WebGPU is a new web standard that enables high-performance graphics and computation capabilities in web applications using C++ commands to interact with the GPU directly.
Here’s a simple example of how to create a WebGPU device in C++:
#include <webgpu/webgpu.h>
int main() {
WGPUInstance instance = wgpuCreateInstance(nullptr);
WGPUAdapter adapter;
wgpuInstanceRequestAdapter(instance, nullptr, &adapter);
WGPUDevice device;
wgpuAdapterRequestDevice(adapter, nullptr, &device);
// Add further code to use the device...
wgpuDeviceRelease(device);
wgpuAdapterRelease(adapter);
wgpuInstanceRelease(instance);
return 0;
}
What is WebGPU?
WebGPU is an emerging graphics API that provides modern capabilities for rendering graphics and handling computational tasks on the GPU. Conceived as a successor to WebGL, it promises better performance and greater flexibility by offering low-level access to graphics hardware. Unlike traditional APIs like OpenGL and WebGL, which abstract many details of the GPU, WebGPU allows developers to harness the full power of the GPU for both graphics rendering and general-purpose computations.
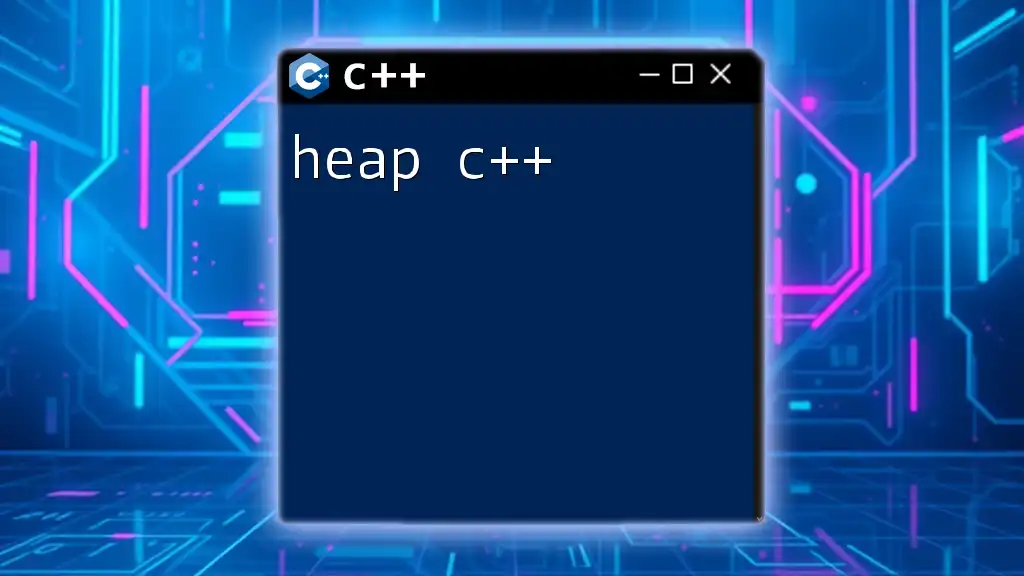
Key Features of WebGPU
WebGPU is designed with several key features that set it apart from its predecessors:
- Low-Level Access: WebGPU provides developers with direct access to GPU resources, enabling more efficient handling of graphics operations and computations. This allows for better performance tuning specific to the applications' needs.
- Performance: Compared to prior web graphics standards, WebGPU is capable of significantly higher performance due to its advanced capabilities and reduced overhead.
- Compute Shaders: WebGPU supports compute shaders, which enables developers to perform complex calculations on the GPU beyond traditional rendering tasks. This gives the ability to tackle a wider range of applications, from simulations to machine learning inference.
