C++ CUDA is an extension of C++ that enables developers to leverage the parallel processing power of NVIDIA GPUs for executing complex computations efficiently.
Here’s a simple code snippet demonstrating how to use CUDA to add two vectors:
#include <iostream>
#include <cuda_runtime.h>
__global__ void addVectors(const float *a, const float *b, float *c, int N) {
int index = threadIdx.x + blockIdx.x * blockDim.x;
if (index < N) {
c[index] = a[index] + b[index];
}
}
int main() {
const int N = 256;
float a[N], b[N], c[N];
// Initialize vectors a and b
for (int i = 0; i < N; i++) {
a[i] = i;
b[i] = i * 2.0;
}
float *dev_a, *dev_b, *dev_c;
cudaMalloc((void**)&dev_a, N * sizeof(float));
cudaMalloc((void**)&dev_b, N * sizeof(float));
cudaMalloc((void**)&dev_c, N * sizeof(float));
cudaMemcpy(dev_a, a, N * sizeof(float), cudaMemcpyHostToDevice);
cudaMemcpy(dev_b, b, N * sizeof(float), cudaMemcpyHostToDevice);
addVectors<<<N/256, 256>>>(dev_a, dev_b, dev_c, N);
cudaMemcpy(c, dev_c, N * sizeof(float), cudaMemcpyDeviceToHost);
// Cleanup
cudaFree(dev_a);
cudaFree(dev_b);
cudaFree(dev_c);
// Output result
for (int i = 0; i < N; i++) {
std::cout << c[i] << " ";
}
std::cout << std::endl;
return 0;
}
What is C++?
C++ is a powerful, high-performance programming language that has become foundational in systems programming, game development, and applications that demand rigorous computational efficiency. It extends the C programming language by adding features like classes, inheritance, and templates, allowing for both high-level abstraction and low-level memory manipulation. C++ is revered for its versatility, enabling developers to create software that ranges from simple console applications to complex systems like operating systems and embedded systems.
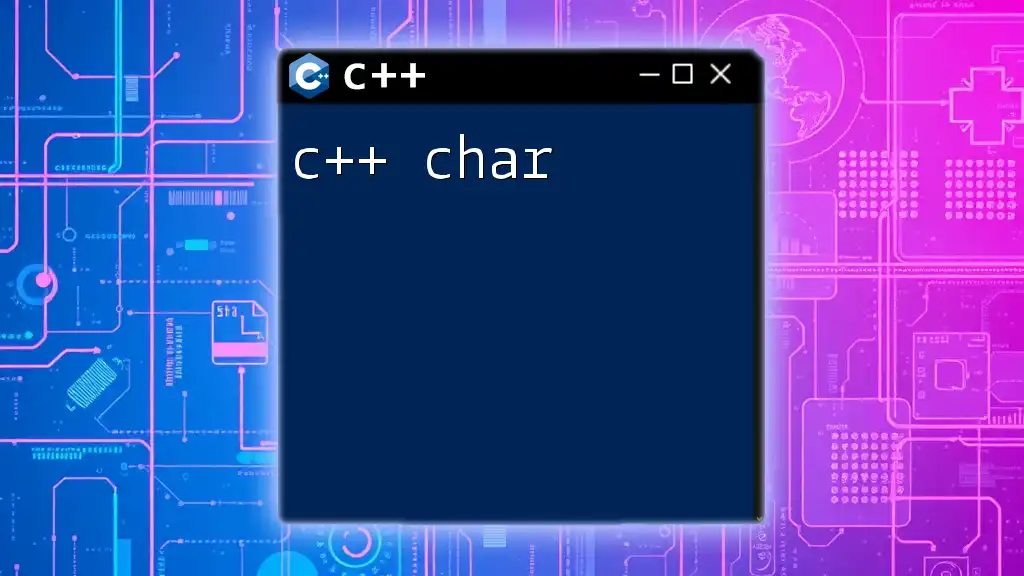
What is CUDA?
CUDA, which stands for Compute Unified Device Architecture, is a parallel computing platform and application programming interface (API) model created by NVIDIA. It allows developers to leverage the power of NVIDIA GPUs (Graphics Processing Units) for general-purpose computing tasks, moving beyond graphics rendering. The major advantage of CUDA is its ability to perform many calculations simultaneously, thereby accelerating compute-intensive applications such as scientific computing, deep learning, and image processing.
