C++ is a powerful, high-performance programming language that supports procedural, object-oriented, and generic programming paradigms.
Here's a simple example demonstrating the basic structure of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is C++?
C++ is a general-purpose programming language created by Bjarne Stroustrup at Bell Labs in the early 1980s. It extends the C programming language by adding features that support object-oriented programming (OOP), which helps manage complex software projects by mimicking real-world entities.
Historically, C++ emerged as an enhancement to C, maintaining C's performance while introducing user-friendly constructs, making it ideal for system software, game development, and applications that require high performance.
Understanding the significance of C++ in today’s technology landscape is pivotal. From operating systems to game engines, it powers some of the most critical applications, underscoring its versatility and reliability.
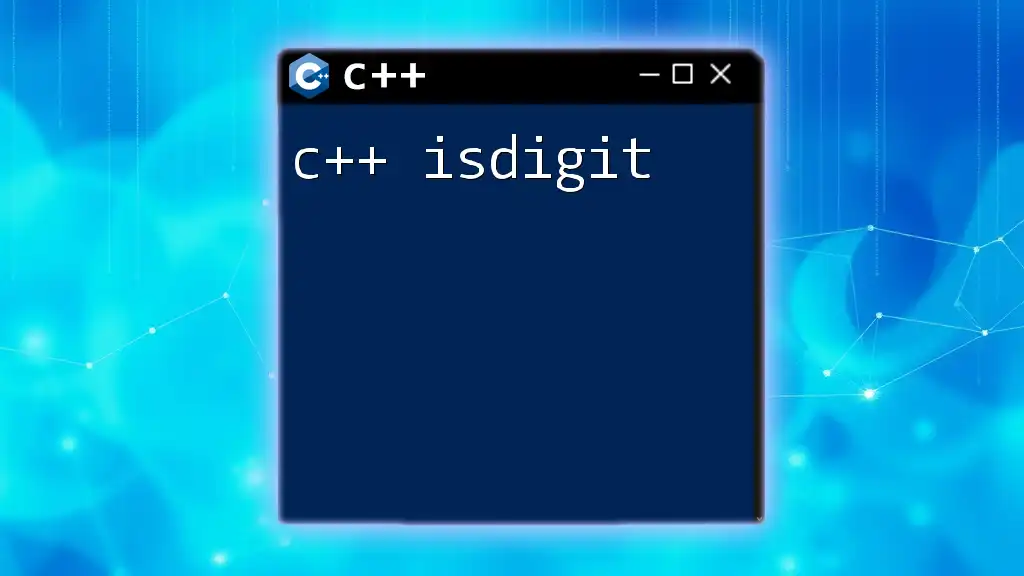
Why Learn C++?
Learning C++ opens up numerous avenues in programming and software development. Some key reasons to learn C++ include:
-
Versatility: C++ is used across various sectors, including finance, gaming, scientific computing, and even embedded systems. Its flexibility allows developers to adapt it to different projects effectively.
-
Performance: C++ is known for its execution speed and memory efficiency. It provides low-level access to memory through pointers and allows for fine-tuned performance optimizations, making it ideal for resource-constrained applications.
-
Object-Oriented Features: C++ is built around object-oriented concepts such as encapsulation, inheritance, and polymorphism, which help developers create maintainable and scalable code. This structure promotes code reuse and better organization in larger projects.
