The `int min` function in C++ is typically used to return the smaller of two integer values provided as arguments. Here’s an example of how to use it:
#include <iostream>
#include <algorithm> // for std::min
int main() {
int a = 5, b = 10;
int minimum = std::min(a, b);
std::cout << "The minimum value is: " << minimum << std::endl;
return 0;
}
What is `int_min` in C++?
Definition of `int_min`
In C++, `int_min` refers to the minimum value that can be represented by an `int` data type. The C++ standard defines the sizes and ranges of various data types, and for a typical `int`, this usually equates to -2,147,483,648 for a 32-bit system, but this can vary depending on the system architecture.
An important distinction to make is between signed and unsigned integers. `int` is a signed type, meaning it can hold both negative and positive values, whereas an `unsigned int` cannot hold negative values and thus starts from 0. The same concept applies for `int_min`: it is only relevant to signed integers.
Where is `int_min` Used?
The `int_min` value is particularly significant in various programming contexts. For instance, validating user input often requires checking whether an integer falls within the acceptable range. When writing algorithms, especially in competitive programming or embedded systems, developers frequently need to check for boundary conditions to avoid overflow and underflow, which could lead to undefined behavior in applications.
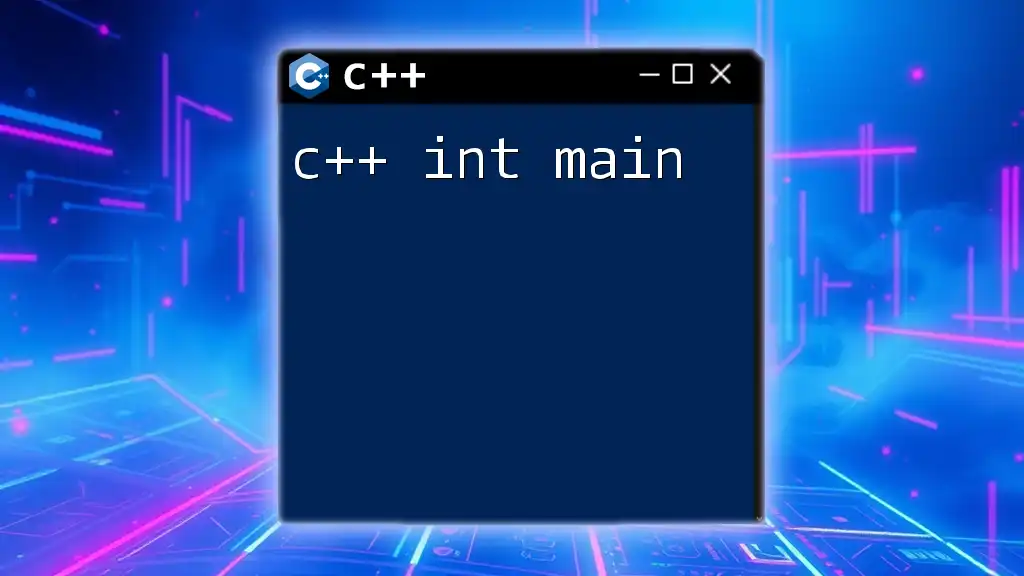
Understanding `int_max`
Definition of `int_max`
Alongside `int_min`, we also have `int_max`, which represents the maximum value that can be stored in an `int`. For a typical 32-bit `int`, this value usually stands at 2,147,483,647. Understanding both `int_max` and `int_min` is crucial for effective memory and data type management in C++.
Practical Applications of `int_max`
Understanding `int_max` is essential when performing operations that might exceed this threshold. For example, when incrementing an integer, if you surpass `int_max`, an overflow occurs, reverting the integer back to `int_min` under certain conditions. This can lead to logical errors in your code and result in unforeseen behaviors, making it imperative for developers to have a firm grasp of these integer limits.