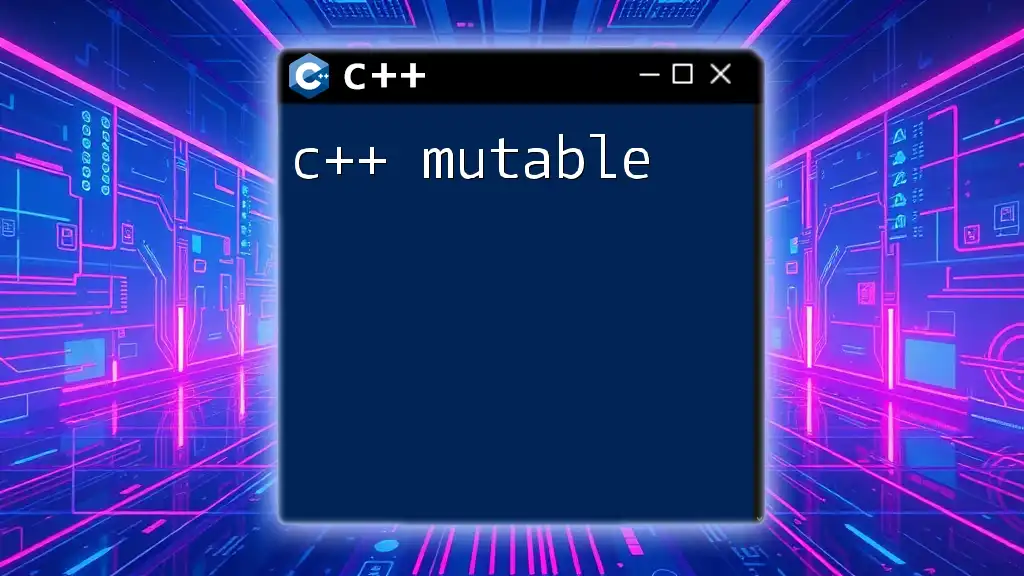
The Relationship between C++ and CUDA
The integration of CUDA with C++ brings significant benefits to developers. CUDA extends C++ with additional keywords and functions that enable programmers to write parallel code more effectively. By using CUDA in C++, developers can accelerate the execution of computational tasks, gaining performance benefits that can be orders of magnitude greater than that achievable on a CPU alone. This combination empowers programmers to harness GPU capabilities in a familiar language syntax, which facilitates quicker learning curves and more robust code development.
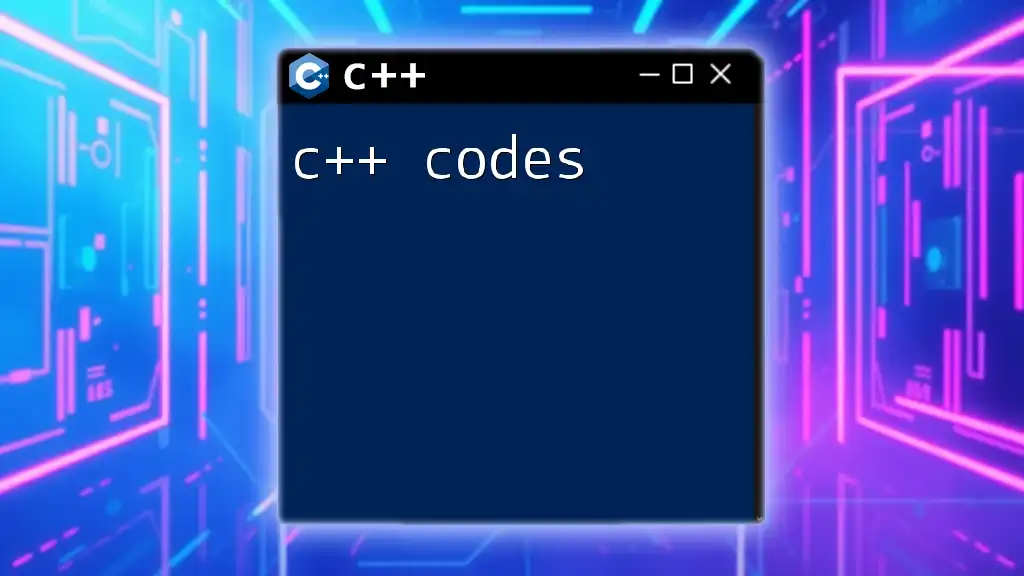
Setting Up Your Environment for CUDA Development
Installing CUDA Toolkit
To get started with C++ CUDA, the first step is to install the CUDA toolkit. This toolkit includes libraries, tools, and documentation necessary for CUDA programming.
