A C++ DLL (Dynamic Link Library) allows multiple applications to share the same code and resources efficiently at runtime, enabling modular application development.
Here's a simple example of a C++ DLL function:
// ExampleDLL.cpp
#include <windows.h>
extern "C" __declspec(dllexport) int Add(int a, int b) {
return a + b;
}
This code defines a function `Add` that sums two integers and can be used by other applications that link to the DLL.
What is a DLL?
A Dynamic Link Library (DLL) is a file that contains code and data that can be used by multiple programs simultaneously. Unlike static libraries, which are linked at compile time, DLLs are loaded at run time, allowing applications to share common code while reducing memory usage and storage requirements.
When using DLLs in C++, applications can leverage existing functionality without recompiling the entire codebase. This modular approach enhances maintainability and allows developers to update functions independently.
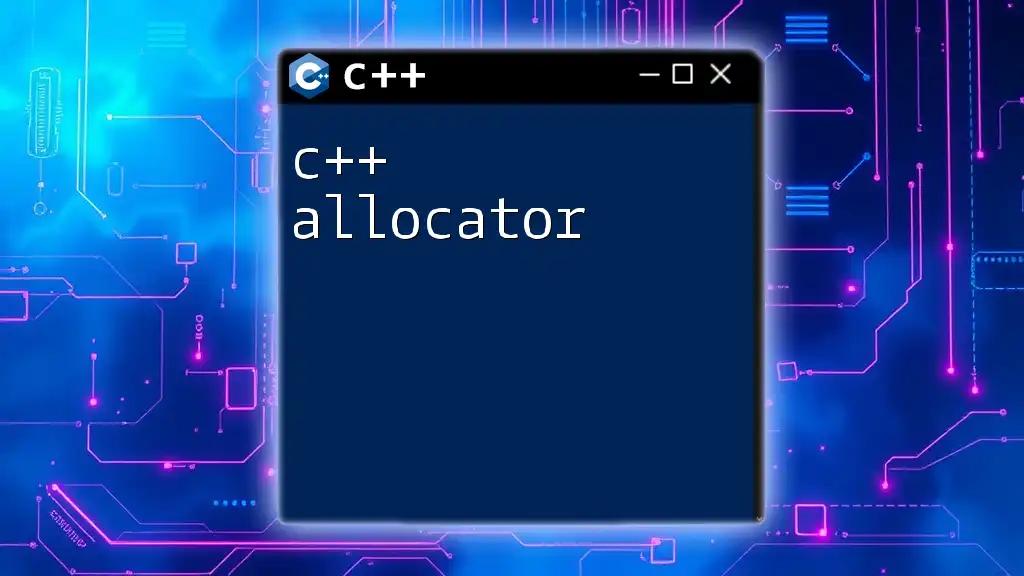
Benefits of Using DLLs in C++
Using DLLs in your C++ applications offers several advantages:
- Memory Management: DLLs enable applications to utilize shared memory, leading to less redundancy and improved memory management.
- Code Reuse and Modular Design: Developers can create reusable code components packaged as DLLs, promoting a modular architecture that enhances maintainability and readability.
- Versioning and Updates: Updating a DLL without recompiling dependent applications simplifies the process of distributing new features or bug fixes.
