The greatest common divisor (GCD) in C++ can be efficiently calculated using the Euclidean algorithm, which repeatedly applies the formula GCD(a, b) = GCD(b, a % b) until one of the numbers becomes zero.
Here's a concise code snippet to demonstrate this:
#include <iostream>
int gcd(int a, int b) {
return b == 0 ? a : gcd(b, a % b);
}
int main() {
int num1 = 48, num2 = 18;
std::cout << "GCD of " << num1 << " and " << num2 << " is " << gcd(num1, num2) << std::endl;
return 0;
}
What is the Greatest Common Divisor?
The Greatest Common Divisor (GCD) is a mathematical concept that refers to the largest positive integer that divides two or more integers without leaving a remainder. For example, the GCD of 8 and 12 is 4 since it is the highest number that can divide both 8 and 12.
Understanding the GCD is not only significant in number theory but also plays an important role in various algorithms, data structures, and programming challenges. In C++, knowledge of GCD can be immensely helpful in optimizing algorithms and solving complex problems efficiently.
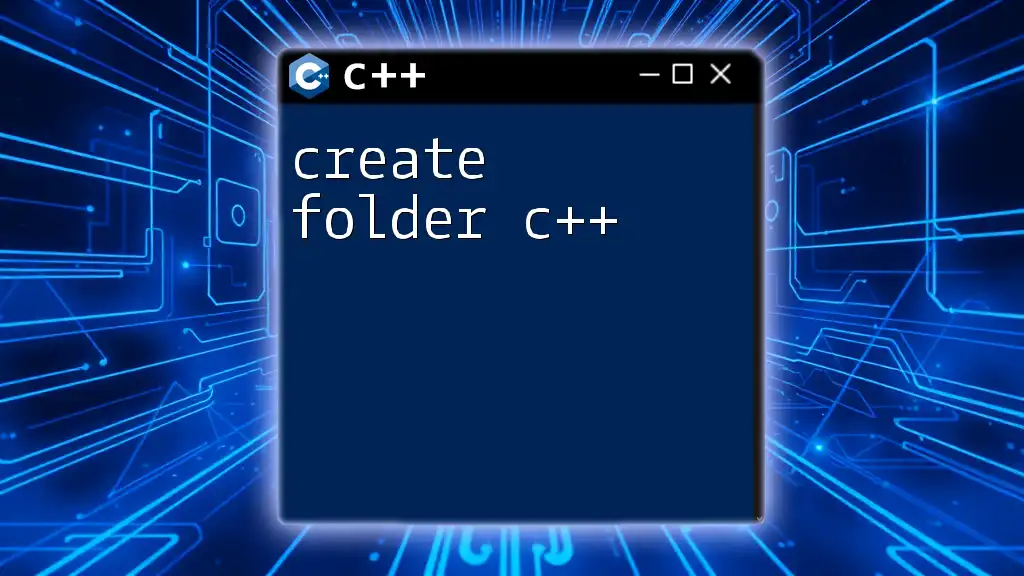
Understanding GCD vs. GCD in C++
While many may get confused by the terms Greatest Common Divisor and Greatest Common Denominator, it’s essential to note that they have different applications. The GCD pertains primarily to whole numbers, whereas the Greatest Common Denominator is often discussed in the context of fractions.
In C++, the concept of the GCD can be especially useful when working with fractions, simplifying calculations, or when optimizing algorithms that may involve common factors among inputs.
