The C++ GCD (Greatest Common Divisor) can be calculated using the Euclidean algorithm; here’s a simple implementation:
#include <iostream>
using namespace std;
int gcd(int a, int b) {
return b == 0 ? a : gcd(b, a % b);
}
int main() {
int num1 = 48, num2 = 18;
cout << "GCD of " << num1 << " and " << num2 << " is " << gcd(num1, num2) << endl;
return 0;
}
What is GCD?
The greatest common divisor (GCD) of two or more integers is the largest positive integer that divides each of the integers without leaving a remainder. Understanding GCD is essential in various fields of mathematics and computer science, particularly in number theory.
The importance of GCD extends to numerous practical applications, including simplifying fractions, solving equations, and optimizing algorithms. By mastering GCD, programmers can enhance their ability to tackle complex problems in a more efficient manner.
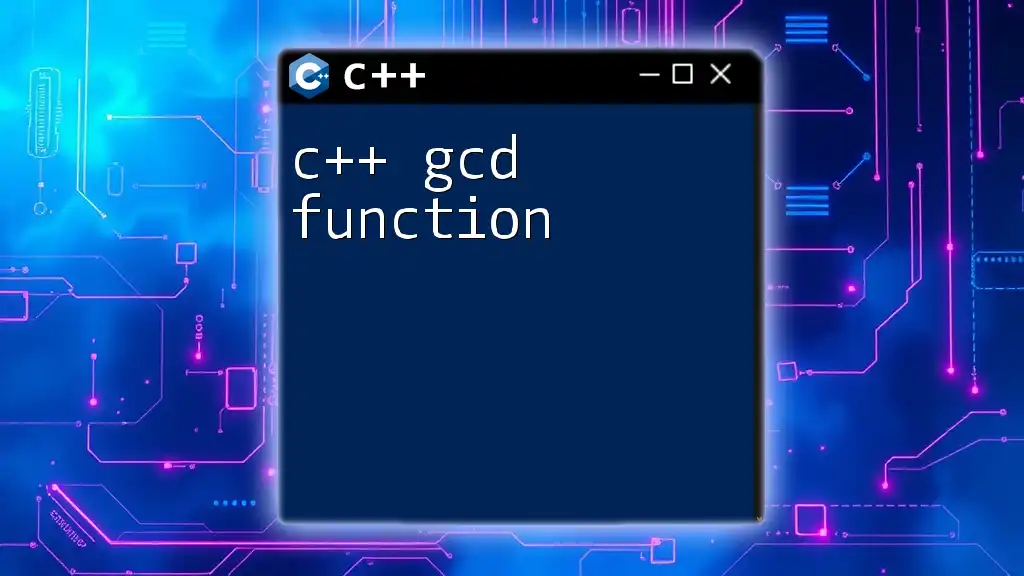
Understanding GCD Function in C++
The GCD function in C++ plays a significant role in streamlining calculations involving integers. It not only simplifies fractions but also serves as a foundation for various algorithms.
Utilizing the GCD allows programmers to perform operations like reducing fractions to their simplest form, which is particularly useful in mathematical computations and data representations.
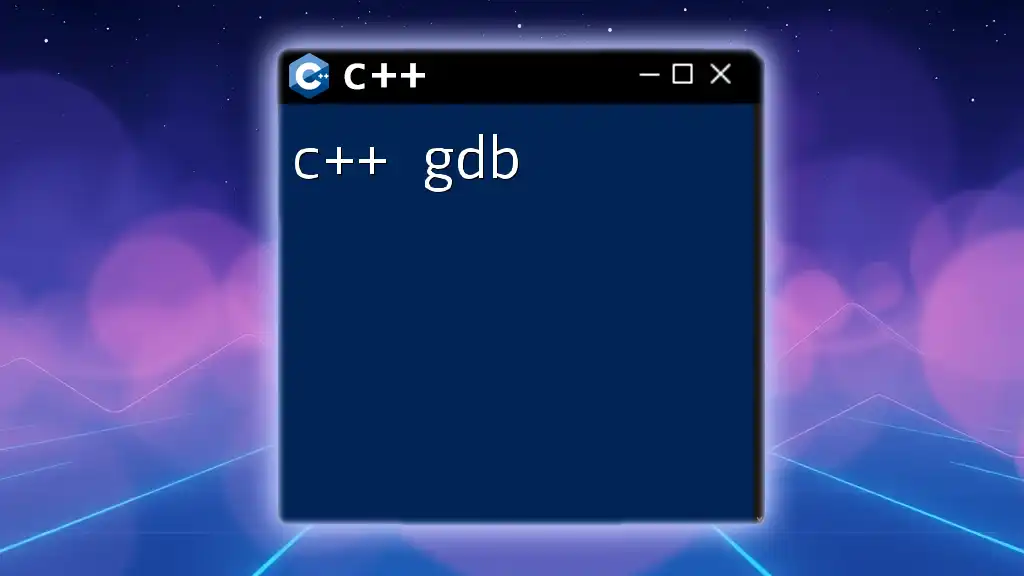
Implementing GCD in C++
Basic GCD Algorithm
The Euclidean algorithm is the most effective method for calculating the GCD. It operates on the principle that the GCD of two numbers also divides their difference. The algorithm steps through a series of divisions until reaching a remainder of zero. The last non-zero remainder is the GCD.
