The Sieve of Eratosthenes is an efficient algorithm to find all prime numbers up to a specified integer by iteratively marking the multiples of each prime starting from 2.
Here’s a simple implementation in C++:
#include <iostream>
#include <vector>
void SieveOfEratosthenes(int n) {
std::vector<bool> primes(n + 1, true);
primes[0] = primes[1] = false; // 0 and 1 are not prime numbers
for (int p = 2; p * p <= n; p++) {
if (primes[p]) {
for (int i = p * p; i <= n; i += p)
primes[i] = false;
}
}
for (int p = 2; p <= n; p++) {
if (primes[p])
std::cout << p << " ";
}
}
int main() {
int n = 30; // Example: Find all primes up to 30
SieveOfEratosthenes(n);
return 0;
}
Understanding Prime Numbers
Definition of Prime Numbers
A prime number is defined as a natural number greater than 1 that cannot be formed by multiplying two smaller natural numbers. This means that a prime number is only divisible by 1 and itself. For example, 2, 3, 5, 7, and 11 are prime numbers, while 4, 6, and 8 are not.
Importance of Prime Numbers in Computing
Prime numbers play a crucial role in various computing fields, particularly in cryptography. Algorithms that secure data transmission often employ prime numbers to generate keys, making them essential for maintaining privacy and security in digital communication. Additionally, prime numbers are significant in creating hash functions, which efficiently store and retrieve data.
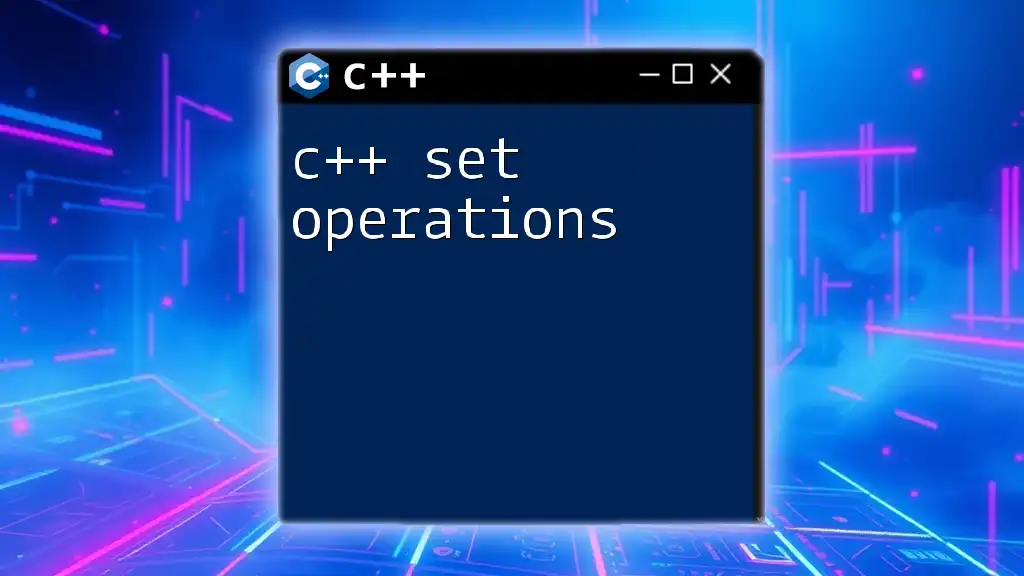
The Basics of the Sieve of Eratosthenes
How the Sieve Works
The Sieve of Eratosthenes is an ancient algorithm designed to generate a list of all prime numbers up to a specified integer, n. By systematically marking the multiples of each prime starting from 2, the algorithm effectively filters out non-prime numbers. The end result is a concise list of prime numbers, and this method is known for its efficiency.
Advantages of Using the Sieve
One of the standout features of the Sieve of Eratosthenes is its time complexity, which operates at O(n log log n). Comparing this with other prime-checking methods like trial division, the sieve is significantly faster for larger values of n. In terms of space complexity, it utilizes an array of boolean values to keep track of prime numbers, making it relatively memory-efficient as well.
