C++ allows for direct interaction with the operating system through the use of system calls and libraries that facilitate tasks such as file manipulation and process control.
Here’s an example of using C++ to create a simple file in the operating system:
#include <fstream>
int main() {
std::ofstream outfile("example.txt");
outfile << "Hello, World!" << std::endl;
outfile.close();
return 0;
}
Understanding Operating Systems
What is an Operating System?
An operating system (OS) serves as the intermediary between users and computer hardware, enabling the execution of programs and managing hardware resources. The OS oversees crucial tasks, such as memory management, process scheduling, and input/output operations, ensuring a seamless user experience.
Components of an Operating System
Operating systems are composed of several key elements:
- Kernel: The core component responsible for managing system resources and communication between hardware and software.
- User Interface: The part that allows users to interact with the OS, which can be command-line based or graphical.
- Device Drivers: Special software that allows the operating system to communicate with hardware devices like printers or graphics cards.
Types of Operating Systems
Operating systems can be classified into several types based on their functionality:
- Batch Operating Systems: Execute batches of jobs without direct user interaction.
- Time-Sharing Operating Systems: Allow multiple users to share computing resources simultaneously.
- Distributed Operating Systems: Manage a group of independent computers and make them appear as a single coherent system.
- Embedded Operating Systems: Designed for specific control functions within larger systems, such as IoT devices or appliances.
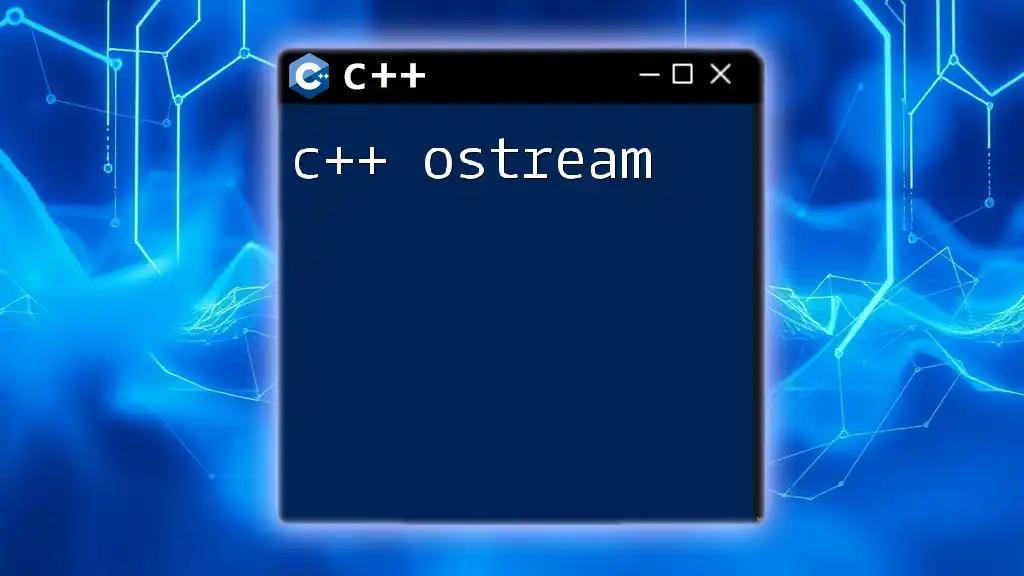
Role of C++ in Operating Systems
Advantages of Using C++ for OS Development
C++ is a popular choice for operating system development due to its powerful features:
- Object-Oriented Programming Features: C++ enables developers to create robust and maintainable code through encapsulation, inheritance, and polymorphism.
- Performance Efficiency: Being a compiled language, C++ offers performance that is closely tied to machine-level operations, making it suitable for system-level programming.
- Memory Management: C++ provides direct control over memory allocation and deallocation. This is crucial for tight resource management in an OS.
- Compatibility with C: C++ maintains strong compatibility with the C programming language, allowing developers to leverage existing C libraries and codebases.
Popular Operating Systems Developed Using C++
Many prominent operating systems have components built with C++:
- MacOS: Apple's operating system utilizes C++ in various frameworks and components, particularly in GUI development.
