Lakos' large scale C++ approach promotes the use of modular programming and encapsulation to manage complexity in large C++ applications effectively.
Here’s a simple code snippet demonstrating the basic structure of a modular C++ program:
// Main.cpp
#include "MyModule.h"
int main() {
MyModule module;
module.performTask();
return 0;
}
// MyModule.h
#ifndef MY_MODULE_H
#define MY_MODULE_H
class MyModule {
public:
void performTask();
};
#endif
// MyModule.cpp
#include "MyModule.h"
#include <iostream>
void MyModule::performTask() {
std::cout << "Task performed!" << std::endl;
}
Understanding Large Scale C++
What is Large Scale C++?
Large Scale C++ refers to the approach and practices associated with developing robust and maintainable software systems written in C++. Traditional programming techniques often become unwieldy as software scales. Without careful management, larger systems can become difficult to understand, maintain, or even operate. Large Scale C++ tackles these challenges by emphasizing structured design, clear organization, and modular architecture.
The Philosophy Behind Large Scale C++
The philosophy underpinning Lakos Large Scale C++ is centered around several fundamental design principles aimed at boosting maintainability and readability. These principles advocate for a modular approach, where the software is divided into separate, well-defined modules or components that can be developed, tested, and maintained independently. This not only enhances clarity but also minimizes interdependencies, which are critical in large-scale systems.
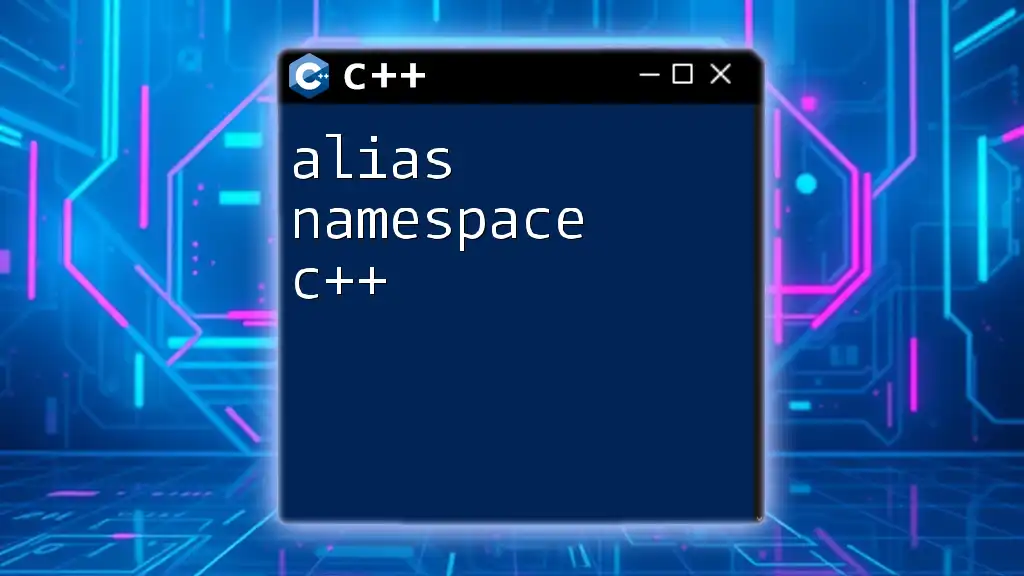
Key Concepts in Lakos Large Scale C++ Software Design
Module-Based Design
In Large Scale C++, modules are defined as self-contained sections of code that encapsulate functionality and hide internal details. This modular design offers several notable benefits:
- Maintainability: Changes in one module do not ripple through other parts of the application.
- Reusability: Modules can be reused across different projects without modification.
- Clarity: Modules can be designed with specific purposes, making codebases easier to navigate.
For instance, consider the following simple module setup for a basic arithmetic operation in C++:
// Example of a basic module structure
module math_operations; // defines a module
export int add(int a, int b) {
return a + b;
}
Dependencies and Coupling
In the context of large-scale software design, coupling refers to how closely connected different modules are. High coupling can lead to tangled dependencies, making systems fragile and hard to maintain. Therefore, reducing coupling is of paramount importance.
To minimize coupling, you can:
- Use interfaces or abstract classes.
- Apply the Dependency Injection pattern, which passes dependencies externally, allowing easy replacements and testing.
