C++ has significantly influenced many programming languages by introducing object-oriented principles, templates, and a standard library that emphasizes efficiency and performance, paving the way for languages like Java, C#, and Python.
Here's a simple example demonstrating object-oriented programming in C++:
#include <iostream>
using namespace std;
class Animal {
public:
void speak() {
cout << "The animal speaks!" << endl;
}
};
class Dog : public Animal {
public:
void speak() {
cout << "The dog barks!" << endl;
}
};
int main() {
Dog myDog;
myDog.speak(); // Outputs: The dog barks!
return 0;
}
The Evolution of Programming Languages
The landscape of programming languages has undergone significant transformations since the inception of computing. In the early days, languages like Assembly provided low-level access to hardware, requiring a deep understanding of computer architecture. As the field evolved, abstract languages emerged, simplifying manual memory management and syntax complexities.
C++ was introduced by Bjarne Stroustrup in the early 1980s as an extension of the C programming language. C++ came with a set of features that were revolutionary at the time, notably Object-Oriented Programming (OOP), Generic Programming, and an emphasis on performance and control. These concepts not only shaped C++ itself but also left a profound mark on various programming languages that followed.
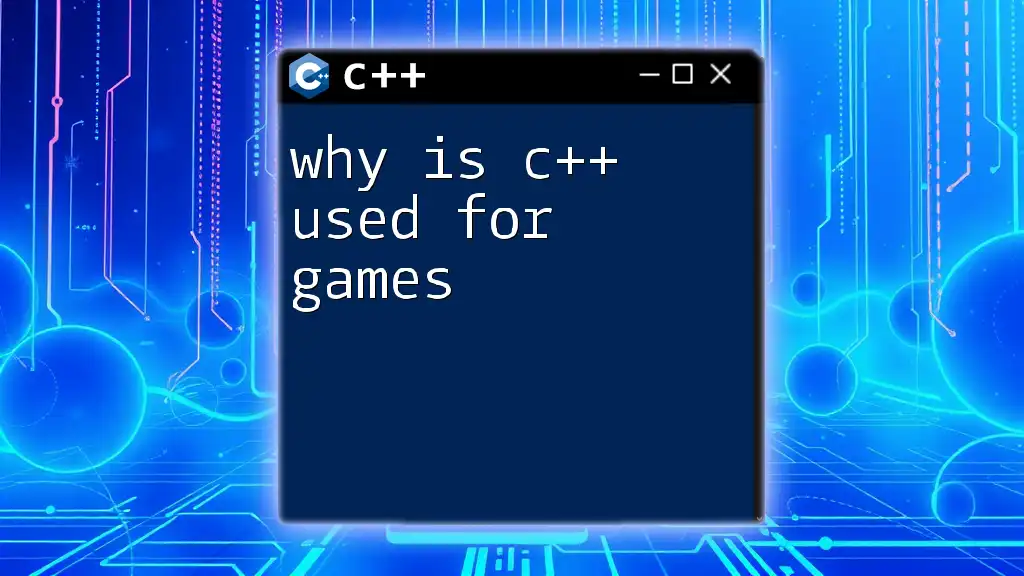
Direct Influences of C++
Java
Java emerged in the mid-1990s with strong ties to C++, particularly in its syntax and structure. Many developers transitioning from C++ to Java find the languages similar due to their shared foundations.
OOP Concepts Java adopted core OOP principles prevalent in C++, such as encapsulation, inheritance, and polymorphism. This shift fundamentally influenced software design, promoting reusability and modularity.
Memory Management While C++ uses manual memory management, Java introduced garbage collection, reducing the risk of memory leaks and pointer-related errors. This allowed developers to focus more on application logic rather than memory management intricacies.
Example of Similar Syntax:
class Animal {
public:
virtual void sound() {
cout << "Generic Animal Sound" << endl;
}
};
class Dog : public Animal {
public:
void sound() override {
cout << "Bark" << endl;
}
};
C#
C#, developed by Microsoft in the early 2000s, drew heavily from the design principles of both C++ and Java.
C++’s Impact on C# Design C# inherited many OOP concepts directly from C++. It streamlined some of the syntax and provided an enhanced focus on safety and productivity.
Features Inspired by C++ C# introduced modern features, such as delegates and events, making event-driven programming easier. This shift was designed to enhance the implementation of robust applications while maintaining the flexibility derived from C++ principles.
Example Comparison:
// C++ Example
class Button {
public:
void click() {
// Handle Click
}
};
// C# Example
class Button {
public void Click() {
// Handle Click
}
}
Python
Python, known for its simplicity and readability, adopted core concepts from C++.
