The acronym "Gaddis" often refers to the works of Tony Gaddis, a well-known author in computer programming education, and in the context of C++, it typically relates to structured learning and practical coding examples.
Here's a simple example of a C++ program that outputs "Hello, World!" to demonstrate basic syntax:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Introduction to C++ and Tony Gaddis
Overview of C++ Programming Language
C++ is one of the most widely used programming languages today. Renowned for its performance and flexibility, C++ serves multiple purposes across various domains, from systems programming to game development and large-scale applications. Its powerful features like Object-Oriented Programming (OOP), enhanced libraries, and low-level memory manipulation make it a preferred choice for developers looking to build robust applications.
Who is Tony Gaddis?
Tony Gaddis is a prominent author and educator in the field of computer programming. He has dedicated a significant part of his career to writing clear and accessible programming textbooks. His series, especially "Starting Out with C++," is highly regarded for its educational effectiveness, guiding beginners through the complexities of programming with ease and clarity. Gaddis's unique approach demystifies tough concepts, making learning C++ an achievable goal for anyone.
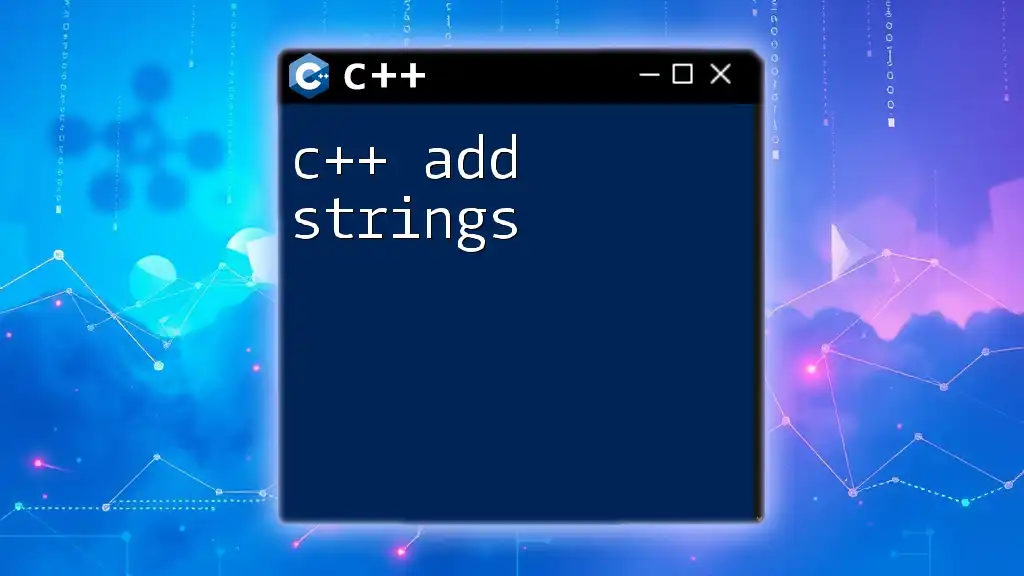
Starting Out with C++
Key Concepts from "Starting Out with C++: From Control Structures through Objects"
Tony Gaddis's book "Starting Out with C++: From Control Structures through Objects" serves as an excellent foundation for aspiring programmers. The text is structured progressively, introducing key concepts in a systematic manner, emphasizing control structures, and culminating with Object-Oriented Programming principles.
Understanding Basic Syntax and Structure
Learning the C++ syntax is critical for anyone starting out. A fundamental aspect of C++ is its straightforward syntax. Here’s a simple example of a program to print "Hello, World!" to illustrate:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this snippet, we include the necessary library and define the `main` function, which is the entry point of any C++ program.
