To compile a C++ program in Linux, you can use the `g++` command followed by the source file name, like this:
g++ -o output_file source_file.cpp
Understanding C++ Compilation in Linux
What is Compilation?
Compilation is the process of converting source code written in a high-level programming language (like C++) into machine code that a computer's processor can execute. This process generally consists of several stages:
- Preprocessing: The compiler processes directives (like `#include`) and prepares the code for the next phase.
- Compilation: The compiler translates the preprocessed code into assembly language.
- Assembly: The assembler translates the assembly code into machine code (binary).
- Linking: The linker combines the object files generated in the assembly phase into a single executable program.
Why Use Linux for C++ Development?
Linux offers a robust environment for C++ development with several compelling advantages. Its stability and performance make it a favored choice among developers. Additionally, Linux provides a rich ecosystem of open-source tools and libraries that help streamline the development process.
The Linux community is vast and supportive, offering a wealth of resources, forums, and tutorials, making it easier for newcomers to learn and innovate. Understanding how to compile C++ in Linux is essential for leveraging these advantages.
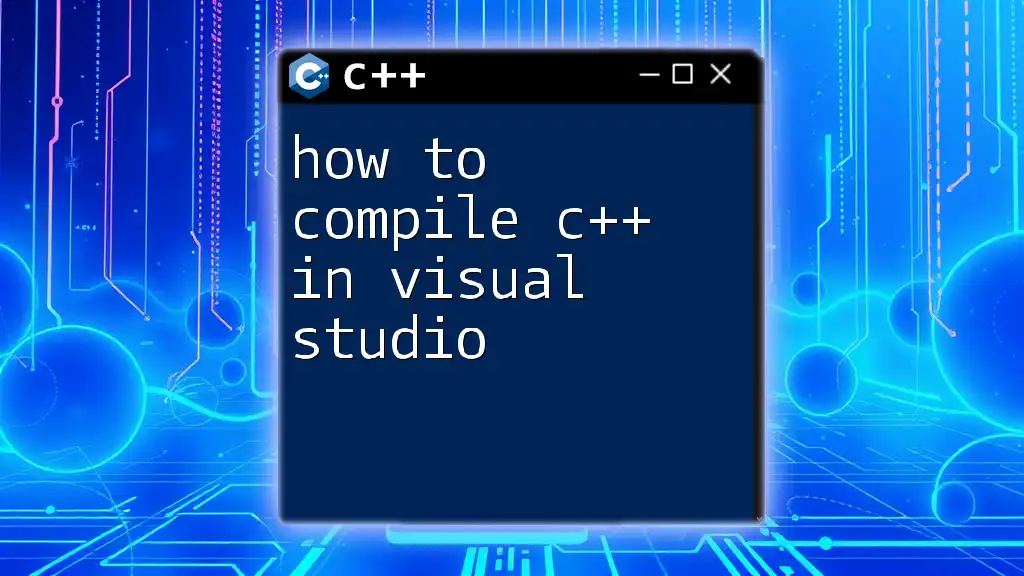
Setting Up Your C++ Compiler on Linux
Choosing a C++ Compiler
Selecting the right C++ compiler is a crucial step in your development journey. Some of the most popular C++ compilers for Linux include:
- GCC (GNU Compiler Collection): A widely used and highly versatile compiler that supports multiple programming languages, including C++.
- Clang: Known for its fast compilation times and high-quality error messages, making it user-friendly for beginners.
- Intel C++ Compiler: Optimized for Intel processors and delivers high-performance code, though it may require a commercial license.