How C++ Inspired Python’s Development While Python is dynamically typed, the influence of C++ is evident in its support for object-oriented programming. Both languages encourage a more intuitive and organized way of structuring code.
Object-Oriented Features Python implements classes and inheritance, ensuring that the OOP principles established by C++ continue to influence modern programming.
Extensions and Libraries C++ libraries allow Python to achieve higher performance when necessary. For instance, popular libraries like NumPy and Pandas utilize C++ under the hood for computation-heavy tasks, proving the versatility of C++ in enhancing Python's capabilities.
Code Snippet Example:
class Animal:
def sound(self):
print("Generic Animal Sound")
class Dog(Animal):
def sound(self):
print("Bark")
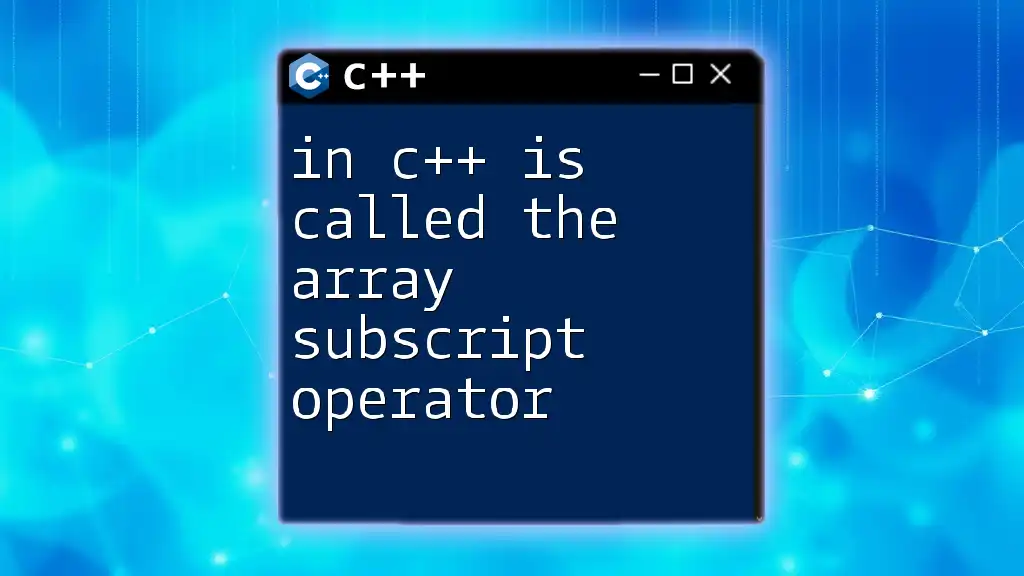
Indirect Influences of C++
JavaScript
JavaScript represents a significant evolution of programming languages influenced by C++. While it is a dynamic language, its growth has been shaped by various OOP principles originating from C++.
Dynamic Language Growth JavaScript borrowed ideas like prototyping, which connects directly to OOP designs. This allows for flexible object creation and inheritance patterns.
Frameworks Inspired by C++ Principles The influence of C++ is also evident in JavaScript frameworks such as Node.js, which prioritize performance in server-side scripting, similar to C++’s goals in system-level programming.
Code Snippet Example:
function Animal() {}
Animal.prototype.sound = function() {
console.log("Generic Animal Sound");
};
function Dog() {}
Dog.prototype = Object.create(Animal.prototype);
Dog.prototype.sound = function() {
console.log("Bark");
};
Swift
Swift, created by Apple in 2014, is a modern programming language that integrates many influences, including C++.
Design Influences While aiming for simplicity and safety, Swift respects the principles set forth by C++. It incorporates features such as optional types and closures, refining many of C++’s object-oriented features.
Combining OOP with Functional Programming Swift allows functional programming styles, yet retains the structured approach to OOP pioneered by C++. This hybrid design has attracted developers looking to leverage the strengths of both paradigms.