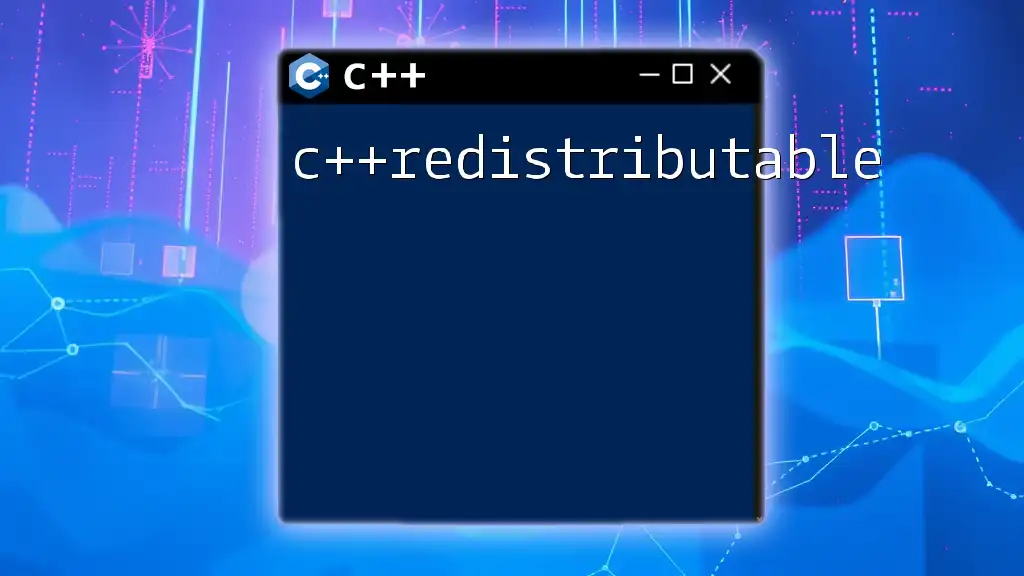
Control Structures in C++
The Importance of Control Structures
Control structures allow programmers to dictate the flow of logic in their code. They play a pivotal role in decision-making and looping, two essential programming tasks. Key control structures include conditional statements like `if`, `else if`, `else`, and looping mechanisms like `for`, `while`, and `do-while`.
Examples and Code Snippets
A basic use of an if statement could look like this:
int score;
cout << "Enter your score: ";
cin >> score;
if (score >= 60) {
cout << "You passed!" << endl;
} else {
cout << "You failed." << endl;
}
Looping with a `for` loop can be illustrated as follows:
for (int i = 0; i < 5; i++) {
cout << "This is loop iteration " << i << endl;
}
These examples demonstrate how control structures can facilitate decision-making and repetitive tasks in programming.
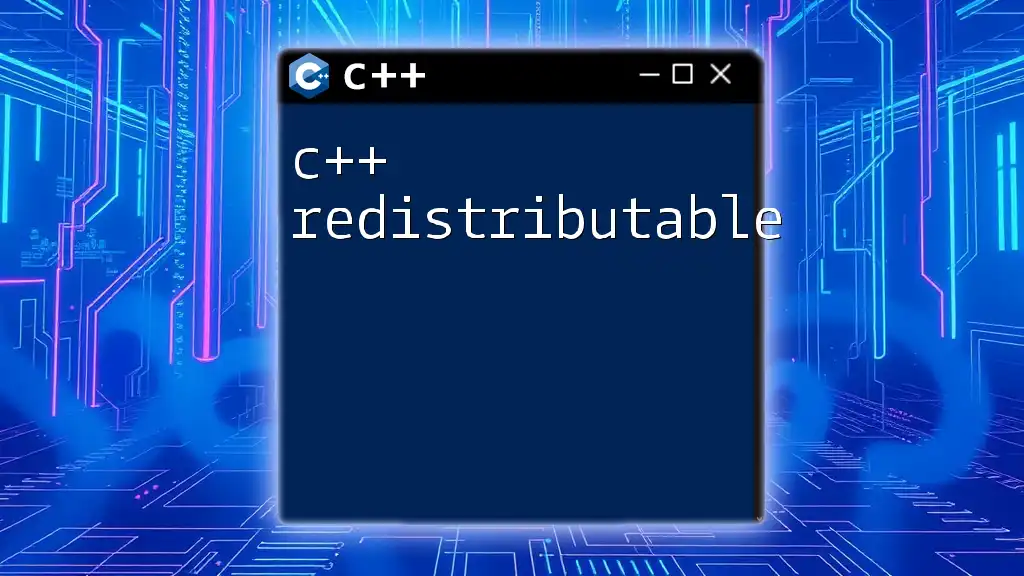
Functions in C++
Defining Functions and Their Importance
Functions are vital in C++ programming as they encapsulate specific tasks, allowing for reusable and organized code. By breaking code into functions, programmers can manage complexity more effectively and improve code clarity.
Examples of Functions
Here is a simple function to calculate the square of a number:
int square(int number) {
return number * number;
}
cout << "The square of 5 is: " << square(5) << endl;
In this code snippet, we define a function `square` that accepts an integer parameter and returns its square. Functions can also have multiple parameters and can return various data types.