Here’s the basic implementation of the GCD function in C++:
int gcd(int a, int b) {
while (b != 0) {
int t = b;
b = a % b;
a = t;
}
return a;
}
In this code snippet, we utilize a loop that continues until `b` becomes zero. At each iteration, we update `a` to be `b`, and `b` to be `a % b`, effectively reducing the problem size. The final value of `a` is returned as the GCD.
GCD Function in C++ Standard Library
C++ offers a built-in function to compute GCD through the standard library. By including the `<numeric>` header, programmers can leverage `std::gcd`, which simplifies the code substantially and enhances readability and performance.
Here's an example of using `std::gcd`:
#include <iostream>
#include <numeric>
int main() {
int a = 56, b = 98;
std::cout << "GCD of " << a << " and " << b << " is: " << std::gcd(a, b) << std::endl;
return 0;
}
In this case, `std::gcd` internally calls an optimized implementation of the Euclidean algorithm, allowing developers to focus on higher-level programming without delving into specific algorithmic details.
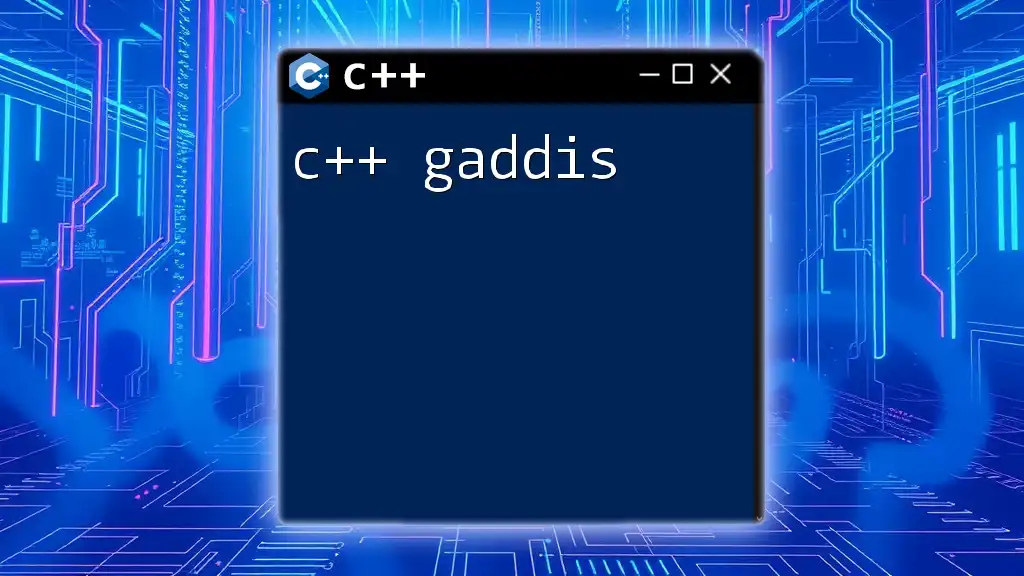
Advanced Topics in GCD
GCD for Multiple Numbers
Calculating the GCD for more than two integers requires extending our approach. The GCD can be found iteratively or recursively by utilizing the GCD of pairs.
Here’s how to compute the GCD of multiple numbers in an array:
int gcd_multiple(int arr[], int n) {
int result = arr[0];
for (int i = 1; i < n; i++) {
result = gcd(result, arr[i]);
}
return result;
}
In this implementation, we begin with the first element of the array as the initial GCD result. As we iterate through the array, we keep updating the result with the GCD of the current result and the next element.
Performance Considerations
When evaluating the performance of GCD algorithms, it is essential to analyze their time complexity. The Euclidean algorithm runs in logarithmic time, specifically O(log(min(a, b))), making it very efficient even for large integers.
Comparing custom implementations with standard library functions like `std::gcd`, developers can often find that the latter is optimized for performance, memory usage, and edge case handling. It is generally recommended to utilize library functions unless specific custom behavior is required, as they are well-tested and optimized.