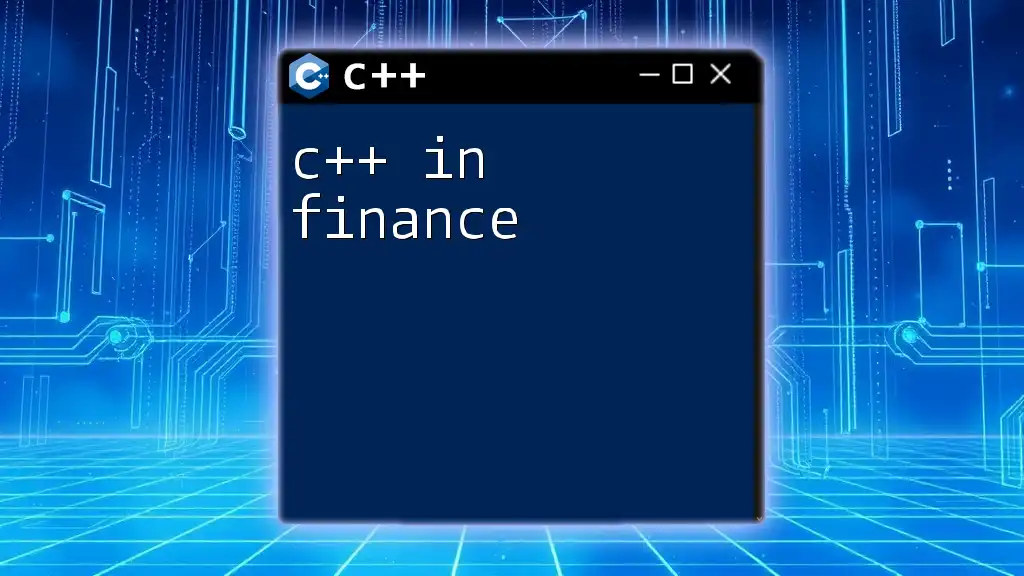
How to Access `int_min` and `int_max` in C++
Using `<limits>` Header
To access `int_min` and `int_max`, C++ programmers should include the `<limits>` header file. The `<limits>` library provides a standardized way to obtain information about the characteristics of arithmetic types.
Here is a simple code snippet to demonstrate how to access these values:
#include <limits>
#include <iostream>
int main() {
std::cout << "Minimum int value: " << std::numeric_limits<int>::min() << std::endl;
std::cout << "Maximum int value: " << std::numeric_limits<int>::max() << std::endl;
return 0;
}
Understanding `std::numeric_limits`
`std::numeric_limits` is a template class in C++ that provides a way to query the properties of fundamental data types. For instance, using `std::numeric_limits<int>::min()` retrieves the minimum value of an `int`, while `std::numeric_limits<int>::max()` returns its maximum counterpart. This capability is beneficial in writing generic code that can adapt to various underlying types without hardcoding limits.
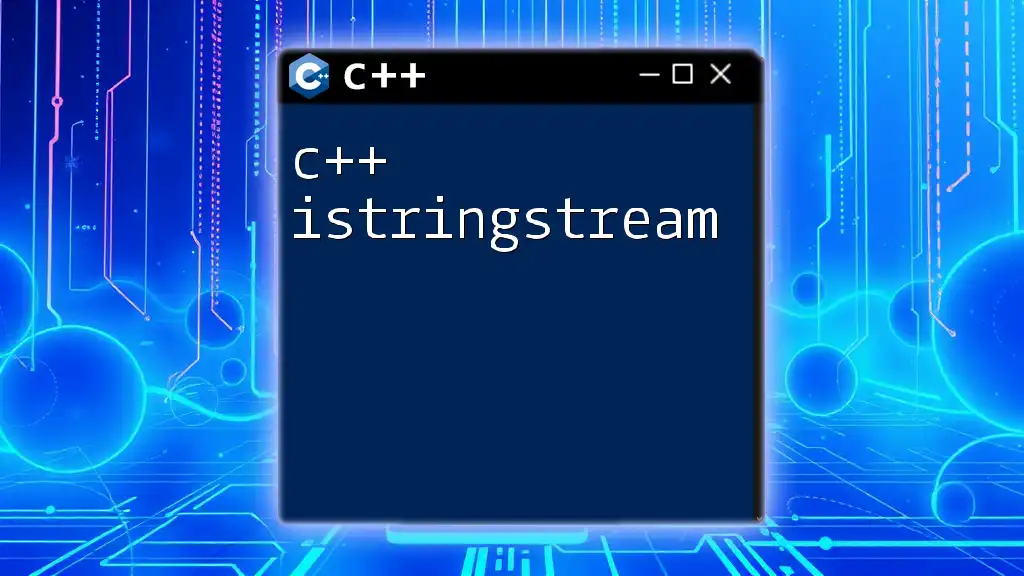
Practical Examples
Example 1: Basic Min/Max Usage
To better illustrate how to work with `int_min` and `int_max`, consider the following code example using C++:
#include <limits>
#include <iostream>
int main() {
int minValue = std::numeric_limits<int>::min();
int maxValue = std::numeric_limits<int>::max();
std::cout << "Min: " << minValue << ", Max: " << maxValue << std::endl;
return 0;
}
When executed, this will display both the minimum and maximum integer values that can be stored in an `int`, making it straightforward to see the range of this data type.
