While Rust offers modern features and memory safety that attract many developers, C++ remains deeply entrenched in legacy systems and performance-critical applications, making it unlikely to be fully replaced anytime soon.
Here's a simple code snippet that illustrates how both languages handle a basic task, such as defining a variable:
// C++ code
int main() {
int number = 10; // Define an integer variable
return 0;
}
// Rust code
fn main() {
let number = 10; // Define an integer variable
}
Understanding C++
The Evolution of C++
C++ has a rich history that dates back to the early 1980s, having emerged as an extension of the C programming language created by Bjarne Stroustrup. The goal was to combine the performance and efficiency of C with additional support for object-oriented programming. Over the years, C++ has undergone numerous changes and enhancements, including the introduction of templates, the Standard Template Library (STL), and features like exceptions and auto key types. These developments have solidified C++ as a versatile and powerful language in various domains, ranging from systems programming to high-performance applications.
Key Attributes of C++
C++ provides several key features that contribute to its longevity and popularity:
- Performance: As a compiled language, C++ typically offers unparalleled performance, making it ideal for system-level programming.
- System-level Access: C++ allows developers low-level memory manipulation, which is essential for writing operating systems or embedded systems.
- Object-oriented Programming (OOP): Its support for OOP facilitates code organization and reusability, which is crucial in large-scale applications.
Use Cases for C++
C++ is widely used across various industries due to its flexible nature and performance capabilities. Common use cases include:
- Game Development: Many game engines, like Unreal Engine, are built using C++ due to its speed and control over hardware.
- Embedded Systems: With its close-to-hardware capabilities, C++ is frequently used in embedded software.
- Financial Systems: Performance-critical applications requiring fast execution often rely on C++ for real-time trading platforms.
C++ remains a robust choice, especially in industries where performance is critical.
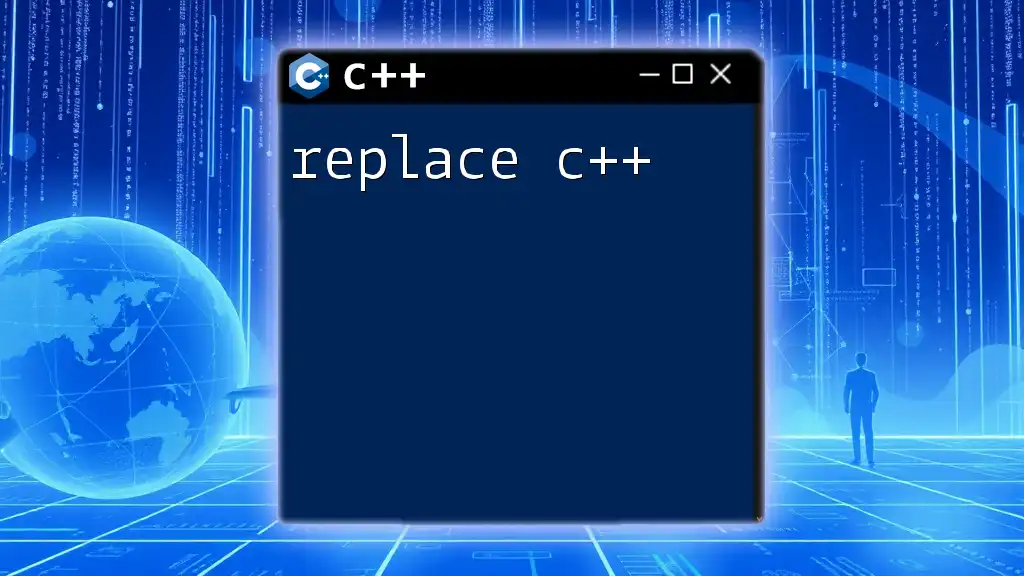
Understanding Rust
The Rise of Rust
Rust was developed by Mozilla, with its first stable release in 2015. Designed to provide safe concurrency and high performance, Rust addresses some common pitfalls found in C and C++. The language introduces a unique ownership model that ensures memory safety without the need for garbage collection.
