Bjarne Stroustrup's book on C++ serves as a foundational resource for understanding the principles and features of the C++ programming language, blending theoretical insights with practical examples.
Here's a code snippet showcasing a simple "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Overview of the Book
C++ Book by Bjarne Stroustrup
Bjarne Stroustrup’s C++ book has a rich history, being one of the first comprehensive resources dedicated to C++. With numerous updates since its initial release, it remains a significant text for both aspiring programmers and seasoned developers.
The book’s main objective is to provide a thorough understanding of C++ as a powerful programming language. It is tailored for a wide range of audiences—from beginners to experienced programmers—looking to deepen their understanding of C++.
In today’s programming landscape, where C++ is heavily utilized in systems programming, game development, and application software, learning from an authoritative source like Stroustrup is vital for success.
The C++ Programming Language, Fourth Edition
The fourth edition of The C++ Programming Language incorporates substantial updates that reflect changes in the language itself. It brings clarity to the Standard Template Library (STL), modern C++ features like lambda functions, and enhanced performance considerations.
One key difference lies in how the material is presented; compared to previous editions, this version emphasizes writing efficient, reusable code and better error handling. It serves not only as a guide to language features but also as a reference for best practices, making it an essential read for anyone serious about C++ programming.
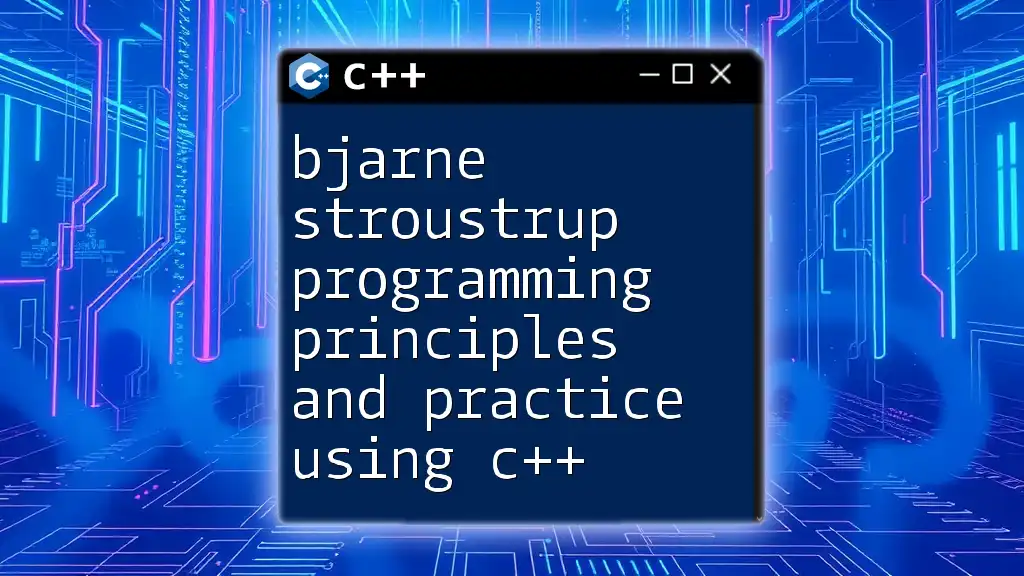
Key Features of the Book
Comprehensive Coverage of C++ Language
The book excels at providing in-depth coverage of the C++ programming language. Each concept is explained thoroughly, enabling readers to grasp core principles without prior experience. For instance, you’ll start from fundamental concepts such as:
#include <iostream>
using namespace std;
int main() {
int number = 5;
cout << "Hello, world! Number: " << number << endl;
return 0;
}
This segment introduces variables, output, and the structure of a basic C++ program.
Real-World Applications
Stroustrup enhances the learning experience by relating C++ concepts to real-world applications. He illustrates how the principles learned can solve actual programming challenges. For instance, creating a simple game engine might use classes and inheritance, central themes in object-oriented programming:
class Player {
public:
void move(int x, int y) {
// Move player to a new position
}
};
This shows how C++ supports organizing code in a manageable way, mirroring real-world entities.
Clear and Concise Explanations
Stroustrup’s writing style is particularly notable for its clarity. He breaks down complex concepts into understandable parts. For example, when discussing pointers, he writes:
int x = 10;
int* p = &x; // p now holds the address of x
This direct approach allows even novice programmers to understand pointers, which are crucial for efficient memory management in C++.