Example 2: Error Handling with `int_min` and `int_max`
Consider a situation where you want to validate user input to ensure it stays within the permissible bounds of an `int`. Here's how you could implement that in C++:
#include <limits>
#include <iostream>
int main() {
int value;
std::cout << "Enter an integer: ";
std::cin >> value;
if (value > std::numeric_limits<int>::max() || value < std::numeric_limits<int>::min()) {
std::cout << "Value is out of range!" << std::endl;
} else {
std::cout << "Value is accepted: " << value << std::endl;
}
return 0;
}
This code snippet prompts the user for an integer and then checks if it lies within the designated limit using `int_min` and `int_max`. If the input value is out of bounds, an error message is displayed, ensuring robust input validation.
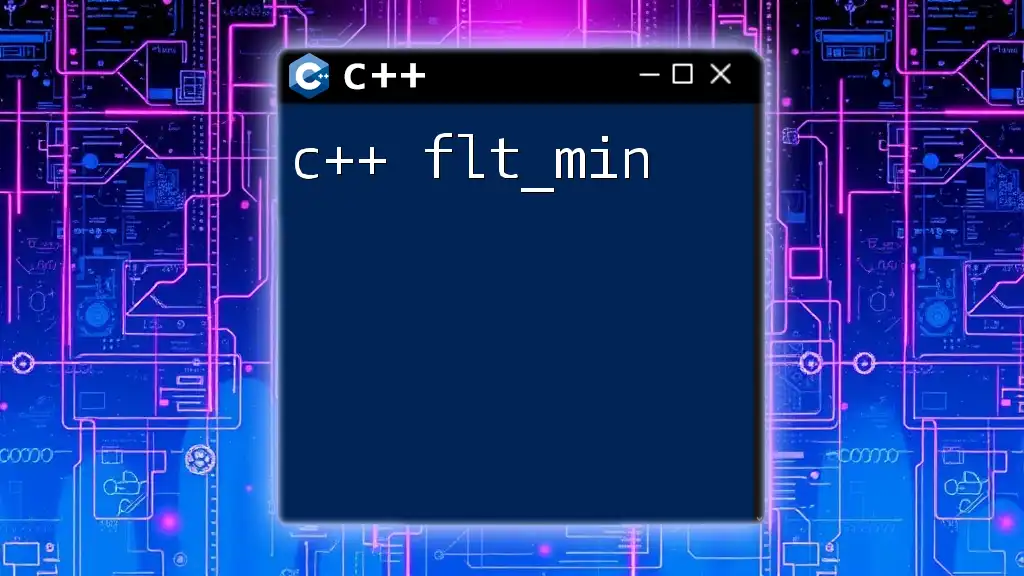
When Should You Use `int_min`?
Situational Considerations
You should consider using `int_min` in several scenarios:
-
Arithmetic Operations: Whenever you're performing calculations that could result in a value lower than `int_min`, you should implement checks to prevent underflows.
-
Input Validation and Sanitization: Always validate numeric input to make sure it fits within the acceptable range defined by `int_min` and `int_max`.
-
Conditional Statements: Utilize `int_min` for control flow when you want to determine if a value is valid based on minimum thresholds.
Common Mistakes to Avoid
Many developers overlook the boundary limits of integers, leading to common pitfalls such as:
-
Overflows and Underflows: This occurs when calculations exceed the defined minimum or maximum values, resulting in erratic behaviors.
-
Uninitialized Variables: If an integer variable is not initialized, it may default to a garbage value, which could unintentionally trigger conditions you didn’t foresee.
-
Ignoring Type Differences: Mixing signed and unsigned integers can lead to unexpected results; always ensures you are using the correct type during comparisons.
