C++ is considered a high-level programming language because it provides abstraction from machine-level code, allowing developers to write programs using more human-readable syntax while still offering the ability to manipulate hardware efficiently.
Here’s a simple example of a C++ program that demonstrates high-level syntax with basic input and output:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is a High-Level Language?
A high-level language can be defined as a programming language that offers strong abstraction from the details of the computer's hardware. Unlike low-level languages like assembly or machine code, high-level languages allow developers to write code that is more recognizable and easier to understand. This abstraction enables programmers to focus on problem-solving and logic, rather than on the intricate workings of the machine.
Characteristics of High-Level Languages
High-level languages possess distinct characteristics that aid in understanding their functionality and advantages:
- Abstraction: High-level languages facilitate the handling of complex tasks by providing built-in functions and data types, thereby making programming more intuitive.
- Readability: The syntax of high-level languages is designed to be more understandable by humans, resembling natural language phrases.
- Portability: Programs written in high-level languages can often be run on different types of systems with minimal modification, promoting broader applicability.
- Ease of Use: These languages typically require less code to perform complex tasks, significantly reducing development time compared to lower-level languages.
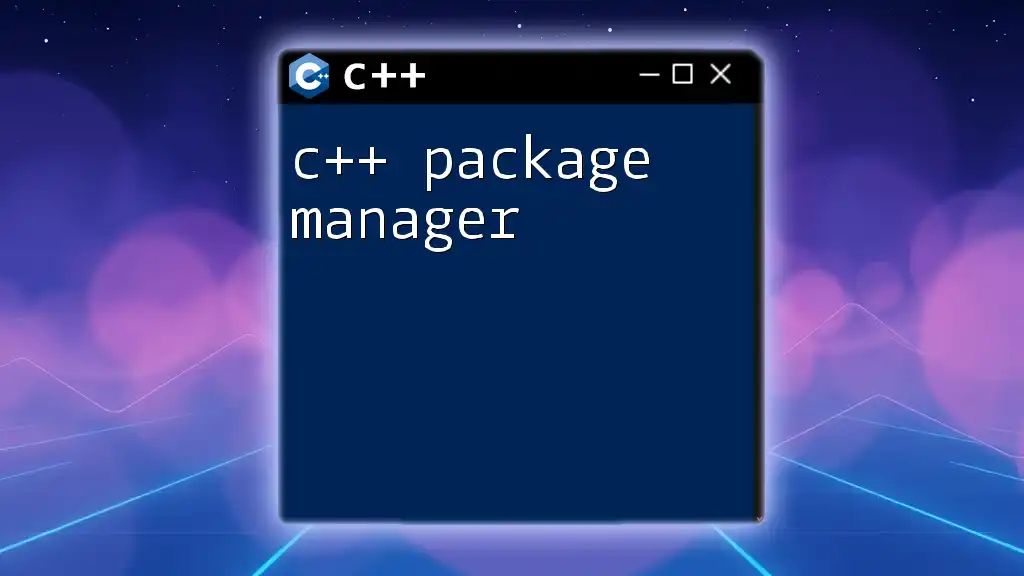
C++: Bridging the Gap Between High-Level and Low-Level
Overview of C++
C++ is a powerful programming language that has evolved since its inception in the 1980s by Bjarne Stroustrup. Initially designed as an extension of the C programming language, C++ offers robust features while maintaining an efficient low-level performance. This duality makes C++ versatile: it can be employed for software development ranging from operating systems to video games.
Features That Afford High-Level Characteristics
Object-Oriented Programming
One of the hallmark features of C++ is its support for object-oriented programming (OOP). OOP principles such as Encapsulation, Abstraction, Inheritance, and Polymorphism are essential attributes that enable developers to create modular and maintainable code.
For instance, consider a simple C++ class implementation:
class Animal {
public:
void speak() {
std::cout << "Animal speaks!" << std::endl;
}
};
In this example, we declare an `Animal` class that defines a method named `speak()`. This encapsulation of data and behavior within a class illustrates how C++ encourages organized coding practices.
Strong Type Checking
C++ provides strong type checking, which enhances the reliability and integrity of code. Variables in C++ have strict data types, allowing the compiler to catch errors at compile-time rather than runtime. This reduces the risk of bugs in more extensive codebases.