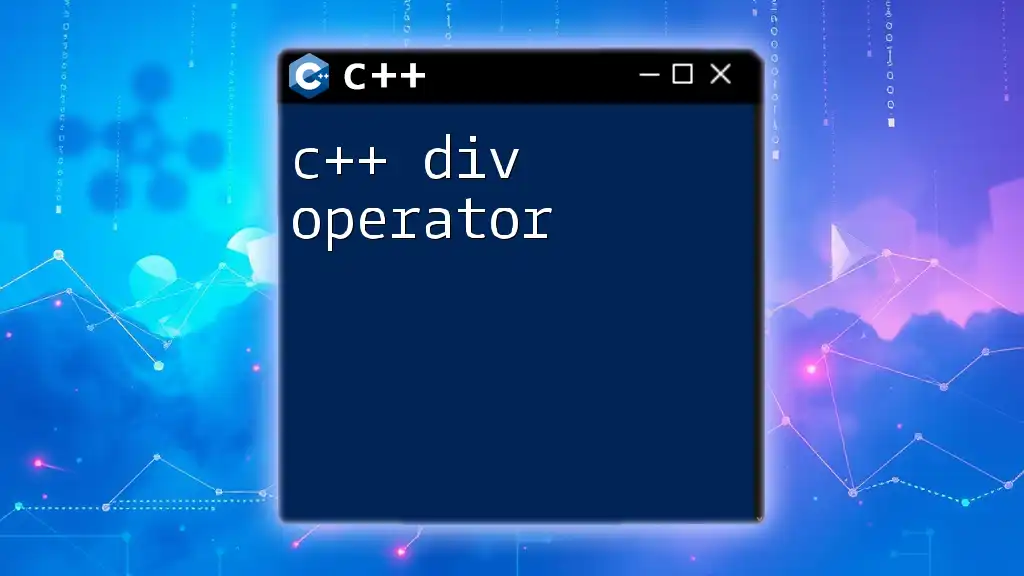
Implementing the Sieve of Eratosthenes in C++
Setting Up the Environment
Before diving into the code, having the right coding environment is essential. Ensure you have a C++ compiler set up, such as g++ or Visual Studio, and familiarize yourself with using basic libraries standard for C++ programming, particularly for console input and output.
Writing the Algorithm: An Example
To implement the Sieve of Eratosthenes in C++, follow this basic structure:
#include <iostream>
#include <vector>
void sieveOfEratosthenes(int n) {
std::vector<bool> prime(n + 1, true);
prime[0] = prime[1] = false;
for (int p = 2; p * p <= n; p++) {
if (prime[p]) {
for (int i = p * p; i <= n; i += p) {
prime[i] = false;
}
}
}
// Print all prime numbers
for (int p = 2; p <= n; p++) {
if (prime[p]) {
std::cout << p << " ";
}
}
}
Explanation of Code
The code initializes a boolean vector named `prime` with all entries set to `true`, indicating that all numbers are initially considered prime. The entries for `0` and `1` are set to `false` since they are not prime. The outer loop starts from `2` and marks multiples of each found prime (starting from its square) as `false`. Finally, it prints all remaining entries marked as `true` in the vector, signifying the prime numbers.
Customizing the Implementation
Finding Primes in a Specific Range
The problem can be extended to find primes within a particular range, allowing for more versatile applications of the algorithm.
#include <iostream>
#include <vector>
void sieveInRange(int low, int high) {
std::vector<bool> prime(high + 1, true);
prime[0] = prime[1] = false;
for (int p = 2; p * p <= high; p++) {
if (prime[p]) {
for (int i = std::max(p * p, (low + p - 1) / p * p); i <= high; i += p) {
prime[i] = false;
}
}
}
// Print all prime numbers in the range
for (int p = low; p <= high; p++) {
if (prime[p]) {
std::cout << p << " ";
}
}
}
Explanation of Customization
In this code snippet, the function `sieveInRange` accepts two parameters, `low` and `high`, representing the range of numbers in which to search for primes. Using the same logic as the base algorithm, we modify the inner loop to use `std::max` for more precise targeting, ensuring we don’t unnecessarily mark primes in lower ranges.
