In C++, every variable must be declared with a specific type, which indicates the kind of data it can hold, as shown in the following example:
int age = 25; // 'int' is the type specifier indicating that 'age' will hold an integer value
Understanding Type Specifiers
What is a Type Specifier?
In C++, a type specifier is a keyword that indicates the data type of a variable or function. The type specifier plays a crucial role in programming languages, particularly those that are statically typed like C++. It informs the compiler of the kind of data that will be stored in a variable, which directly influences how the program allocates memory, performs operations, and handles errors.
Common Type Specifiers in C++
C++ offers a variety of type specifiers that can be broadly classified into fundamental data types and user-defined data types.
Fundamental Data Types include:
- `int`: for integer values
- `float`: for floating-point values
- `double`: for double-precision floating-point values
- `char`: for single characters
Example:
int age = 25;
float height = 5.9;
User-Defined Data Types allow developers to create complex data structures:
- `struct`: combines different data types
- `class`: supports object-oriented programming
- `enum`: defines a variable that can hold a set of predefined constants
Example:
struct Person {
std::string name;
int age;
};
Pointer Types are also essential in C++ as they store memory addresses, allowing for dynamic memory management and the creation of complex data structures.
Example:
int* ptr = nullptr;
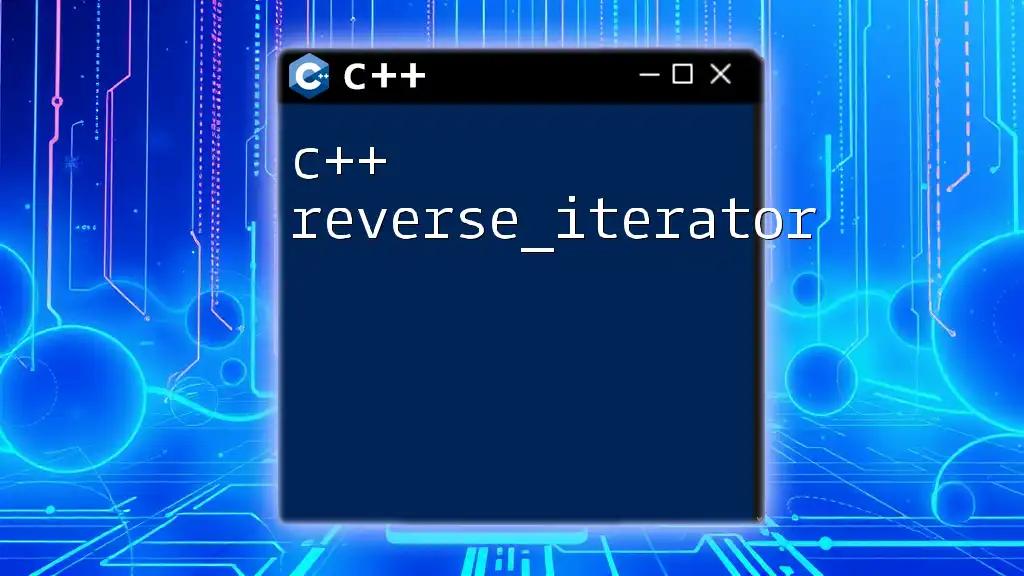
Why Type Specifiers Are Mandatory
Compiler Necessity
C++ requires a type specifier for all declarations primarily because the compiler relies on this information to generate efficient and optimized code. Type information is essential for the compiler to perform various tasks such as memory allocation, instruction selection, and error checking. Without knowing the type, the compiler cannot determine how much memory to allocate or what operations can be performed on the variable.
Type Safety
Another vital reason is type safety. Type specifiers enhance code safety by ensuring that variables are used consistently throughout their lifecycle. When a type is declared, the compiler enforces that only compatible values can be assigned to that variable. This reduces the risk of errors and undefined behavior in programs.
Consider the following incorrect declaration:
var number = 10; // Error: 'var' is not a type
In this example, omitting a type specifier results in a compilation error, highlighting the necessity of declaring types for clarity and correctness.

Declaring Variables in C++
Syntax of Variable Declaration
The general syntax for variable declaration in C++ follows this structure:
type variableName;
This requires the programmer to explicitly define the type alongside the variable name, ensuring both clarity and functionality.