- Download: Go to NVIDIA's [official CUDA Toolkit page](https://developer.nvidia.com/cuda-downloads) and choose the version compatible with your operating system.
- Install: Follow the installation instructions provided by NVIDIA. Pay special attention to choosing the right components for your needs.
- Verify Installation: You can verify that the installation was successful by running the included sample programs.
Configuring Your C++ IDE for CUDA
After installing the CUDA toolkit, you'll need to configure your C++ development environment to support CUDA development. Most popular IDEs like Visual Studio, CLion, or even command line tools can be used effectively.
- For Visual Studio, ensure that you have the CUDA integration plugin installed during installation. You can create new projects or add CUDA files (.cu) to your existing C++ projects.
- If you are using CLion, integrate the CMake configuration to include CUDA compile flags and link necessary libraries.
- For those who prefer command-line tools, ensure that the CUDA compiler (nvcc) is available in your path to compile .cu files.
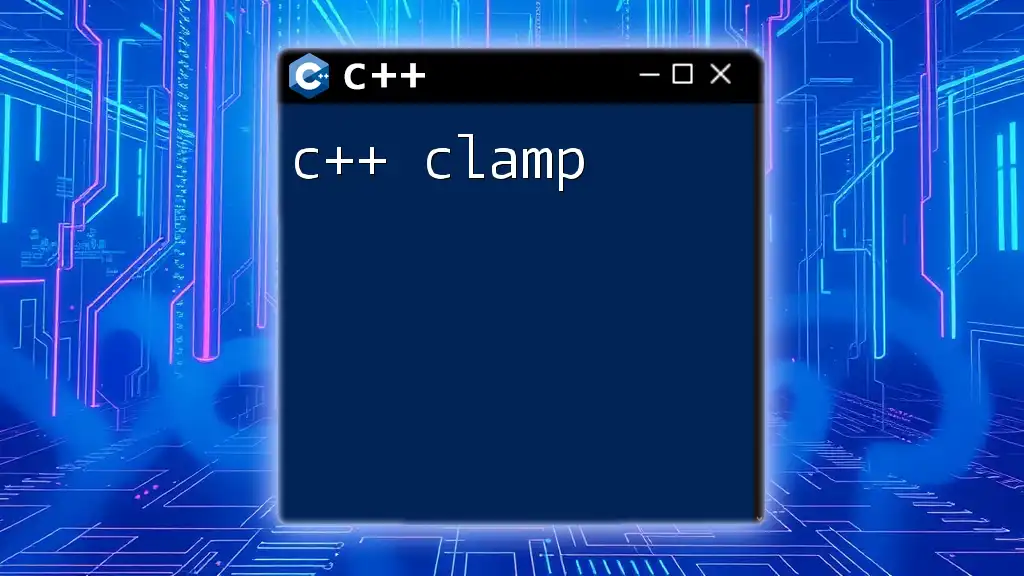
Understanding CUDA Architecture
CUDA Architecture
At the heart of C++ CUDA programming lies the compute unified device architecture. CUDA architecture focuses on the use of parallel processing and consists of:
- Kernels: Functions that run on the GPU but are called from the CPU. They execute on multiple threads in parallel.
- Grids and Blocks: Kernels are executed in grids, which consist of a number of blocks. Each block contains a number of threads, effectively organizing how tasks are distributed over the GPU.
Memory Hierarchy in CUDA
Understanding memory types in CUDA is crucial for optimizing performance. CUDA divides its memory into several types:
- Global Memory: Accessible by all threads, but relatively slow.
- Shared Memory: Much faster, shared among threads in the same block, useful for inter-thread communication.
- Local Memory: Private to each thread, used for automatic variables.
