The Annotated C++ Reference Manual is a comprehensive resource that provides detailed explanations and annotations for C++ programming commands, enhancing the understanding of syntax and functionality.
Here’s a simple code snippet demonstrating a basic C++ program structure:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
History of C++ and the Reference Manual
C++ has come a long way since its inception in the early 1980s. Originally developed by Bjarne Stroustrup at Bell Labs, C++ has evolved significantly over the years, impacting not just its users but also influencing many programming paradigms. As the language grew in complexity, the need for a comprehensive guide became apparent.
The Annotated C++ Reference Manual, commonly referred to as the ARM, was created as a response to this need. Authored by notable figures such as Bjarne Stroustrup and others, the manual was designed to provide a thorough reference that could accompany developers as they navigated the intricacies of C++. The ARM aimed not just to document the language but to provide context and deeper understanding through annotations.
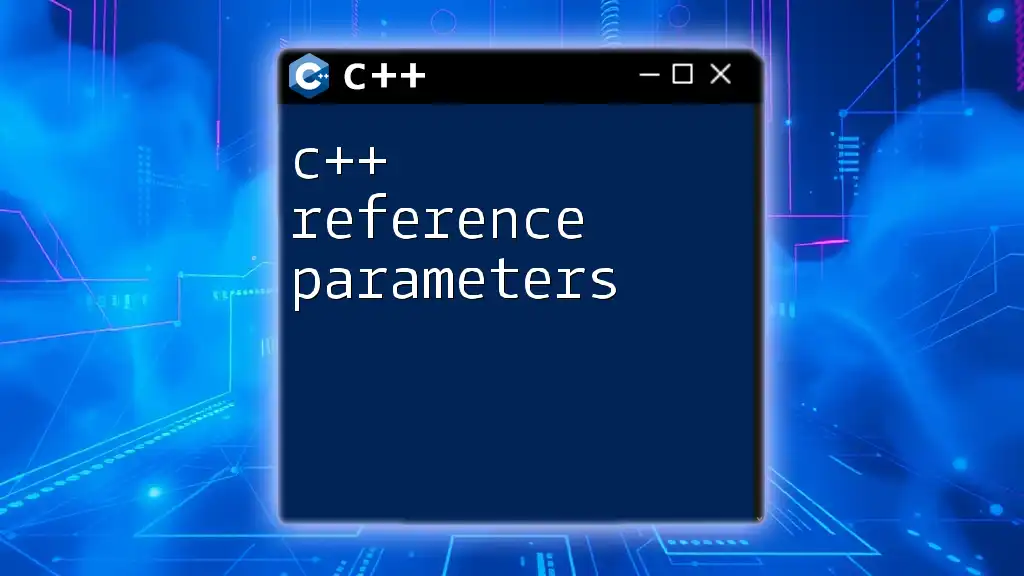
Key Features of the Annotated C++ Reference Manual
Comprehensive Coverage
One of the standout features of the annotated C++ reference manual is its extensive scope. The manual covers every significant aspect of the C++ language, from the fundamental syntax to the more advanced features introduced in later standards. This breadth ensures that whether you are a beginner or an experienced coder, you have access to essential information.
In addition to describing syntax and semantics, the manual also contains comparisons between different C++ standards, which helps developers understand the evolution of the language. For example, the transition from C++98 to C++11 introduced essential features like auto keyword, range-based loops, and smart pointers that fundamentally changed the way C++ is used.
Annotations and Explanatory Text
The annotations within the manual provide a critical layer of context that transforms simple definitions into meaningful explanations. These clarifications are especially important given C++'s complexity. Annotations can help demystify aspects that might otherwise confuse the reader.
For instance, consider the definition of a class:
class MyClass {
public:
int myVariable; //Variable to store an integer
void display(); //Function to display variable
};
In the manual, an annotation might elaborate on accessing class members, the significance of public/private modifiers, and the concept of encapsulation. This additional commentary enhances the reader's understanding of not just what a piece of code does, but why it’s written that way.