Core Features of Rust
Rust is built around several defining principles:
- Ownership Model: This guarantees memory safety at compile time, thus preventing issues like dangling pointers or memory leaks.
- Concurrency: Rust’s design allows for safe concurrent programming, minimizing data races, which is a common problem in C++.
- Type Safety: Extensive compile-time checking helps catch a large number of potential errors before the code even runs.
Advantages of Rust
Rust has gained traction in recent years, thanks to several advantages over C++:
- Performance and Safety: Rust maintains performance levels comparable to C++, while eliminating entire classes of bugs related to memory safety.
// Rust
fn main() {
let arr = vec![1, 2, 3, 4, 5]; // Automatic memory management
println!("{:?}", arr);
}
- Focus on Concurrency: With its borrowing and ownership principles, Rust ensures that data cannot be accessed simultaneously in unsafe ways. This is vital for modern multi-threaded applications.
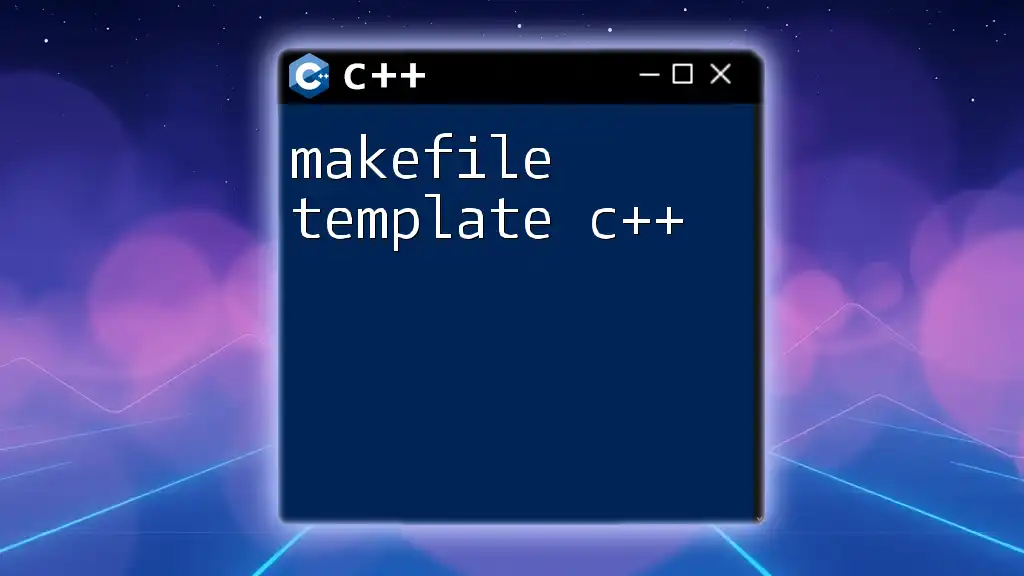
Comparing C++ and Rust
Direct Comparisons
One of the most significant areas of comparison between C++ and Rust is memory management.
In C++, memory management is manual and can often lead to errors if not handled correctly:
// C++
int* arr = new int[5]; // Manual management
delete[] arr; // Error-prone if not handled correctly
In contrast, Rust automates memory management through its ownership system, significantly reducing the chances of memory leaks and undefined behavior.
Another point of comparison is error handling. C++ relies on exceptions, which can lead to runtime errors if exceptions are not correctly managed. Rust uses a combination of `Result` and `Option` types for error handling, allowing for safer compile-time checks.
Community and Ecosystem
When comparing the ecosystems of C++ and Rust, both have unique strengths. C++ has been around for decades, leading to a vast number of libraries and frameworks like Boost and the STL. This ecosystem enables developers to leverage pre-existing solutions and support for nearly every conceivable application.
On the other hand, Rust has a rapidly growing ecosystem supported by its package manager, Cargo. While still maturing, Rust's libraries (or crates) offer innovative solutions for modern programming needs, such as asynchronous programming and web assembly development.