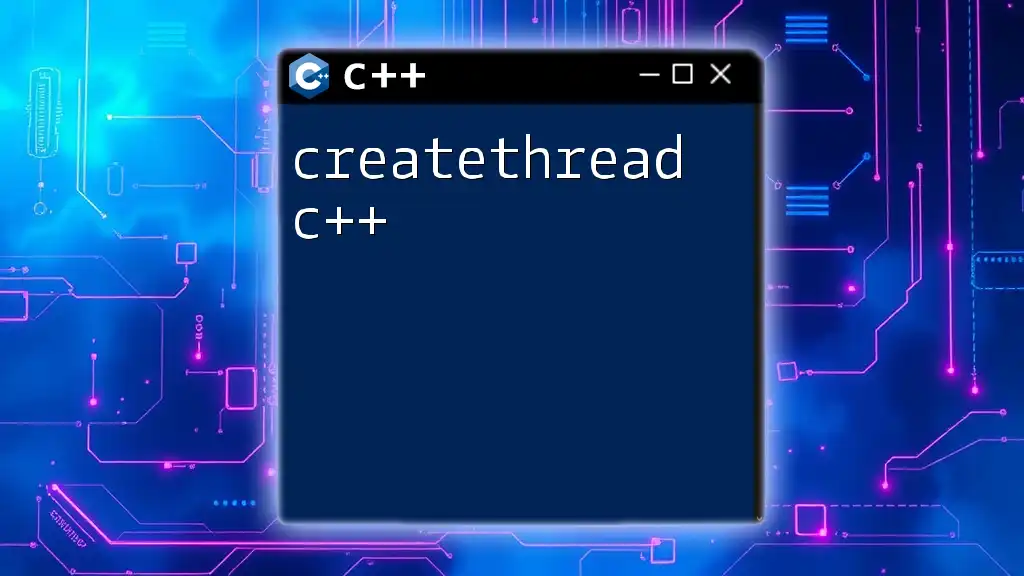
Mathematical Background of GCD
Basic Properties of GCD
The GCD has several key properties that make it easier to compute and understand:
- Commutative Property: GCD(a, b) = GCD(b, a)
- Associative Property: GCD(a, GCD(b, c)) = GCD(GCD(a, b), c)
Using these properties, one can initiate a series of mathematical operations to compute the GCD of more than two numbers efficiently.
Example: For the numbers 24, 36, and 60, we can compute the GCD as:
- GCD(24, 36) = 12
- GCD(12, 60) = 12
Thus, the GCD of 24, 36, and 60 is 12.
Real-World Applications of GCD
The GCD is not just a theoretical concept but has practical applications in:
- Simplifying Fractions: By finding the GCD of the numerator and denominator, fractions can be reduced to their simplest form. For example, the fraction 10/25 can be simplified to 2/5 by finding that GCD(10, 25) = 5.
- Cryptography: The GCD plays an essential role in various cryptographic algorithms where factors of large numbers are needed.
- Algorithm Optimization: Certain algorithms rely on GCD calculations for performance improvements, such as in number theory problems or sorting tasks.
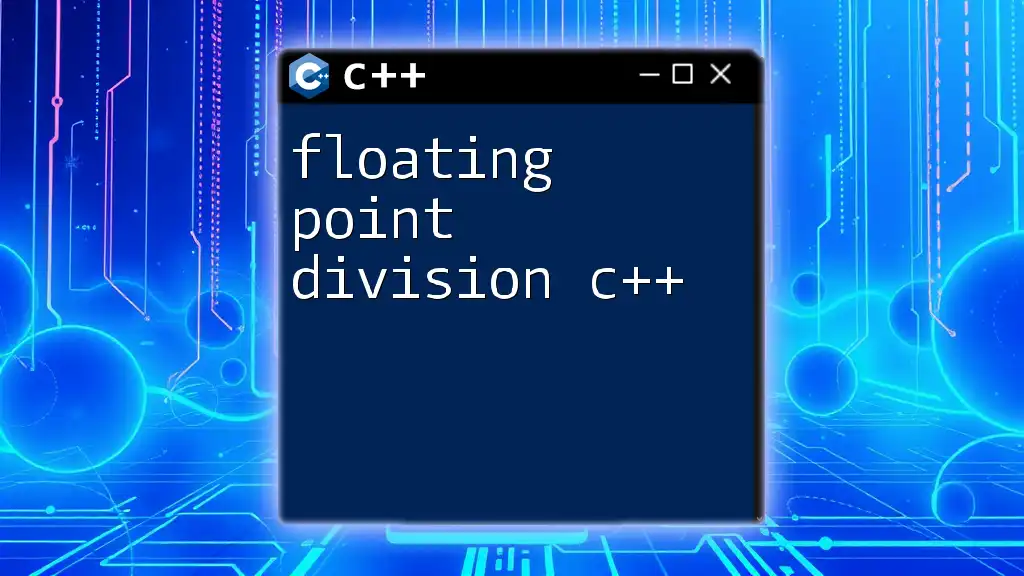
Implementing GCD in C++
Using the Euclidean Algorithm
The Euclidean Algorithm is a time-tested method for computing the GCD of two integers. The basic premise utilizes the property that GCD(a, b) is equal to GCD(b, a % b) until one of the integers becomes zero.