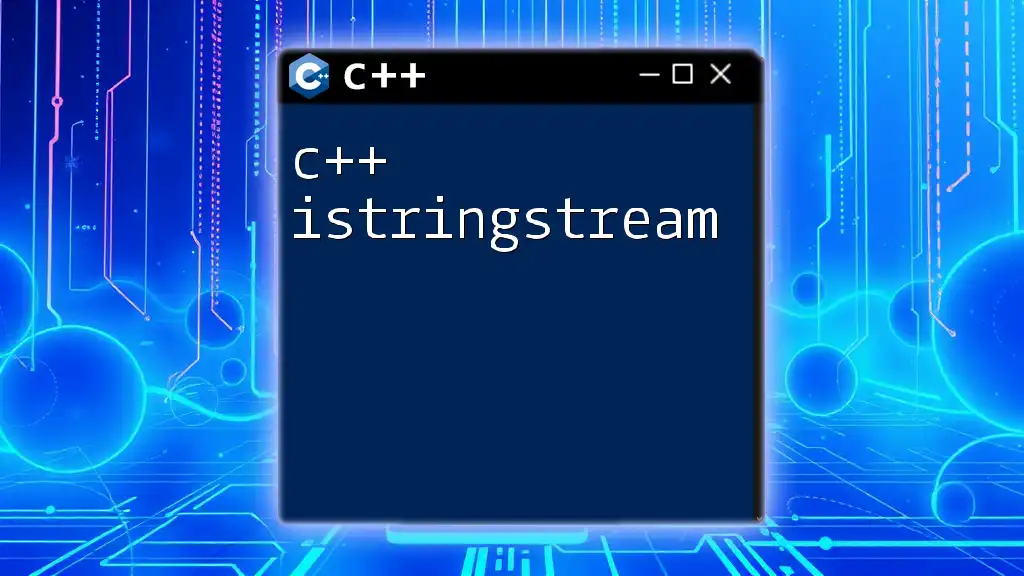
Core Features of C++
General Syntax and Structure
C++ maintains a straightforward syntax inherited from C, comprised of essential components. A basic C++ program typically includes headers, a main function, and code statements.
Here is a simple example of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This program outputs "Hello, World!" to the console. The `#include <iostream>` directive is necessary for input and output stream handling, while `std::cout` is used for printing.
Data Types and Variables
C++ supports a variety of data types, allowing developers to define variables that suit their needs. Fundamental data types include:
- int: for integers
- float: for single-precision floating-point numbers
- double: for double-precision floating-point numbers
- char: for single characters
- bool: for boolean (true/false values)
Custom data types can also be defined using structures or classes. For instance:
int age = 25;
double salary = 55000.50;
In this example, we define an integer variable to store age and a double variable for salary.
Control Structures
Control structures in C++ dictate the flow of program execution. They include:
-
Conditional Statements: Utilize `if`, `else`, and `switch` to perform different actions based on varying conditions.
-
Looping Mechanisms: Employ `for`, `while`, and `do-while` loops to repeat actions. Here’s an example of a for loop:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
Functions in C++
Defining and Calling Functions
Functions in C++ are used to encapsulate code into reusable blocks. A basic function is defined with a return type, name, and parameters:
int sum(int a, int b) {
return a + b;
}
In this example, the function `sum` takes two integer parameters and returns their total.
Function Overloading
C++ supports function overloading, allowing multiple functions to have the same name but differ in parameter type or number. For example:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
This feature enhances code readability and usability.
Object-Oriented Programming (OOP) in C++
Key Concepts of OOP
C++ is fundamentally built around the principles of object-oriented programming, which includes:
-
Classes and Objects: A class is a user-defined blueprint, which creates objects. It encapsulates data and functions that operate on the data.
-
Abstraction: Hiding complex implementation details and exposing only essential features.
-
Encapsulation: Keeping data safe from outside interference and misuse.
-
Inheritance: Deriving new classes from existing ones, enabling code reuse.
-
Polymorphism: Allowing methods to do different things based on the object that it is acting upon.
Implementing Classes
Creating classes in C++ allows for data abstraction and encapsulation. Here’s an example:
class Car {
public:
std::string model;
int year;
void display() {
std::cout << model << " - " << year << std::endl;
}
};
In this snippet, we define a `Car` class with public attributes and a method to display its details.
Inheritance and Polymorphism
Inheritance enables a new class to inherit properties and methods from an existing class. Here’s an example of a simple inheritance case:
class Vehicle {
public:
void start() {
std::cout << "Vehicle started" << std::endl;
}
};
class Car : public Vehicle {
};
The `Car` class inherits from `Vehicle`, allowing it to use the `start` method.
Polymorphism can be exhibited through method overriding and late binding, allowing derived classes to implement methods defined in base classes differently.