Examples and Code Snippets
Examples are crucial in C++ education, as they provide practical insights into how theoretical concepts work in reality. The annotated C++ reference manual comes packed with code snippets that illustrate complex ideas, bridging the gap between comprehension and application.
For example, templates are a powerful feature of C++ that allows for generic programming. The manual provides an illustration of how to effectively use templates:
template <typename T>
T add(T a, T b) {
return a + b; //Function to add two generic types
}
The annotations accompanying this snippet could explain features like type deduction, compile-time polymorphism, and the benefits of writing reusable code.
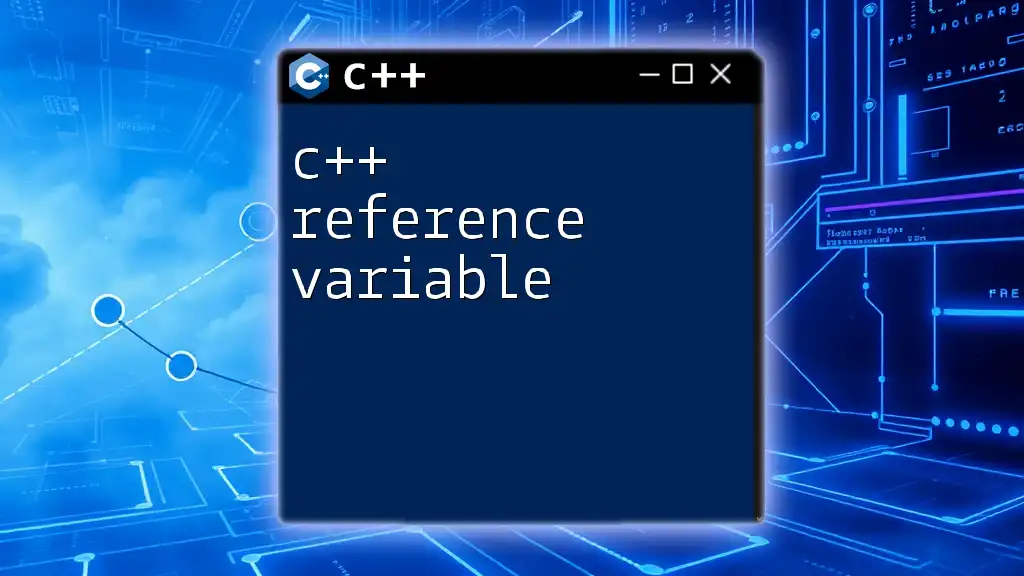
Structure of the Manual
Sections and Organization
The annotated C++ reference manual is meticulously structured to facilitate easy navigation. It is organized into several core sections that align closely with the language's framework.
- Syntax: Detailed breakdowns of the syntax rules.
- Semantics: In-depth explanations of how the language behaves.
- Libraries: Comprehensive coverage of the Standard Template Library (STL) and other vital libraries.
This organization makes it simple for users to look up specific topics or to study sections like object-oriented programming or memory management in isolation.
Index and Appendices
A well-crafted index is essential for any technical reference, and the ARM excels in this regard. Users can quickly locate topics of interest, ensuring a smoother reference experience.
Additionally, the appendices included in the manual offer supplementary resources, touching upon advanced topics such as concurrency, template metaprogramming, and the nuances of the C++ memory model.