Here’s a code snippet to illustrate memory allocation on the device:
float *d_a; // Device pointer
cudaMalloc((void**)&d_a, size * sizeof(float)); // Allocating memory on the device
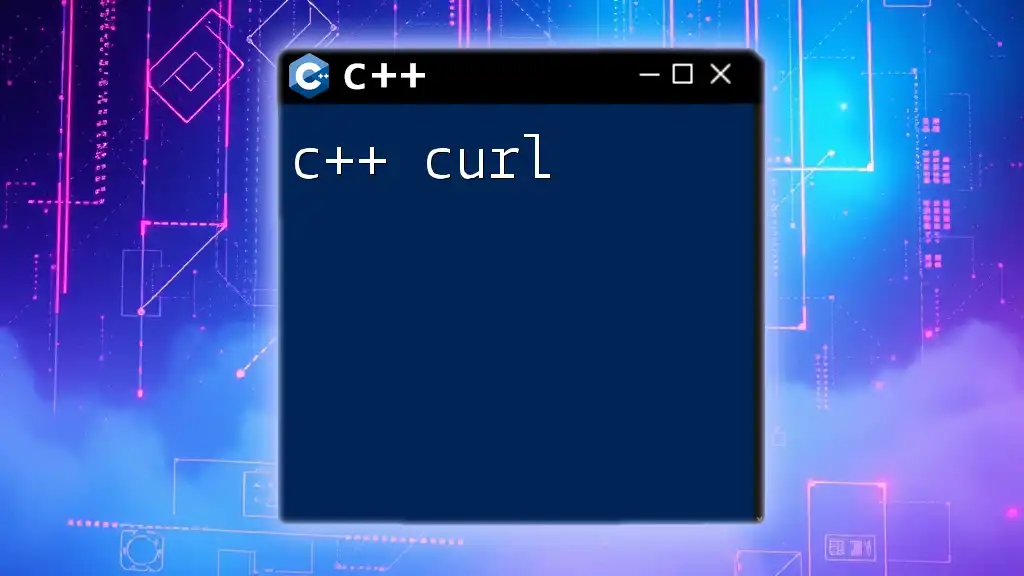
Writing Your First CUDA Program in C++
Basic Structure of a CUDA Program
A CUDA program typically consists of host code (executed on the CPU) and device code (executed on the GPU). To define a kernel, you use the `global` keyword.
Hello World Example in CUDA
Here’s a simple example of a CUDA kernel that prints "Hello from CUDA":
#include <iostream>
__global__ void helloCUDA() {
printf("Hello from CUDA\n");
}
int main() {
helloCUDA<<<1, 10>>>(); // Launch kernel with 1 block and 10 threads
cudaDeviceSynchronize(); // Wait for GPU to finish
return 0;
}
In this example, we define a kernel function that uses `printf` to print a message. The kernel is invoked with the `<<<1, 10>>>` syntax, which specifies one block with ten threads.
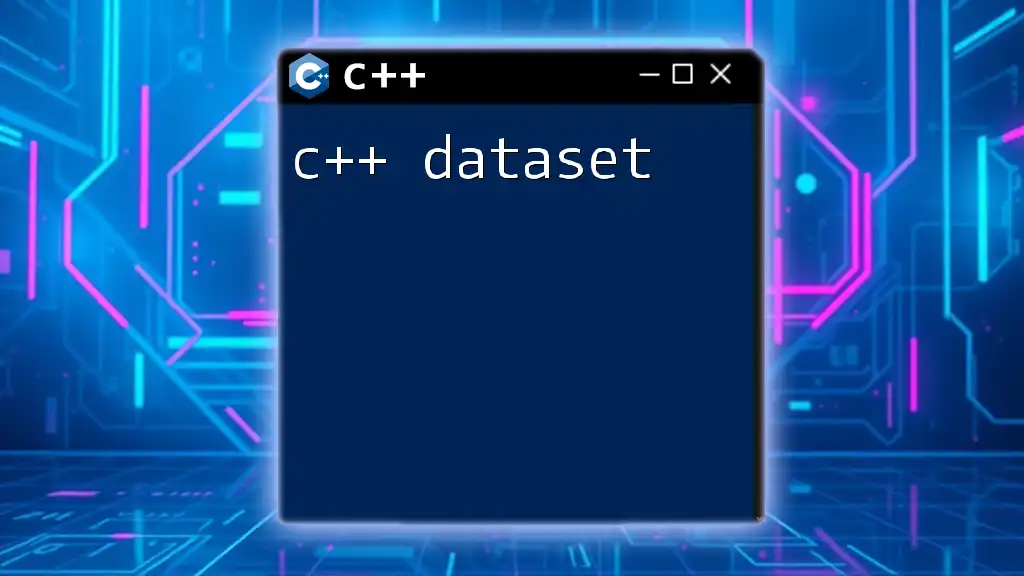
Advanced CUDA Concepts in C++
Memory Management
Efficient memory management is critical in C++ CUDA applications. Proper techniques help minimize bottlenecks. For instance, utilizing shared memory where threads need to collaborate can greatly improve performance.
