In C++, a pointer to an incomplete class type is not allowed because the compiler needs to know the size and layout of the class for memory management, as demonstrated in the following example:
class Incomplete; // Forward declaration
class Holder {
public:
Incomplete* ptr; // Error: pointer to incomplete type
};
class Incomplete {
int data;
};
What Is an Incomplete Class Type?
Definition of Incomplete Types
In C++, an incomplete type is a type that has been declared but not fully defined. A class type becomes incomplete when you declare it without providing its full implementation. For example:
class MyClass; // Forward declaration
At this point, `MyClass` is considered incomplete because the compiler does not know its members or methods yet. This is commonly used to avoid circular dependencies between classes.
Why Are Incomplete Types Used?
Incomplete types are primarily used to solve the problem of circular references between classes. They allow you to declare class types without needing the entire structure immediately available. This can help in keeping code modular and organized, especially in scenarios with complex class interactions.
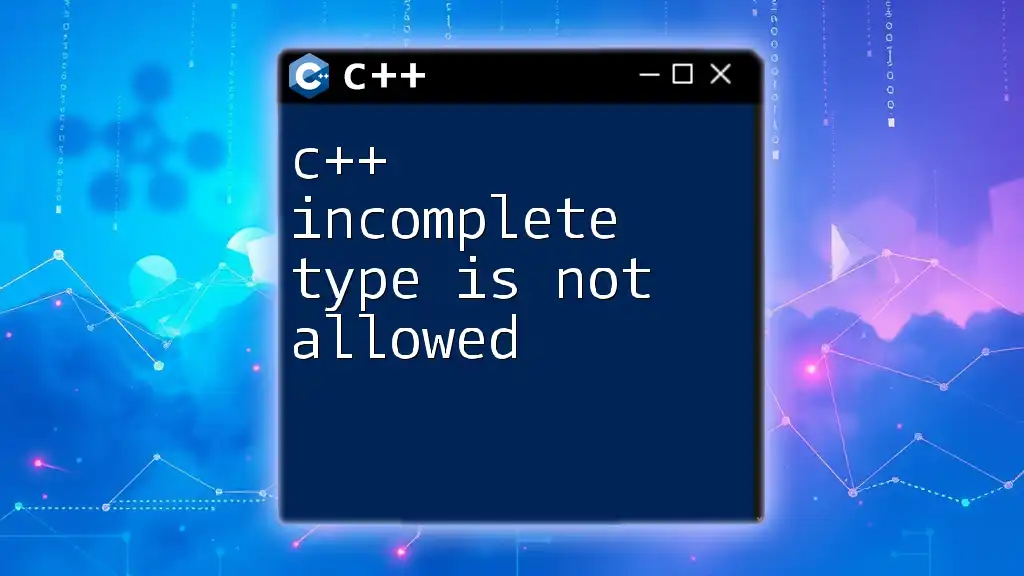
Pointers in C++
What Are Pointers?
A pointer is a variable that stores the address of another variable. They are a vital feature in C++ as they allow dynamic memory management and enable the creation of complex data structures like linked lists and trees.
How Pointers Work with Classes
When using pointers with classes, you can create dynamic instances of classes. For instance, to create a pointer to a class type defined as:
class MyClass {
public:
void display() {
// Method implementation
}
};
MyClass* ptr = new MyClass(); // Pointer to MyClass instance
Here, `ptr` holds the address of a newly created instance of `MyClass`. This is fundamental to using classes efficiently in C++.