Example of Correct vs. Incorrect Declarations
Correct Declaration:
float temperature = 23.5;
In this example, the variable `temperature` is clearly defined as a floating-point value, which informs both the developer and the compiler about its intended use.
Incorrect Declaration:
variable temperature; // Error: Unknown type 'variable'
This example attempts to declare a variable without a valid type specifier, demonstrating the need for correct syntax to avoid errors.
Scope and Lifetime Considerations
Type specifiers also impact the scope and lifetime of variables. Local variables declared within a function only exist during that function's execution, while global variables remain in memory throughout the program's runtime. Declaring types explicitly helps maintain code clarity and manage the lifecycle of variables effectively.

Advanced Type Usage in C++
Type Modifiers
C++ provides several type modifiers that further refine the types of data that can be stored. These include `signed`, `unsigned`, `short`, and `long`, allowing developers to specify whether a variable can hold negative values or to define the storage capacity.
Examples:
unsigned int count = 100; // Count cannot be negative
long int planets = 8; // Long int for larger integer capacity
Type Inference with `auto`
While C++ requires explicit type specifications, type inference allows the use of the `auto` keyword, which deduces the type of a variable during compilation. This feature enhances code conciseness while maintaining type safety.
Example:
auto weight = 65.0; // Type deduced as double
In this case, the compiler automatically infers that `weight` is of type `double`, making code easier to read while still adhering to type specification rules.
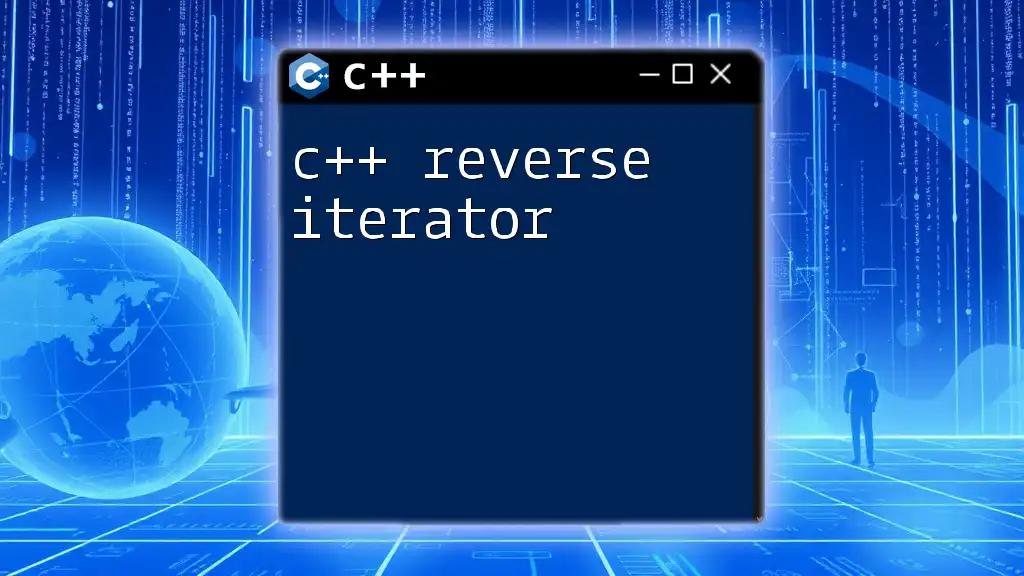
Best Practices for Declaring Variables
Clarity and Readability
When declaring variables, clarity and readability should be prioritized. Choosing descriptive type specifiers alongside intuitive variable names greatly enhances code understandability. For instance:
Clear:
std::string firstName = "John";
Unclear:
auto x = "John"; // Less informative
Consistent Naming Conventions
Implementing consistent naming conventions in conjunction with type specifiers further improves code quality. Following established guidelines, such as using meaningful variable names and adhering to camelCase or snake_case formats, significantly aids in code maintenance and collaboration.