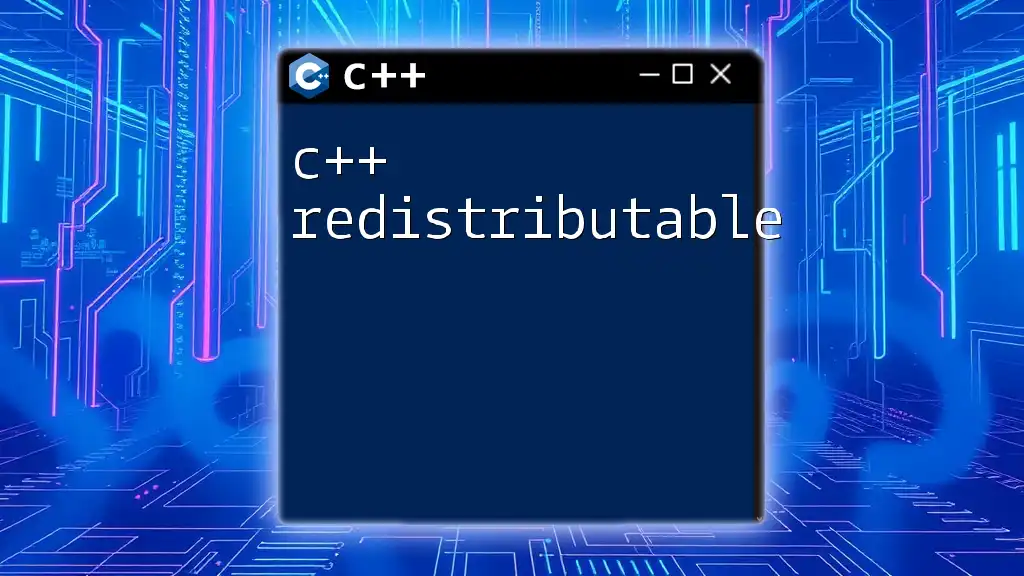
Practical Applications of GCD in C++
Simplifying Fractions
One of the most practical uses of GCD is in simplifying fractions. Whenever a fraction can be expressed in lower terms, it is typically done using the GCD. For example, when simplifying the fraction 40/60, the GCD is 20. Thus, the simplified fraction is 2/3.
Here’s a function to simplify a fraction using GCD:
#include <iostream>
#include <numeric>
void simplify_fraction(int& numerator, int& denominator) {
int divisor = std::gcd(numerator, denominator);
numerator /= divisor;
denominator /= divisor;
}
This function takes a reference to the numerator and denominator, computes the GCD, and divides both by it to yield the simplified fraction.
Solving Problems Using GCD
GCD finds numerous applications in programming challenges, including routing algorithms, resource allocation, and optimization problems. For instance, GCD can be employed to compute the least common multiple (LCM) of two numbers. As LCM is derived from GCD, programmers can use the following function for efficient calculation:
int lcm(int a, int b) {
return (a * b) / gcd(a, b);
}
This snippet effectively relates GCD to LCM, presenting an efficient and straightforward solution that many mathematical problems require.
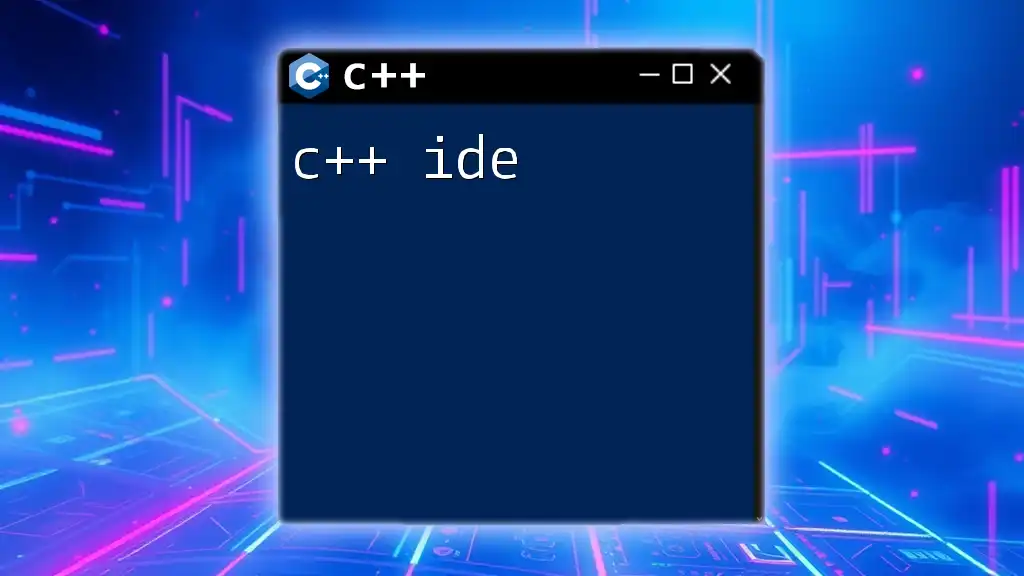
Conclusion
In summary, the C++ GCD is an essential mathematical tool that enhances both programming efficiency and understanding of number theory. From implementing basic algorithms to utilizing powerful standard library functions, mastering GCD expands a programmer's toolkit.
By mastering GCD and its applications, programmers can not only simplify problems but also optimize their solutions in a wide range of applications. Expanding your knowledge in this area will undoubtedly improve your coding skills and problem-solving abilities.