Here's an example of decoupling components in a project through forward declaration:
// Decoupling example
class Database; // Forward declaration for decoupling
class UserService {
private:
Database* db;
public:
UserService(Database* database) : db(database) {}
};
Code Organization and Structure
File Structure
Organizing files properly in large projects is critical. A clear file structure aids developers in quickly finding components and understanding their connections. A recommended file structure could be:
/project_root
/src
/modules
/math_operations
math_operations.cpp
math_operations.h
/user_service
user_service.cpp
user_service.h
/test
/include
Naming Conventions
Consistent naming conventions enhance clarity and help in maintaining code. Adopting a systematic approach, such as using camelCase for variable names and PascalCase for class names, can save time spent deciphering code.
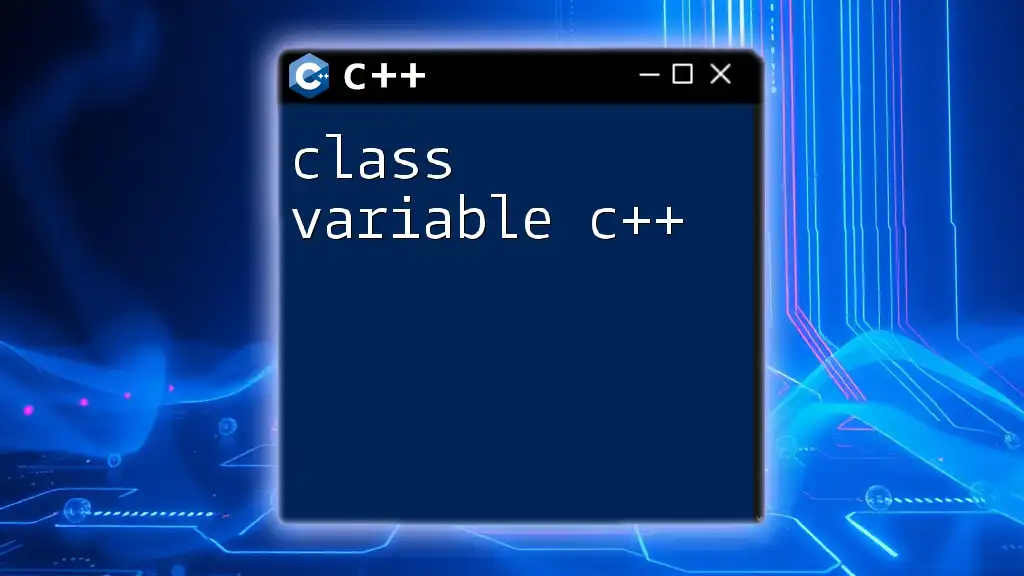
Advanced Topics in Lakos Large Scale C++
Effective Use of Templates
Templates in C++ allow for writing generic and reusable code. This is especially beneficial in large-scale software where similar functionalities may be applied across different data types. For example:
// Template example
template<typename T>
T add(T a, T b) {
return a + b;
}
This approach helps in reducing redundancy and enhancing the maintainability of the code.
Exception Handling in Large Scale Applications
Robust error handling is essential in large-scale systems. Exceptions should be managed via consistent and systematic approaches, ensuring that error states are handled efficiently to avoid application crashes.
Here’s a basic example of an error handling strategy:
try {
// code that may throw
} catch (const std::exception &e) {
// handle exception
std::cerr << "An error occurred: " << e.what() << std::endl;
}
Performance Considerations
Performance is a vital aspect of large scale applications. As complexity increases, so too can the performance hits. Important techniques for optimizing performance include:
- Minimizing memory allocations.
- Avoiding unnecessary copies (using move semantics or references).
- Profiling the application to identify bottlenecks.
By keeping performance in mind throughout the development process, you can ensure that your large-scale applications run smoothly and efficiently.