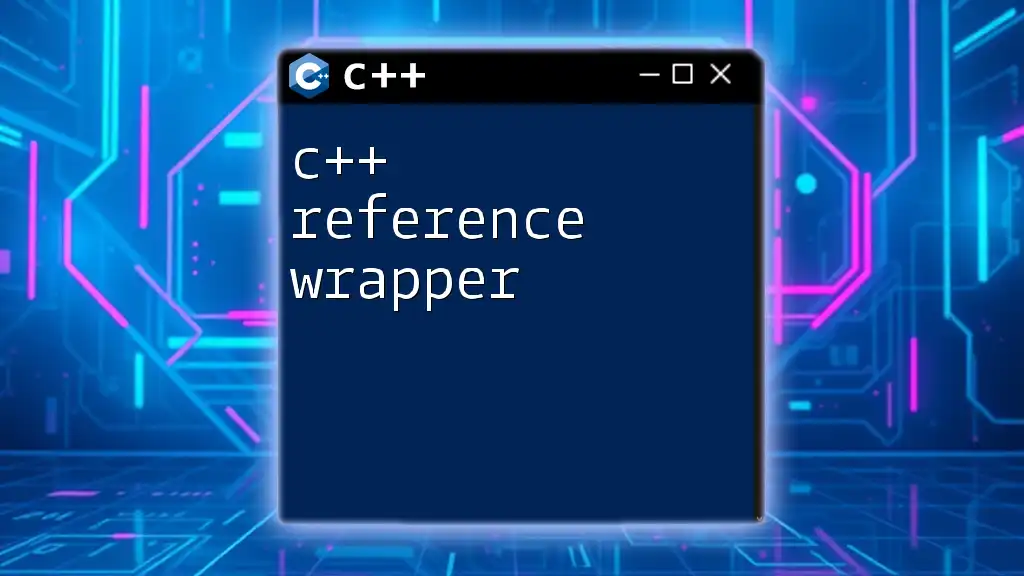
How to Use the Annotated C++ Reference Manual Effectively
Finding Information Quickly
To maximize the benefits of the annotated C++ reference manual, it’s crucial to master the skill of quickly finding information. Start by familiarizing yourself with the index and table of contents. This familiarity will enable you to navigate effectively without wasting time scrolling through pages.
Integrating the Manual with Learning
The ARM should not be treated as a standalone resource. Pair it with hands-on practice and modern tutorials. Engaging with real-world projects while referencing the manual can solidify concepts and enhance retention.
Recommended Study Practices
To absorb the material effectively, consider chunking information into manageable segments. This strategy helps combat cognitive overload and allows for better retention. Revisit complex topics regularly to deepen your understanding — repetition is key in mastering programming.
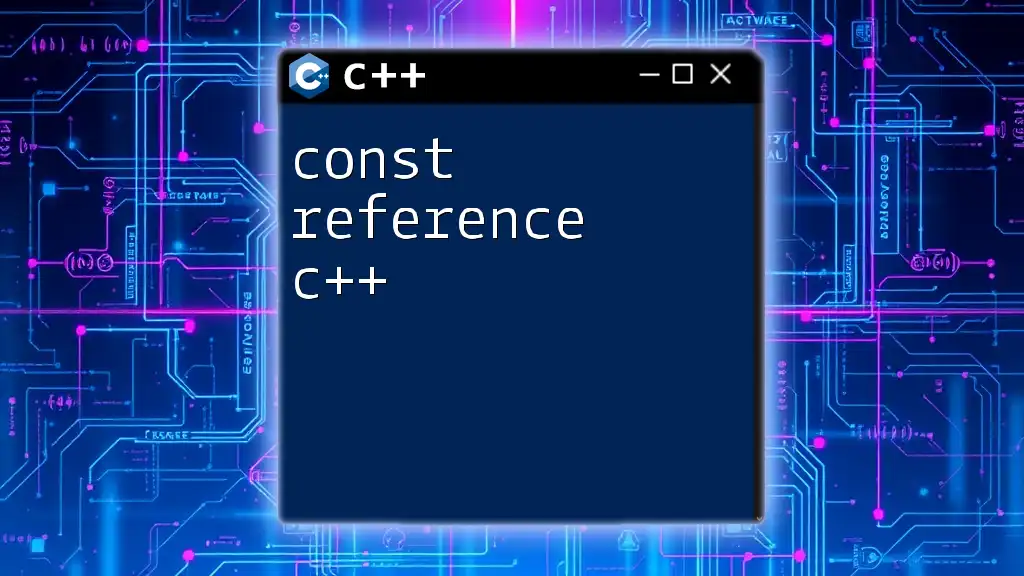
The Role of the Annotated C++ Reference Manual in Current Development
Modern C++ Standards
The annotated C++ reference manual remains relevant even in the ever-evolving landscape of C++. It is crucial for understanding the nuances of modern C++ standards such as C++11, C++14, C++17, and C++20. Each of these standards introduced significant features that changed the way developers approach coding tasks.