Installing GCC on Linux
Installing the GCC compiler is typically straightforward, as it is available in the software repositories of most Linux distributions. Here’s how to install GCC on different distributions:
-
Debian/Ubuntu:
sudo apt install g++
-
Fedora:
sudo dnf install gcc-c++
-
Arch Linux:
sudo pacman -S gcc
Once installed, verify the installation by checking the version:
g++ --version
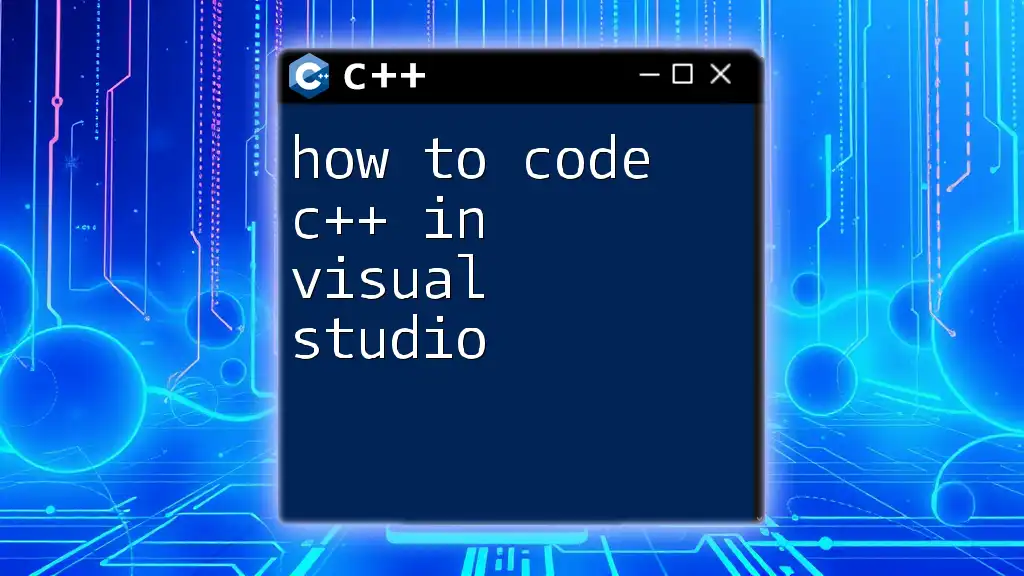
Compiling C++ Programs in Linux
Preparing Your C++ Source Code
Before diving into compilation, let's write a simple C++ program. This "Hello World" program will be our starting point:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Create a file named `hello.cpp` and paste the above code into it.
How to Compile C++ on Linux
Compiling your C++ program in Linux is as simple as executing a single command in the terminal. The basic command is:
g++ hello.cpp -o hello
In this command:
- `g++` is the C++ compiler.
- `hello.cpp` is the name of the source file you want to compile.
- `-o hello` specifies the name of the output executable file.
Running Your Compiled Program
After successfully compiling your program, you can run it by typing:
./hello
If everything went well, the output on your terminal will be:
Hello, World!
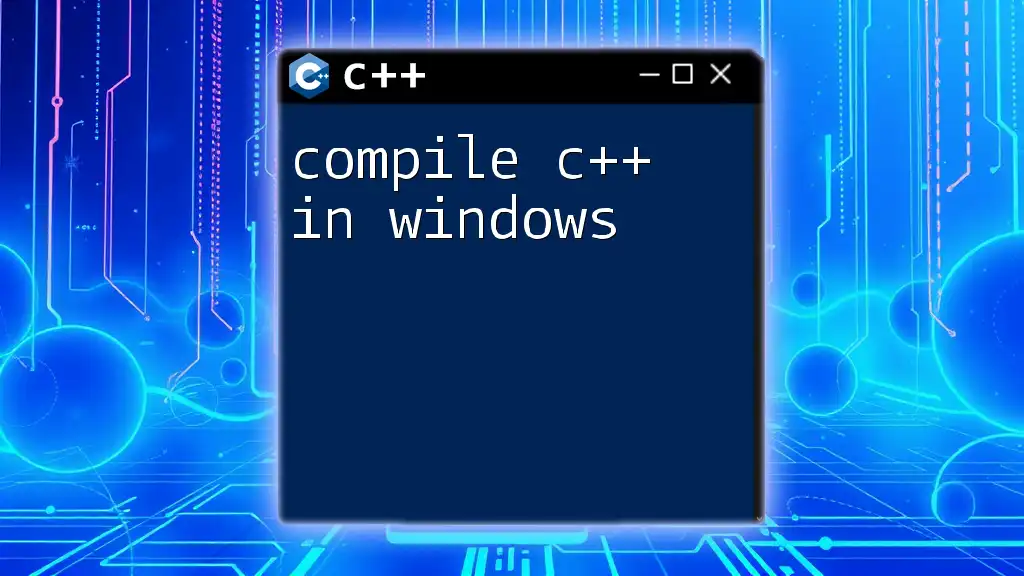
Advanced Compilation Techniques
Compiling Multiple Source Files
As your projects grow, you may find it necessary to split your code into multiple files for better organization. Compiling multiple source files is straightforward. For instance, if you have `file1.cpp` and `file2.cpp`, you would compile them as follows:
g++ file1.cpp file2.cpp -o output_program
This command compiles both source files and links them into a single executable named `output_program`.
Using Makefiles for Compilation
For larger projects with many source files, managing compilation manually can become tedious. This is where Makefiles come in handy. A Makefile automates the build process and handles dependencies.
Here’s a simple example of a Makefile:
all: main
main: file1.o file2.o
g++ file1.o file2.o -o main
%.o: %.cpp
g++ -c $< -o $@
In this Makefile:
- `all` is the default target.
- `main` is dependent on `file1.o` and `file2.o`, which are compiled object files derived from their corresponding source files.
Introduction to Compiler Flags
Commonly Used Compiler Flags
Compiler flags can modify the behavior of the compiler. Here are a few commonly used flags:
- `-Wall`: Enables all warning messages to catch potential issues early.