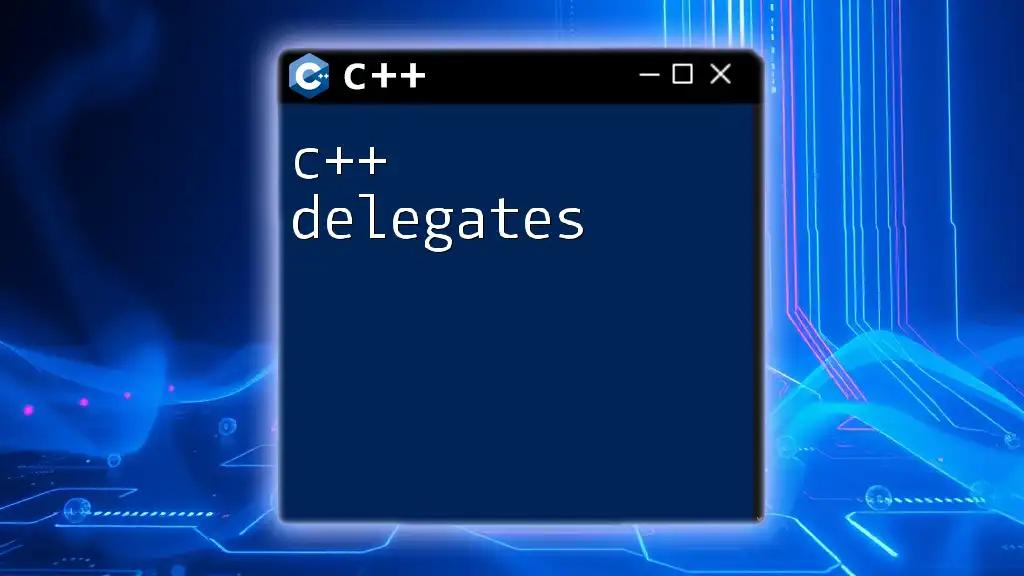
Key Concepts of DLLs
Understanding the core concepts of DLLs is essential for effective use:
Dynamic Linking vs. Static Linking
- Dynamic Linking: Functions are linked during execution, allowing for greater flexibility and shared code.
- Static Linking: Functions are compiled into the application, increasing size and reducing flexibility.
Exporting and Importing Functions
In a DLL, certain functions are designated for external use via exporting, while others are internal. This distinction facilitates clear interfaces and proper encapsulation.
How C++ Handles DLLs
C++ provides specific keywords to manage DLL exports and imports:
- `__declspec(dllexport)`: Used to indicate that a function or variable should be exported from the DLL.
- `__declspec(dllimport)`: Indicates that a function or variable is being imported from a DLL.
Additionally, developers must pay attention to calling conventions, which affect function signature alignment and parameter handling.
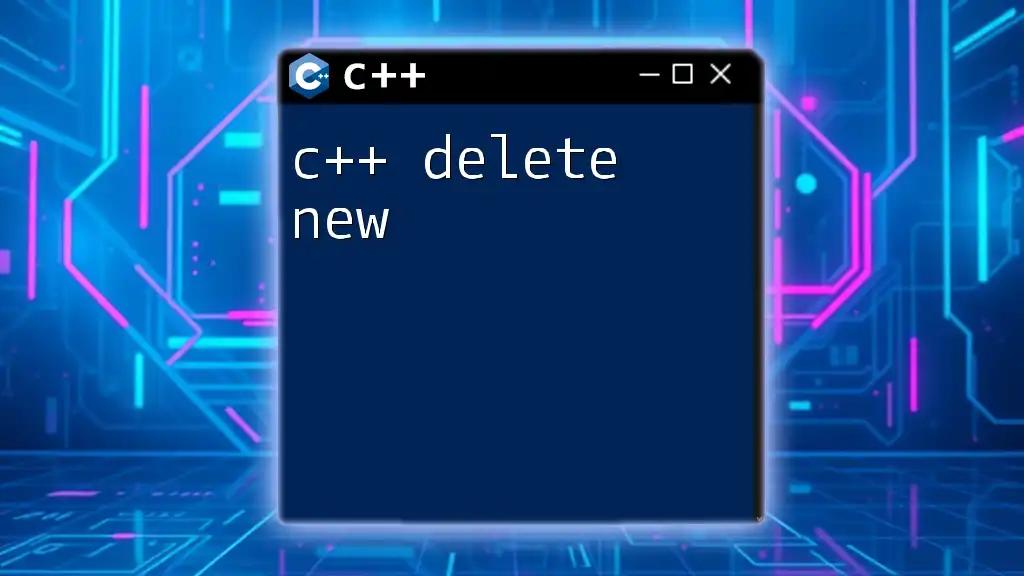
Creating a Simple C++ DLL
Setting Up Your Environment
Before creating a C++ DLL, ensure you have the necessary environment set up:
- IDE: A compatible IDE such as Visual Studio.