For instance, see the following code:
int number = 5; // Integer type
double decimalNumber = 5.0; // Double type
// This would give a compile-time error
// decimalNumber = "text";
Attempting to assign a string to a double variable would introduce an error that the compiler identifies before execution, ensuring robust and reliable coding practices.
Standard Template Library (STL)
The Standard Template Library (STL) is an invaluable feature of C++. It provides a collection of useful data structures and algorithms that enhance the speed and efficiency of development processes. By using STL, developers can implement complex data manipulations with minimal code.
Here is a practical example of using a vector from STL:
#include <vector>
#include <iostream>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
for (int i : vec) {
std::cout << i << " ";
}
}
The above code initializes a vector containing integers and iterates over them, printing the values. This showcases how C++ simplifies working with collections.
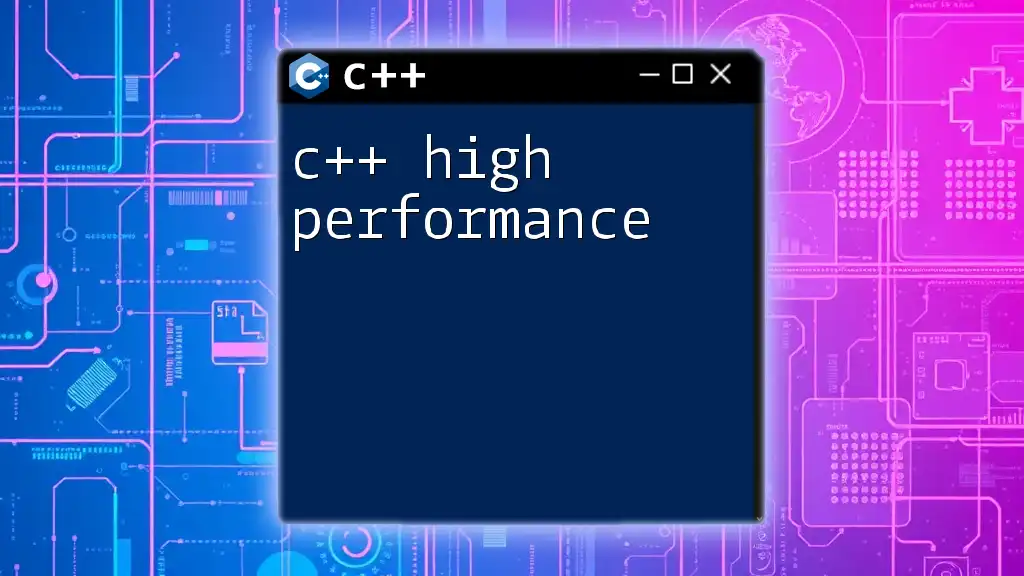
Advantages of C++ as a High-Level Language
Enhanced Productivity
C++ significantly enhances productivity by allowing programmers to accomplish more tasks with less code. The high level of abstraction means that developers can concentrate on achieving functional outcomes rather than getting bogged down in details. For instance, one can use libraries and frameworks that ease the development process rather than writing every feature from scratch.
Extensive Libraries and Frameworks
C++ boasts a rich ecosystem of libraries that cater to a wide range of applications. Libraries enable developers to leverage pre-built functions and tools, expediting the development process. For example, the Boost library offers numerous utilities to facilitate better programming practices. Another popular library is OpenCV, which is extensively used for image processing tasks.
The following code is an example of utilizing the Boost library:
#include <boost/algorithm/string.hpp> // Example of using Boost
std::string str = "Boost C++ Libraries";
boost::to_upper(str);
std::cout << str; // Outputs: "BOOST C++ LIBRARIES"
Strong Community Support
The C++ community has a wealth of resources and support networks that help both novices and experienced programmers. Numerous online forums, websites, and documentation exist to guide users in solving problems and building upon their C++ knowledge. This community support is invaluable for learning resources and enhancing coding skills.