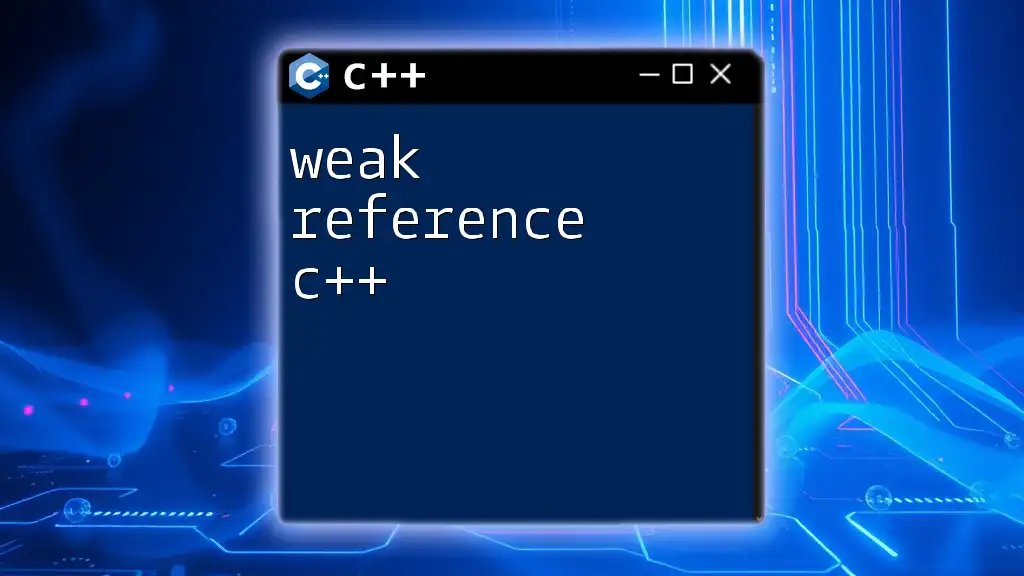
Challenges and Limitations
C++’s Challenges
Despite its strengths, C++ has its challenges:
-
Complexity and Learning Curve: Due to its vast feature set and intricacies, C++ can present a steep learning curve for new programmers. The multitude of features leads to challenges in writing clear, maintainable code.
-
Legacy Code and Maintenance: The existence of extensive legacy C++ codebases can create hurdles. Maintaining or upgrading these systems can be laborious and error-prone.
Rust’s Challenges
While Rust presents several advantages, it is not without its challenges:
-
Learning Curve: The concepts of ownership and borrowing can be challenging for newcomers. This complexity can deter some developers from adopting Rust.
-
Ecosystem Maturity: Although growing rapidly, Rust's ecosystem doesn't yet match the sheer volume of existing C++ libraries. This may limit Rust’s applicability in legacy systems or specific domains.
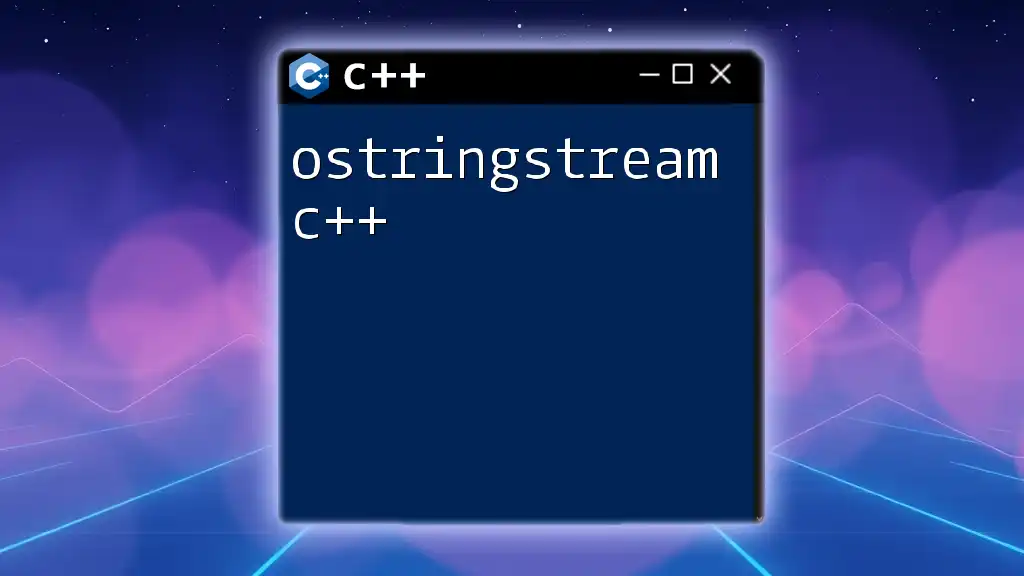
Future Prospects
Industry Adoption Trends
Rust is increasingly finding its place in modern software development, particularly in areas where safety and concurrency are paramount. Significant companies, including Microsoft and Google, have taken an interest in Rust for critical systems development. As the industry continues to prioritize security and reliability, Rust's appeal is likely to grow.