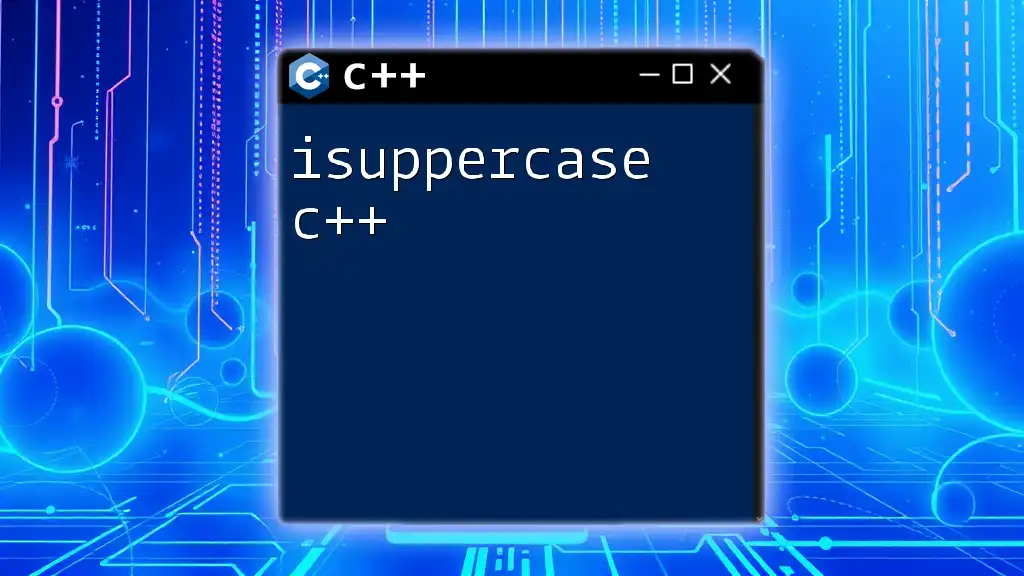
Best Practices for Lakos Large Scale C++ Development
Code Review and Collaboration
Code reviews are essential in maintaining high code quality, especially in large projects with multiple contributors. Implementing automated tools such as GitHub's pull request mechanism can streamline this process, ensuring that every piece of code is reviewed before being merged.
Testing Strategies
In large scale software design, testing is paramount to ensure functionality and reliability. Employing unit testing frameworks, such as Google Test or Catch2, can simplify the process of writing tests.
Here’s a simple unit test example using Google Test:
// Simple unit test example
#include <gtest/gtest.h>
TEST(MathOperations, Add) {
EXPECT_EQ(add(1, 2), 3);
}
Continuous Integration/Continuous Deployment (CI/CD)
Integrating CI/CD into your development workflow is crucial for quickly identifying issues and ensuring code quality. CI/CD pipelines can automate testing and deployment processes, making it easier to manage large applications.
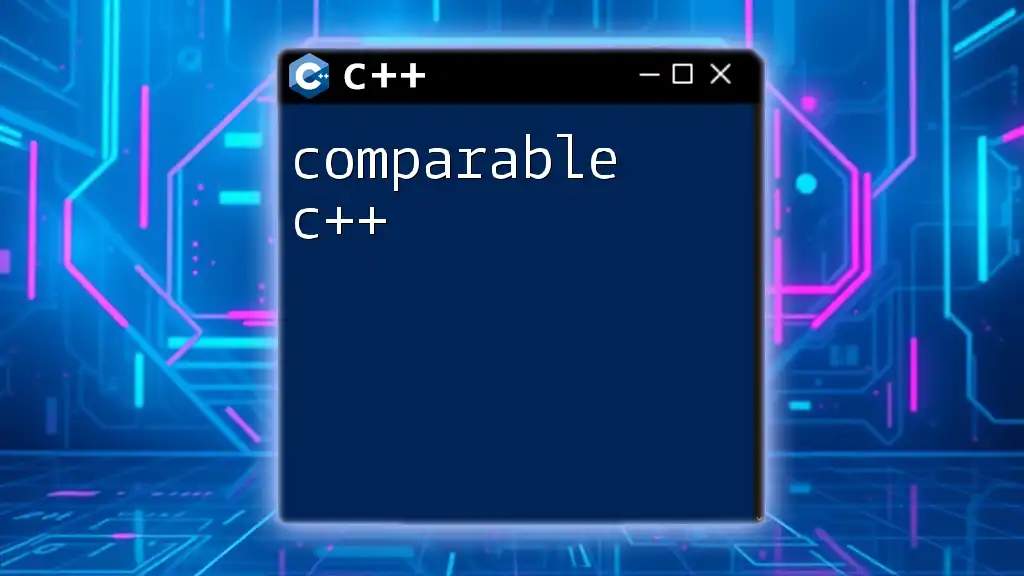
Conclusion
By understanding and implementing the principles of Lakos Large Scale C++, developers can enhance the maintainability, clarity, and robustness of their software systems. Emphasizing modular design, effective dependency management, clear organization, and advanced testing methods are all vital for mastering large-scale C++ development. As you apply these principles, you will not only produce better software but also foster a collaborative and productive development environment.