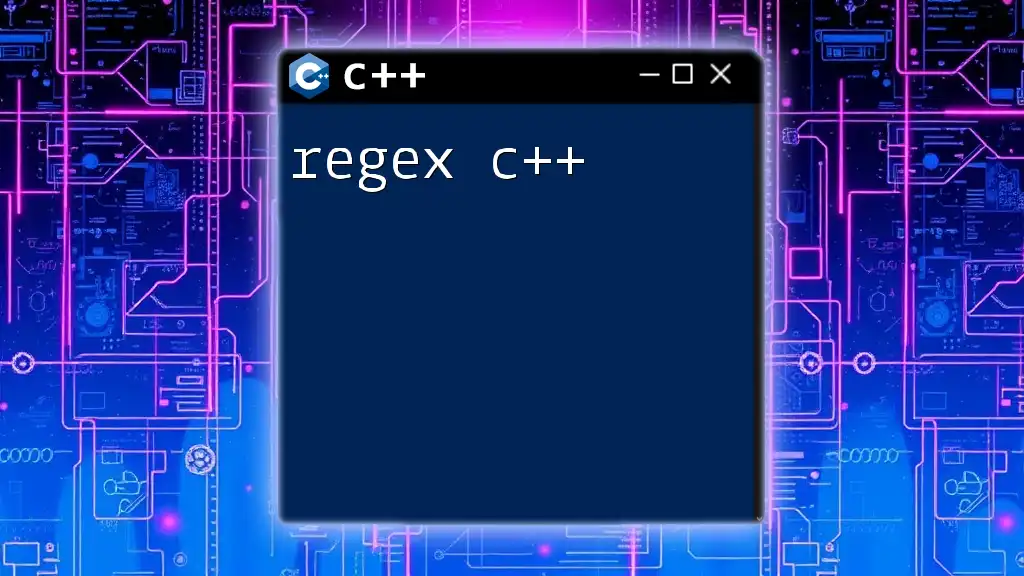
Setting Up Your Environment
Prerequisites
Before diving into WebGPU development, ensure you have the right software and tools:
- A compatible browser (Chrome or Firefox) with WebGPU enabled.
- Node.js for any necessary server-side functionalities.
- A C++ development environment (e.g., Visual Studio, GCC, or Clang).
Installing WebGPU
To leverage WebGPU in your browser, you may need to enable it first:
- For Chrome, navigate to `chrome://flags/#enable-webgpu` and enable the feature.
- For Firefox, check `about:config` and set `gfx.webgpu.enabled` to `true`.
This may require restarting your browser.
Setting Up a C++ Project
Creating a new C++ project integrated with WebGPU involves a few steps:
- Set up your C++ project structure.
- Include necessary libraries and dependencies, such as bindings for WebGPU. Libraries like `webgpu.h` can be vital for interfacing with WebGPU.
A sample `CMakeLists.txt` could look like this:
cmake_minimum_required(VERSION 3.10)
project(WebGPUExample)
set(CMAKE_CXX_STANDARD 17)
find_package(WebGPU REQUIRED)
add_executable(webgpu_example main.cpp)
target_link_libraries(webgpu_example PRIVATE WebGPU::webgpu)
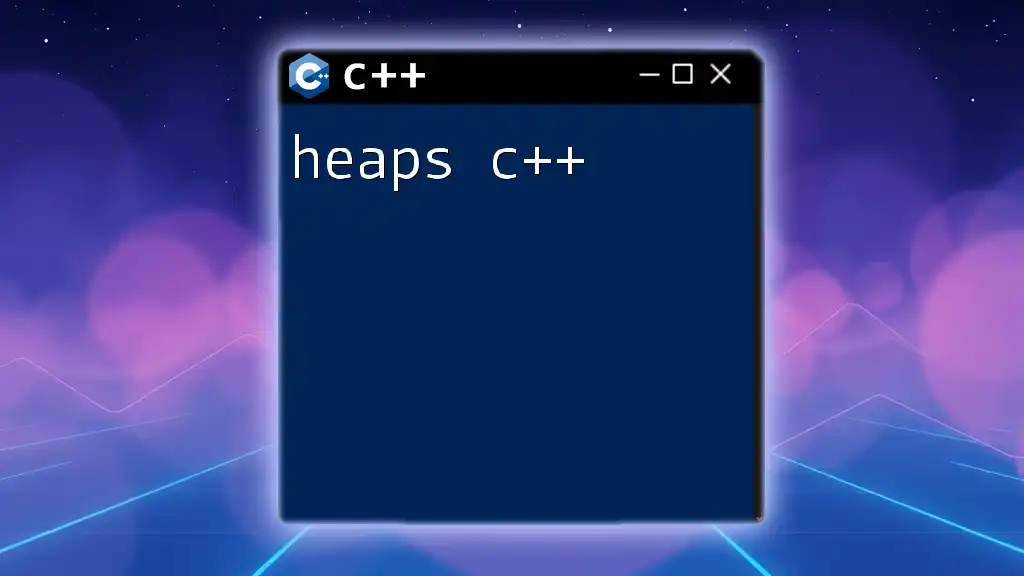
Understanding WebGPU Basics with C++
Core Concepts of WebGPU
GPUDevice and GPUContext
At the heart of working with WebGPU is the GPUDevice. It is responsible for creating commands and resources for the GPU. When constructing a GPU application, your first task is to request a device. The GPUContext plays a key role as well, facilitating interactions with resources like command buffers and pipelines.
