C++ vectors provide dynamic array functionality with fast random access, while lists offer efficient insertion and deletion at the cost of slower access times.
#include <iostream>
#include <vector>
#include <list>
int main() {
// Using vector
std::vector<int> vec = {1, 2, 3};
vec.push_back(4);
// Using list
std::list<int> lst = {1, 2, 3};
lst.push_back(4);
// Accessing elements
std::cout << "Vector Element: " << vec[0] << std::endl;
auto it = lst.begin(); std::advance(it, 0);
std::cout << "List Element: " << *it << std::endl;
return 0;
}
Understanding C++ Containers
What are C++ Containers?
C++ containers are essential data structures that enable efficient storage and manipulation of data. They encapsulate data and provide member functions to manage that data, making them crucial for effective programming. C++ offers several types of containers, ranging from simple arrays to more complex structures like lists and vectors.
Types of C++ Containers
C++ containers can be categorized into three main types:
- Sequence Containers: Such as `vector`, `list`, and `deque`, allow data to be stored in a linear sequence.
- Associative Containers: Such as `set` and `map`, hold data in an organized way, allowing efficient searches.
- Unordered Associative Containers: Like `unordered_set` and `unordered_map`, maintain no specific order but offer improved performance for certain operations.
Understanding these categories aids in the selection of the right container for specific needs.
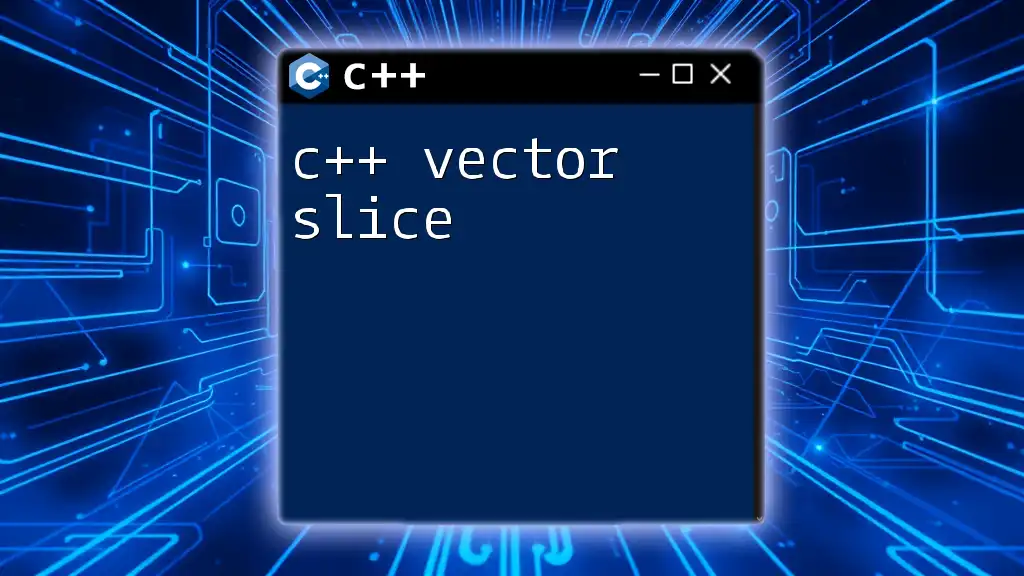
C++ Vector
What is a C++ Vector?
`std::vector` is a dynamic array that can change size during program execution. It is part of the Standard Template Library (STL), which allows developers to store collections of data efficiently. Vectors are characterized by their ability to provide fast random access due to their contiguous memory allocation.
Advantages of Using Vectors
Using vectors comes with several benefits:
- Performance: Vectors offer O(1) time complexity for access operations, making it very efficient to retrieve elements.
- Memory Management: They automatically manage memory, resizing as needed when elements are added or removed.
- Standard Library Integration: Vectors are compatible with various STL algorithms, enhancing their usability in conjunction with other functions.
Limitations of Vectors
Despite their advantages, vectors have some limitations:
- Inefficiency in Insertions and Deletions: Adding or removing elements, especially in the middle, can lead to O(n) complexity, as elements need to be shifted.
- Memory Overhead in Resizing Operations: When the vector exceeds its current capacity, a new memory block is allocated, and existing elements are copied, incurring additional overhead.