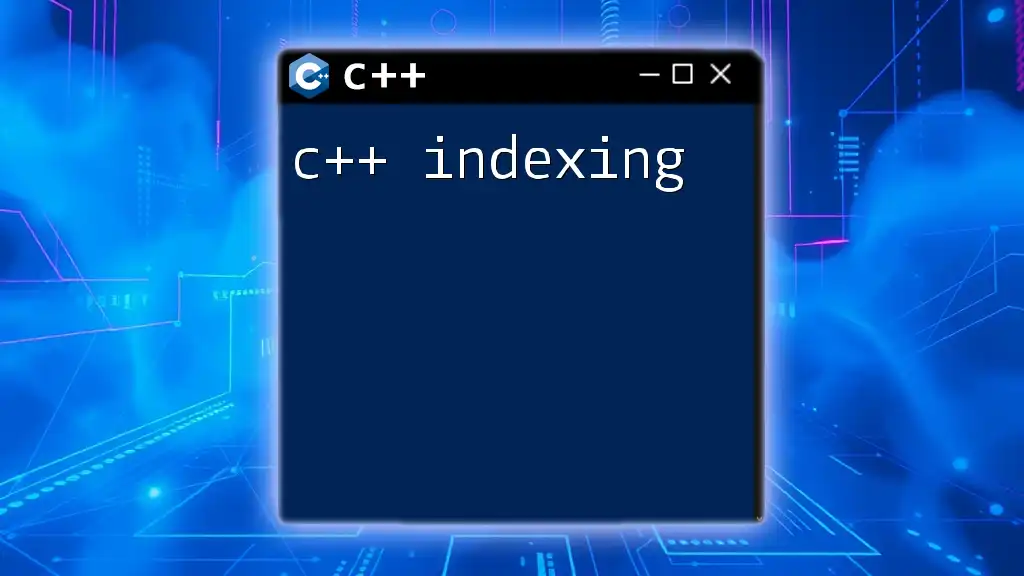
Conclusion
In summary, understanding `c++ int min` is crucial for effective programming. By leveraging `int_min` and `int_max` correctly, developers can safeguard against logic errors and ensure their applications behave predictably. Knowledge of these integer boundaries not only helps in writing robust code but also leads to better algorithm design and memory management. Emphasize practicing these concepts in real coding scenarios to solidify your understanding and improve your skill set as a C++ programmer.
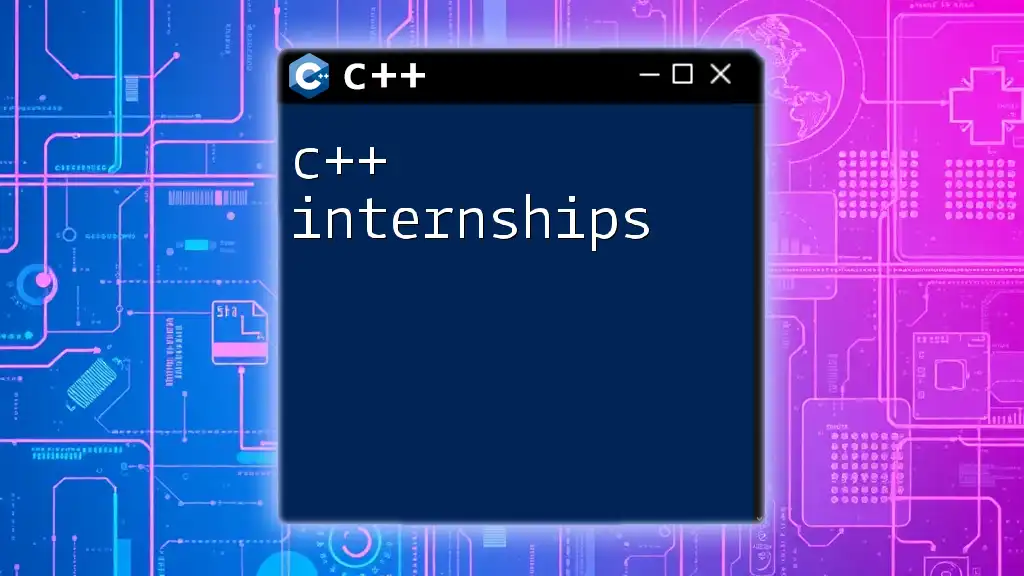
Additional Resources
For further reading, refer to official C++ documentation and explore recommended books and online resources dedicated to mastering C++ programming. Engaging with communities and forums can also provide valuable insights and answer questions as you continue to deepen your understanding.