- Compilers: Ensure you have the required compilers appropriately configured.
Writing Your First DLL
Let’s create a simple "Hello World" DLL to illustrate the process.
- Create a new C++ project in your IDE and select the DLL option.
- Write the header file to define the function you’ll export.
Here's what your header might look like:
// HelloWorld.h
#ifdef HELLODLL_EXPORTS
#define HELLODLL_API __declspec(dllexport)
#else
#define HELLODLL_API __declspec(dllimport)
#endif
extern "C" HELLODLL_API void HelloWorld();
- Now, implement the function.
// HelloWorld.cpp
#include "HelloWorld.h"
#include <iostream>
extern "C" HELLODLL_API void HelloWorld() {
std::cout << "Hello, World from DLL!" << std::endl;
}
Compiling Your DLL
Compile your project in the IDE. This process will generate a DLL file and a corresponding LIB file for linkage.
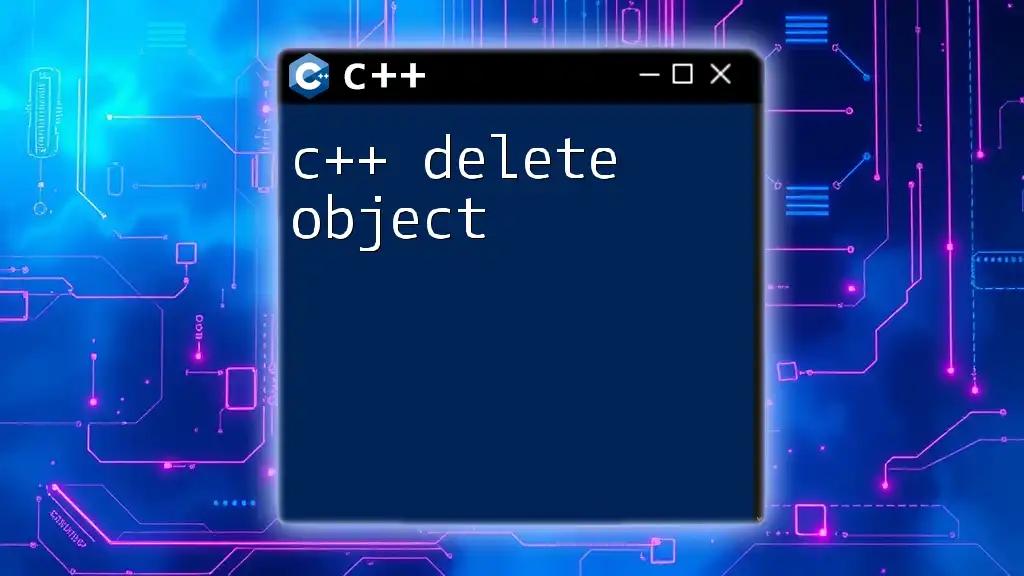
Using the C++ DLL
Creating a Client Application
With your DLL ready, it's time to create an application that uses it.
- Start a new C++ project and set it up as an executable.
- Link to the DLL by including the generated header file (`HelloWorld.h`) and linking against the LIB file produced during DLL creation.
