C++ RNG (Random Number Generation) allows developers to generate pseudo-random numbers using functions from the `<random>` library, enabling the creation of varied and unpredictable outcomes in programs.
Here's a simple code snippet demonstrating how to use C++ RNG:
#include <iostream>
#include <random>
int main() {
std::random_device rd; // Obtain random number from hardware
std::mt19937 eng(rd()); // Seed the generator
std::uniform_int_distribution<> distr(1, 100); // Define the range
// Generate and print a random number between 1 and 100
std::cout << "Random number: " << distr(eng) << std::endl;
return 0;
}
Understanding the Basics of Random Numbers in C++
What is a Random Number?
A random number is a value generated in such a way that each potential value has an equal chance of being selected. In programming, random numbers are crucial for creating unpredictable outcomes in simulations, games, and various algorithms.
Why Use Random Numbers in C++?
Random numbers serve several critical purposes in programming. They are used for generating unique identifiers, creating randomized simulations, improving the realism of game mechanics, and conducting randomized testing. Their unpredictability adds a layer of complexity and engagement to various applications.
Random vs. Pseudorandom Numbers
It is essential to distinguish between true random numbers and pseudorandom numbers. True random numbers are derived from genuine random phenomena, while pseudorandom numbers are generated through algorithms, providing sequences that only mimic randomness. C++ primarily deals with pseudorandom number generation, which is sufficient for most applications.
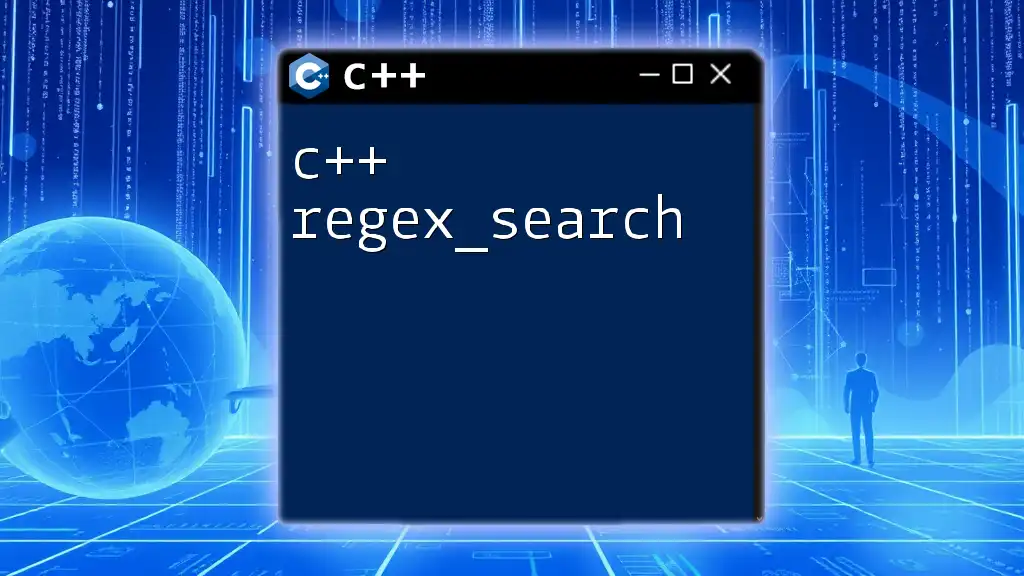
The Standard Library for Random Number Generation in C++
Introduction to `<random>` Library
C++ provides a powerful and flexible library for random number generation through the `<random>` header. This library enables developers to create random numbers with specified distributions and offers more control compared to older C-style methods.
Reasons for Using the Standard Library Over Legacy Methods
Using the `<random>` library over legacy functions like `rand()` and `srand()` ensures:
- Better randomness quality
- More customizable distributions
- Flexibility and ease of use in complex applications
Components of the `<random>` Library
The `<random>` library consists of two main components: random number engines and distributions.
Random Number Engines
Random number engines are algorithms that generate sequences of numbers. Key engines include:
- `std::default_random_engine`: The standard random engine used in C++. It offers a good compromise between speed and quality.
- `std::mt19937`: A specific implementation of the Mersenne Twister engine known for its high quality and long period.
Example: Initializing and Using an Engine
To create a basic random number engine, you can use the following code:
#include <random>
std::default_random_engine engine; // Example engine
Distributions
Distributions define how the random numbers are spread or generated within a specific range. Common distributions include:
- Uniform Distribution: Generates numbers in a specific range where each has an equal chance.