- Windows: Microsoft’s Windows OS includes numerous drivers and APIs written in C++.
- Some Linux Distributions: While the Linux kernel is primarily written in C, many applications and interfaces within various distributions leverage C++.
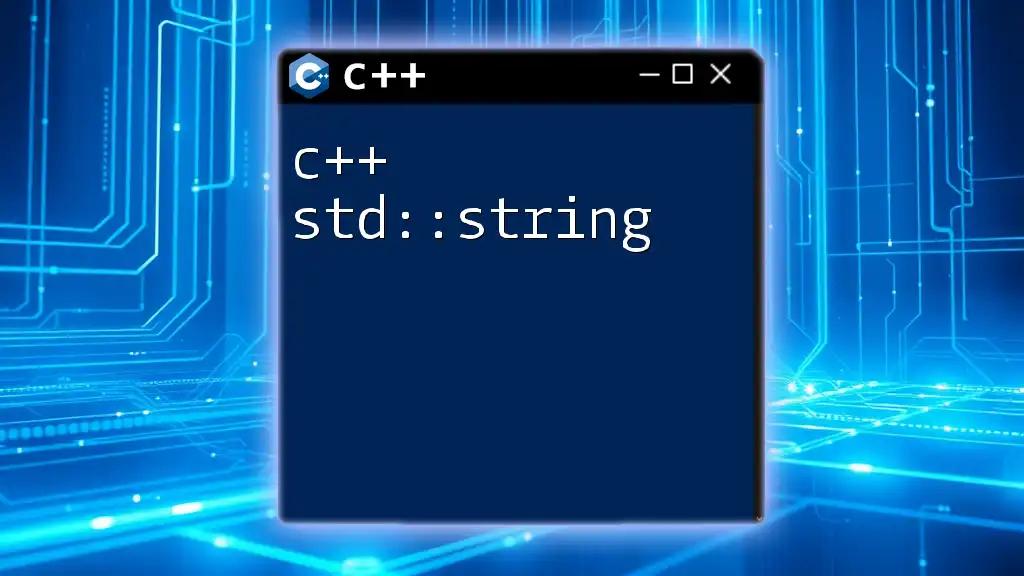
C++ Commands in OS Development
Key C++ Commands Used in OS
C++ provides several commands that are essential for developing operating systems.
Memory Management Commands
Memory management is critical in an OS context. C++ employs the `new` and `delete` operators for dynamic memory allocation and deallocation, ensuring efficient use of resources. For instance:
int* arr = new int[10]; // Allocating memory for an array
delete[] arr; // Deallocating memory
This snippet illustrates allocating an array of integers and subsequently deallocating that memory, helping prevent memory leaks.
File Handling Commands
C++ facilitates effective file management through file streams. With classes such as `ifstream`, `ofstream`, and `fstream`, developers can easily read and write files. An example of writing to a file is shown below:
std::ofstream outfile("example.txt");
outfile << "Hello, C++ OS!" << std::endl;
outfile.close();
This snippet demonstrates the process of creating a file and writing a string to it, which is fundamental for OS commands relating to file operations.
Thread Management Commands
C++ supports multi-threaded programming through the `std::thread` class, allowing the execution of concurrent threads. A simple implementation is as follows:
void function() {
std::cout << "Thread is running!" << std::endl;
}
std::thread t(function);
t.join(); // Wait for the thread to finish
This code snippet defines a function that outputs a message, creates a thread for that function, and ensures the main program waits until the thread completes its execution.