To copy data from the host to the device and vice versa, you can use the `cudaMemcpy` function. Here’s an example:
float *h_a; // Host pointer
float *d_a; // Device pointer
size_t size = 1024;
// Allocate memory on host
h_a = (float*)malloc(size * sizeof(float));
// Allocate memory on device
cudaMalloc((void**)&d_a, size * sizeof(float));
// Copy data from host to device
cudaMemcpy(d_a, h_a, size * sizeof(float), cudaMemcpyHostToDevice);
Error Handling in CUDA
Always include error handling in your CUDA applications to identify problems early. CUDA provides error codes that can be checked after calls. Here's an example to check for errors:
cudaError_t err = cudaMalloc((void**)&d_a, size * sizeof(float));
if (err != cudaSuccess) {
std::cerr << "Error: " << cudaGetErrorString(err) << std::endl;
}
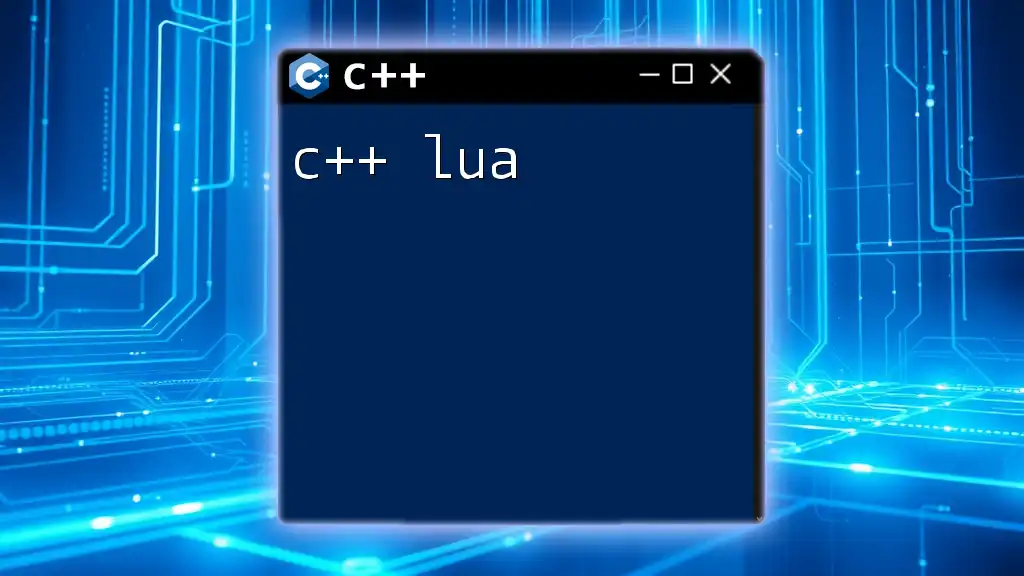
Debugging and Profiling CUDA Applications
Using CUDA-GDB and Nsight for Debugging
Debugging CUDA applications may present unique challenges due to the parallel nature of execution. Tools such as CUDA-GDB (for command-line debugging) and NVIDIA Nsight (for graphical debugging and performance analysis) are invaluable. They help track down bugs, inspect variables, and navigate through device code.
Profiling Techniques and Tools
Once your code is running, performance profiling becomes essential. NVIDIA Visual Profiler and Nsight Compute are notable tools for understanding execution bottlenecks. Profiling assists in identifying opportunities for optimization.