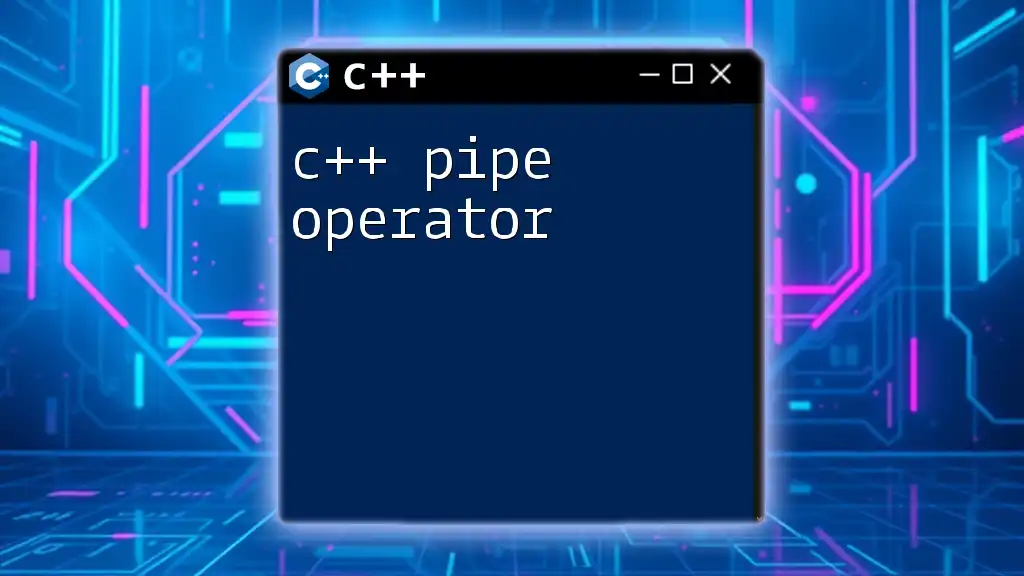
Performance Considerations
Optimizing the Sieve of Eratosthenes in C++
Memory Efficiency Techniques
One way to enhance memory efficiency while using the sieve is to implement bitwise representation rather than a standard boolean vector. By using bits instead of bytes for each number, we can significantly reduce the overall memory consumption.
Multithreading and Parallel Execution
For applications requiring extremely high efficiency, especially when generating large lists of primes, a parallel version of the sieve can be considered. This implementation divides the task among multiple threads, which can process even larger values of n in a fraction of the time. However, this requires careful management of data, as concurrent writes to shared resources can lead to data corruption.
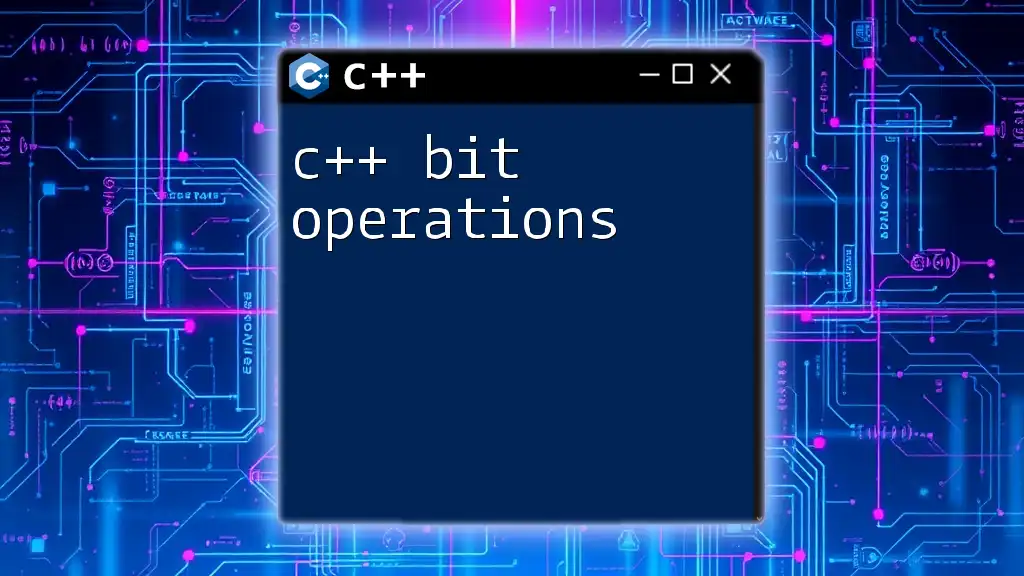
Visualizing the Sieve
Concept of Visualization
Understanding the Sieve of Eratosthenes can be greatly enhanced through visualization. By representing the sieve’s process graphically, learners can gain a clearer understanding of how primes are discovered and how the algorithm operates over time.
Example of a Visual Representation
Using libraries like matplotlib-cpp, you can create visual representations of the algorithm in action, which demonstrates prime number generation step-by-step. By integrating these visual aids, you foster a more engaging learning experience and make concepts easier to grasp.