Code Snippet Example:
class Animal {
func sound() {
print("Generic Animal Sound")
}
}
class Dog: Animal {
override func sound() {
print("Bark")
}
}
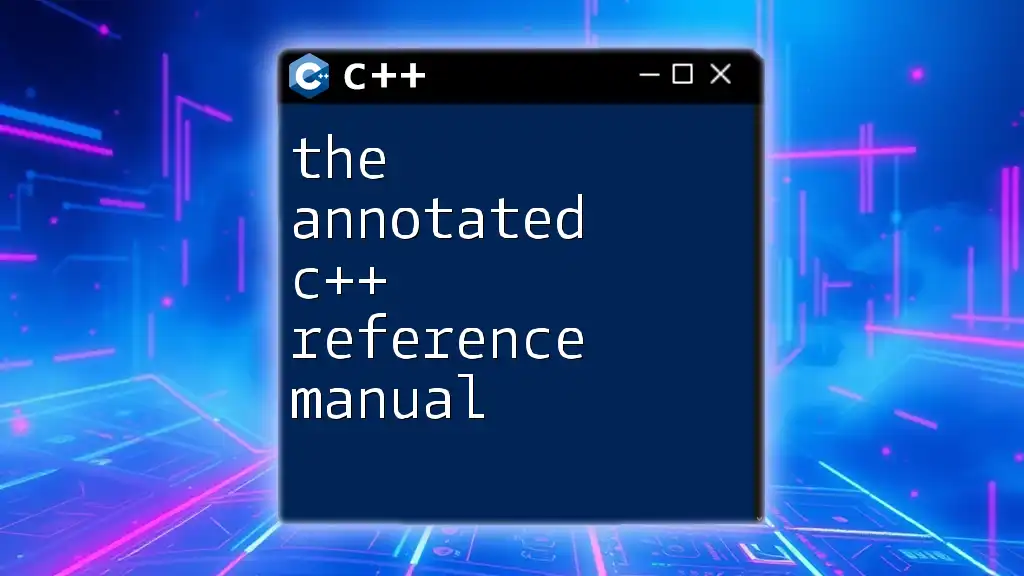
Key Concepts and Features Transferred from C++
OOP Principles
The introduction of OOP in C++ has laid the groundwork for many modern programming languages. C++ emphasized encapsulation, inheritance, and polymorphism, influencing how software is architected. These principles promote a modular approach, enhancing code maintainability and scalability.
Template Programming
C++’s groundbreaking support for template programming propagated generic programming concepts across languages like Rust and C#. This feature allows developers to create functions and data structures that work with any data type while maintaining type safety—a vital asset in the current landscape of programming.
Code Example:
template<typename T>
class MyVector {
public:
void push_back(T value) { /*...*/ }
};
Performance and Resource Management
C++ is often celebrated for its close-to-the-metal programming capabilities, allowing developers fine-grained control over system resources. This emphasis on performance and efficient memory management has influenced system programming languages, particularly Rust, which emphasizes safety without sacrificing speed.
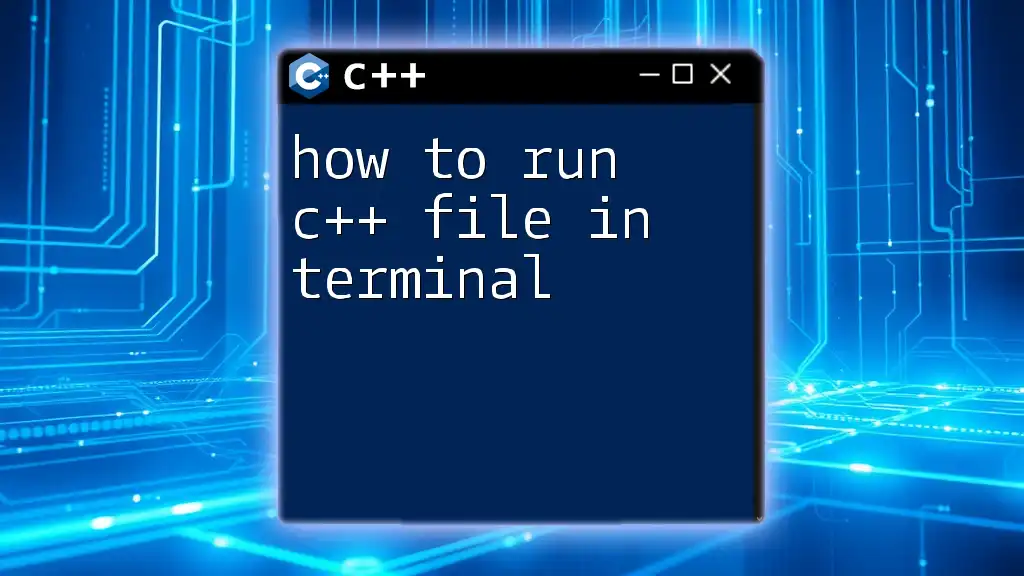
Conclusion
Reflecting on the evolution of programming languages reveals C++’s pivotal role in shaping the programming landscape. Its principles of OOP, performance, and resource management have significantly influenced numerous languages, creating a ripple effect that continues to resonate with modern developers. Understanding how C++ has influenced other languages is essential, not only for historical context but also for current software design practices. As programming languages evolve, the legacy of C++ will undoubtedly remain integral to their development.