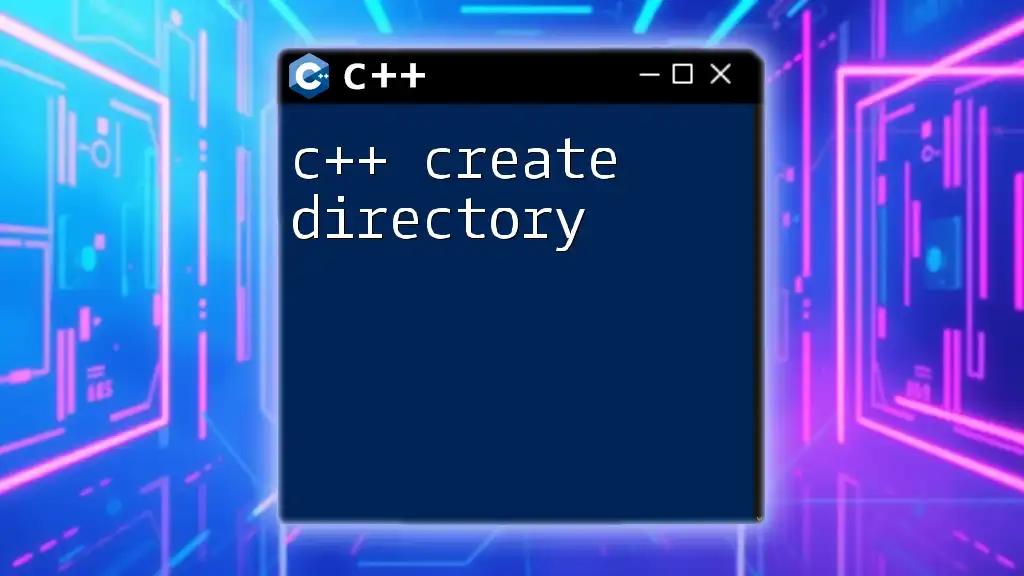
Conclusion
In summary, C++ requires a type specifier for all declarations to ensure efficient compilation, enforce type safety, and support clear and maintainable code. By grasping the significance of type specifiers, you will elevate your C++ programming skills and enhance your overall coding proficiency. As you continue to learn, practice declaring variables with appropriate types, and consider joining specialized courses to further refine your understanding of this critical concept in C++.
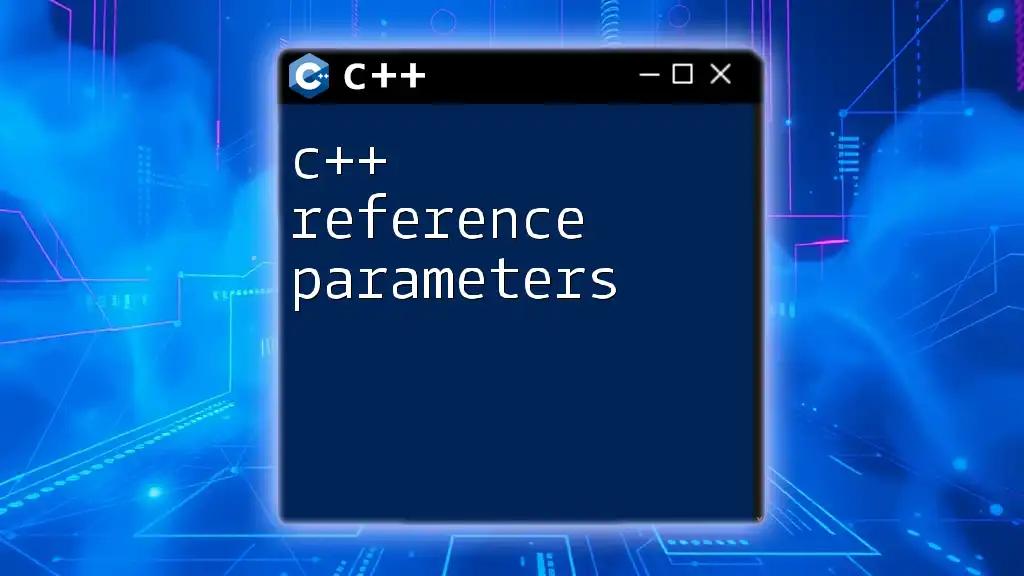
Additional Resources
For further readings, tutorials, and documentation, numerous online resources are available, alongside coding practice platforms that can help solidify your understanding of type specifiers in C++.