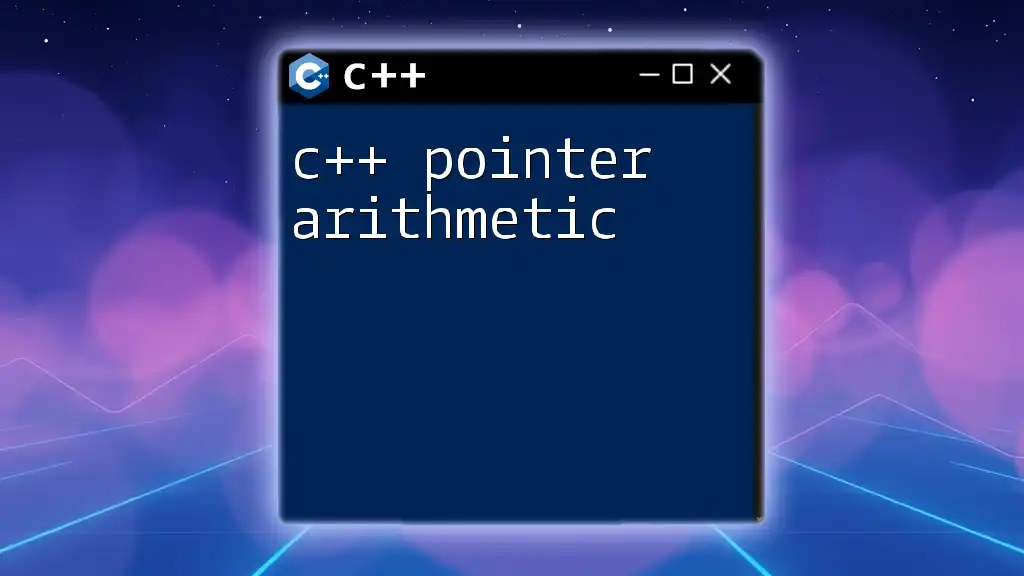
The Rule: Pointers to Incomplete Class Types Are Not Allowed
Explanation of the Compiler Error
When you attempt to use a pointer to an incomplete class type, you will encounter a compiler error. This occurs because the compiler cannot determine the size or layout of the class since it has not yet been fully defined.
For example:
class IncompleteClass; // Forward declaration
void func(IncompleteClass* obj) { // Compiler error occurs here
// Attempting to use the pointer before class definition
}
The message typically states: "pointer to incomplete class type is not allowed."
Why This Rule Exists
This rule is in place to ensure type safety. The compiler must know the complete structure of a class to manage memory correctly and ensure that operations on the class pointers are safe. Attempting to manipulate an incomplete type can lead to undefined behavior, which is a critical concern in C++ programming.
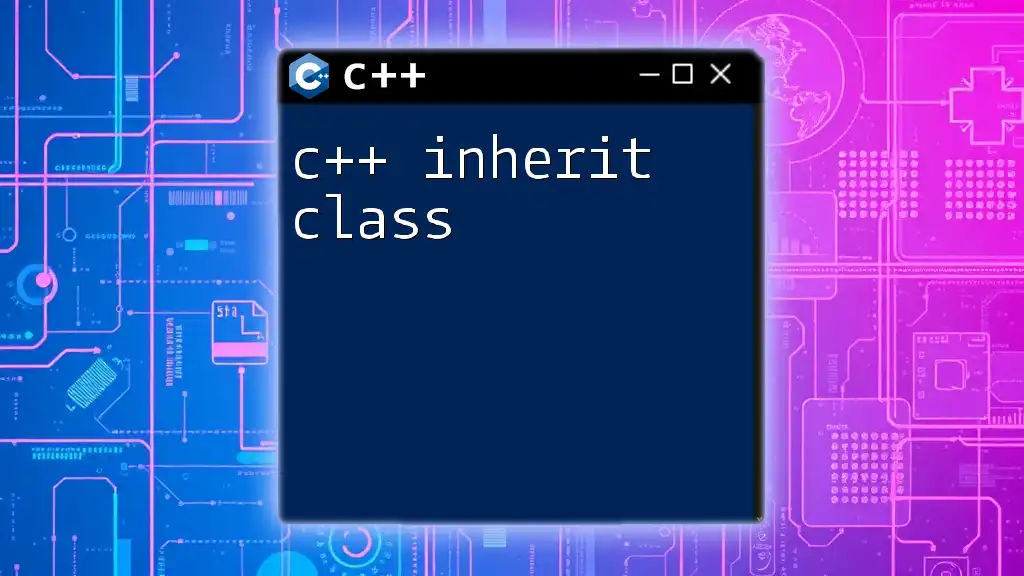
Working with Incomplete Classes and Pointers
Correct Usage of Pointers with Incomplete Types
While the use of pointers to incomplete classes generates errors, you can still structure your code correctly to avoid these issues. Here's an example of proper forward declaration and using pointers:
class IncompleteClass; // Forward declaration of IncompleteClass
class CompleteClass {
public:
void useIncomplete(IncompleteClass* obj);
};
class IncompleteClass {
// Complete implementation
};
By ensuring that the pointer is being used within the scope of the complete definition, you circumvent the error.