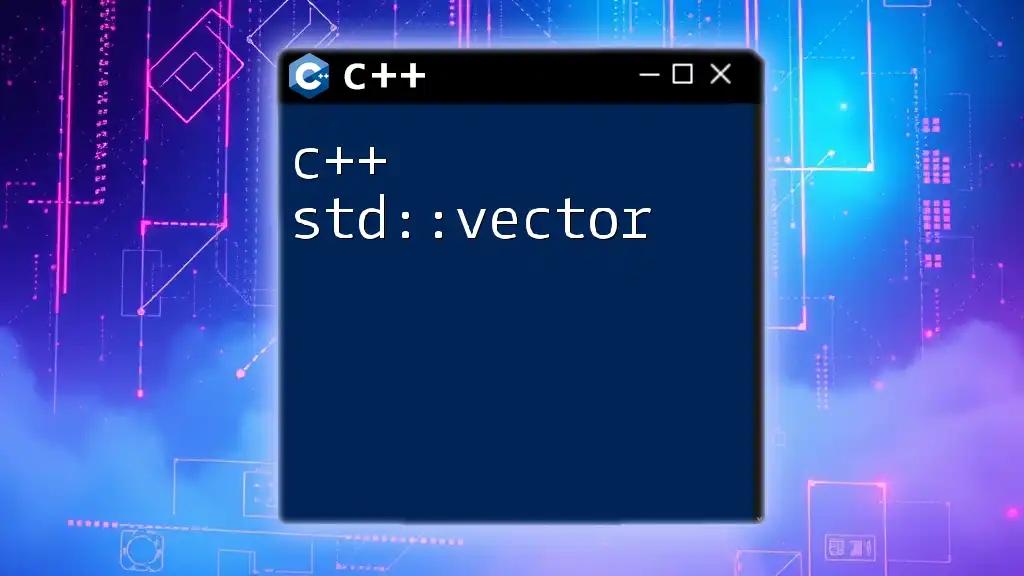
Essential Concepts of C++ in OS Design
Abstraction and Encapsulation
Abstraction in operating systems allows developers to hide complex implementation details, presenting users with simpler interfaces. Encapsulation ensures that data and code are bundled together, preventing unintended interference. For example, a class in C++ can encapsulate all the necessary details for managing a process:
class Process {
public:
void start() {/* Start process logic */}
void stop() {/* Stop process logic */}
private:
int processID;
// Other private members
};
Multi-threading in C++
Multi-threading is a cornerstone of modern operating systems, facilitating efficient CPU usage and responsiveness. With C++, developers can create responsive applications that perform multiple operations concurrently, improving the overall performance of the OS.
Error Handling in C++
Effective error handling is paramount in OS development to prevent crashes and maintain stability. C++ provides robust mechanisms through exceptions. Using `try`, `catch`, and `throw`, developers can gracefully handle errors:
try {
// OS operation code
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
This snippet demonstrates how to catch exceptions, providing informative error messages to the user or logging systems.
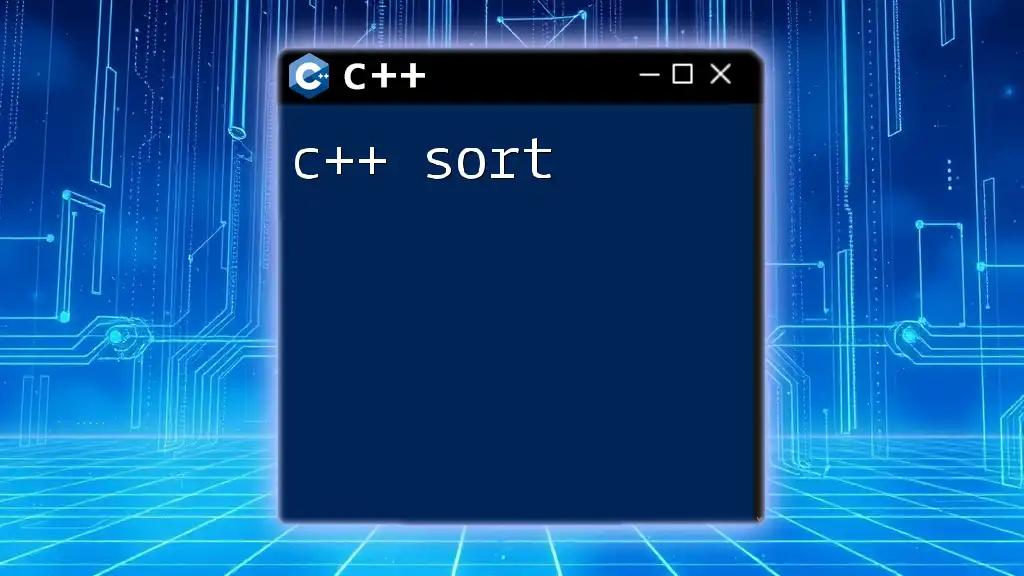
Building Simple C++ Components for Operating Systems
Creating a Simple C++ File Manager
A basic file manager serves as a practical application for understanding file operations. It can include functionalities to create, read, and delete files. Below is a skeleton code for a simple file manager:
class FileManager {
public:
void createFile(const std::string& filename) {
std::ofstream outfile(filename);
// Handle file creation logic
}
void readFile(const std::string& filename) {
std::ifstream infile(filename);
// Handle file reading logic
}
void deleteFile(const std::string& filename) {
std::remove(filename.c_str());
}
};
Developing a Basic Task Scheduler
A task scheduler is essential in an OS for managing process execution. A simple structure could look like this:
class TaskScheduler {
public:
void scheduleTask(std::function<void()> task) {
// Logic to schedule tasks
task(); // Execute the task immediately for simplification
}
};
This code snippet illustrates how a scheduler could be implemented to manage task execution, albeit in a very simplified form.