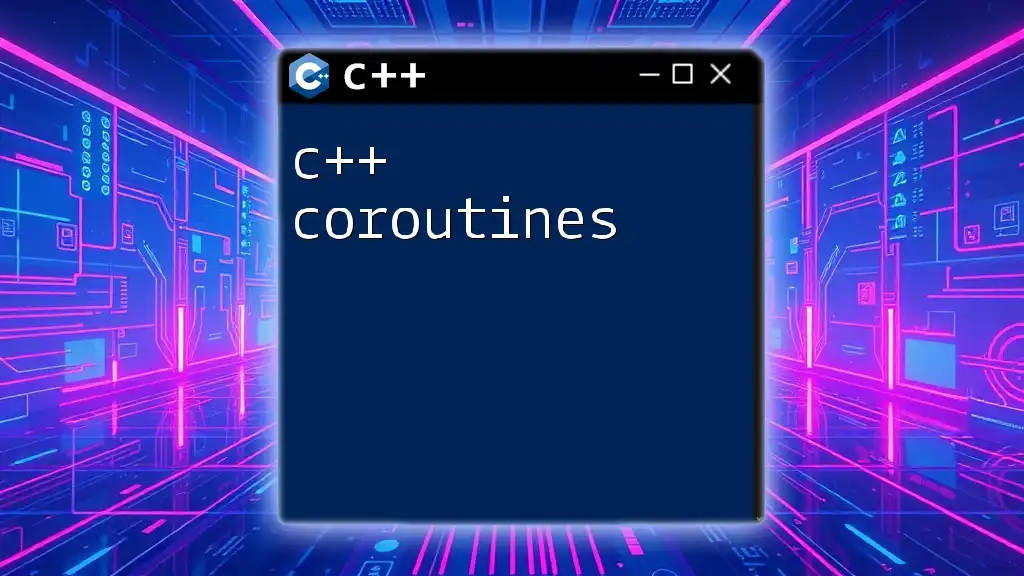
Common Mistakes to Avoid
Errors in Implementation
When coding the Sieve of Eratosthenes, beginners can easily fall victim to common errors, such as incorrectly initializing the boolean vector or miscalculating loop boundaries. Always verify the initializations and consider edge cases, particularly when n is small.
Misunderstanding the Algorithm
Many might misconceive that the Sieve of Eratosthenes can efficiently generate primes for any value of n, irrespective of optimization techniques. It’s crucial to understand the limitations and performance implications, particularly related to memory usage for large values.
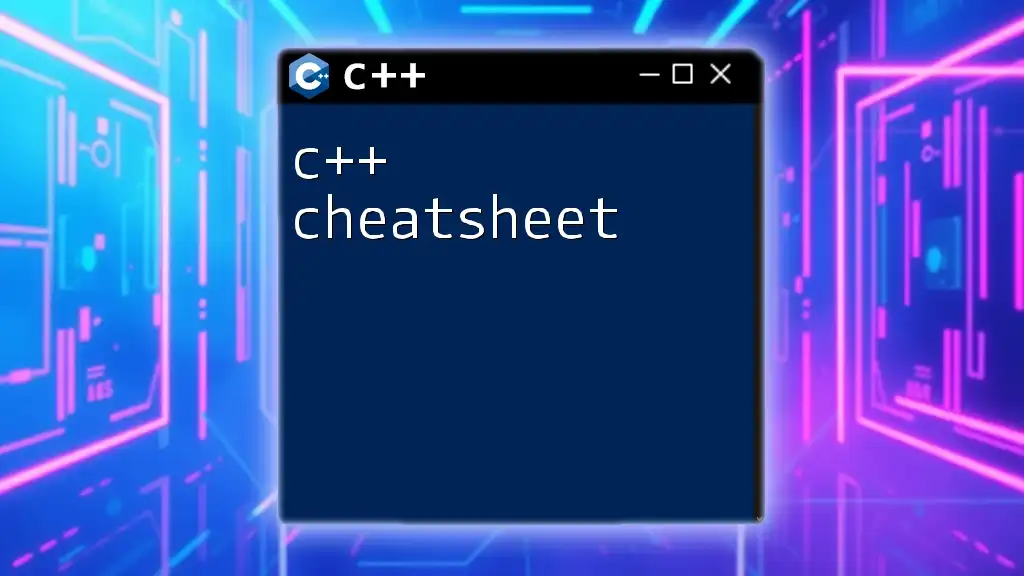
Conclusion
The C++ Sieve of Eratosthenes is not only a powerful algorithm for generating primes but also serves as an excellent educational tool for learning efficient coding practices and algorithmic thinking. Through mastering this algorithm, both new and experienced programmers can enhance their skills in a fundamental area of computer science.