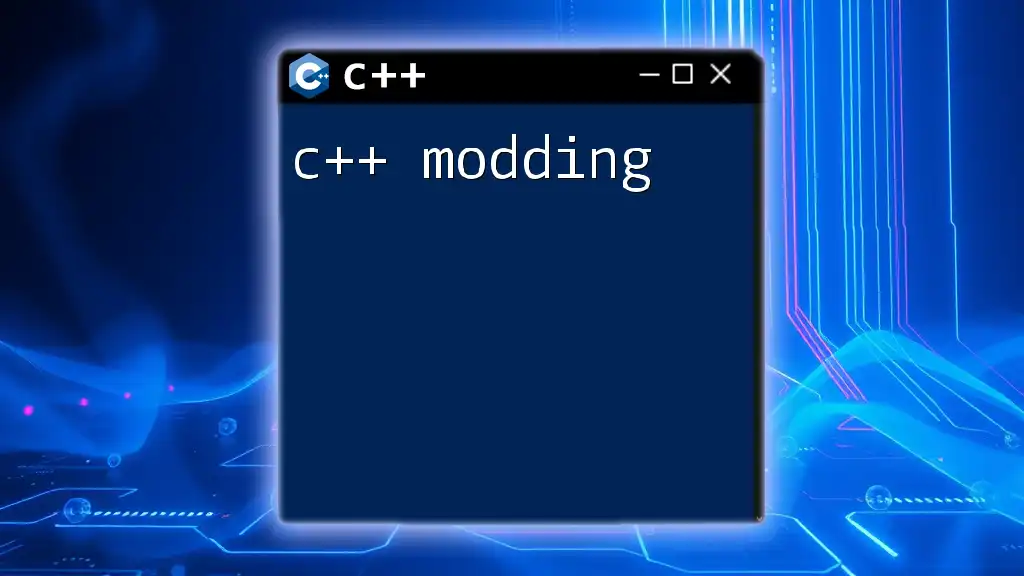
Object-Oriented Programming (OOP) in C++
Introduction to OOP Concepts
Object-Oriented Programming is a paradigm that enables developers to structure code in a more modular fashion. Key principles include encapsulation, inheritance, and polymorphism. Gaddis effectively integrates these principles into his teaching to help students grasp these essential programming concepts.
Classes and Objects
In C++, a class acts as a blueprint for creating objects. An example of creating a class for a simple bank account is as follows:
class BankAccount {
private:
double balance;
public:
BankAccount() : balance(0.0) {}
void deposit(double amount) {
balance += amount;
}
double getBalance() {
return balance;
}
};
Here, the `BankAccount` class encapsulates the account balance and provides methods to manipulate it. This structure enhances data safety and provides a clear interface for users of the class.
Inheritance and Polymorphism
Inheritance allows one class to inherit properties and behaviors from another, promoting code reuse. To demonstrate:
class SavingsAccount : public BankAccount {
private:
double interestRate;
public:
SavingsAccount(double initialRate) : interestRate(initialRate) {}
void applyInterest() {
deposit(getBalance() * interestRate);
}
};
In the example above, `SavingsAccount` inherits from `BankAccount` and adds functionality for applying interest, showcasing how inheritance enriches the base class’s functionality.
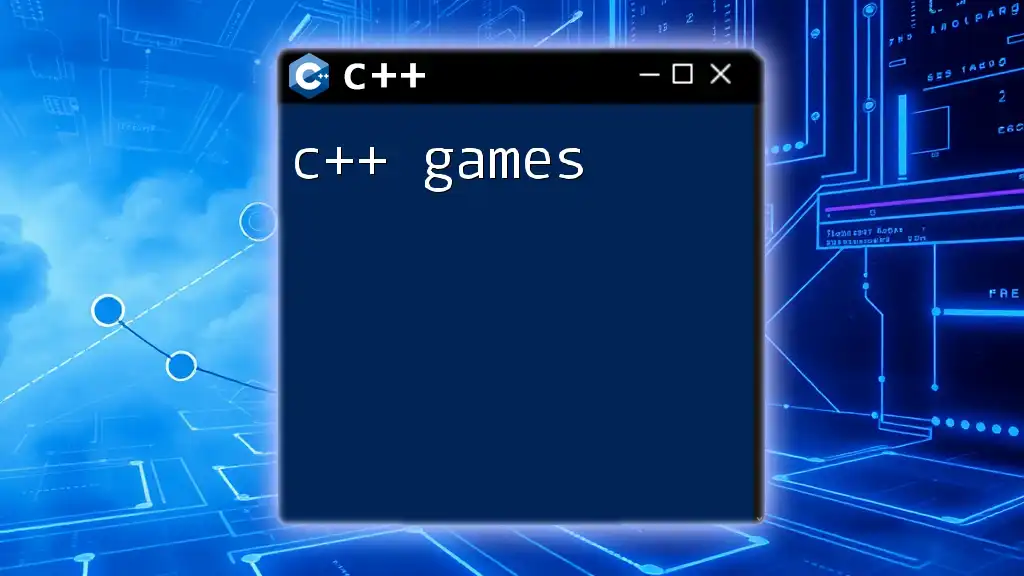
Advanced Topics Covered in Gaddis' C++
Templates and Standard Template Library (STL)
Templates are a powerful feature of C++. They enable the creation of generic and reusable code. Gaddis covers this topic thoroughly and introduces the Standard Template Library (STL). The STL contains a rich set of predefined classes and functions for common data structures and algorithms, significantly improving coding efficiency.