Strategies to Avoid Incomplete Type Errors
Use of Forward Declarations
Utilizing forward declarations carefully is essential. You must ensure that any functions or methods that interact with the incomplete classes are defined appropriately later in the code where the complete definition exists.
class IncompleteClass; // Forward declaration
void processClass(IncompleteClass* obj); // Valid forward declaration
class IncompleteClass {
// Implementation goes here
};
Proper Class Structure
The organization of class definitions in your program plays a crucial role as well. Properly structuring classes can prevent incomplete types. It is best practice to define classes before creating pointers to them.
Complete Class Definition
Once you define a class completely, you can safely use pointers to its instances. Here's how:
class Complete {
public:
void display() {
// Implementation of the method
}
};
void func() {
Complete* ptr = new Complete(); // Valid, as Complete is fully defined
ptr->display(); // Safe to call method
}
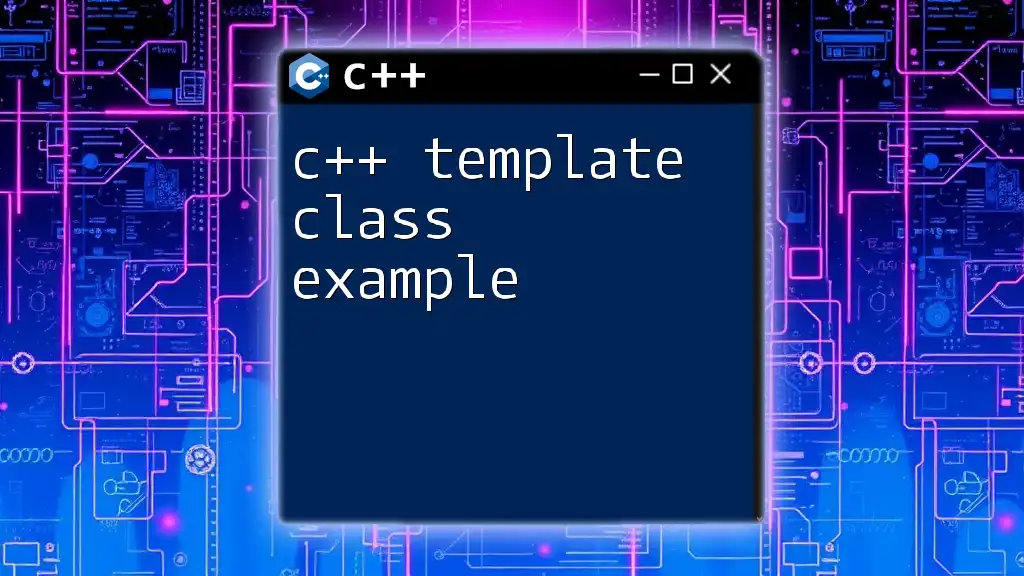
Common Mistakes and How to Avoid Them
Mistake 1: Misunderstanding Forward Declarations
New programmers often misinterpret how forward declarations work. Remember: a forward declaration does not allow the use of pointers as if the class is fully defined. Ensure you ever interact with a pointer to an incomplete type only when its definition is fully available.
Mistake 2: Using Pointers Before Definition
One of the most common pitfalls is attempting to use a pointer before the class is fully defined. In the following code, an error will occur:
class IncompleteClass; // Forward declaration
IncompleteClass* ptr = new IncompleteClass(); // Error: incomplete type
Tips for Debugging
To debug pointer-related issues effectively, ensure that:
- You always verify that complete class definitions are available when using their pointers.
- You meticulously check your forward declarations.
Ensure you're not trying to create instances of incomplete types, which can save time in the long run.