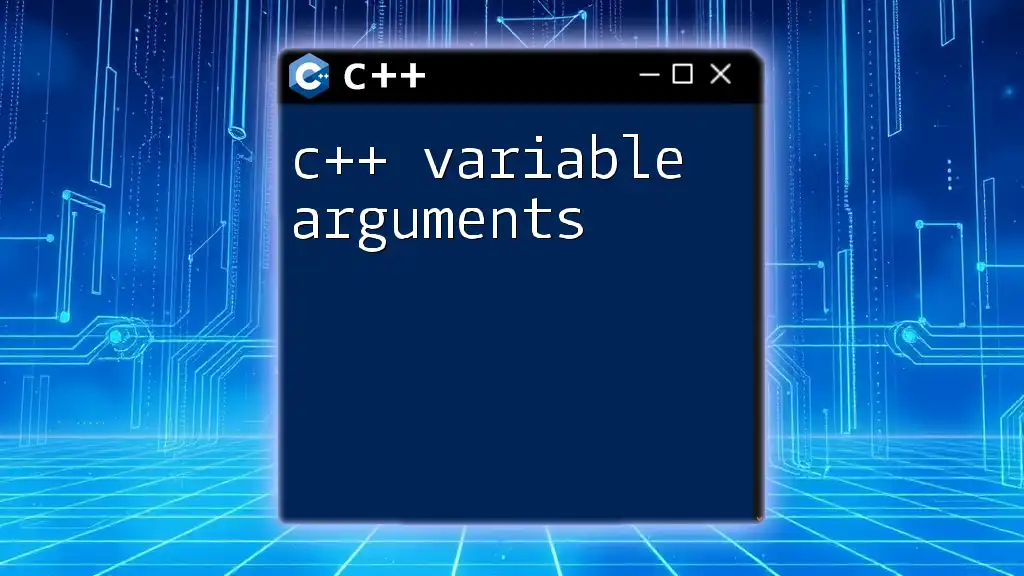
Common Misconceptions About C++
C++ is Just a Middle-Level Language
A prevalent misconception is that C++ is merely a middle-level language. Such terminology can obscure the true nature of C++. While it encompasses some characteristics of low-level languages, it excels in high-level capabilities. Ultimately, categorization can be limiting, as C++ provides unique features not exclusively tied to low or high levels.
C++ Cannot Be Used for High-Level Applications
Some may believe that C++ is not suitable for high-level applications, which is a misconception. C++ is extensively used in various high-level applications, including game development, desktop applications, and even systems programming. For example, popular game engines like Unreal Engine leverage C++ for performance and efficiency while offering high-level design capabilities.
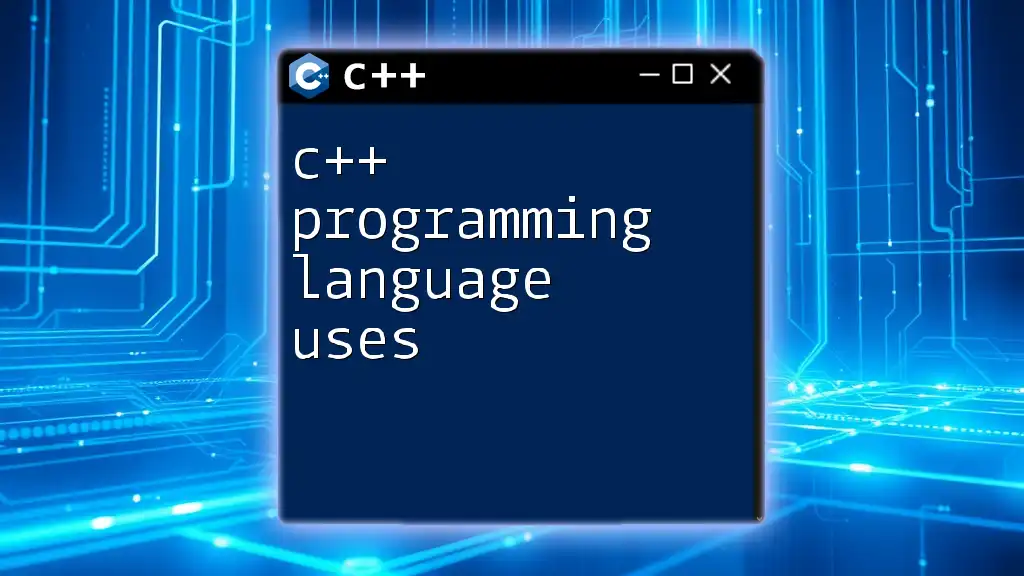
Conclusion
In summary, C++ is undoubtedly a high-level language with many advantages that facilitate efficient programming practices. Its high level of abstraction, support for object-oriented design, and rich ecosystem of libraries make it a preferred choice for many developers. By understanding C++’s strengths, programmers can effectively harness its capabilities and navigate the modern programming landscape.