Exception Handling
Exception handling is another critical area for robust programming. It provides a way to respond to unexpected circumstances gracefully. Here's a simple example using try-catch:
try {
int a = 10, b = 0;
if (b == 0) {
throw "Divide by zero exception!";
}
cout << "Result: " << a / b << endl;
} catch (const char* msg) {
cerr << "Error: " << msg << endl;
}
In this case, the program throws an exception if there’s an attempt to divide by zero, promoting safe and predictable code execution.
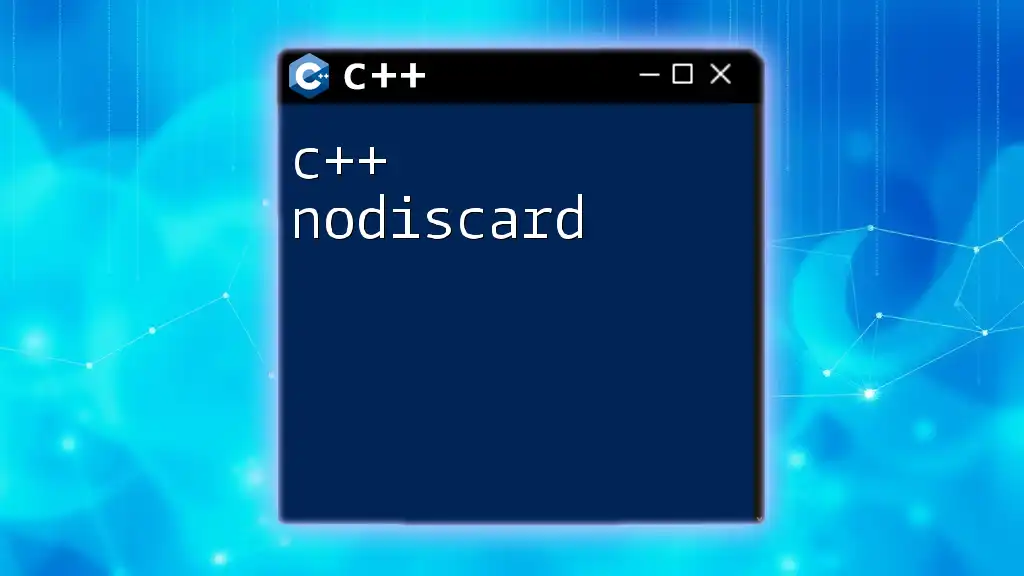
Additional Resources from Tony Gaddis
Online Resources and Tutorials
For those inspired to dive deeper into C++, Gaddis's website and affiliated educational platforms offer a variety of tutorials, practice exercises, and supplemental materials. Engaging with these resources can dramatically enhance your programming skills.
Connecting with the Programming Community
Participating in online forums and tech communities, such as Stack Overflow or Reddit's programming threads, is a recommended approach for both learning and networking. Sharing your experiences, asking questions, and contributing can lead to valuable insights and new opportunities.