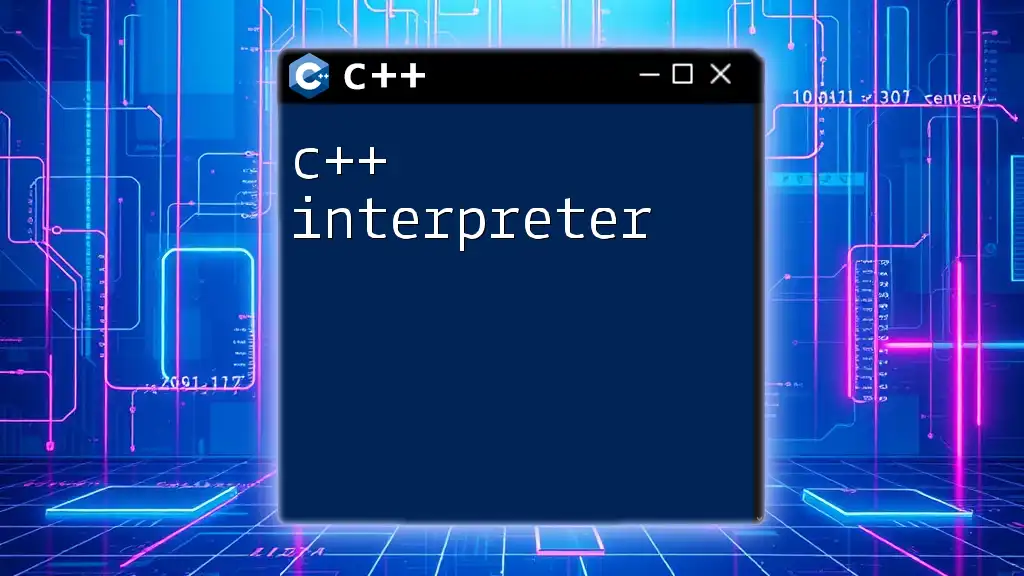
Additional Resources
Recommended Reading
For those looking to further their understanding of C++, several resources can help enhance knowledge:
- "The C++ Programming Language" by Bjarne Stroustrup
- Online tutorials and forums such as Cplusplus.com, GeeksforGeeks, and Stack Overflow
Community and Support
Joining communities centered around C++ can also provide valuable support. Platforms like Reddit, Stack Overflow, or dedicated programming forums can be great places to ask questions and share experiences.
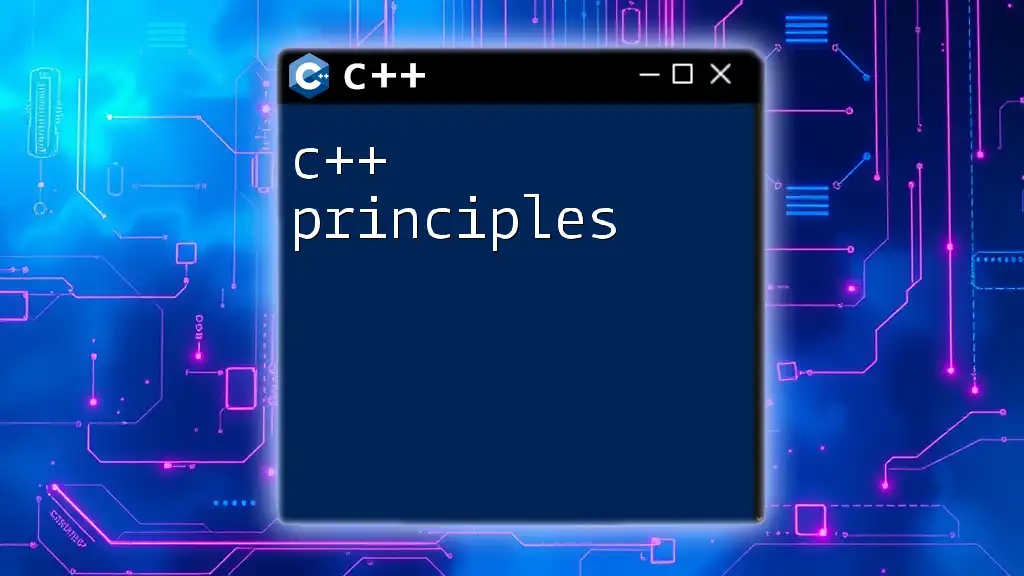
Conclusion
Understanding why "C++ pointer to incomplete class type is not allowed" is crucial for any C++ developer. It leads to better memory management, clearer code structure, and overall safer programming practices.
By reinforcing your grasp on pointers and incomplete types, you position yourself for better programming efficiency. Engage actively with the community and continue learning, as C++ provides a vast landscape filled with intricacies to explore.