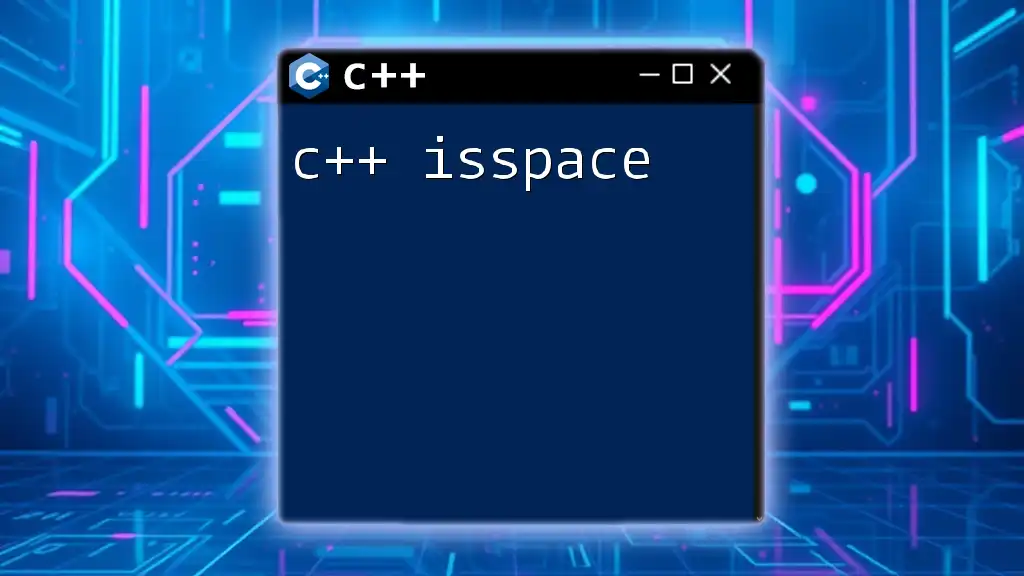
Advanced C++ Concepts
Templates in C++
What are Templates?
Templates allow the creation of generic classes and functions in C++. This means you can write functions and classes that work with any data type without overloading your code unnecessarily.
Implementing Function Templates
Here’s how to define a function template:
template <typename T>
T add(T a, T b) {
return a + b;
}
With this template, you can call `add` with integers, floats, or any other types without redefining the function.
Exception Handling
C++ offers robust exception handling to manage errors. Utilizing `try`, `catch`, and `throw` allows developers to create graceful error management.
try {
throw std::runtime_error("An error occurred");
} catch (std::runtime_error &e) {
std::cout << e.what() << std::endl;
}
This mechanism captures exceptions and prevents program crashes, ensuring enhanced user experience.
C++ Standard Library
Overview of STL (Standard Template Library)
The Standard Template Library (STL) is a powerful subset of the C++ Standard Library that includes a set of common classes and functions. It encompasses data structures (like vectors, lists, queues), algorithms (sorting, searching), and iterators (for traversing collections).
By utilizing STL, programmers can significantly increase their productivity and reduce development time thanks to reusable components.
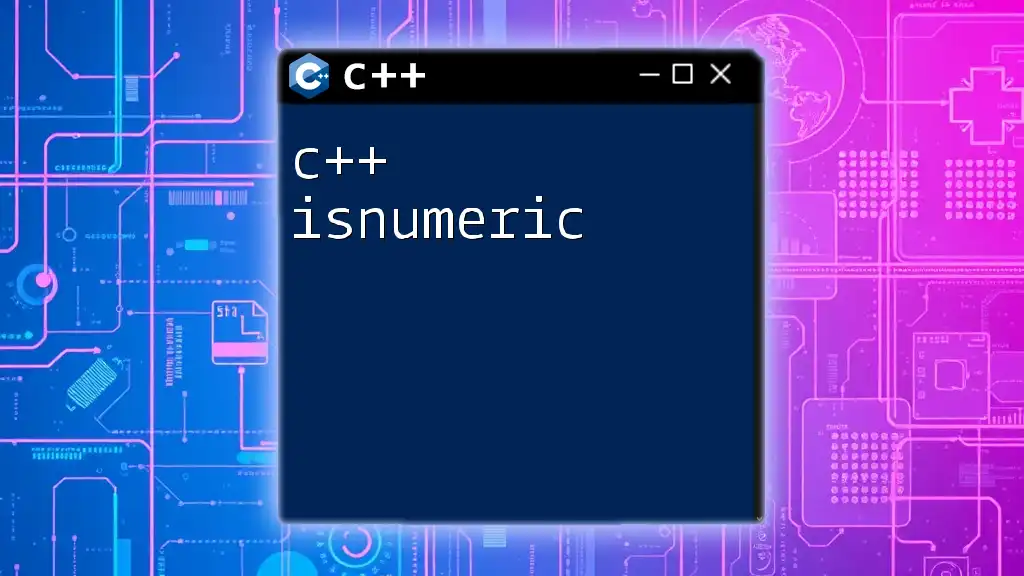
Practical Applications of C++
C++ in Game Development
C++ holds a prominent position in game development, being the backbone of many popular gaming engines such as Unreal Engine. Its performance is critical when rendering graphics and processing real-time interactions, making it the go-to language for game developers.