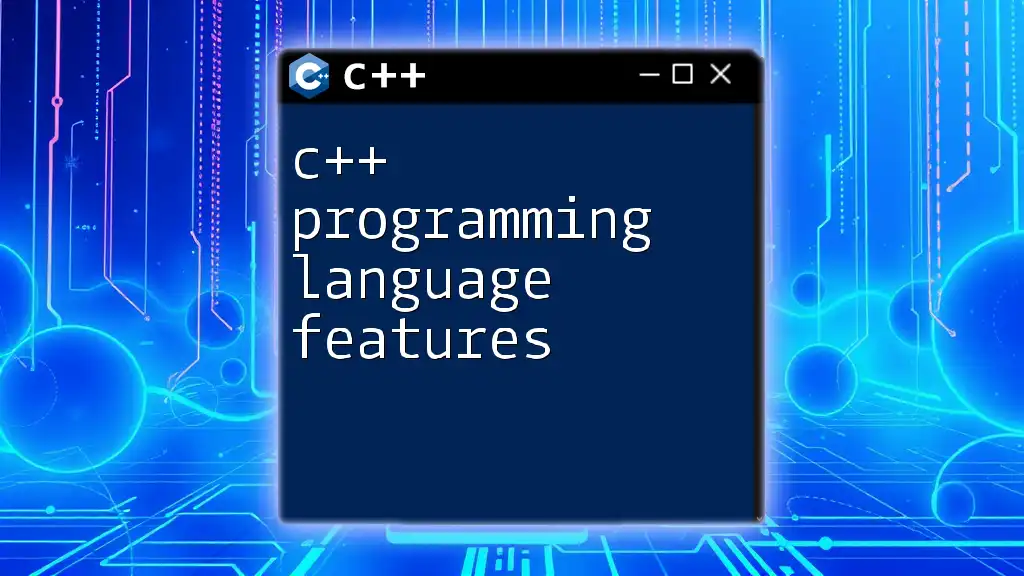
Call to Action
If you’re eager to dive deeper into the world of C++, consider exploring our tutorials and courses to enhance your skills. Whether you’re a beginner or an experienced programmer, the C++ community thrives on sharing knowledge. Join the conversation in our forums, where you can exchange insights and learn from your peers’ experiences.
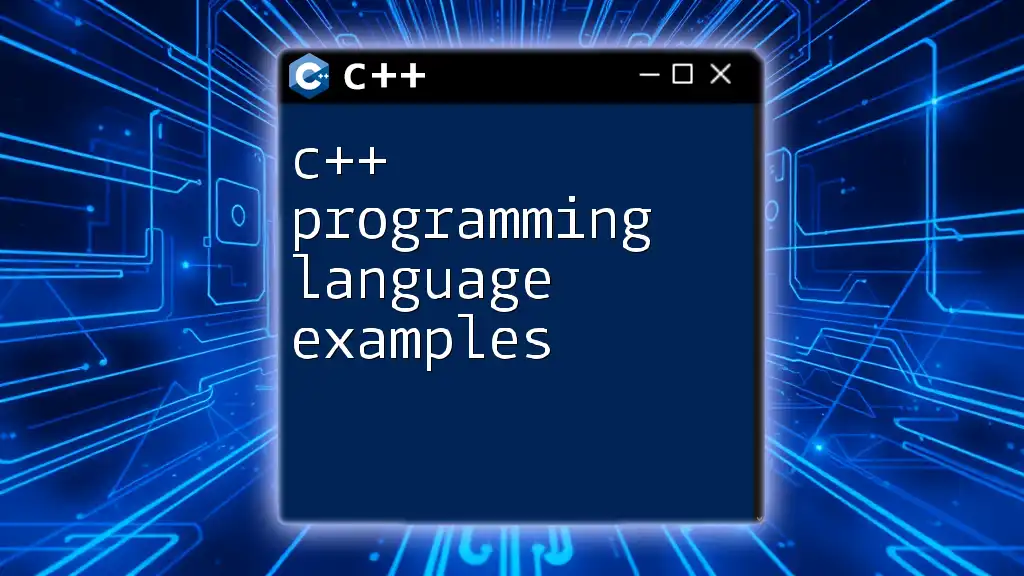
Additional Resources
To further your understanding of C++, refer to the recommended reading materials and links to online courses you can explore. Engage with forums where you can seek help and connect with fellow learners dedicated to mastering this versatile and powerful high-level programming language.