To create a GPUDevice, you might use the following C++ code snippet:
gpu::Device device = gpu::RequestDevice();
Buffers and Textures
Buffers and textures are foundational elements of WebGPU rendering. Buffers are used to store vertex data, indices, and uniform data, while textures are used for images mapped onto geometric surfaces.
Here’s an example of how to create a buffer:
gpu::BufferDescriptor bufferDesc = {
.size = size,
.usage = gpu::BufferUsage::Vertex | gpu::BufferUsage::CopyDst,
};
auto buffer = device.CreateBuffer(&bufferDesc);
This allocates space on the GPU and specifies how you plan to use that space.
Pipeline Setup
Rendering Pipeline Overview
The rendering pipeline is a systematic path that graphics data takes from input to output. Key stages include vertex shading, rasterization, and fragment shading.
Creating a Render Pipeline
To define a render pipeline, you will specify various states and stages of processing. A simplified example of creating a render pipeline is as follows:
gpu::RenderPipelineDescriptor pipelineDesc;
pipelineDesc.vertexStage.module = vertexModule;
pipelineDesc.fragmentStage.module = fragmentModule;
pipelineDesc.primitiveTopology = gpu::PrimitiveTopology::TriangleList;
auto renderPipeline = device.CreateRenderPipeline(&pipelineDesc);
This code snippet outlines the configuration of your desired rendering stages.
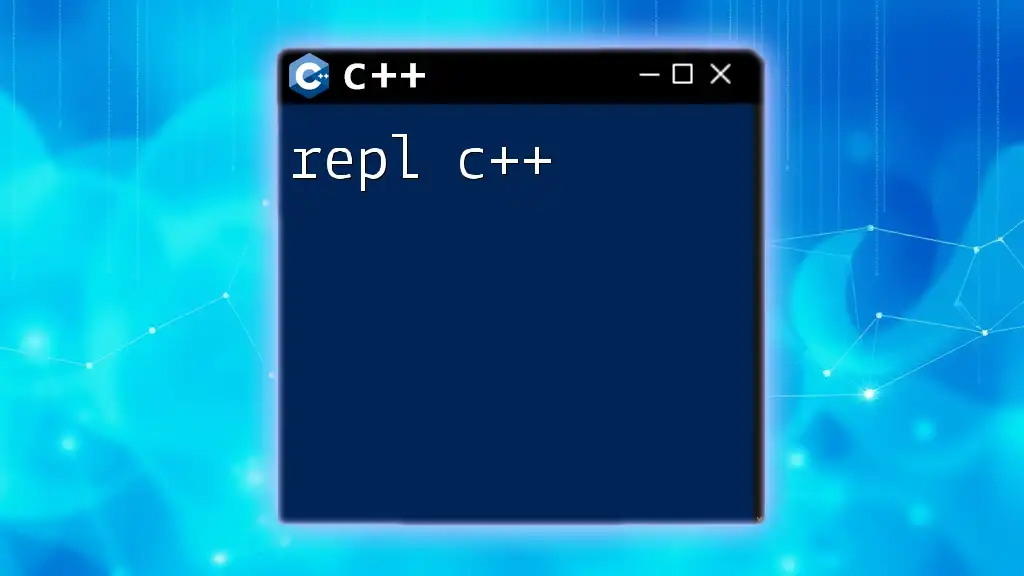
Writing Your First WebGPU Application
Setting Up Shaders
Vertex and Fragment Shaders
Shaders are programs executed on the GPU, processing vertices and fragments (pixels). Understanding shader languages like GLSL is crucial.