Potential for C++ and Rust Coexistence
Rather than a simple replacement, C++ and Rust may coexist, each serving distinct niches. C++ continues to dominate areas like game development and systems programming, while Rust is carving out a reputation for its safety features, especially in web server development and cloud applications.
Predictions for the Future
While the question remains, will Rust replace C++?, the answer might not be a clear yes or no. Instead, we could see Rust gradually becoming the language of choice for new projects, mainly where safety and concurrency are crucial, while C++ remains integral to existing systems and applications.
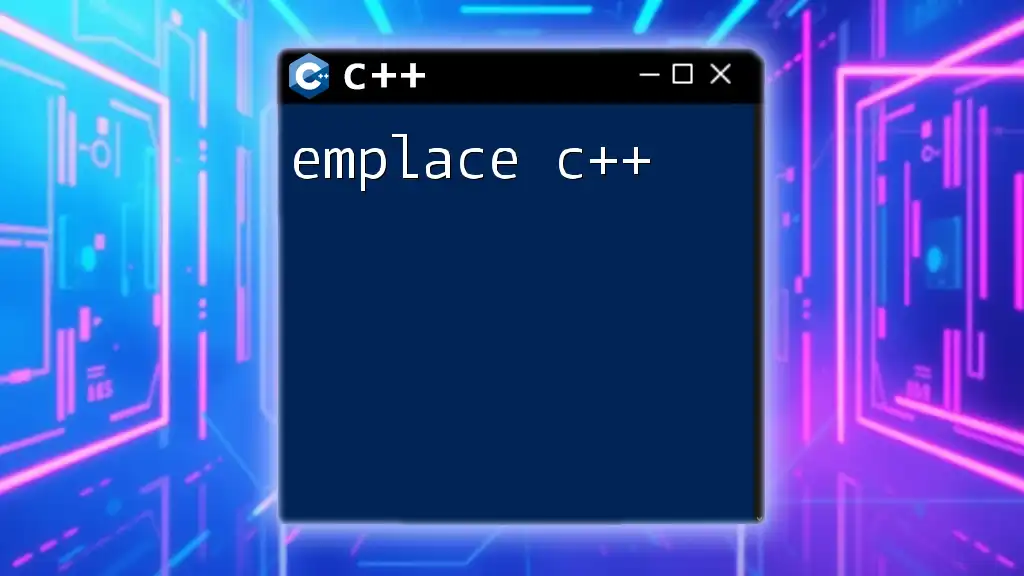
Conclusion
In summary, both C++ and Rust present formidable advantages and distinct challenges. C++ continues to thrive in performance-sensitive applications, while Rust offers a promising future in safe and concurrent programming. Although Rust has garnered significant attention, the communities around both languages offer essential insights and resources that developers can leverage. Whether one language will outright replace the other remains uncertain; however, their coexistence may very well define the future of software development.