Linking to the DLL
Here’s how you can utilize the exported function:
#include <iostream>
#include "HelloWorld.h" // Include your DLL's header
int main() {
HelloWorld(); // Call function from the DLL
return 0;
}
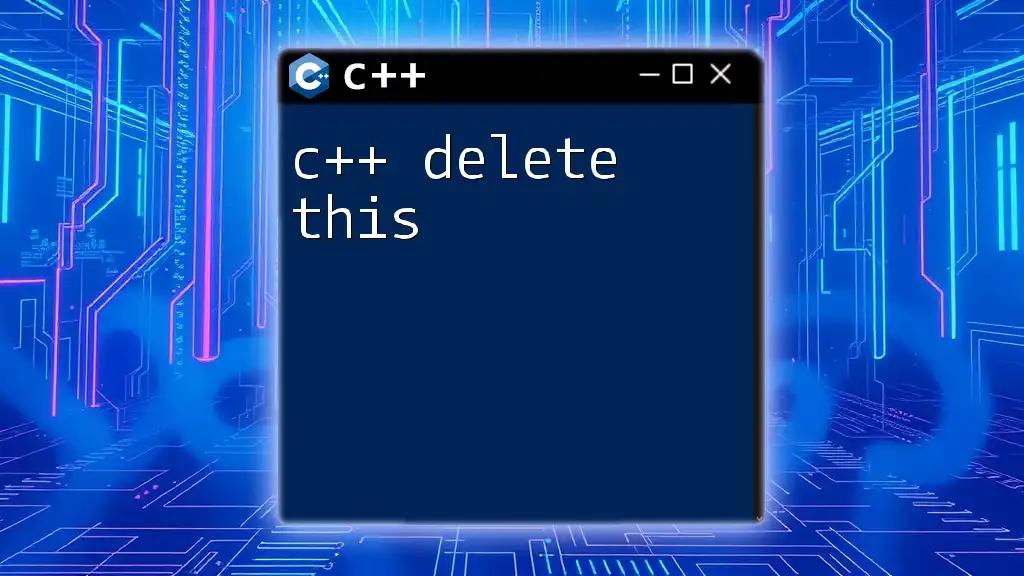
Advanced DLL Concepts
Versioning and Side-by-Side Assemblies
Versioning is crucial for managing updates to DLLs. Implement side-by-side assemblies to allow different versions of the same DLL to exist and be used by various applications without conflict. This practice enhances compatibility and stability in Windows environments.
Debugging C++ DLLs
Debugging DLLs can be challenging but essential for troubleshooting issues. Common techniques include:
- Debugging symbols: Always ensure debugging symbols are generated during compilation for better clarity during analysis.
- Adapt logging: Integrate logging to capture function calls and data flow, assisting in pinpointing issues.
Common Pitfalls and How to Avoid Them
- Mismatched Calling Conventions: Ensure that both the DLL and the client application share the same calling conventions to avoid runtime errors.
- Resource Management: Handle memory and resource allocation carefully across DLL boundaries to prevent leaks and crashes.
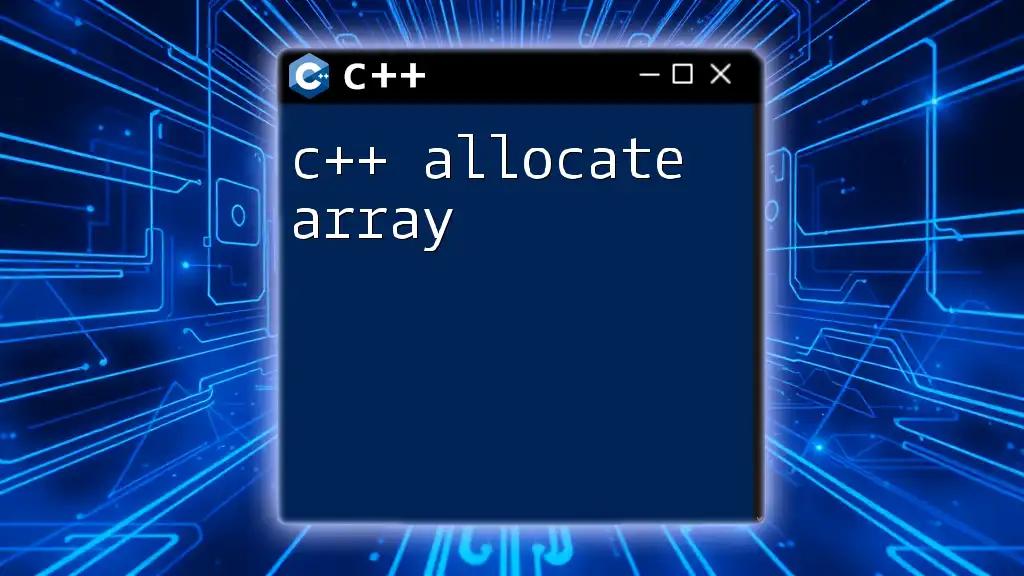
Best Practices for C++ DLL Development
Design Considerations
Design your DLL with a clean interface in mind. This practice not only fosters good encapsulation but also promotes ease of use and comprehension for those who will interact with your DLL.
