In the latest updates in C++, developers can now leverage features like the new `std::format` library for simpler string formatting, enhancing code readability and efficiency. Here's a quick example:
#include <format>
#include <iostream>
int main() {
std::string name = "world";
std::string greeting = std::format("Hello, {}!", name);
std::cout << greeting << std::endl;
return 0;
}
Recent Updates in C++
New Standards and Features
C++23 Overview
C++23 anticipates several exciting features and improvements that aim to enhance the language’s functionality and usability. Among the most notable additions are:
-
Concepts: These allow developers to specify constraints on template parameters, leading to clearer and safer code.
-
Ranges: Ranges enable more expressive manipulation and interaction with collections. They streamline code that deals with algorithms and iterators, making it more intuitive.
-
Updates to the Standard Library: C++23 is set to introduce many new library features, such as enhanced support for format and year/month/time manipulation, contributing to improved date and time handling.
Example of Concepts
Concepts make template programming more robust and enable better error diagnostics. Here’s a simple implementation demonstrating concepts in action:
template<typename T>
concept Addable = requires(T a, T b) {
{ a + b } -> std::same_as<T>;
};
template<Addable T>
T add(T a, T b) {
return a + b;
}
In the example above, the `Addable` concept ensures that any type passed to the `add` function can be added together.
Compiler Changes
Latest Compiler Releases
Staying updated with the latest compiler advancements is crucial for maximizing the potential of C++. Major compilers like GCC, Clang, and MSVC have each announced significant upgrades, which often focus on:
-
Performance Improvements: These enhancements aim to make compiled programs run faster and more efficiently.
-
Adoption of New Features: Compilers are continually adapting to accommodate new language features with each iteration of the C++ standard.
Code Snippet Demonstration
Compiler optimizations can significantly impact the performance of your code. Consider the following example, where a new optimization feature is utilized:
#pragma optimize on
void fastComputation() {
int total = 0;
for (int i = 0; i < 10000; ++i) {
total += i;
}
}
Here, the `#pragma optimize on` directive signals the compiler to optimize the following function for enhanced performance.
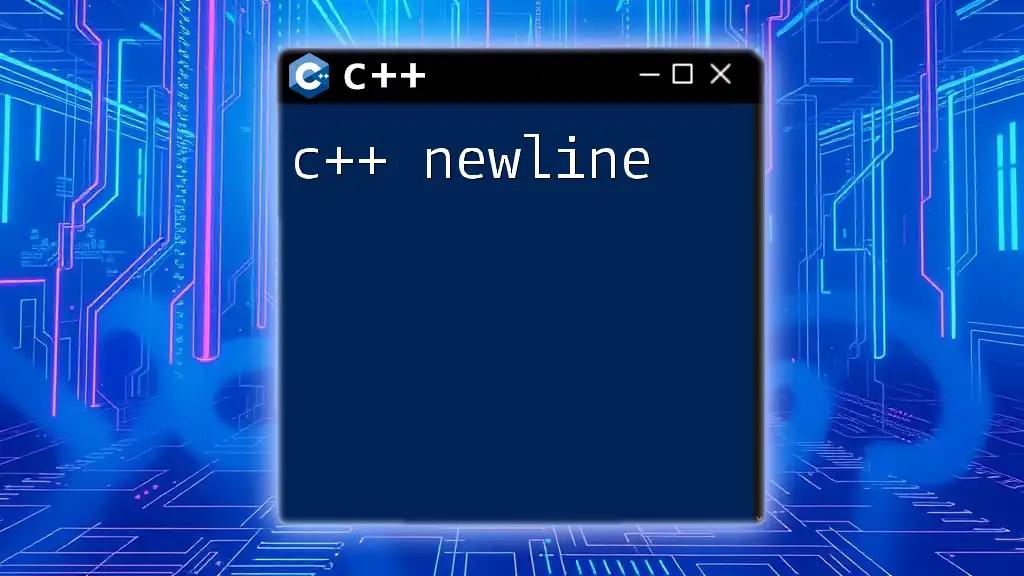
Trends in C++ Development
C++ in Emerging Technologies
C++ and AI
The rise of artificial intelligence and machine learning has opened new avenues for C++. Its performance and efficiency make it an ideal choice for developers working with high-demand applications. Frameworks such as TensorFlow and PyTorch, which have backends written in C++, exemplify its growing importance in the AI field.