Best Practices for Using C++ in OS Development
Code Optimization Techniques
To ensure optimal performance in operating systems, developers should adhere to best practices in code optimization:
- Reduce Memory Footprint: Aim to use memory efficiently by avoiding unnecessary allocations.
- Improve Performance: Use algorithms and data structures that suit the OS’s needs.
Testing and Debugging
Testing plays a critical role in OS development. Employing systematic testing ensures that various components operate as expected, minimizing bugs that lead to system failures. Tools such as gdb or Valgrind assist in debugging C++ applications, providing insights into memory usage and runtime behavior.

Conclusion
C++ has carved a niche in operating system development due to its performance efficiency, robust features, and ability to manage system resources effectively. Understanding C++ commands and principles is essential for any aspiring OS developer. With the ongoing evolution of technology, C++ will continue to play an integral role in driving the development of innovative operating systems.
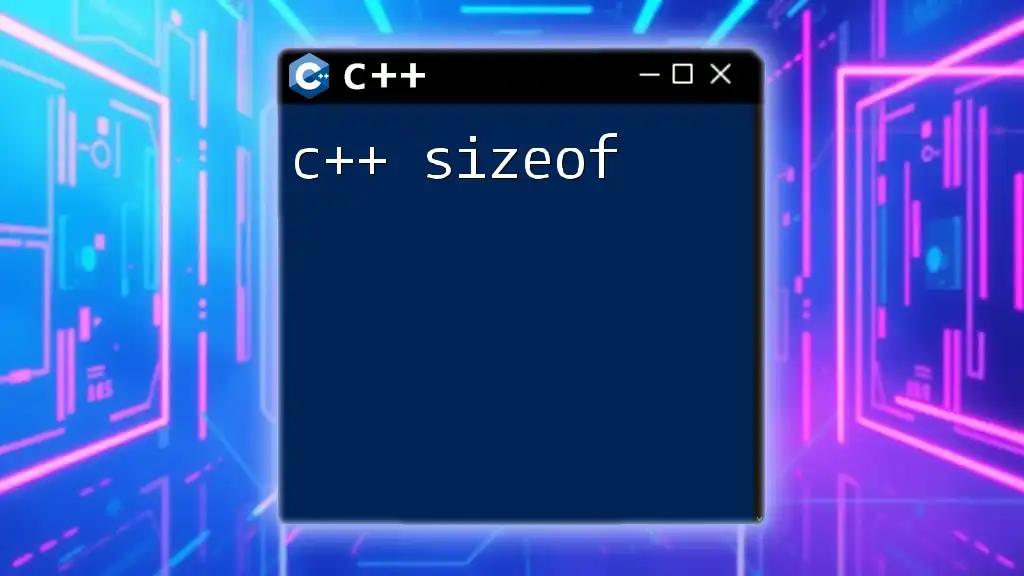
Call to Action
If you’re eager to delve deeper into C++ OS commands and applications, now is the perfect time to explore dedicated learning platforms and resources. Join our community and take your first steps in mastering C++ for operating systems!