Here is a basic example of a vertex shader:
// Example: Simple vertex shader
#version 450
layout(location = 0) in vec2 position;
void main() {
gl_Position = vec4(position, 0.0, 1.0);
}
This shader processes vertex positions to create geometry.
Compiling Shaders for WebGPU
To integrate shaders into your C++ application, you must compile them to the appropriate format and link them with the GPU resources.
Drawing with WebGPU
Setting Up Command Buffers
Command buffers allow you to package a sequence of drawing commands. Here’s how you can create a command buffer:
gpu::CommandBufferDescriptor commandBufferDesc;
auto commandBuffer = device.CreateCommandBuffer(&commandBufferDesc);
Drawing Calls
Finally, to perform drawing operations through your command buffer, you can utilize the `Draw` command:
commandBuffer.BeginRenderPass(&renderPassDescriptor);
commandBuffer.Draw(3); // Draw a triangle
commandBuffer.EndPass();
This sequence of commands sets the stage for rendering graphics on the canvas.
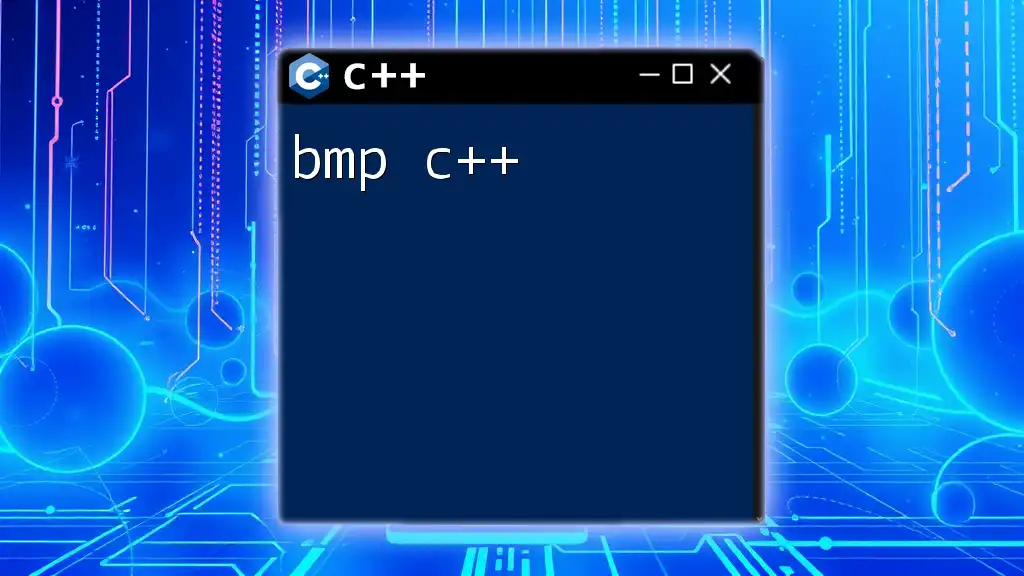
Advanced WebGPU Techniques
Handling Textures and Images
Incorporating images and textures into your applications adds depth and realism. This involves loading texture data into the GPU and bind them to shaders, allowing your graphics to utilize photorealistic textures.
Compute Shaders with WebGPU
Compute shaders extend the capabilities of WebGPU beyond mere graphics rendering. They allow for complex computations to be processed on the GPU, enabling richer applications. Developing compute shaders requires similar setup steps as rendering shaders but with specific configurations tailored for compute operations.
Here’s a sample compute shader snippet:
// Example: Simple compute shader
#version 450
layout(local_size_x = 16, local_size_y = 16) in;
void main() {
// Implement some compute operations
}
Optimizing Performance
To ensure your WebGPU applications run effectively, consider the following optimization tips:
- Minimize data transfers between CPU and GPU.
- Use efficient data formats and alignments.
- Profile your application to identify bottlenecks.
Common pitfalls include over-usage of resources and less-than-ideal command buffer management.