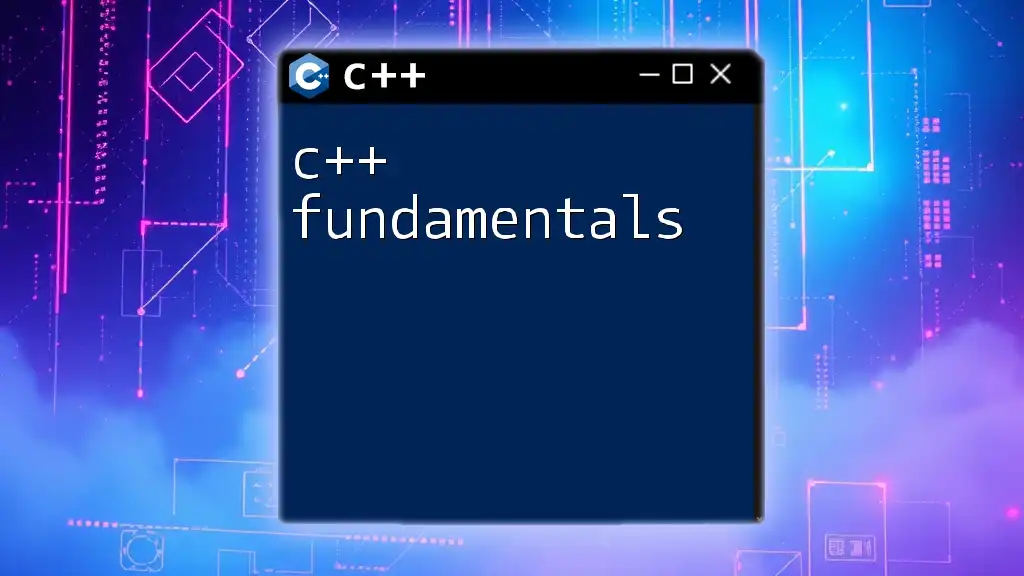
Optimizing C++ Code with CUDA
Best Practices for Writing Efficient CUDA Code
To maximize the efficiency of your C++ CUDA applications, follow these best practices:
- Memory Access Patterns: Ensure coalesced accesses to global memory and minimize bank conflicts in shared memory.
- Kernel Launch Overhead: Aim to consolidate smaller kernels into larger ones to reduce kernel launch time.
Using Thrust Library for High-Level CUDA Programming
Thrust is an advanced C++ template library for CUDA, similar to the C++ Standard Template Library (STL). It abstracts some of the complexities and allows for elegant parallel programming.
Here’s a simple example of vector addition using the Thrust library:
#include <thrust/host_vector.h>
#include <thrust/device_vector.h>
thrust::host_vector<int> h_vec(1000, 1); // Host vector with 1000 elements initialized to 1
thrust::device_vector<int> d_vec = h_vec; // Copy host vector to device
// Further operations...
Thrust simplifies many common tasks, making it easier to implement parallel algorithms.
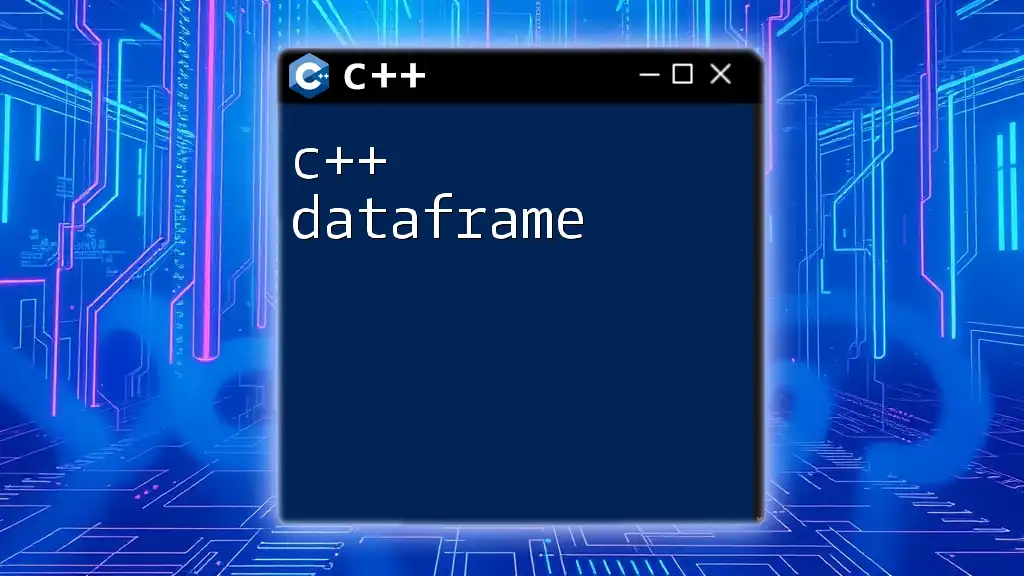
Summarizing Key Points
In this guide, we've explored the crucial elements of integrating C++ with CUDA, revealing the capabilities that GPU computing offers. By understanding the architecture, programming model, and best practices, developers can significantly enhance the performance of their applications.