By providing historical context and practical examples, the ARM aids developers in transitioning smoothly through different C++ standards, ensuring that they can utilize the most current techniques and best practices.
Best Practices Derived from the Manual
The insights offered in the annotated manual contribute directly to coding standards and best practices within the industry. Developers are encouraged to internalize the practices discussed in the manual, as they are rooted in extensive research and real-world applications.
For instance, consistency in naming conventions and understanding RAII (Resource Acquisition Is Initialization) are best practices deeply informed by the principles laid out in the ARM.
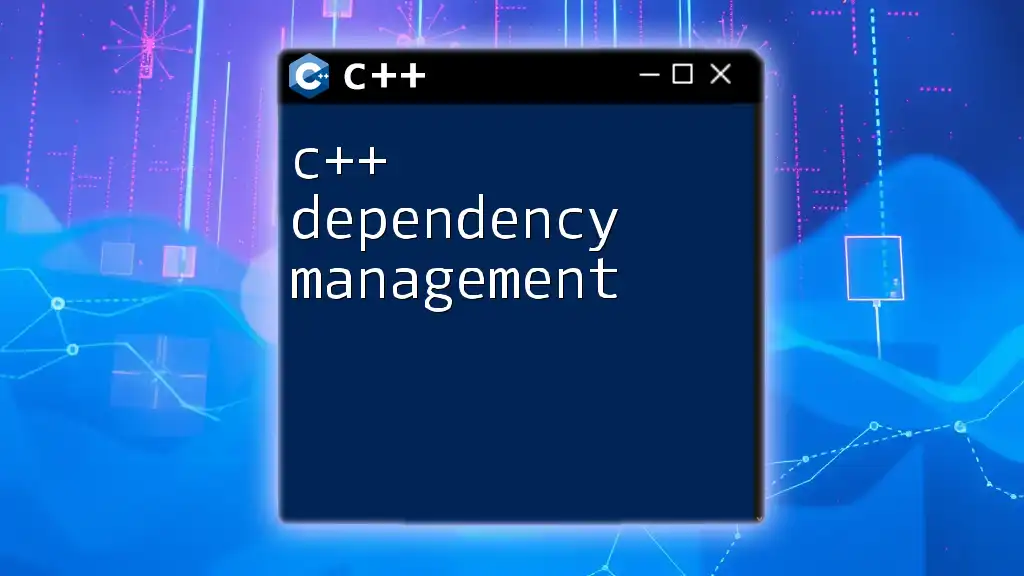
Conclusion
The annotated C++ reference manual serves as an indelible resource for anyone seeking to master C++. Its detailed annotations, exhaustive examples, and structured organization take the complexity of C++ and make it accessible to all levels of programmers. Embracing the manual not only enhances your programming skills but also deepens your appreciation for the language and its continued evolution.