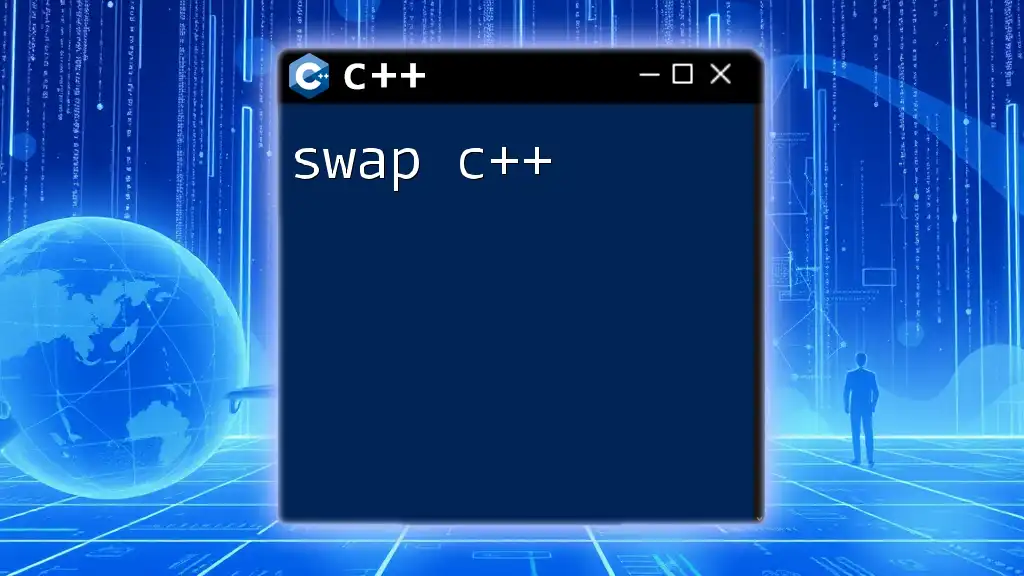
Debugging and Troubleshooting
Debugging WebGPU in C++
Debugging WebGPU applications can be challenging, but vital tools can assist you. Browser developer tools often include GPU debugging capabilities. Profiling tools can help track performance and resource usage.
For troubleshooting common issues, ensure your GPU drivers are up to date. Review error logs generated by the WebGPU API to diagnose misconfigurations or coding errors.
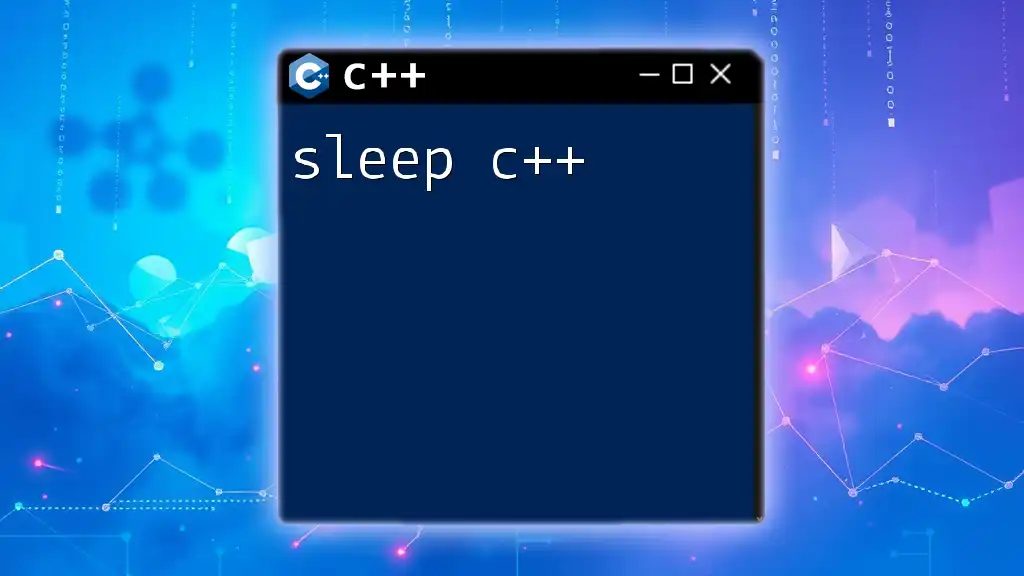
Conclusion
As WebGPU evolves, it opens new avenues for web graphics programming and computation. By leveraging its constructs within C++, developers can create high-performance applications that rival native performance. Experiment with the various features WebGPU offers, and feel empowered to push the boundaries of what’s possible on the web!