- `-O2`: Optimizes the code for better performance.
- `-g`: Includes debugging information for debugging tools like `gdb`.
You can combine these flags in a compilation command as follows:
g++ -Wall -O2 hello.cpp -o hello
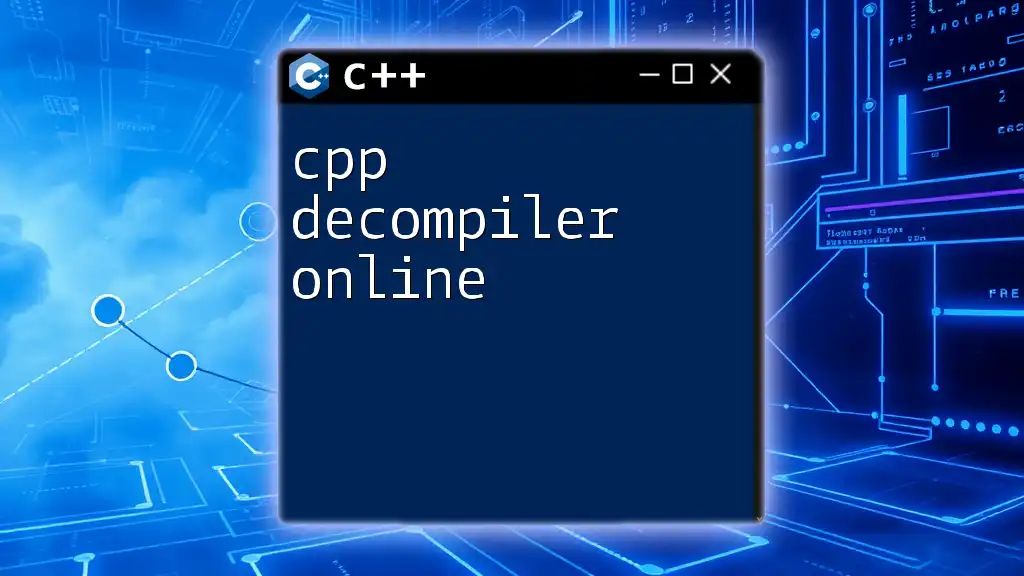
Troubleshooting Compilation Errors
Understanding Error Messages
When your code doesn’t compile, the compiler will provide error messages. Understanding these messages is essential for debugging. They generally indicate the type of error and the line number where it occurred. Common error types include:
- Syntax errors: Mistakes in the code that violate the programming language’s rules, e.g., missing semicolons.
- Linking errors: Occur when the compiler can’t find a function or variable definition.
Debugging Tips
If you encounter runtime errors or crashes after successful compilation, debugging is your next step. Using `gdb` (GNU Debugger) can help you dig into the problem. You start by compiling your code with debugging information:
g++ -g hello.cpp -o hello
Then, run the debugger:
gdb ./hello
From here, you can run your program, set breakpoints, and inspect variables to identify the source of the problem.
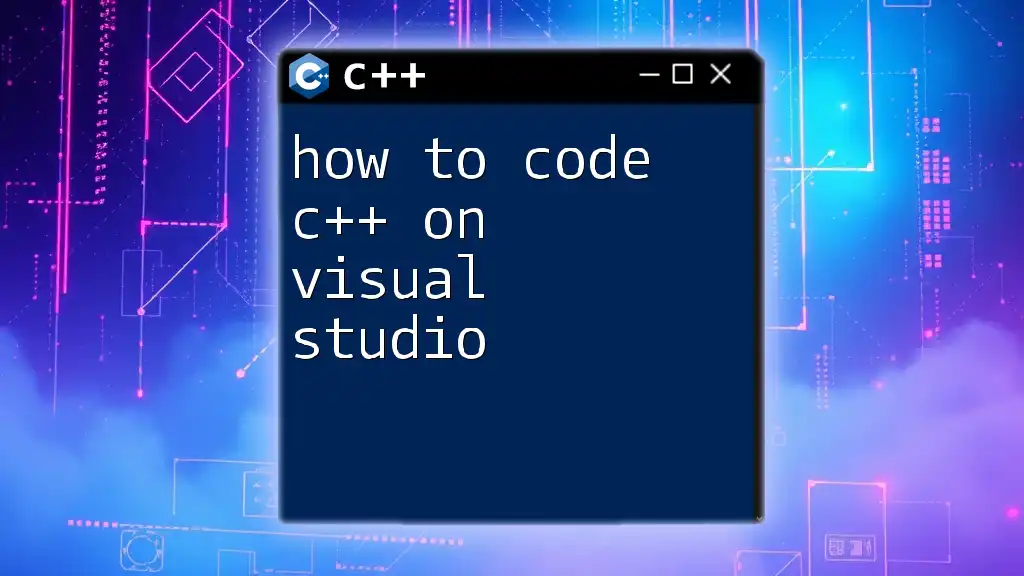
Conclusion
Knowing how to compile cpp in linux is a crucial skill for any developer working in C++. By mastering the compilation process, from writing simple programs to utilizing advanced techniques like Makefiles, you're setting the groundwork for success in programming with C++. Remember to embrace the debugging tools available, as they will only enhance your ability to write efficient, error-free code.