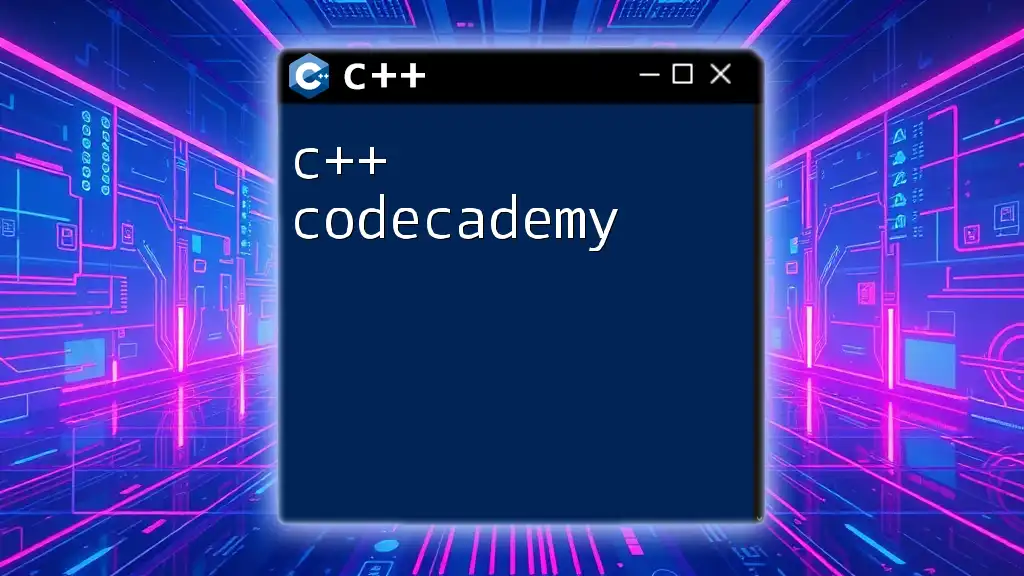
Call to Action
I encourage you to dive deeper into C++ CUDA programming. Explore complex algorithms, experiment with performance optimization techniques, and leverage NVIDIA's powerful architecture to elevate your software development skills. The world of GPU computing awaits!
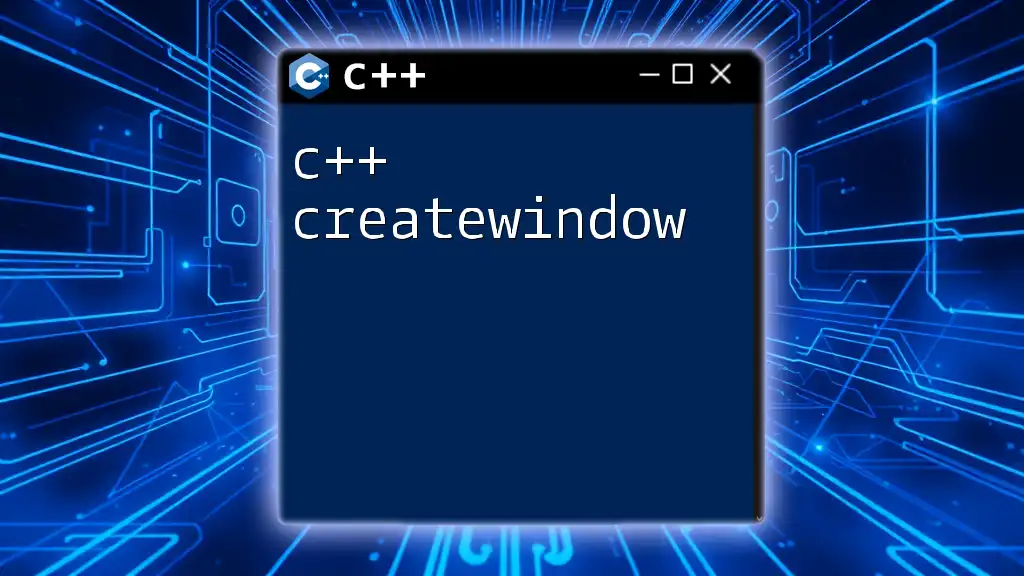
Additional Resources
For further exploration of C++ and CUDA, consider diving into recommended books and online courses, referring to NVIDIA's documentation, or engaging with community forums that focus on CUDA development. These resources will enrich your learning experience and keep you updated with the latest advancements in GPU computing.