Example Code for Vectors
Here’s a simple implementation demonstrating basic vector operations:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.push_back(6); // Add an element
vec.erase(vec.begin() + 1); // Remove second element
for (int num : vec) {
std::cout << num << " ";
}
return 0;
}
This code initializes a vector with five integers, adds a new integer, and removes an existing one. It concludes by printing the elements in the vector.
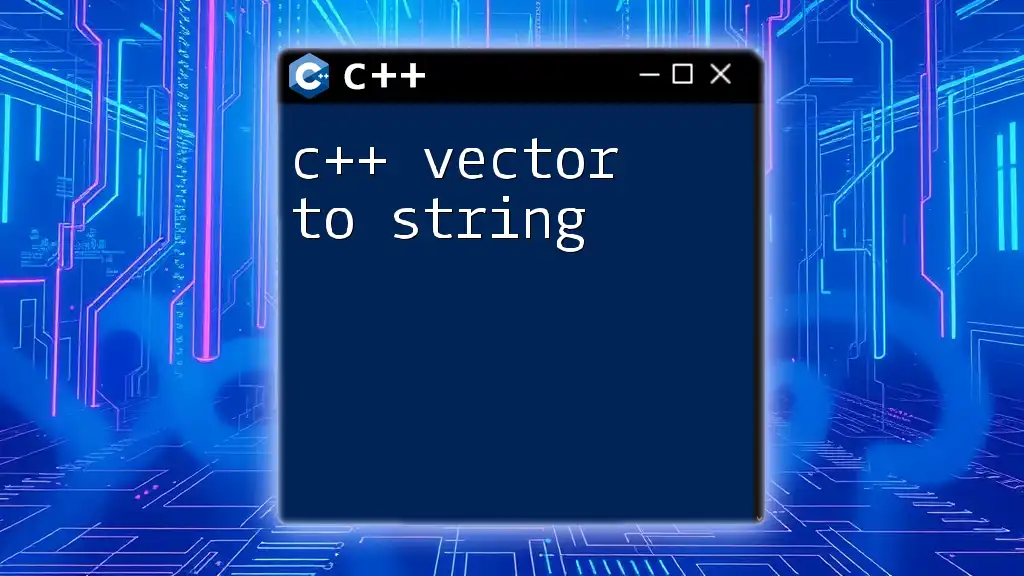
C++ List
What is a C++ List?
`std::list` represents a doubly linked list. Unlike vectors, lists store data elements in nodes, each containing a pointer to the next and previous nodes, allowing for efficient insertions and deletions.
Advantages of Using Lists
Lists provide unique advantages:
- Insertions/Deletions: Lists excel when adding or removing elements, as these operations are O(1) when the position is known, making them suitable for applications that require frequent modifications.
- Memory Efficiency: Lists do not require contiguous memory allocation, which can be beneficial in scenarios with unknown data sizes.
Limitations of Lists
However, lists have their drawbacks:
- Slower Random Access: Accessing an element in a list has a time complexity of O(n) since traversal is necessary.
- Increased Memory Usage: Each element requires additional memory for pointer storage, which may lead to higher overall memory consumption.
Example Code for Lists
The following code illustrates basic list operations:
#include <iostream>
#include <list>
int main() {
std::list<int> lst = {1, 2, 3, 4, 5};
lst.push_back(6); // Add an element
lst.remove(2); // Remove element with value 2
for (int num : lst) {
std::cout << num << " ";
}
return 0;
}
In this example, a list is initialized with five integers. A new integer is added, and an existing one is removed before displaying the remaining elements.
