases. Start by modularizing distinct aspects of your application rather than attempting to refactor everything at once.
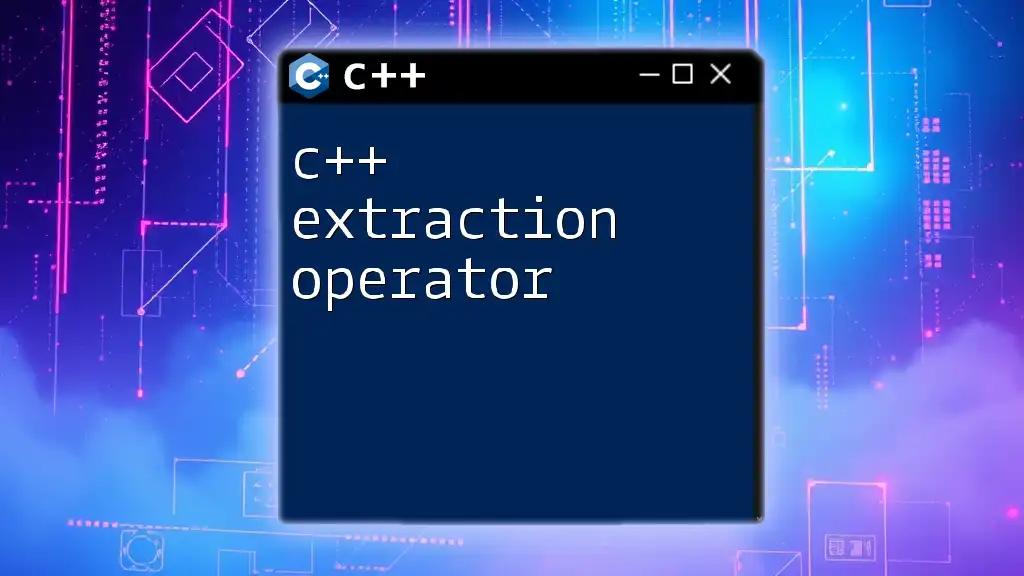
Future Directions in C++ Development
The landscape of C++ is continually evolving, with active proposals and discussions surrounding potential features for future standards. Engaging with the C++ community through forums, reading proposals, and contributing to discussions on platforms like GitHub is a proactive way for developers to influence and stay informed about the direction of C++.

Conclusion
C++ Next is an exciting frontier for developers aiming to create robust, efficient, and maintainable software. By understanding and applying features such as ranges, concepts, coroutines, and modules, you can enhance your coding practices and stay ahead in the rapidly changing software development landscape. Embrace the power of modern C++, and experiment with these features to see the improvements they bring to your coding workflow.

Additional Resources
To deepen your knowledge, explore the official C++ documentation, recommended books, and reputable online courses tailored for learning the latest C++ features. Engaging with community channels can also foster discussions and provide valuable practice opportunities as you advance in your C++ development journey.