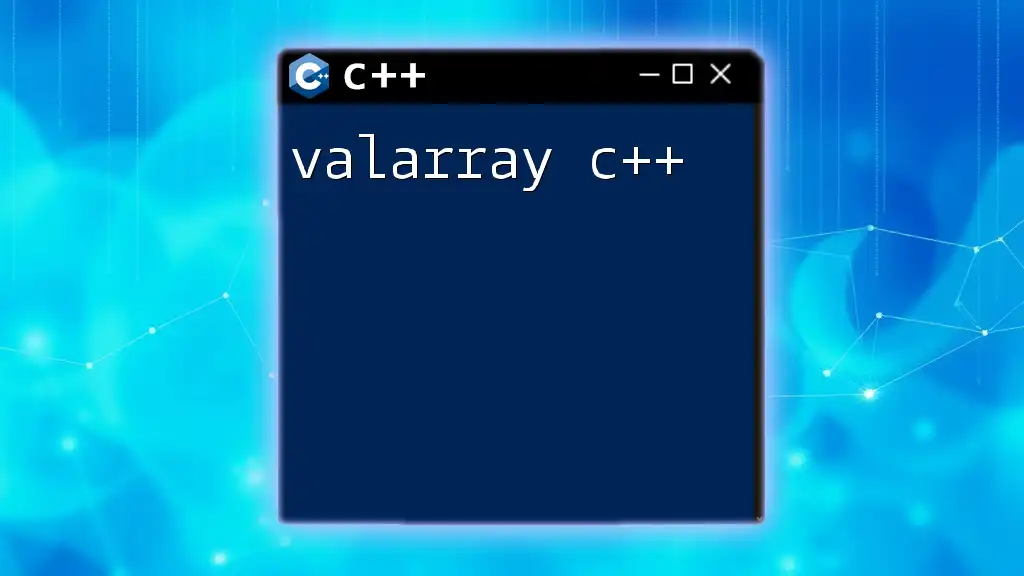
Additional Resources
Further learning opportunities include researching recommended books, articles, online courses, and community forums focused on Large Scale C++, providing aspiring developers with additional expertise and guidance.
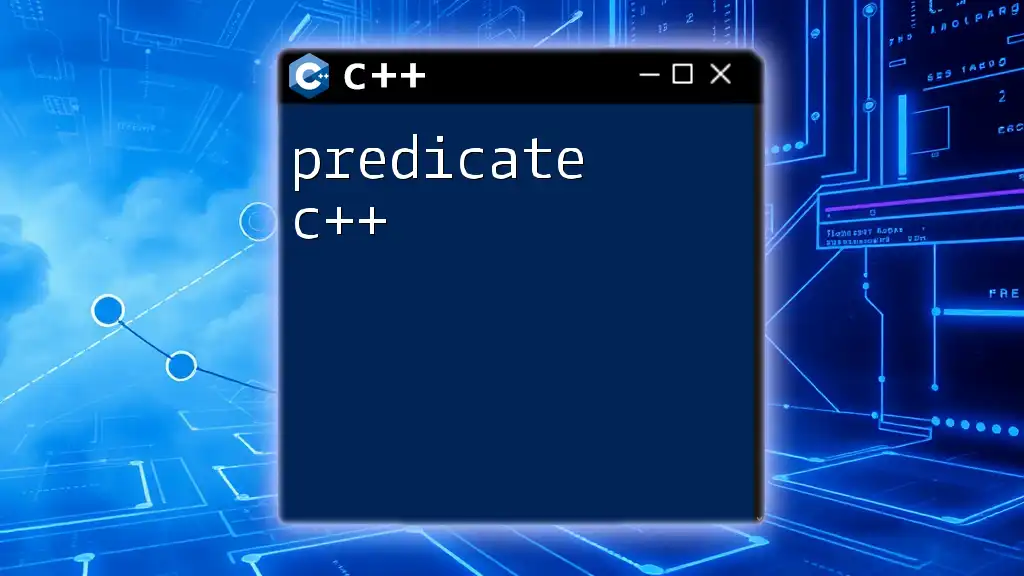
FAQs
What are the main benefits of using Lakos Large Scale C++?
Implementing Lakos Large Scale C++ enhances maintainability, decreases complexity, improves code quality, and fosters easier collaboration among teams.
How can I transition my existing codebase to a more modular design?
Start by identifying tightly coupled components and refactor them into modules, introducing interfaces where necessary. This requires a dedicated effort but pays off with improved architecture.
Are there any common pitfalls to avoid when adopting these practices?
Yes, common pitfalls include over-engineering solutions, neglecting proper documentation, and failing to establish a consistent workflow for collaboration and testing. Avoiding these can lead to a smoother implementation process.
By following the guidance laid out in this article, you will be well-equipped to embrace best practices in Lakos Large Scale C++, paving the way for the development of high-quality software systems.