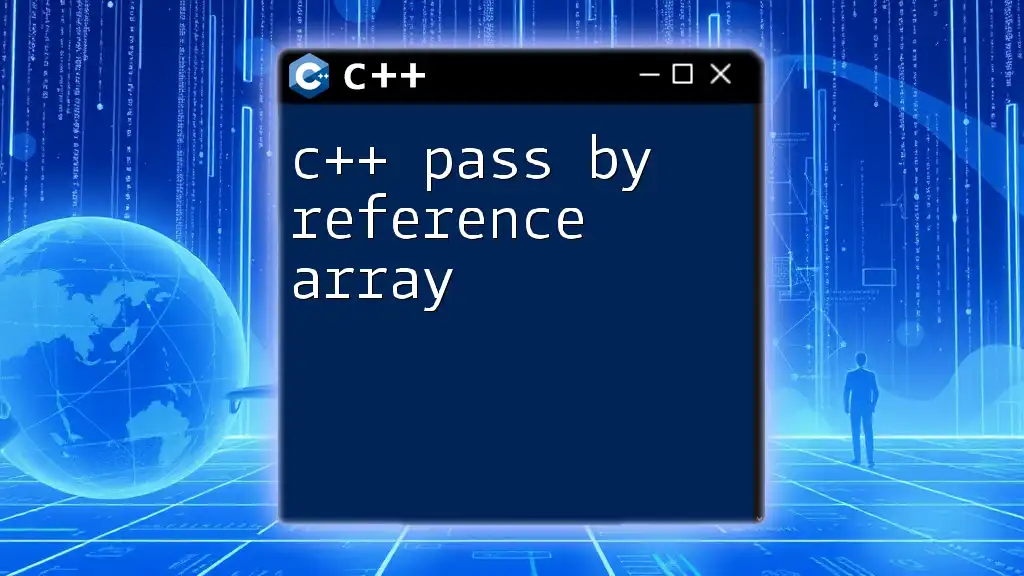
Call to Action
I invite you to share your experiences with the annotated C++ reference manual. How has it impacted your programming journey? Also, don’t forget to subscribe to our newsletter or follow us on social media for more tips and resources as you continue your exploration of C++.
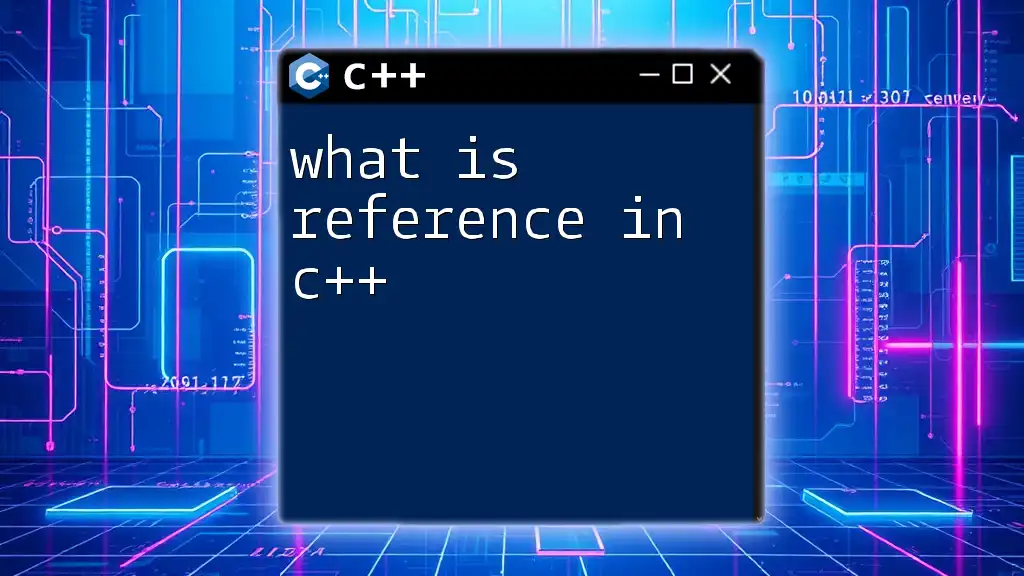
FAQs About the Annotated C++ Reference Manual
While diving into C++ using the annotated C++ reference manual, you may have some common questions. This section aims to clarify common queries regarding the manual's contents and usage, ensuring you're fully equipped to leverage this invaluable resource.