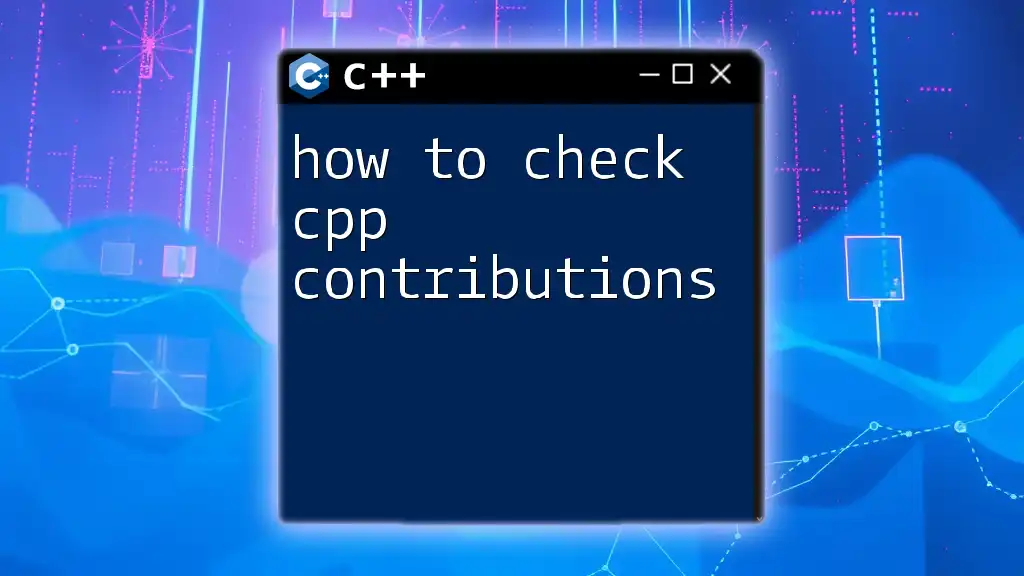
Call to Action
If you found this guide helpful, consider sharing it with others interested in learning C++. Join our community for more tutorials and resources on C++ and Linux programming!
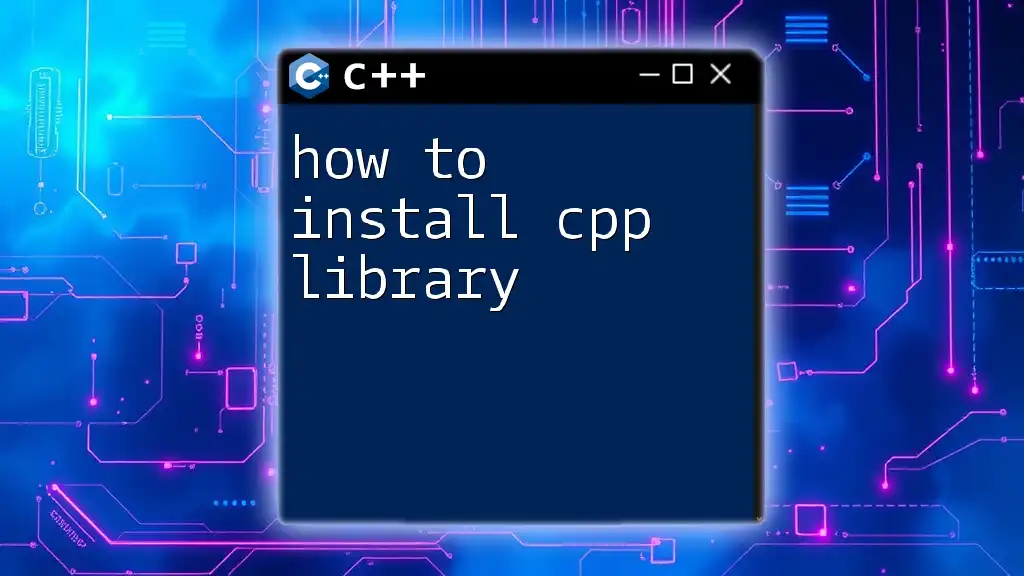
References
For further learning and deeper understanding of C++ compilation on Linux, consult the following resources:
- Official GCC documentation
- C++ programming tutorials
- Online forums and community support platforms