Here’s a simple implementation in C++:
int gcd(int a, int b) {
while (b != 0) {
int temp = b;
b = a % b;
a = temp;
}
return a;
}
Explanation
In this code snippet, the algorithm repeatedly replaces the larger number with the remainder of dividing the larger number by the smaller. When the smaller number reaches zero, the larger number is the GCD.
Input and Output Example:
- For input `gcd(48, 18)`, the output will be `6`.
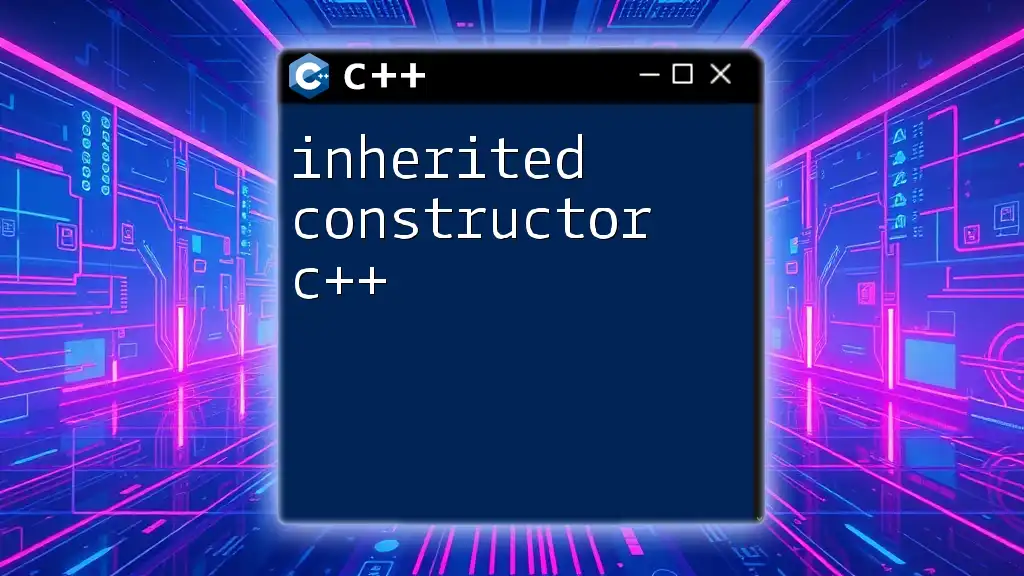
Advanced GCD Techniques
Using Recursion to Compute GCD
An elegant way to compute GCD is through a recursive function, reducing the need for iterative loops but requiring careful handling of base cases.
int gcd(int a, int b) {
if (b == 0)
return a;
return gcd(b, a % b);
}
Pros and Cons of the Recursive Method
Advantages:
- Cleaner and more expressive code
- Less boilerplate compared to iterative solutions
Disadvantages:
- May lead to stack overflow for large inputs
- Generally, iterative solutions have better performance due to lower overhead
Finding GCD of Multiple Numbers
To extend the GCD calculation to an array of numbers, one can iteratively apply the GCD function between the elements of the array:
int gcd(int a, int b) {
return b == 0 ? a : gcd(b, a % b);
}
int findGCD(int arr[], int n) {
int result = arr[0];
for (int i = 1; i < n; i++)
result = gcd(arr[i], result);
return result;
}
Explanation
This implementation initializes the result with the first element of the array and iteratively computes the GCD with every other element.
Use Cases: This method is particularly useful in scenarios where you have a list of numbers, and you need to determine their common divisors, such as in mathematical analyses or cryptographic settings.