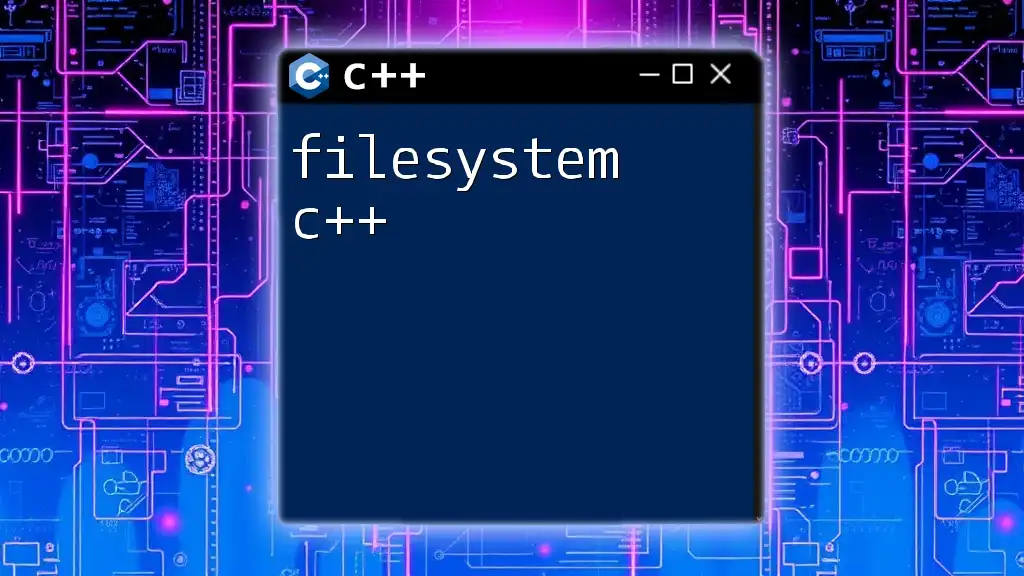
Call to Action
What are your thoughts on the rivalry between C++ and Rust? Join the conversation below and share your experiences! If you're looking to learn C++ commands in a quick and effective manner, don't hesitate to reach out. Explore both languages and expand your programming toolkit!
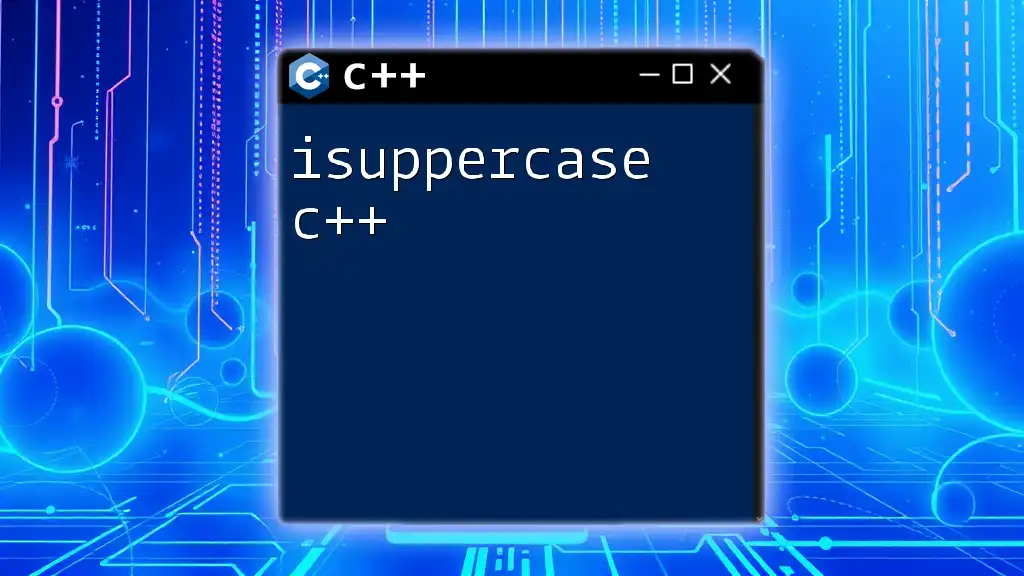
Additional Resources
Recommended Books
- "The C++ Programming Language" by Bjarne Stroustrup
- "The Rust Programming Language" by Steve Klabnik and Carol Nichols
Online Courses
- Coursera courses for both C++ and Rust programming
Community Links
- C++ and Rust forums, Discord channels, and other resources for networking and learning opportunities.
Engage with fellow developers and stay updated on both languages!