Security Concerns
Security is paramount when developing DLLs. Implement these best practices:
- Input Validation: Always validate input to prevent exploitation.
- Avoiding Common Vulnerabilities: Be aware of DLL injection attacks and take preventive measures.
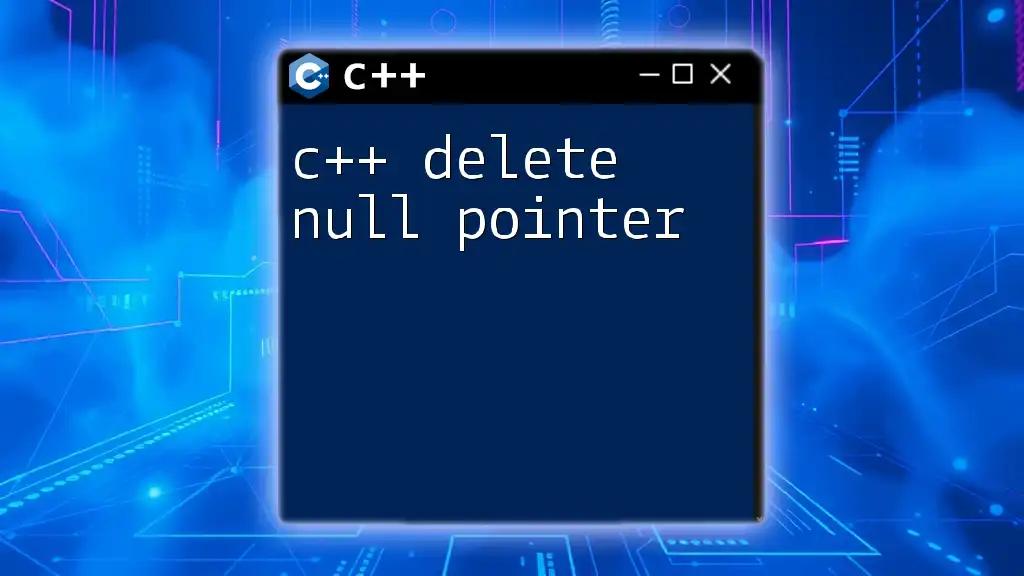
Conclusion
Mastering the principles and practices of C++ DLL development is essential for creating efficient and maintainable applications. By leveraging the advantages of dynamic linking, you can significantly enhance your coding prowess.