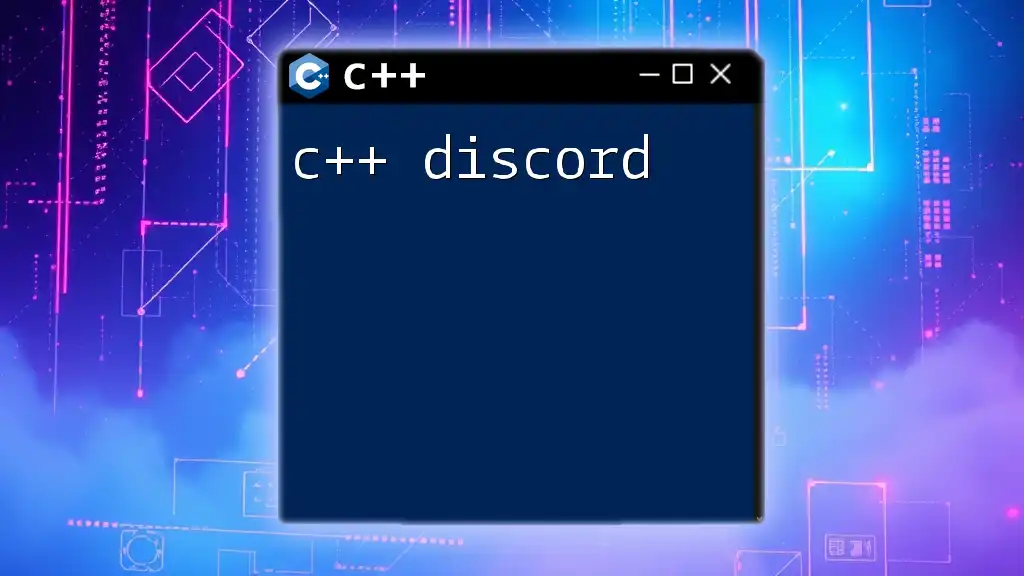
Conclusion
Recap of Key Points
This guide has walked you through vital aspects of C++ programming under the tutelage of Tony Gaddis. From control structures to the foundations of Object-Oriented Programming, each section has laid out essential skills and concepts critical for effective coding.
Encouragement to Learn and Practice
The journey to mastering C++ is paved with practice and continual learning. As you explore coding challenges and work on projects, remember that consistent engagement with programming will catalyze your growth as a developer.
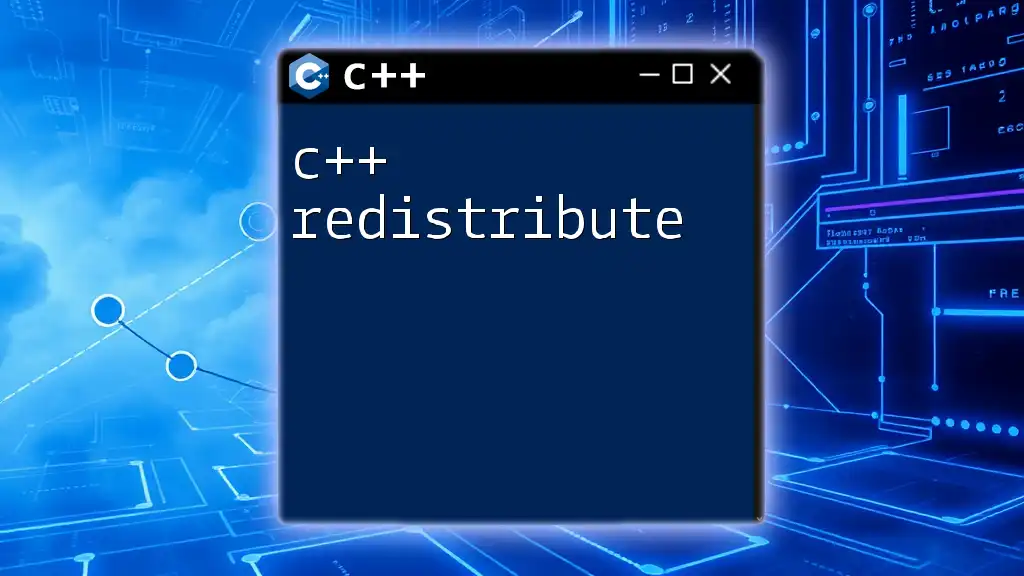
Frequently Asked Questions (FAQs)
Common Challenges When Learning C++
It's not uncommon to face hurdles while learning C++. Struggling with complex syntax or debugging is part of the process and should be viewed as an opportunity for growth.
Best Practices for Learning C++
Approach your learning systematically. Break down complex topics into smaller sections and practice regularly to reinforce new concepts.
The Future of C++ Programming
With its continual evolution, C++ remains relevant and powerful. Engaging with the language today prepares you for exciting opportunities in the future of technology. Embrace C++ and unlock your potential in programming!