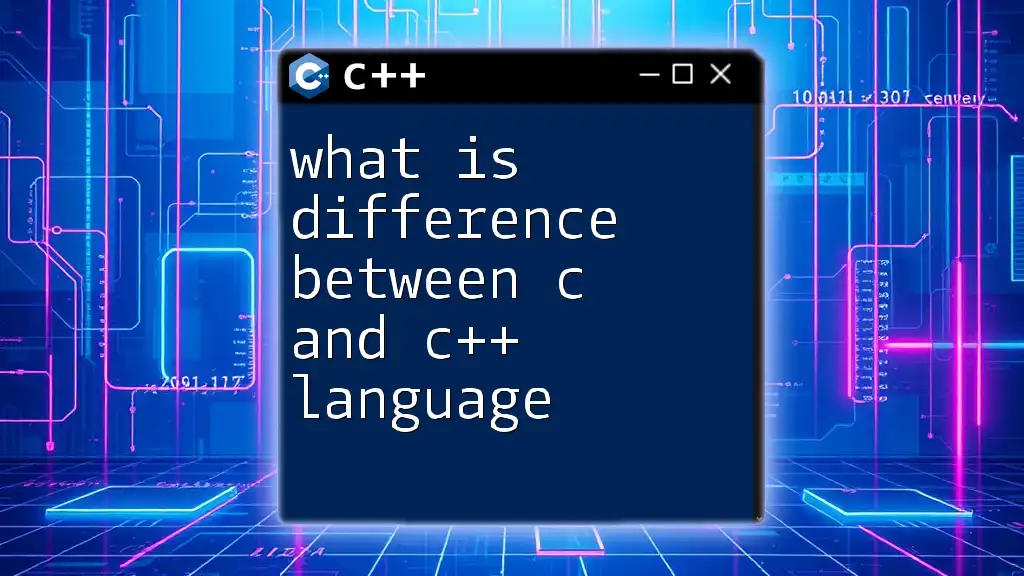
Call to Action
Consider diving into C++ to appreciate its robust architecture and features that underpin many modern languages. Mastering C++ commands can heighten your programming skills, providing a solid foundation for understanding other languages inspired by it.
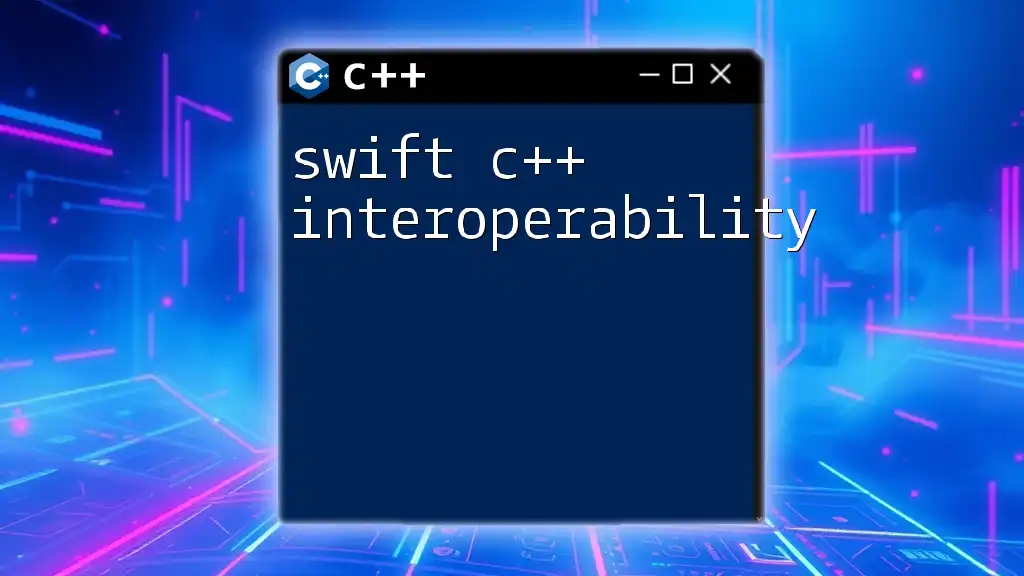
Additional Resources
Explore recommended books and online courses to grasp the intricacies of C++ and its lasting influence on other programming languages. Engage in community discussions about programming language evolution to enhance your understanding and stay updated on the industry's trends.