C++ in System/Software Development
C++ plays a vital role in software that manages hardware, such as operating systems. It is used in many applications due to its close-to-metal capabilities, which allow developers to write effective system-level code. Major software like Adobe and some parts of Microsoft Windows are built using C++.
C++ in Competitive Programming
The language is favored in competitive programming due to its speed and efficiency. C++ offers powerful features, such as STL, which accelerates implementation time for algorithms and data structures that competitors often require to solve complex problems swiftly.
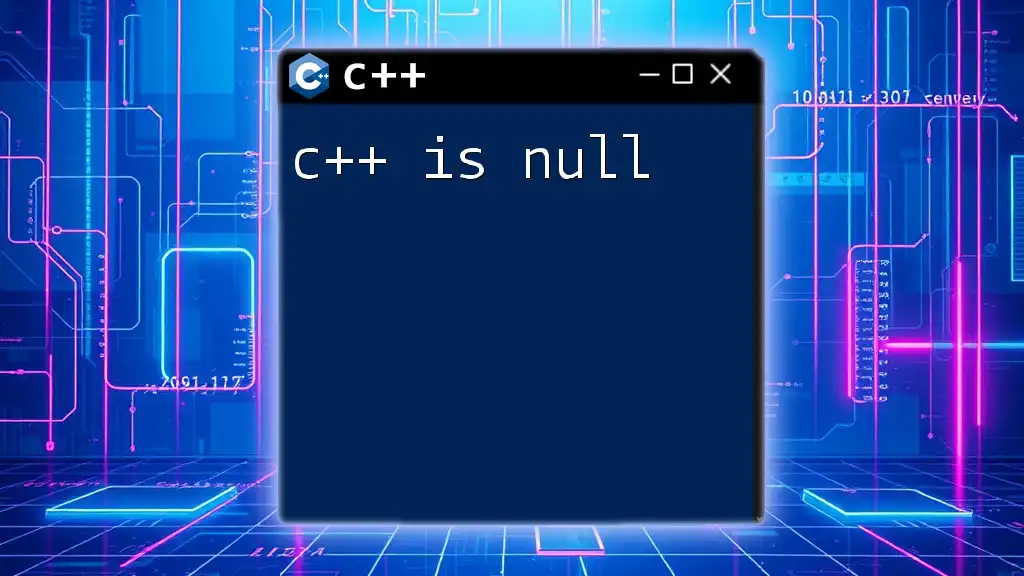
Conclusion
C++ is a vital language in the programming ecosystem, known for its versatility and performance. From developing high-performance applications to working in diverse sectors, mastering C++ opens doors to endless opportunities.
Learning about modern features and keeping up with industry trends will empower developers to make the most of this powerful language. Whether you aim to build systems software, games, or enhance your code efficiency, C++ is an invaluable skill to acquire.
The Future of C++
As C++ continues to evolve, it remains relevant in the ever-changing tech landscape. With ongoing developments and resources available for learners, there is no better time than now to dive into C++ and explore its depths. To further your journey, consider utilizing available materials and courses designed to enhance your understanding and mastery of this programming jewel.