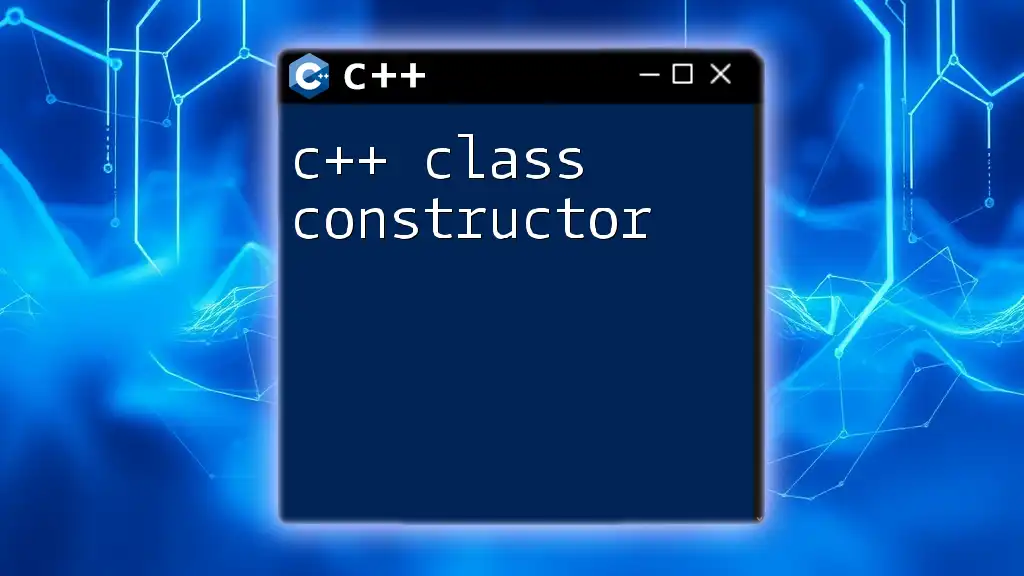
Learning Approach in the Book
Structured Learning Path
The organization of the book is designed with a logical progression in mind—starting from the basics and moving towards advanced topics. This structure enables learners to build upon their knowledge systematically.
Key sections are purposefully designed to reinforce previously learned material, ensuring that foundational topics effectively support more complex concepts.
Code Examples and Exercises
Each chapter is filled with hands-on exercises that encourage readers to put theory into practice. These exercises are essential for solidifying understanding. For example:
Exercise: Write a function that calculates the factorial of a number.
Solution:
int factorial(int n) {
return (n <= 1) ? 1 : n * factorial(n - 1);
}
This exercise not only reinforces function usage but also introduces recursion—an important programming paradigm in C++.
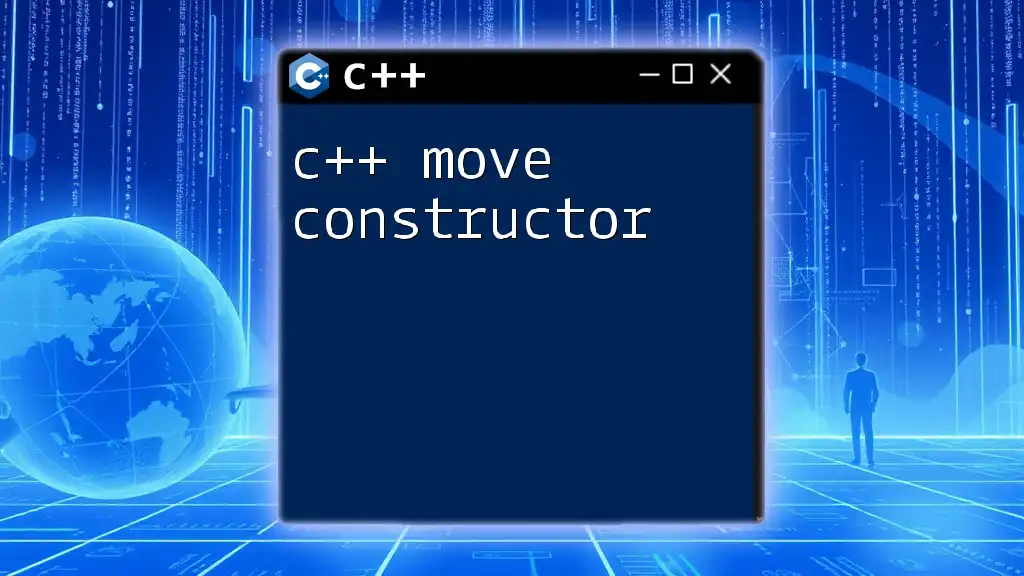
Who Should Read This Book?
Beginners
For beginners, Stroustrup's book serves as a welcoming introduction to C++. It covers the essentials of programming, making it suitable for those unfamiliar with coding. Key sections that cater to novices include data types, control structures, and the basics of functions.
Experienced Programmers
Even seasoned developers will find value in the depth of insights shared in the book. The coverage of advanced topics, such as templates and multi-threading, enriches the knowledge base of experienced programmers. The exploration of the STL, for instance, demonstrates efficient ways to store and manage data.
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {5, 3, 8, 1};
std::sort(numbers.begin(), numbers.end());
return 0;
}
This snippet showcases utilizing libraries for efficiency—an essential practice for proficient developers.