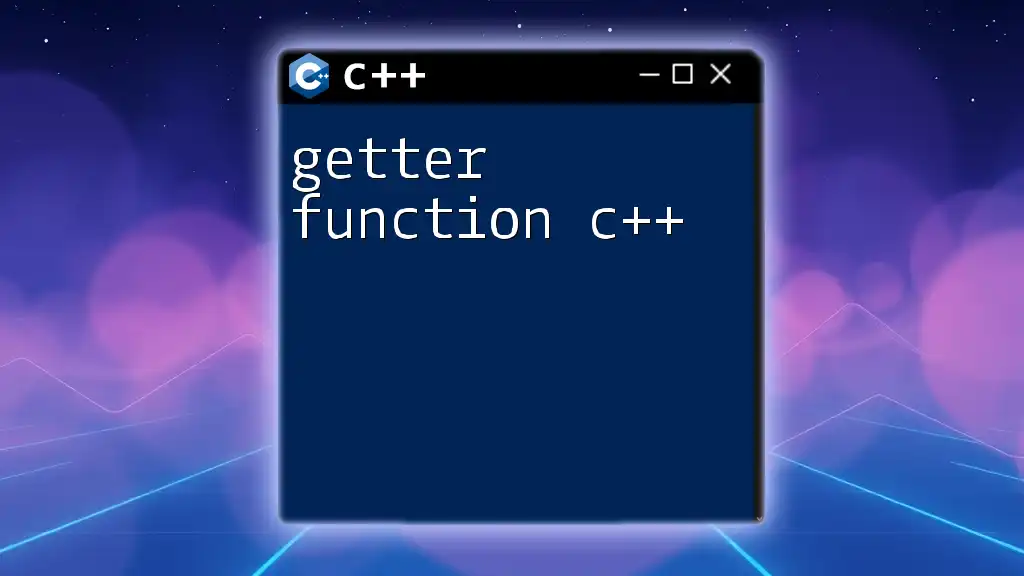
Common Pitfalls and Troubleshooting
Common Errors When Calculating GCD
- Using Incorrect Data Types: The GCD function should typically be designed to handle integers. If floats or other types are mistakenly used, it may lead to unexpected behaviors.
- Handling Negative Numbers: GCD should ideally be defined for non-negative integers. To handle negative inputs, one could use `abs(a)` and `abs(b)` in the GCD calculation.
Performance Considerations
Understanding the time complexity of various GCD algorithms is crucial for performance tuning. The Euclidean Algorithm has a complexity of O(log(min(a, b))), making it efficient even for larger integers, whereas naive methods that use more redundant computations can be significantly slower.
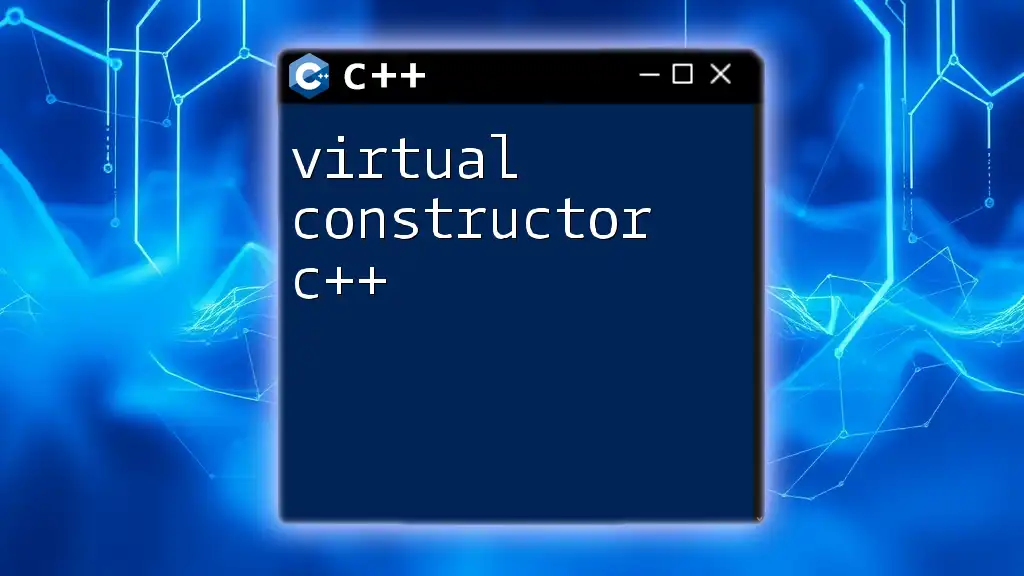
GCD Utility in Competitive Programming
Why GCD Is a Crucial Concept
In competitive programming, GCD frequently appears as a requirement for problems involving number theory and combinatorics. Efficient implementation of GCD functions can be the deciding factor in achieving optimal solutions within time limits.
Quick Tips for Mastering GCD in C++
- Practice Regularly: Solve problems involving GCD on popular platforms like LeetCode and Codeforces.
- Understand Algorithms: Deep dive into variations of the GCD algorithms to fully grasp their application.
- Optimize Your Code: Focus on efficient coding practices by minimizing unnecessary computations.