- Normal Distribution: Generates numbers centered around a specific mean with a specified standard deviation.
Example: Generating Random Numbers Using Uniform Distribution
Here's how you can generate a random integer between 1 and 100:
std::uniform_int_distribution<int> dist(1, 100); // Range between 1 and 100
int random_number = dist(engine);
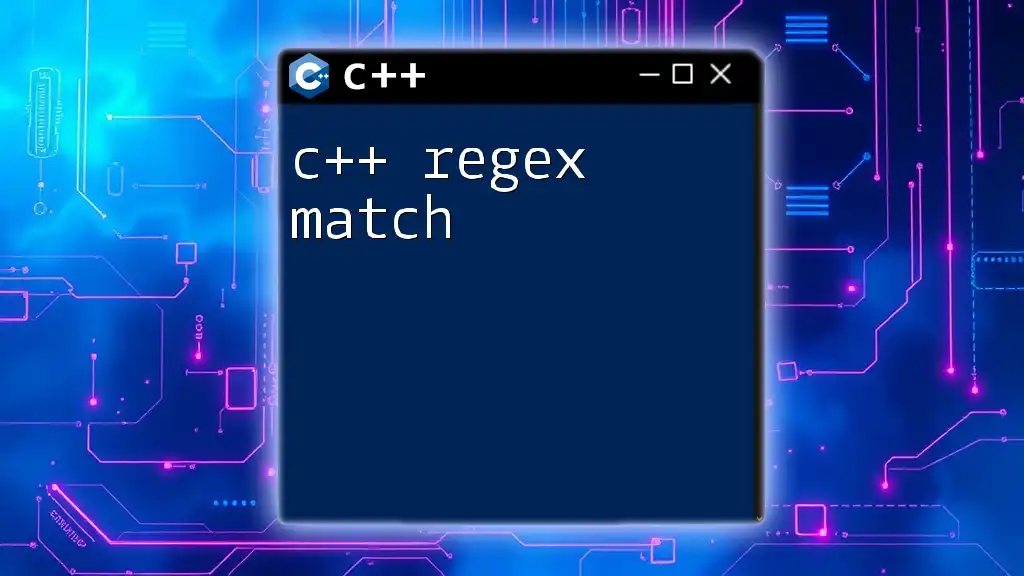
Setting Up a Random Number Generator in C++
Including the Necessary Headers
To leverage the features of the `<random>` library, ensure to include it at the top of your C++ program:
#include <random>
Creating and Seeding the Random Engine
Seeding the random engine is crucial for producing different sequences of results each time the program runs. A common practice is to seed it with the current time, ensuring that each run generates unique outcomes.
Example: Using the Current Time as a Seed
#include <chrono>
std::default_random_engine engine(static_cast<unsigned int>(std::chrono::system_clock::now().time_since_epoch().count()));
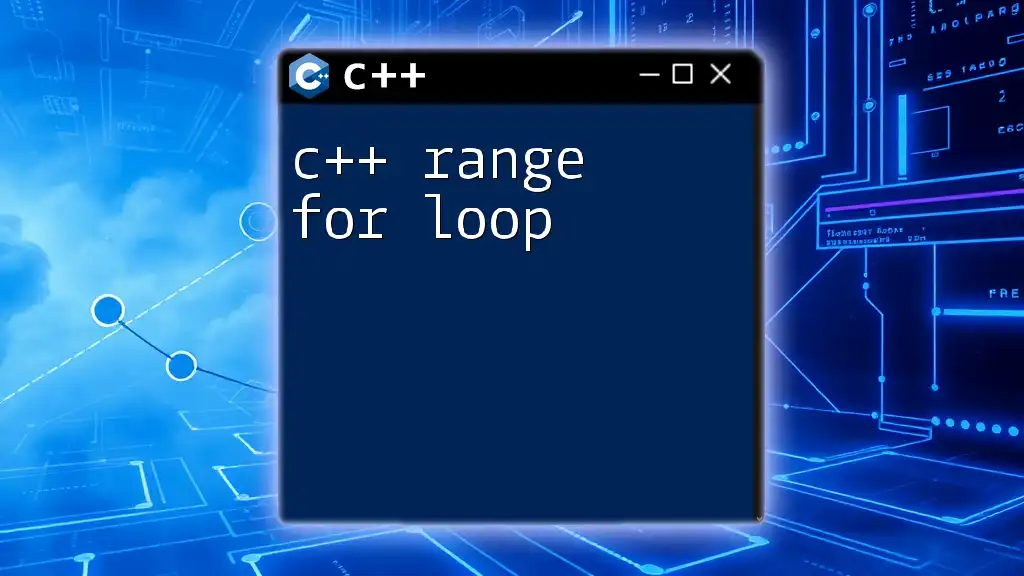
Practical Examples of C++ RNG
Example 1: Generating a Simple Random Integer
In this example, we will generate a random integer from 1 to 100:
#include <iostream>
#include <random>
int main() {
std::default_random_engine engine;
std::uniform_int_distribution<int> dist(1, 100);
std::cout << "Random Number: " << dist(engine) << std::endl;
return 0;
}
Example 2: Generating Random Floats
To generate a random floating-point number between 0 and 1, you can utilize the uniform real distribution as follows:
#include <iostream>
#include <random>
int main() {
std::default_random_engine engine;