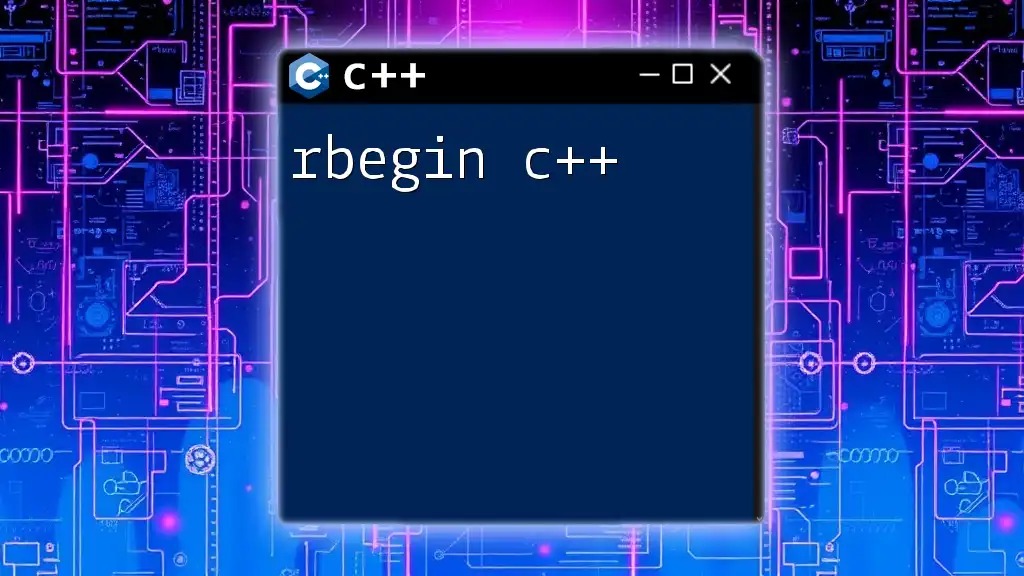
Additional Resources
To further enhance your knowledge, explore recommended readings, video courses, and participate in online forums dedicated to WebGPU. Engaging with the community can provide valuable insights and support as you delve deeper into GPU programming.
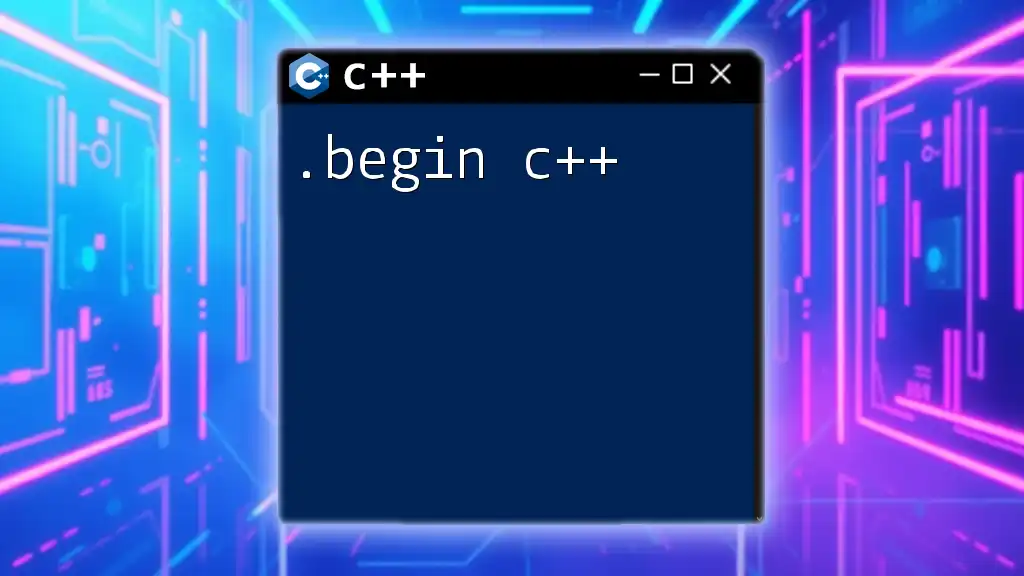
Call to Action
Embark on your WebGPU journey today by applying the concepts and code outlined in this guide. Don't hesitate to share your experiments, projects, and questions within the community, and consider signing up for more advanced courses or newsletters to stay updated on WebGPU developments!