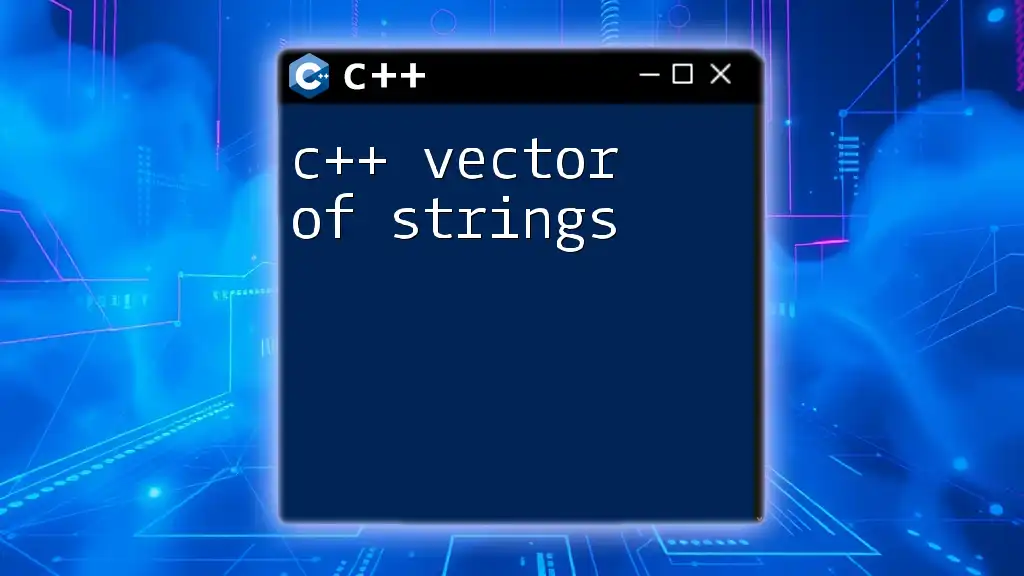
Key Differences: List vs Vector C++
Memory Allocation
A significant difference between C++ vector vs list is in their memory allocation strategies. Vectors allocate memory contiguously, providing fast access but potentially leading to copying overhead during resizing. Lists, on the other hand, allocate memory for each node separately, facilitating dynamic operations but increasing memory overhead due to additional pointers.
Performance Comparison
In terms of performance, vectors provide faster access times due to their contiguous nature, making them ideal for scenarios requiring frequent reading. Conversely, lists shine in situations where insertions and deletions occur frequently across various positions, as they are designed for efficient node manipulation.
Use Cases for Vectors and Lists
Vectors are optimal for applications needing constant-time access and a stable size, such as numeric computations and games with static object counts. Lists are preferred for data structures like queues and stacks, where rapid insertion and removal of elements are essential.
Choosing Between Vector and List
When selecting between `std::vector` and `std::list`, consider:
- Performance Needs: If your application demands fast access and minimal modifications, opt for a vector. If the application requires frequent insertions or deletions, a list is preferable.
- Memory Usage: Evaluate memory requirements. Vectors can lead to excessive overhead with large amounts of data changes, while lists may use more memory per element due to pointers.
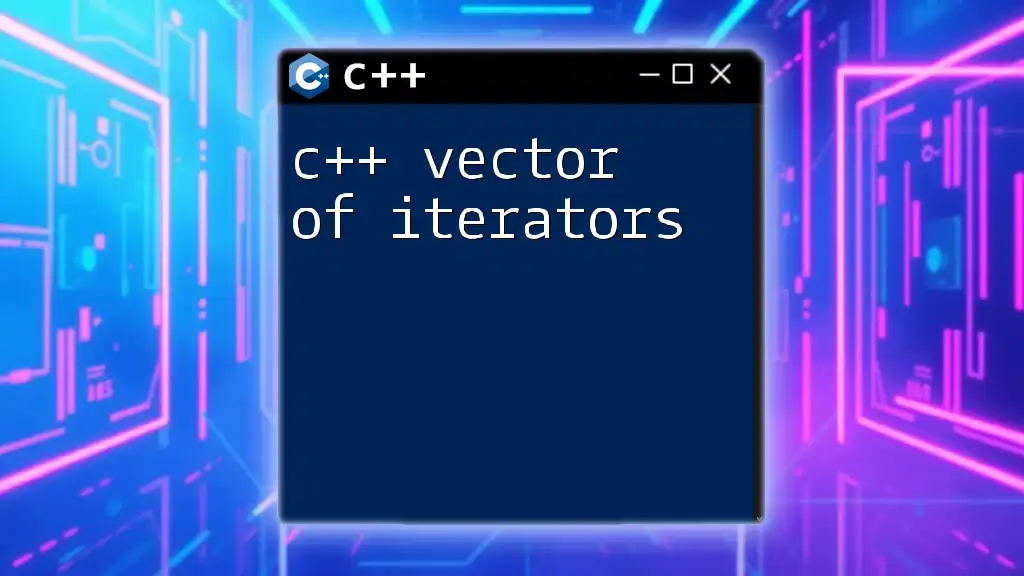
Conclusion
In the discussion of C++ vector vs list, it becomes clear that both containers have their respective strengths and weaknesses. Selecting the appropriate container hinges upon understanding their distinct properties, performance implications, and the specific needs of your application. Experimenting with both data structures can deepen your understanding, ultimately enhancing your programming skill set in C++.