std::uniform_real_distribution<double> dist(0.0, 1.0);
double random_float = dist(engine);
std::cout << "Random Float: " << random_float << std::endl;
return 0;
}
Example 3: Rolling a Die Simulator
Here’s a complete example that simulates rolling a six-sided die:
#include <iostream>
#include <random>
int main() {
std::default_random_engine engine;
std::uniform_int_distribution<int> dist(1, 6); // 6-sided die
std::cout << "Rolling a die: " << dist(engine) << std::endl;
return 0;
}
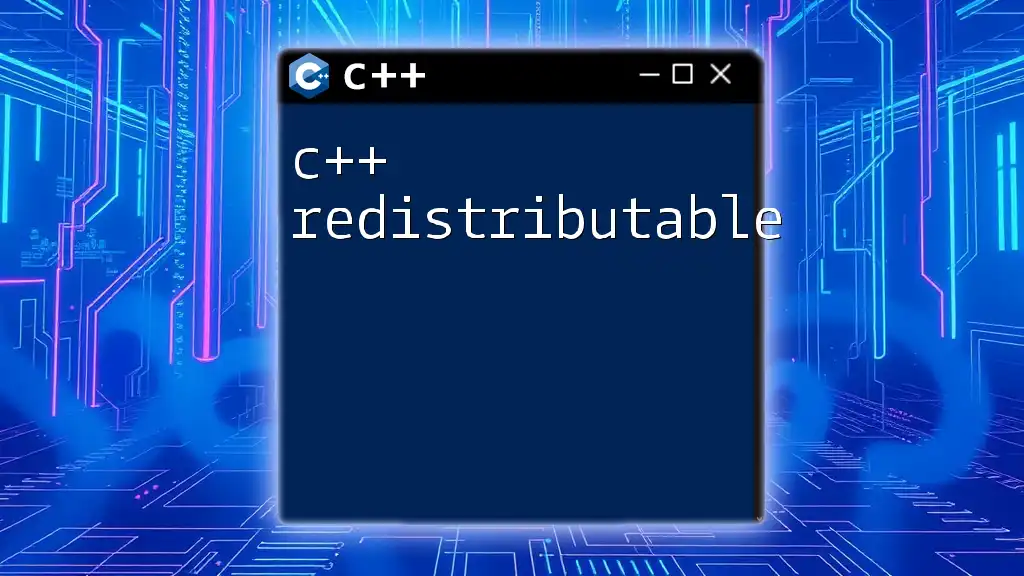
Advanced Concepts in Random Number Generation
Understanding Different Algorithms and Their Performance
The effectiveness of random number generation often relies on the algorithm applied. For example, the Mersenne Twister (MT19937) is widely favored due to its long period and high-quality output. On the other hand, simpler algorithms, like Linear Congruential Generators (LCGs), may produce quick results but might lack the necessary randomness quality for certain applications.
Thread-Safe Random Number Generation
In multithreaded applications, it’s essential to ensure that random number generation is thread-safe. Each thread should ideally have its own instance of the random engine to prevent data races and maintain the uniqueness of the generated numbers. A common practice is to use thread-local storage for engines.
Using Random Generators for Cryptography
In cryptographic applications, randomness quality is paramount. C++ does not directly provide a cryptographic RNG in the standard library, but you can integrate libraries like OpenSSL or the system's random sources to enhance security.