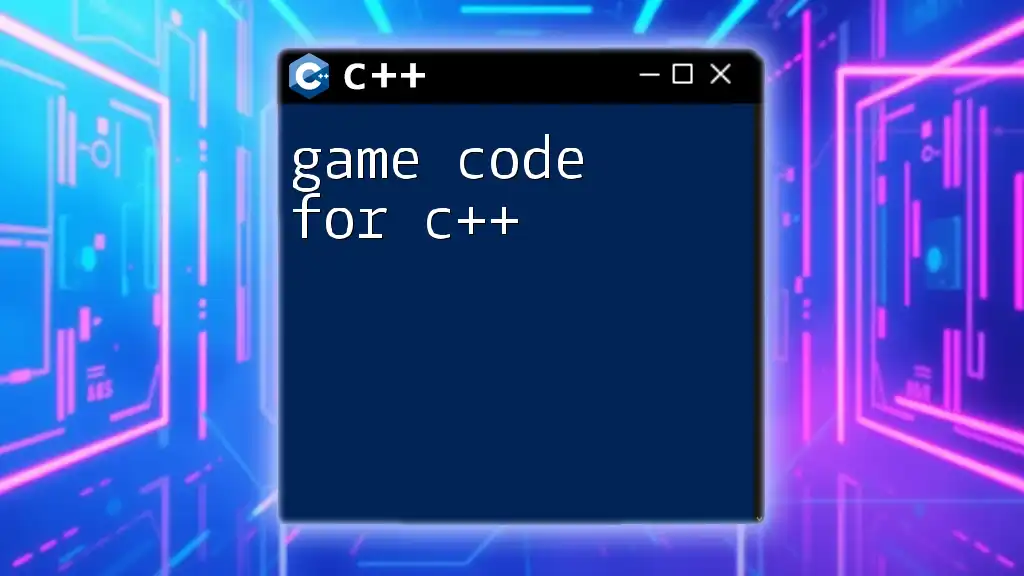
Conclusion
The Greatest Common Divisor is a fundamental concept in mathematics and programming, particularly in C++. Understanding and applying GCD effectively can significantly enhance one’s problem-solving skills and algorithmic efficiency in numerous scenarios.
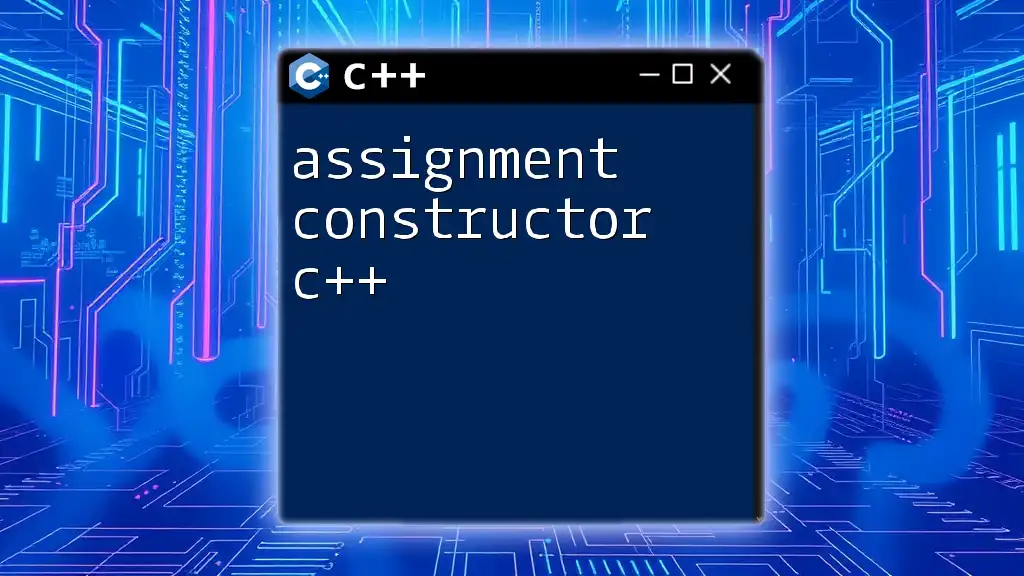
Additional Resources
To further explore the topic of GCD and related algorithms, consider looking into C++ libraries that provide built-in functions, websites offering practice problems, and books on algorithms and number theory for in-depth learning.