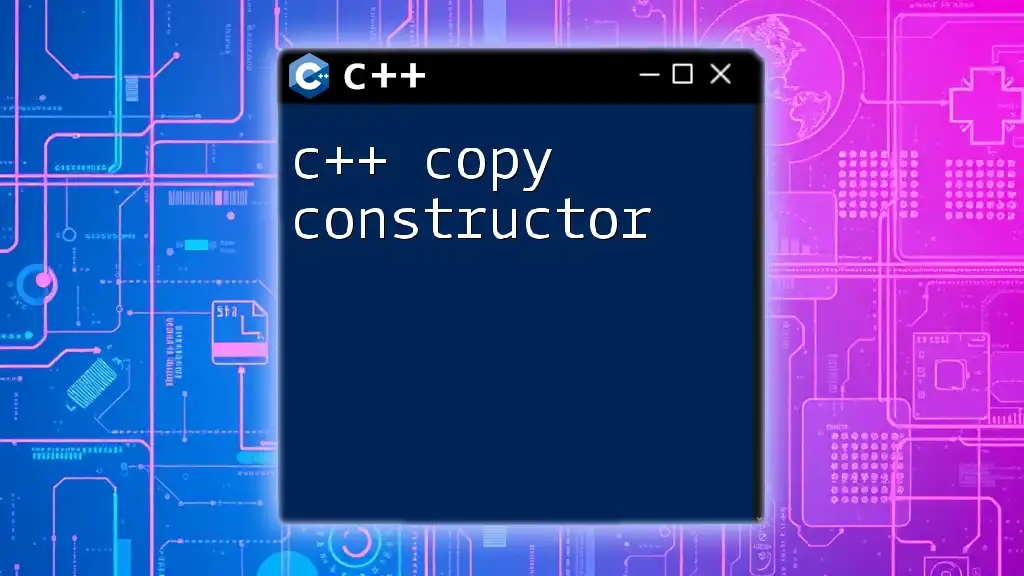
Practical Tips for Reading the Book
Setting Up a Study Schedule
To effectively digest the material, consider setting up a study schedule that allows consistency without overwhelming yourself. Aim for a few chapters each week, giving time to practice the included exercises.
Using Supplementary Resources
While the book is comprehensive, using supplementary resources can enhance your understanding. Websites like Stack Overflow and forums such as the C++ subreddit provide valuable insights and community support. Video tutorials on platforms like YouTube can also illustrate complex concepts effectively.
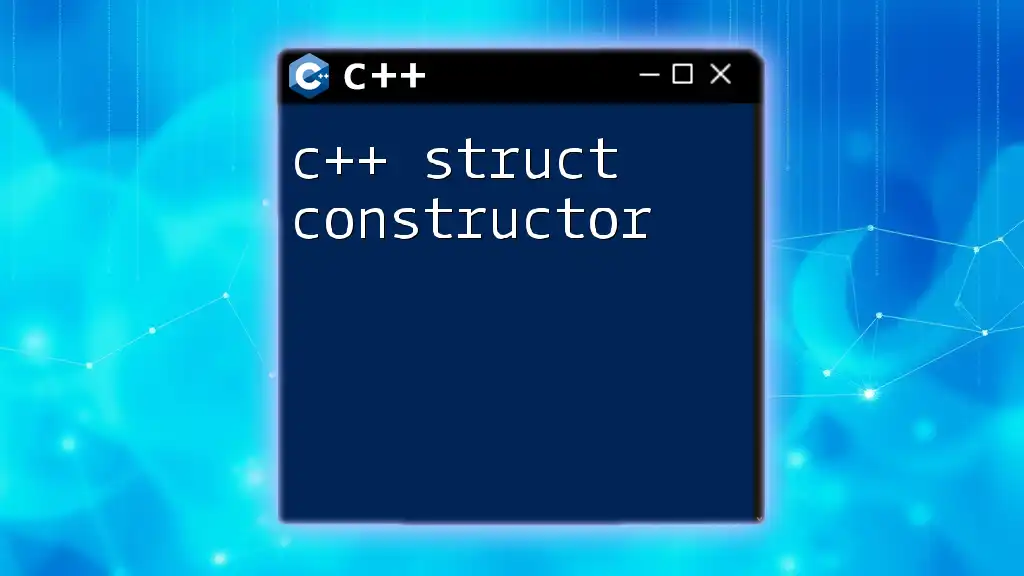
Conclusion
Bjarne Stroustrup's C++ book is a critical resource for anyone looking to master C++. Its structured approach, real-world applications, and practical examples offer unmatched value. Embrace this resource not just as a reading material but as a foundation for your programming journey. Regularly practicing coding will be the key to advancing your skills.