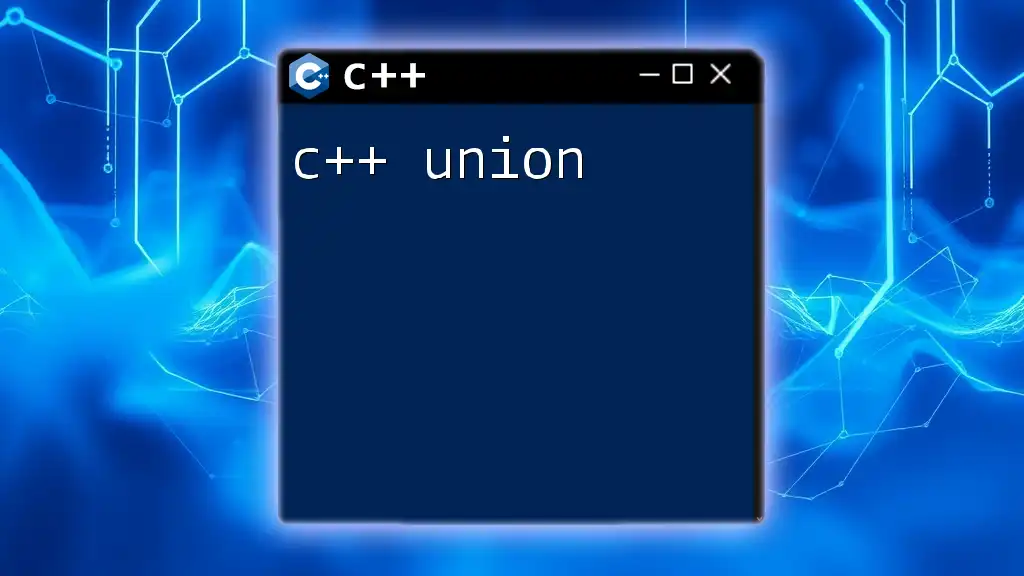
Best Practices for Using RNG in C++
Avoiding Repeating Patterns
To ensure the randomness quality of generated numbers, avoid the use of hard-coded seeds or patterns. Utilize a time-based seed for more variation in outcomes. In scenarios where controlled randomness is vital (e.g., testing), consider external entities like configuration files or user input.
Testing Randomness
Verifying the randomness of generated numbers can be done through statistical tests such as frequency tests or chi-square tests. These tests help ensure that the distribution of your random numbers behaves as expected and doesn't exhibit unwanted patterns.
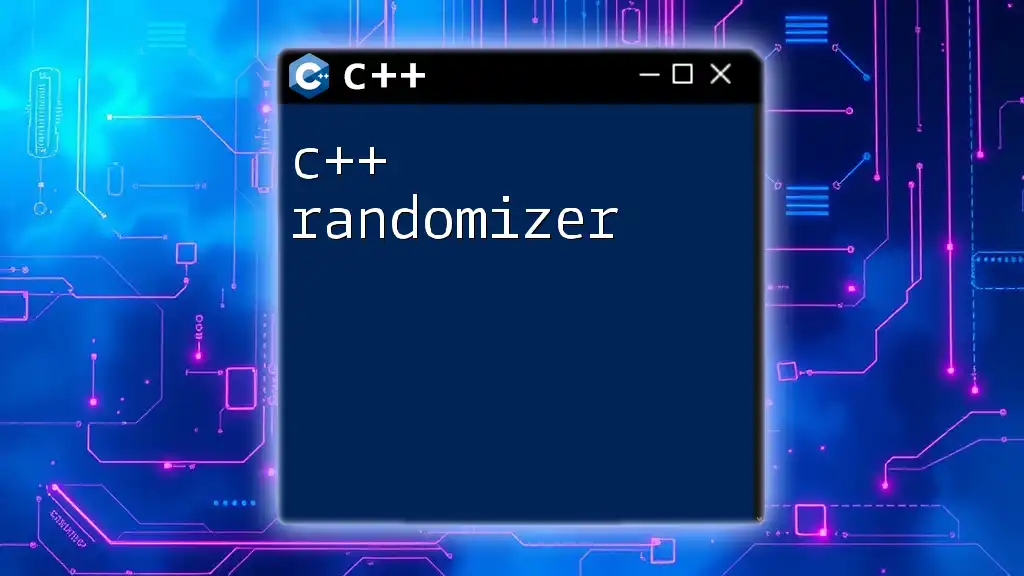
Conclusion
Understanding C++ RNG and utilizing the `<random>` library equips you with the tools necessary to create robust applications that require randomness. From simple simulations to complex gaming mechanics, mastering random number generation can significantly enhance your programming projects. Don't hesitate to dive deeper into the numerous features and techniques available, and implement them in your code to experience the full potential of what randomness can offer in programming!