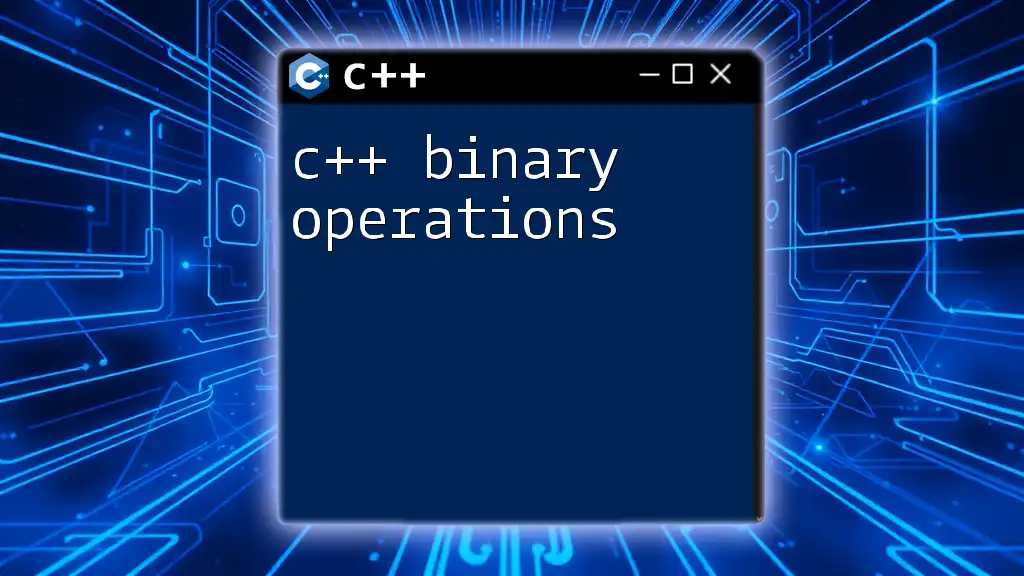
Further Learning Resources
For those eager to dive deeper into understanding prime numbers or the Sieve of Eratosthenes, several resources can significantly aid your learning journey. Books focused on algorithms, online courses, and coding platforms such as LeetCode or HackerRank offer practical exercises and forums for discussion.
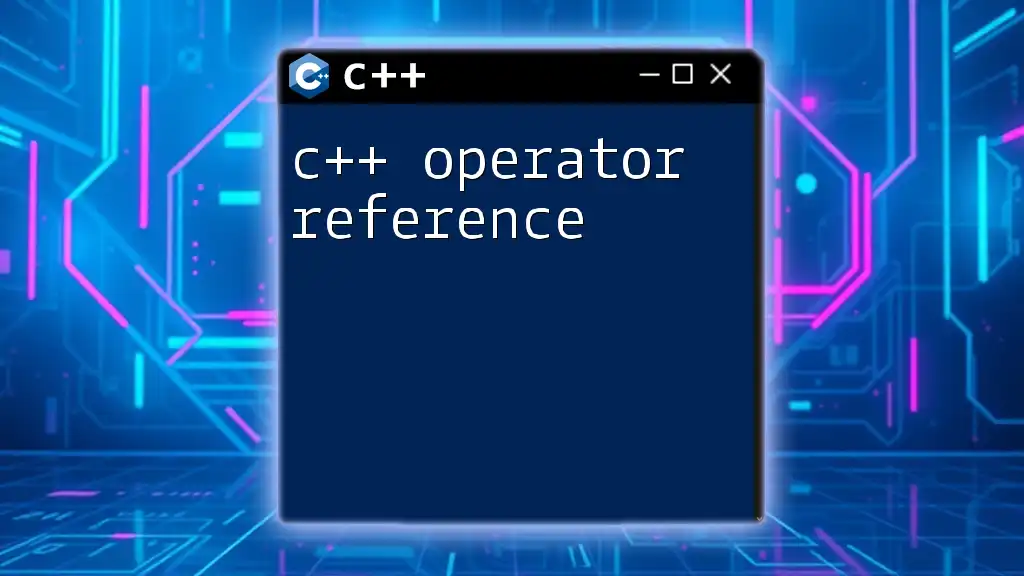
Call to Action
I encourage you to start experimenting with the Sieve of Eratosthenes in C++! Implement the algorithm, modify it, and explore its various extensions. Feel free to share your results, improvements, and experiences on social media or within coding communities. Happy coding!