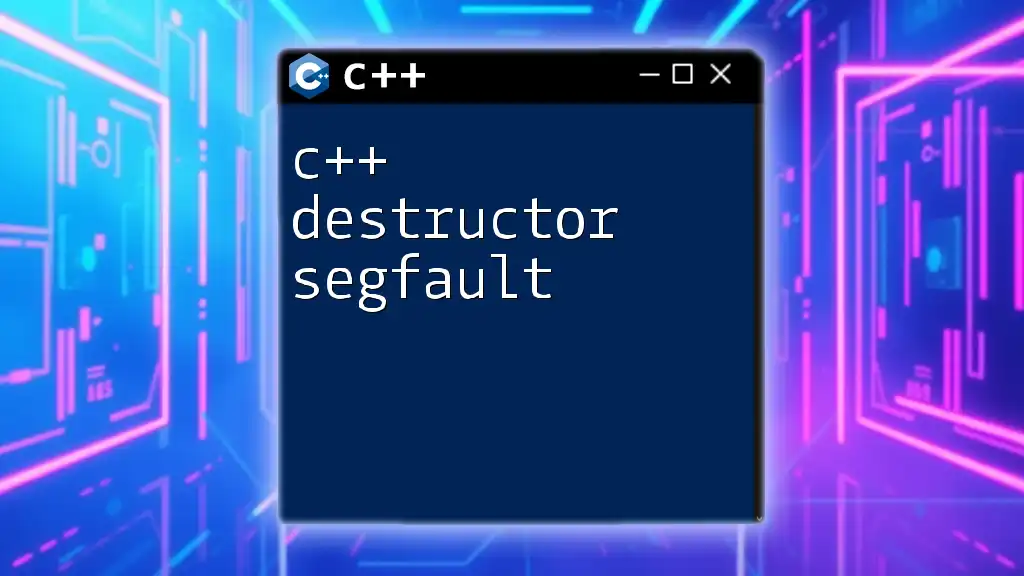
FAQs about Bjarne Stroustrup's Book
What is unique about this C++ book?
What sets this book apart from other C++ programming books is its authoritative perspective, given that Bjarne Stroustrup is the creator of the language. The thoroughness and clarity with which concepts are explained is also unmatched.
Is the book suitable for self-study?
Absolutely. The book is designed with self-learners in mind, featuring clear explanations and exercises that encourage practice. With dedication, learners can navigate through it independently.
How often is the book updated?
The book is updated periodically, reflecting changes in the C++ standard. Each edition aims to incorporate modern practices and techniques that are evolving in the programming community.
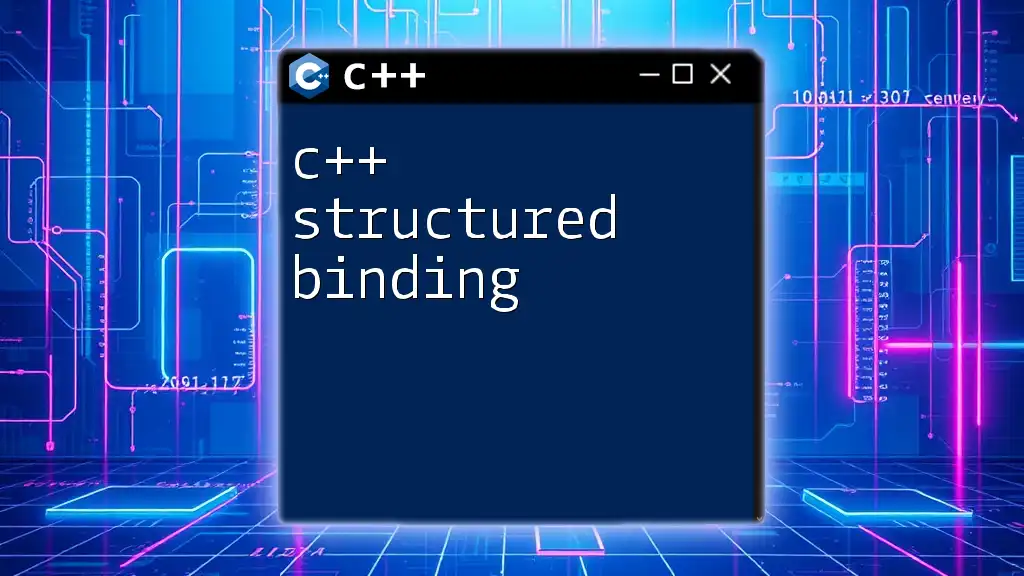
Call to Action
Join Our Learning Community
To further enhance your learning experience, consider enrolling in our concise C++ course designed to deliver essential programming skills quickly. You'll gain access to community support and invaluable resources to guide you along your programming journey.
Purchase Information
You can purchase The C++ Programming Language from various platforms like Amazon or local bookstores. Choosing the right edition based on your skill level will greatly benefit your learning process.