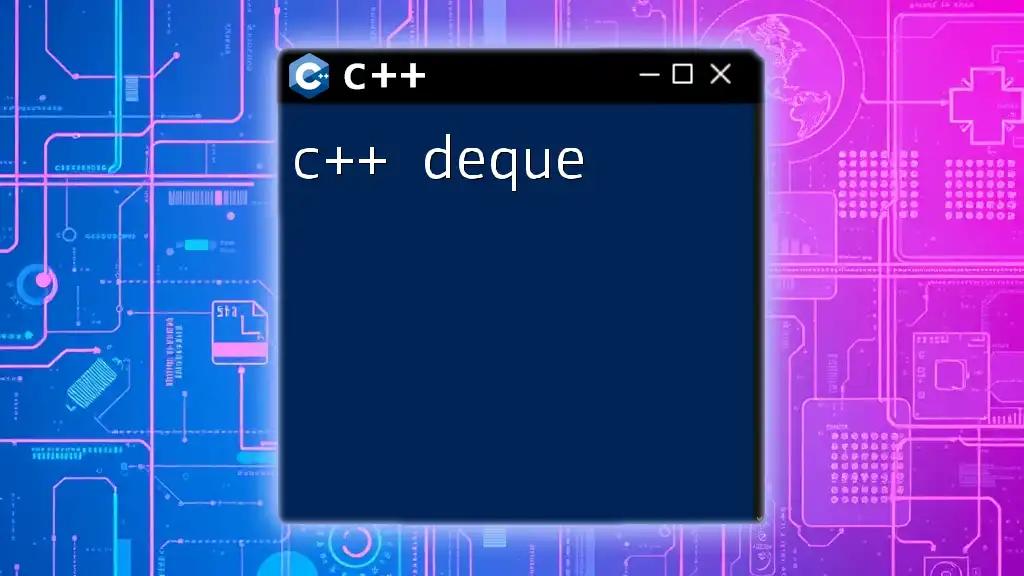
Further Resources and Learning Paths
For further study, consider exploring books on C++ programming, online tutorials specific to DLL development, and courses that delve into software design methodologies.
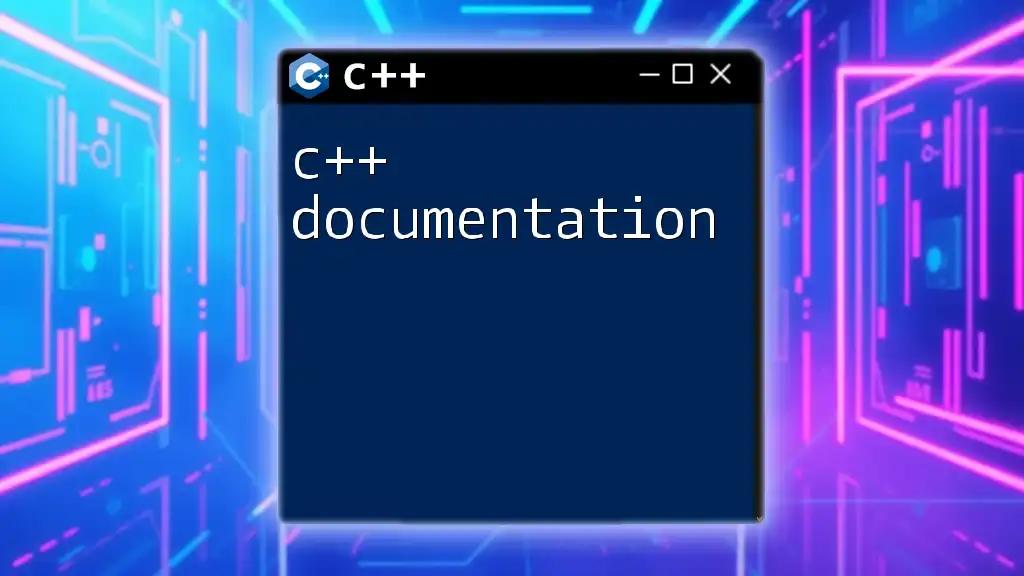
Frequently Asked Questions (FAQ)
What are some common uses for C++ DLLs?
C++ DLLs are used in various scenarios, including game development, database management systems, and as plugins in software applications, facilitating extensibility without major overhauls.
Can DLLs be used cross-platform?
While DLLs are traditionally a Windows concept, similar dynamic linking libraries exist on other platforms, such as `.so` files on Unix-like systems. However, portability requires careful consideration of function signatures and compile settings.
How to manage memory across DLL boundaries?
Memory management across DLL boundaries requires strict adherence to allocate/deallocate conventions. Ensure consistent use of functions like `new` and `delete` or `malloc` and `free` across boundaries to prevent resource leaks and crashes.