Code Example: Using C++ with AI Libraries
Implementing a simple AI operation using a C++ library can look like this:
#include <tensorflow/core/public/session.h>
#include <tensorflow/core/platform/env.h>
// Example of initializing a TensorFlow session
tensorflow::Session* session;
tensorflow::NewSession(tensorflow::SessionOptions(), &session);
This snippet shows how C++ can seamlessly integrate with AI libraries to leverage its speed and efficiency.
C++ for Game Development
The Increasing Popularity of C++ in Gaming
C++ remains the backbone of game development, primarily due to its performance optimization capabilities and low-level memory manipulation. High-performance game engines, like Unreal Engine and Unity, heavily rely on C++ for developing demanding applications.
Code Snippet: Game Loop Implementation
A fundamental aspect of game programming is the game loop, which handles the game state and rendering. Here’s an example of a basic game loop in C++:
bool gameRunning = true;
while (gameRunning) {
processInput();
updateGameLogic();
renderGraphics();
}
This simple loop structure reflects how C++ enables real-time interactions, fundamental in gaming environments.
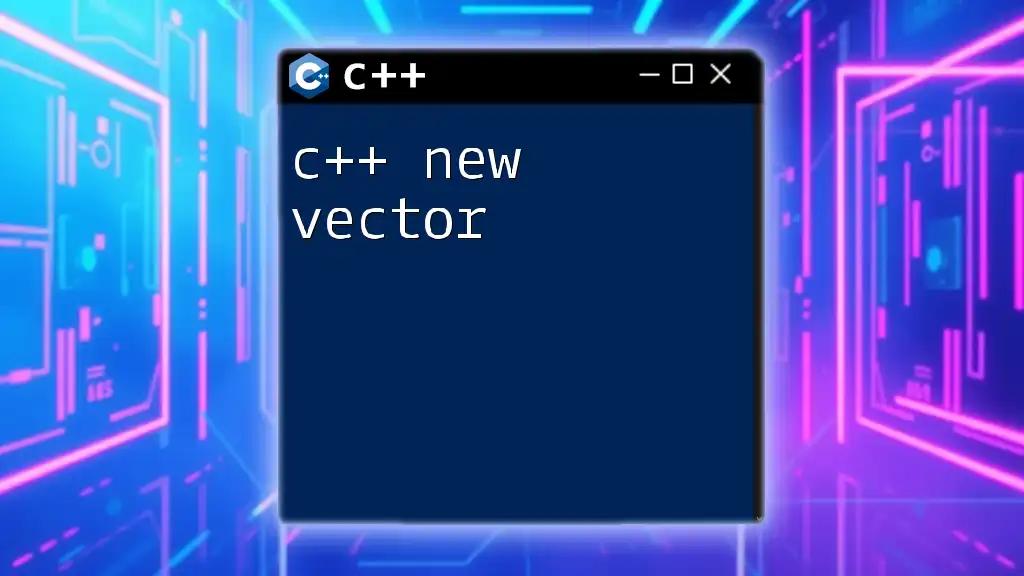
C++ Community News
Contributions and Events
Open Source Projects
The C++ community is vibrant, with numerous open-source projects driving innovation and growth. Many developers contribute to libraries and frameworks, resulting in continual improvement and expanded functionality for the language.
Upcoming Conferences and Events
Keeping up with C++ trends also involves participating in community events. Events like CPPCon and C++ Now provide excellent opportunities for learning and networking, where developers share their experiences and present new ideas.
Interviews with Experts
Spotlight on C++ Innovators
Gaining insights from leading figures in the C++ community can provide valuable perspective on where the language is headed. Interviews often reveal trends that are shaping the future of C++, insights into best practices, and advice on overcoming common challenges.

Future Outlook
What Lies Ahead for C++?
Expected Developments
As technology evolves, so will the demands placed on programming languages like C++. Future iterations of C++ are expected to prioritize simplicity, safety, and developer experience, continually adapting to the needs of developers.
How to Keep Learning and Adapting
For developers wishing to remain competitive and informed in the field of C++, numerous resources are available:
- Join C++ forums and discussion groups to share knowledge and ask questions.
- Follow prominent C++ blogs and YouTube channels for tutorials and news.
- Engage with online courses and workshops designed to keep skills sharp.

Conclusion
Staying informed about the latest C++ news is essential for developers wishing to remain relevant and effective in their craft. By understanding recent updates and trends, developers can leverage the advancements in C++ to create robust, high-performance applications. Engaging with the C++ community, contributing to projects, and participating in events will ensure continuous growth and mastery of the language.